如何最佳化Go語言開發中的網路連線池管理
摘要:網路連線池是大部分後端開發中常用到的技術。在Go語言中,我們可以利用goroutine和channel等特性來實現高效率的連結池管理。本文將介紹如何利用Go語言開發中的一些最佳化技巧來最大程度提升網路連接池的效能和穩定性。
關鍵字:Go語言、網路連線池、最佳化、效能、穩定性
一、引言
網路連線池是一種常見的技術,它用於重複使用和管理網路連線。在大部分後端開發中,我們需要經常與資料庫、快取伺服器或其他服務進行通信,而每次建立和關閉連接都會帶來一定的開銷。透過使用連接池,我們可以在需要時從池中獲取一個可用連接,而無需每次都重新建立連接,從而提升效率和效能。
二、Go語言中的連結池
在Go語言中,我們可以透過goroutine和channel的特性來實現高效率的連結池管理。我們可以使用一個channel來儲存連接,透過在channel上進行發送和接收操作來實現連接的獲取和釋放。
- 連接池的結構定義
首先,我們需要定義一個連接池的結構體,如下所示:
type ConnectionPool struct { pool chan net.Conn }
- 連接池的初始化
接下來,我們需要對連線池進行初始化。在初始化過程中,我們可以建立一定數量的連接,並將它們儲存到channel中。
func NewConnectionPool(size int, factory func() (net.Conn, error)) (*ConnectionPool, error) { pool := make(chan net.Conn, size) for i := 0; i < size; i++ { conn, err := factory() if err != nil { return nil, errors.New("failed to create connection") } pool <- conn } return &ConnectionPool{pool: pool}, nil }
- 連接的獲取和釋放
在實際應用中,我們可以透過呼叫GetConn()函數來從連接池中取得一個可用連接,透過呼叫ReleaseConn()函數來釋放連線。
func (p *ConnectionPool) GetConn() net.Conn { return <-p.pool } func (p *ConnectionPool) ReleaseConn(conn net.Conn) { p.pool <- conn }
- 連接池的使用範例
下面是一個簡單的使用範例,展示如何建立和使用連接池。
func main() { pool, err := NewConnectionPool(10, func() (net.Conn, error) { return net.Dial("tcp", "localhost:8080") }) if err != nil { fmt.Println("failed to create connection pool") return } conn := pool.GetConn() // do something with the connection pool.ReleaseConn(conn) }
三、最佳化技巧
除了基本的連線池管理之外,我們還可以透過一些最佳化技巧來提升網路連線池的效能和穩定性。
- 逾時設定
在連線池中,我們可以設定一個逾時時間,當連線在指定時間內未被使用時,我們可以關閉並丟棄它,以避免不必要的資源佔用。
func (p *ConnectionPool) GetConn() (net.Conn, error) { select { case conn := <-p.pool: return conn, nil case <-time.After(timeout): return nil, errors.New("connection pool timeout") } }
- 連接的健康檢查
我們可以定期對連接進行健康檢查,以確保連接池中的連接都是可用的。如果偵測到某條連線不可用,我們可以關閉並丟棄它,並重新建立一個新的連線。
func (p *ConnectionPool) CheckConn() { for conn := range p.pool { // do health check on conn // if conn is not healthy, close and discard it // create a new connection and put it into pool } }
- 連線池的動態調整
我們可以根據實際情況動態調整連線池的大小。當連接池中的連接不足時,我們可以根據負載情況動態地增加連接的數量;而當連接池中的連接過多時,我們可以適當減少連接的數量。
func (p *ConnectionPool) AdjustPoolSize() { // check current workload // increase or decrease pool size accordingly }
四、總結
在Go語言開發中,網路連線池管理是一項非常重要的技術。透過合理利用goroutine和channel等特性,我們可以實現高效的連接池管理,並透過一些優化技巧來提升連接池的效能和穩定性。希望本文所介紹的內容對讀者在Go語言開發中的網路連結池管理有所幫助。
參考文獻:
- Go語言中文網:https://studygolang.com/
- Go語言標準庫文件:https://golang.org /pkg/
以上是如何最佳化Go語言開發中的網路連線池管理的詳細內容。更多資訊請關注PHP中文網其他相關文章!
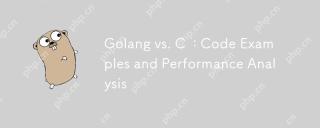
Golang適合快速開發和並發編程,而C 更適合需要極致性能和底層控制的項目。 1)Golang的並發模型通過goroutine和channel簡化並發編程。 2)C 的模板編程提供泛型代碼和性能優化。 3)Golang的垃圾回收方便但可能影響性能,C 的內存管理複雜但控制精細。
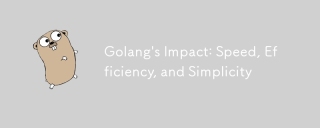
goimpactsdevelopmentpositationality throughspeed,效率和模擬性。 1)速度:gocompilesquicklyandrunseff,IdealforlargeProjects.2)效率:效率:ITScomprehenSevestAndardArdardArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增強的Depleflovelmentimency.3)簡單性。
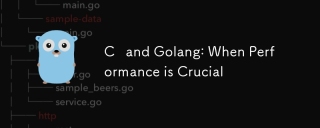
C 更適合需要直接控制硬件資源和高性能優化的場景,而Golang更適合需要快速開發和高並發處理的場景。 1.C 的優勢在於其接近硬件的特性和高度的優化能力,適合遊戲開發等高性能需求。 2.Golang的優勢在於其簡潔的語法和天然的並發支持,適合高並發服務開發。
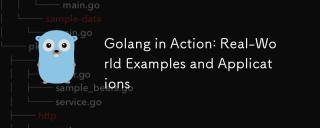
Golang在实际应用中表现出色,以简洁、高效和并发性著称。1)通过Goroutines和Channels实现并发编程,2)利用接口和多态编写灵活代码,3)使用net/http包简化网络编程,4)构建高效并发爬虫,5)通过工具和最佳实践进行调试和优化。
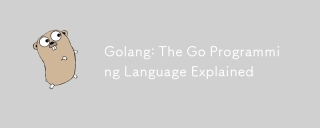
Go語言的核心特性包括垃圾回收、靜態鏈接和並發支持。 1.Go語言的並發模型通過goroutine和channel實現高效並發編程。 2.接口和多態性通過實現接口方法,使得不同類型可以統一處理。 3.基本用法展示了函數定義和調用的高效性。 4.高級用法中,切片提供了動態調整大小的強大功能。 5.常見錯誤如競態條件可以通過gotest-race檢測並解決。 6.性能優化通過sync.Pool重用對象,減少垃圾回收壓力。
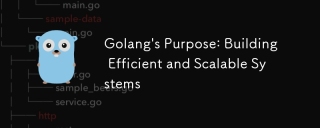
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
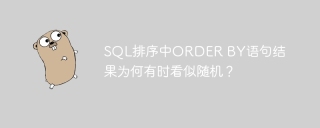
關於SQL查詢結果排序的疑惑學習SQL的過程中,常常會遇到一些令人困惑的問題。最近,筆者在閱讀《MICK-SQL基礎�...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
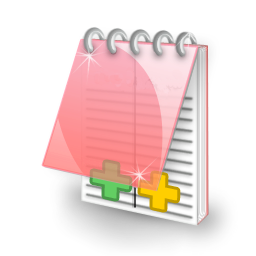
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
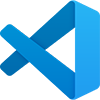
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SublimeText3 Linux新版
SublimeText3 Linux最新版

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中