在當前行動網路時代,頁面效果的設計對於網站和行動應用程式來說已經越來越重要。為了提高客戶體驗和受歡迎程度,我們需要藉助諸如仿特效大師等工具,以高品質的效果來設計我們的頁面。但是,如果你使用Vue框架來實現仿特效大師的設計,將會為你提供兩個巨大的好處。首先,你可以使用Vue框架的元件化程式模式設計一個可重複使用的元件庫來增強您的設計能力。其次,Vue框架具有強大的單向資料綁定和響應式資料原理,可以更快地更新DOM元素並且提高使用者體驗。
下面我們將詳細介紹如何使用Vue實現仿特效大師的頁面設計。
1.準備工作
在開始之前,請確保您已經安裝了最新版本的Vue.js。您可以從Vue.js官方網站下載並使用CDN來包含Vue.js。這裡我們將使用Vue.js 2.6.12。
第一步: 使用Vue CLI 建立一個新的專案
vue create vue-effect-design cd vue-effect-design
第二步: 安裝所需的依賴函式庫
npm install axios vue-router vuex
現在您已經準備好開始使用Vue了!接下來,一步一步介紹如何使用Vue實現仿特效大師的頁面設計。
2.建立基礎頁面
在開始頁面建立之前,請確保您已經安裝了適當的編輯器。我們推薦使用Visual Studio Code或Brackets。
第一步: 建立一個名為App.vue
的文件,並在該文件中加入以下程式碼:
<template> <div id="app"> <router-view/> </div> </template> <script> export default { name: 'App' } </script> <style> #app { min-height: 100vh; display: flex; justify-content: center; align-items: center; font-size: 2rem; background-color: #f7f8fc; color: #333; font-weight: bold; font-family: Arial, Helvetica, sans-serif; } </style>
在以上程式碼中,我們建立了一個Vue元件,該元件使用了路由器視圖,因此Vue將讀取元件的路由器視觀,並根據路由器視圖渲染元件。
第二步: 建立一個名為Home.vue
的文件,並在該文件中加入以下程式碼:
<template> <div> <header/> <main> <card> <img src="/static/imghwm/default1.png" data-src="imageUrl" class="lazy" : :alt="imageAlt"> <h2 id="title">{{ title }}</h2> <p>{{ message }}</p> </card> </main> <footer/> </div> </template> <script> import Header from '@/components/Header.vue' import Footer from '@/components/Footer.vue' import Card from '@/components/Card.vue' export default { name: 'Home', components: { Header, Footer, Card }, data () { return { title: 'Hello World', message: 'Welcome to Vue.js!', imageUrl: 'https://picsum.photos/400/300', imageAlt: 'Random image from Picsum' } } } </script>
在以上程式碼中,我們使用了Header
、Footer
與Card
三個元件,而且使用了v-bind
對img
標籤進行了資料綁定。現在,我們可以啟動我們的開發伺服器,並確保頁面正常運作:
npm run serve
3.建立可重複使用的元件庫
接下來,我們將建立一個可重複使用的元件庫,該庫可以幫助我們更快地創建頁面。
第一步: 建立一個名為Header.vue
的文件,並在該文件中加入以下程式碼:
<template> <header> <h1 id="siteTitle">{{ siteTitle }}</h1> </header> </template> <script> export default { name: 'Header', data () { return { siteTitle: '仿特效大师' } } } </script> <style scoped> header { background-color: #fff; margin-bottom: 2rem; text-align: center; border-bottom: 1px solid #ccc; } h1 { margin: 0; font-weight: bold; font-size: 2.6rem; font-family: Arial, Helvetica, sans-serif; } </style>
在以上在程式碼中,我們建立了一個名為Header
的元件,其中包含了一個標題和一個文字標籤。
第二步: 建立一個名為Footer.vue
的文件,並在該文件中加入以下程式碼:
<template> <footer> <slot/> </footer> </template> <script> export default { name: 'Footer' } </script> <style scoped> footer { background-color: #fff; margin-top: 2rem; text-align: center; border-top: 1px solid #ccc; padding: 2rem; } </style>
在以上在程式碼中,我們建立了一個名為Footer
的元件,並使用了一個插槽來放置任何內容。
第三個步驟: 建立一個名為Card.vue
的文件,並在該文件中加入以下程式碼:
<template> <div class="card"> <slot/> </div> </template> <script> export default { name: 'Card' } </script> <style scoped> .card { background-color: #fff; padding: 2rem; box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1); border-radius: 6px; margin-bottom: 2rem; } img { max-width: 100%; } </style>
在以上程式碼中,我們創建了名為Card
(卡)的元件,在該元件中包含了一個插槽並使用CSS樣式進行了美化。
4.使用Axios和API
我們將使用Axios函式庫從外部API取得資料。 Axios是用來從Web客戶端發出HTTP請求的函式庫,它將傳回Promise對象,可以使我們比較簡單地從外部API取得資料。
第一步: 在上述步驟的基礎上,取代我們的Home元件中的data
屬性,以便我們從外部API取得資料。程式碼如下:
<template> <div> <header/> <main> <div v-if="isLoading" class="loading"></div> <card> <img src="/static/imghwm/default1.png" data-src="imageUrl" class="lazy" : :alt="imageAlt"> <h2 id="title">{{ title }}</h2> <p>{{ message }}</p> </card> </main> <footer/> </div> </template> <script> import axios from 'axios' import Header from '@/components/Header.vue' import Footer from '@/components/Footer.vue' import Card from '@/components/Card.vue' export default { name: 'Home', components: { Header, Footer, Card }, data () { return { title: '', message: '', imageUrl: '', imageAlt: '', isLoading: false } }, created () { this.fetchData() }, methods: { fetchData () { this.isLoading = true axios.get('https://jsonplaceholder.typicode.com/posts/1') .then(response => { this.isLoading = false this.title = response.data.title this.message = response.data.body this.imageUrl = `https://picsum.photos/400/300?random=${response.data.id}` this.imageAlt = response.data.title }) .catch(error => { console.log(error) this.isLoading = false }) } } } </script> <style scoped> header { background-color: #fff; margin-bottom: 2rem; text-align: center; border-bottom: 1px solid #ccc; } h1 { margin: 0; font-weight: bold; font-size: 2.6rem; font-family: Arial, Helvetica, sans-serif; } .loading { border: 16px solid #f3f3f3; border-top: 16px solid #3498db; border-radius: 50%; width: 120px; height: 120px; animation: spin 2s linear infinite; margin: 2rem auto 0; } @keyframes spin { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } } </style>
在上述程式碼中,我們做了以下變更:
- #刪除了在
data
屬性中未使用的預設屬性。 - 建立了一個名為
fetchData
的新方法以使用Vue元件呼叫外部API來取得資料。 - 將API回應的標題,文字和URL屬性指派給資料屬性。
- 新增了一個載入指示器以顯示載入過程,該指示器透過CSS樣式產生旋轉效果。
- 在
created
生命週期內呼叫了新建立的fetchData
方法。
5.動態路由
最終,我們將學習如何使用Vue Router動態路由。
第一步: 建立一個名為Design.vue
的文件,並在該文件中加入以下程式碼:
<template> <div> <header/> <main> <card v-for="item in designs" :key="item.id"> <router-link :to="{ name: 'Details', params: { id: item.id }}"> <img src="/static/imghwm/default1.png" data-src="item.image" class="lazy" : :alt="item.title"> </router-link> <h2 id="item-title">{{ item.title }}</h2> <p>{{ item.description }}</p> </card> </main> <footer/> </div> </template> <script> import Header from '@/components/Header.vue' import Footer from '@/components/Footer.vue' import Card from '@/components/Card.vue' export default { name: 'Design', components: { Header, Footer, Card }, data () { return { designs: [ { id: 1, title: 'Design 1', description: 'Description of Design 1', image: 'https://picsum.photos/400/300' }, { id: 2, title: 'Design 2', description: 'Description of Design 2', image: 'https://picsum.photos/400/300' }, { id: 3, title: 'Design 3', description: 'Description of Design 3', image: 'https://picsum.photos/400/300' } ] } } } </script>
在以上在程式碼中,我們建立了一個名為Design
的Vue元件,並在其中使用了三個範例資料進行實驗。
第二步: 更新router.js
文件,並在該文件中加入以下程式碼:
import Vue from 'vue' import VueRouter from 'vue-router' import Home from './views/Home.vue' import Design from './views/Design.vue' import Details from './views/Details.vue' Vue.use(VueRouter) const routes = [ { path: '/', name: 'Home', component: Home }, { path: '/designs', name: 'Design', component: Design }, { path: '/details/:id', name: 'Details', component: Details } ] const router = new VueRouter({ mode: 'history', base: process.env.BASE_URL, routes }) export default router
在上述程式碼中,我們更新了路由。新增了一個名為Design
的新路由和Details
這個動態的路由。
第三個步驟: 建立一個名為Details.vue
的文件,並在該文件中加入以下程式碼:
<template> <div> <header/> <main> <card> <img src="/static/imghwm/default1.png" data-src="selectedDesign.image" class="lazy" : :alt="selectedDesign.title"> <h2 id="selectedDesign-title">{{ selectedDesign.title }}</h2> <p>{{ selectedDesign.description }}</p> </card> </main> <footer/> </div> </template> <script> import Header from '@/components/Header.vue' import Footer from '@/components/Footer.vue' import Card from '@/components/Card.vue' export default { name: 'Details', components: { Header, Footer, Card }, data () { return { selectedDesign: {} } }, created () { this.fetchData() }, methods: { fetchData () { const id = this.$route.params.id // 从外部API获取数据 } } } </script>
在以上代码中,我们创建了一个名为Details
的Vue组件,并使用了数据属性selectedDesign
来保存所选设计的详细信息。另外,我们还创建了一个名为fetchData
的新方法以与外部API通信的方法获取动态的数据。
第四步: 在fetchData
方法的代码中,我们使用了this.$route.params.id
来获取具体的路由参数,并这些参数使用外部API获取具体路由的数据。
axios.get(`https://jsonplaceholder.typicode.com/posts/${id}`) .then(response => { this.selectedDesign = response.data this.isLoading = false }) .catch(error => { console.log(error) this.isLoading = false })
以上代码将请求数据,并在响应中将选择的设计属性分配到数据属性中。
这样,我们就可以使用Vue路由动态显示数据到页面中,并完成了仿特效大师的页面设计。
总结:
在本文中,我们研究了如何使用Vue.js实现仿特效大师的页面设计。我们首先介绍了Vue组件,组件化编程模式以及Vue强大的单向数据绑定和响应式数据原理的基本概念。接下来我们创建了一个简单的基本页面,然后创建了一个可重用的组件库,以帮助我们更快地编写页面。我们学习了如何使用Axios与外部API进行通信,最后使用Vue Router动态路由显示数据从而完成了页面的设计。希望这篇文章对您有所帮助,谢谢!
以上是如何使用 Vue 實現仿特效大師的頁面設計?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

Vue.js是由尤雨溪在2014年發布的漸進式JavaScript框架,用於構建用戶界面。它的核心優勢包括:1.響應式數據綁定,數據變化自動更新視圖;2.組件化開發,UI可拆分為獨立、可複用的組件。

Netflix使用React作為其前端框架。 1)React的組件化開發模式和強大生態系統是Netflix選擇它的主要原因。 2)通過組件化,Netflix將復雜界面拆分成可管理的小塊,如視頻播放器、推薦列表和用戶評論。 3)React的虛擬DOM和組件生命週期優化了渲染效率和用戶交互管理。

Netflix在前端技術上的選擇主要集中在性能優化、可擴展性和用戶體驗三個方面。 1.性能優化:Netflix選擇React作為主要框架,並開發了SpeedCurve和Boomerang等工具來監控和優化用戶體驗。 2.可擴展性:他們採用微前端架構,將應用拆分為獨立模塊,提高開發效率和系統擴展性。 3.用戶體驗:Netflix使用Material-UI組件庫,通過A/B測試和用戶反饋不斷優化界面,確保一致性和美觀性。

NetflixusesAcustomFrameworkcalled“ Gibbon” BuiltonReact,notReactorVuedIrectly.1)TeamSperience:selectBasedonFamiliarity.2)ProjectComplexity:vueforsimplerprojects:reactforforforproproject,reactforforforcompleplexones.3)cocatizationneedneeds:reactoffipicatizationneedneedneedneedneedneeds:reactoffersizationneedneedneedneedneeds:reactoffersizatization needefersmoreflexibleise.4)

Netflix在框架選擇上主要考慮性能、可擴展性、開發效率、生態系統、技術債務和維護成本。 1.性能與可擴展性:選擇Java和SpringBoot以高效處理海量數據和高並發請求。 2.開發效率與生態系統:使用React提升前端開發效率,利用其豐富的生態系統。 3.技術債務與維護成本:選擇Node.js構建微服務,降低維護成本和技術債務。

Netflix主要使用React作為前端框架,輔以Vue用於特定功能。 1)React的組件化和虛擬DOM提升了Netflix應用的性能和開發效率。 2)Vue在Netflix的內部工具和小型項目中應用,其靈活性和易用性是關鍵。

Vue.js是一種漸進式JavaScript框架,適用於構建複雜的用戶界面。 1)其核心概念包括響應式數據、組件化和虛擬DOM。 2)實際應用中,可以通過構建Todo應用和集成VueRouter來展示其功能。 3)調試時,建議使用VueDevtools和console.log。 4)性能優化可通過v-if/v-show、列表渲染優化和異步加載組件等實現。

Vue.js適合小型到中型項目,而React更適用於大型、複雜應用。 1.Vue.js的響應式系統通過依賴追踪自動更新DOM,易於管理數據變化。 2.React採用單向數據流,數據從父組件流向子組件,提供明確的數據流向和易於調試的結構。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

Atom編輯器mac版下載
最受歡迎的的開源編輯器

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。
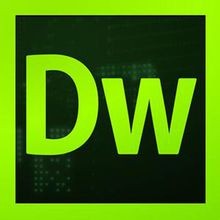
Dreamweaver CS6
視覺化網頁開發工具