隨著前端技術的不斷更新,Vue 作為一個流行的前端框架,已經成為許多開發者的首選。在實際專案中,常常需要使用 tab 元件以及多標籤頁來實現不同功能模組的切換和管理。在本文中,我們將介紹如何利用 Vue 實作一個簡單的 tab 元件以及多標籤頁。
一、實作一個簡單的tab 元件
- 建立Tab.vue 元件
在專案中建立一個Tab.vue 的元件,用於顯示tab 清單和目前選取的tab 頁的內容。
<template> <div> <div class="tab-header"> <div class="tab-item" v-for="(tab, index) in tabs" :key="index" :class="{ active: index === currentIndex }" @click="handleTab(index)"> {{ tab.title }} </div> </div> <div class="tab-content"> <slot></slot> </div> </div> </template> <script> export default { name: 'Tab', props: { tabs: { type: Array, required: true, }, currentIndex: { type: Number, default: 0, }, }, methods: { handleTab(index) { this.$emit('tabchange', index); }, }, }; </script>
在這個元件中,我們透過使用 v-for 指令來遍歷傳入的 tabs 數組,將每個 tab 的標題渲染為一個 tab-item。選取的 tab 頁的 currentIndex 會在 tab-item 上新增 active class,以便高亮顯示目前選取的 tab 頁。 tab 元件附有 slot 插槽,用於顯示 tab 頁內容。
- 在父元件中使用Tab 元件
在需要使用tab 元件的頁面上,將Tab 元件匯入後在template 中使用,將需要展示的tab頁作為tabs 陣列的成員傳入至Tab 元件中,並透過currentIndex 屬性指定目前選取的tab 頁的下標。此外,在 Tab 元件中監聽 tabchange 事件,以便在父元件中變更 currentIndex 的值來控制目前選取的 tab 頁。
<template> <div> <Tab :tabs="tabs" :currentIndex="currentIndex" @tabchange="handleChange"> <div v-for="(tab, index) in tabs" :key="index"> {{ tab.content }} </div> </Tab> </div> </template> <script> import Tab from './Tab.vue'; export default { name: 'App', components: { Tab, }, data() { return { currentIndex: 0, tabs: [ { title: 'tab1', content: '这是第一个 tab 页的内容', }, { title: 'tab2', content: '这是第二个 tab 页的内容', }, ], }; }, methods: { handleChange(index) { this.currentIndex = index; }, }, }; </script>
在該父元件中,我們首先需要匯入 Tab 元件,然後建立 tabs 數組,用於儲存每個 tab 頁的標題和內容。在 template 需要傳入 tabs 和 currentIndex 兩個屬性,分別用於初始化 Tab 元件。在 handleChange 方法中,我們監聽了 tabchange 事件,從而可以在父元件中更新 currentIndex 的值,來實現選取不同的 tab 頁。
二、實作多標籤頁元件
- 建立頁面佈局及路由配置
在專案中建立一個具有多標籤頁功能的頁面,首先需要考慮頁面的佈局。在本文中,我們使用了 Element UI 的 Layout 佈局元件來建立頁面基礎結構,然後透過 Vue Router 來完成路由設定。在多標籤頁場景下,每個 tab 頁可視為路由頁面,因此需要在路由表中新增對應路由資訊。
<template> <div class="page-container"> <el-container> <el-header> <h1 id="pageTitle">{{ pageTitle }}</h1> </el-header> <el-container> <el-aside style="width: 200px; height: 100%"> <el-menu :default-active="activeMenu" @select="handleSelect" unique-opened :collapse="isCollapse"> <el-submenu v-for="menuGroup in menuList" :key="menuGroup.name" :index="menuGroup.name"> <template slot="title"> <i :class="menuGroup.icon"></i> <span>{{ menuGroup.title }}</span> </template> <el-menu-item v-for="menuItem in menuGroup.children" :key="menuItem.path" :index="menuItem.path"> <i :class="menuItem.icon"></i> <span slot="title">{{ menuItem.title }}</span> </el-menu-item> </el-submenu> </el-menu> </el-aside> <el-main> <router-view/> </el-main> </el-container> </el-container> </div> </template> <script> export default { name: 'PageLayout', data() { return { pageTitle: '多标签页示例', menuList: [ { name: 'group1', title: '分组 1', icon: 'el-icon-s-home', children: [ { title: '页面 1', path: '/page1', icon: 'el-icon-s-order', }, { title: '页面 2', path: '/page2', icon: 'el-icon-s-data', }, ], }, { name: 'group2', title: '分组 2', icon: 'el-icon-s-management', children: [ { title: '页面 3', path: '/page3', icon: 'el-icon-s-flag', }, { title: '页面 4', path: '/page4', icon: 'el-icon-menu', }, ], }, ], activeMenu: '', isCollapse: false, }; }, methods: { handleSelect(index) { this.activeMenu = index; this.$router.push(index); }, }, }; </script>
在父元件 PageLayout.vue 中,我們首先設定了頁面標題 pageTitle、選單清單 menuList 和兩個狀態值 activeMenu 和 isCollapse,用於選單的展開與關閉。接著利用 Element UI 的 Layout 元件建立了頁面的基本結構,左側為選單欄,右側為標籤頁內容。在選單元件el-menu 中,我們透過v-for 循環渲染了選單分組和選單項,其中選單項目綁定了對應的路由path,點擊選單項目後會觸發handleSelect 方法,將路由path 傳入$router. push 方法中跳轉至對應頁面。在該元件中我們需要使用 router-view 去展示對應的路由元件。
- 實作Tab 元件及多標籤頁功能
在完成頁面佈局和路由配置後,我們需要在頁面中實作tab 元件及多標籤頁功能,並書寫對應的js 程式碼。在多標籤頁功能中,首先需要實作標籤列元件 TabBar.vue,用於展示各個標籤頁的標題和控制標籤頁的新增、刪除等操作。
<template> <div class="tab-bar" :class="{ fixed: isFixed }"> <div class="tab-bar-content"> <div v-for="(tab, index) in tabs" :key="index" class="tab-item" :class="{ active: currentIndex === index }" @click="handleChange(index)"> {{ tab.title }} <i class="el-icon-close" @click.stop="handleRemoveTab(index)"></i> </div> <i class="el-icon-circle-plus add-tab-btn" @click.stop="handleAddTab"></i> </div> </div> </template> <script> export default { name: 'TabBar', props: { tabs: { type: Array, required: true, }, currentIndex: { type: Number, default: 0, }, isFixed: { type: Boolean, default: false, }, }, methods: { handleChange(index) { this.$emit('tabchange', index); }, handleAddTab() { this.$emit('addtab'); }, handleRemoveTab(index) { this.$emit('removetab', index); }, }, }; </script>
在這個元件中,我們透過使用 v-for 迴圈來渲染傳入的 tabs 數組,將每個 tab 的標題渲染為一個 tab-item。選取的 tab 頁面會在 tab-item 上新增 active class,以便高亮顯示目前選取的 tab 頁。 tab-bar 元件自帶三個事件:tabchange 用於監聽 tab 切換事件,addtab 和 removetab 用於監聽 tab 新增和刪除事件,以便在父元件中更新 tabs 陣列的值。
在父元件PageLayout.vue 中,我們需要在頁面結構下方新增TabBar 元件,並為其傳入tabs 和currentIndex 屬性,然後在對應事件方法中對tabs 陣列進行更新操作,以便操作對應的標籤頁。
<template> <div class="page-container"> ... <el-main> <tab-bar :tabs="tabs" :currentIndex="currentIndex" :isFixed="isFixed" @tabchange="handleChange" @addtab="handleAddTab" @removetab="handleRemoveTab"/> <router-view v-if="$route.meta.keepAlive"/> <keep-alive> <router-view v-if="!$route.meta.keepAlive"/> </keep-alive> </el-main> </div> </template> <script> import TabBar from '../components/TabBar.vue'; export default { name: 'PageLayout', components: { TabBar, }, data() { return { tabs: [ { title: '首页', path: '/', }, ], currentIndex: 0, isFixed: false, }; }, created() { this.$router.afterEach((to, from) => { const index = this.tabs.findIndex(tab => tab.path === to.path); if (index !== -1) { this.currentIndex = index; this.isFixed = false; } else { this.addTab(to); } }); }, methods: { handleChange(index) { this.$router.push(this.tabs[index].path); }, handleAddTab() { const isExist = this.tabs.find(tab => tab.path === this.$route.path); if (!isExist) { this.tabs.push({ title: this.$route.meta.title || '', path: this.$route.path, }); this.currentIndex = this.tabs.length - 1; this.isFixed = false; } else { this.currentIndex = this.tabs.indexOf(isExist); this.isFixed = false; } this.$router.push(this.$route.path); }, handleRemoveTab(index) { const targetTab = this.tabs[index]; const targetPath = targetTab.path; this.tabs.splice(index, 1); if (targetPath === this.$route.path) { const lastIndex = this.tabs.length - 1; const lastTab = this.tabs[lastIndex]; this.currentIndex = lastIndex; this.$router.push(lastTab.path); } else { this.currentIndex = this.tabs.findIndex(tab => tab.path === this.$route.path); } }, }, }; </script>
在 PageLayout.vue 中,我們首先建立了 tabs 數組,用於儲存各個標籤頁的路由資訊。在 created 鉤子函數中,我們監聽了目前路由的變化,並在 tabs 陣列中尋找對應的標籤頁路由。如果存在,則直接定位到對應的標籤頁,否則將目前路由新增為新的標籤頁。在 handleAddTab 方法中,如果目前路由對應的標籤頁已存在,則直接選取已有標籤頁,否則在 tabs 陣列中加入新的標籤頁。在 handleRemoveTab 方法中,我們利用陣列的 splice 方法對已有標籤頁進行刪除操作,並在 tabs 陣列中定位到目前選取的標籤頁,並更新 currentIndex 和 $router 的跳躍資訊。
三、總結
在本文中,我們介紹如何利用 Vue 框架及相關外掛程式實作 tab 元件及多標籤頁功能。實際實作過程中,需要根據具體場景對元件或程式碼進行彈性調整,以便更好地適應實際專案需求。總的來說,tab 元件和多標籤頁功能可以為專案提供更好的可用性和使用者體驗,更好的解決前端頁面的架構問題。
以上是Vue 中如何實作 tab 元件及多標籤頁?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

Netflix在前端技術上的選擇主要集中在性能優化、可擴展性和用戶體驗三個方面。 1.性能優化:Netflix選擇React作為主要框架,並開發了SpeedCurve和Boomerang等工具來監控和優化用戶體驗。 2.可擴展性:他們採用微前端架構,將應用拆分為獨立模塊,提高開發效率和系統擴展性。 3.用戶體驗:Netflix使用Material-UI組件庫,通過A/B測試和用戶反饋不斷優化界面,確保一致性和美觀性。

NetflixusesAcustomFrameworkcalled“ Gibbon” BuiltonReact,notReactorVuedIrectly.1)TeamSperience:selectBasedonFamiliarity.2)ProjectComplexity:vueforsimplerprojects:reactforforforproproject,reactforforforcompleplexones.3)cocatizationneedneeds:reactoffipicatizationneedneedneedneedneedneeds:reactoffersizationneedneedneedneedneeds:reactoffersizatization needefersmoreflexibleise.4)

Netflix在框架選擇上主要考慮性能、可擴展性、開發效率、生態系統、技術債務和維護成本。 1.性能與可擴展性:選擇Java和SpringBoot以高效處理海量數據和高並發請求。 2.開發效率與生態系統:使用React提升前端開發效率,利用其豐富的生態系統。 3.技術債務與維護成本:選擇Node.js構建微服務,降低維護成本和技術債務。

Netflix主要使用React作為前端框架,輔以Vue用於特定功能。 1)React的組件化和虛擬DOM提升了Netflix應用的性能和開發效率。 2)Vue在Netflix的內部工具和小型項目中應用,其靈活性和易用性是關鍵。

Vue.js是一種漸進式JavaScript框架,適用於構建複雜的用戶界面。 1)其核心概念包括響應式數據、組件化和虛擬DOM。 2)實際應用中,可以通過構建Todo應用和集成VueRouter來展示其功能。 3)調試時,建議使用VueDevtools和console.log。 4)性能優化可通過v-if/v-show、列表渲染優化和異步加載組件等實現。

Vue.js適合小型到中型項目,而React更適用於大型、複雜應用。 1.Vue.js的響應式系統通過依賴追踪自動更新DOM,易於管理數據變化。 2.React採用單向數據流,數據從父組件流向子組件,提供明確的數據流向和易於調試的結構。

Vue.js適合中小型項目和快速迭代,React適用於大型複雜應用。 1)Vue.js易於上手,適用於團隊經驗不足或項目規模較小的情況。 2)React的生態系統更豐富,適合有高性能需求和復雜功能需求的項目。
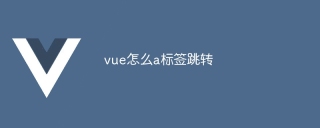
實現 Vue 中 a 標籤跳轉的方法包括:HTML 模板中使用 a 標籤指定 href 屬性。使用 Vue 路由的 router-link 組件。使用 JavaScript 的 this.$router.push() 方法。可通過 query 參數傳遞參數,並在 router 選項中配置路由以進行動態跳轉。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
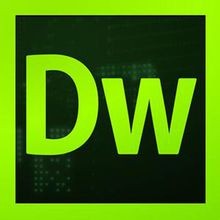
Dreamweaver CS6
視覺化網頁開發工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

SublimeText3 Linux新版
SublimeText3 Linux最新版

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。
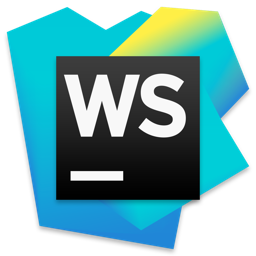
WebStorm Mac版
好用的JavaScript開發工具