在開發PHP專案時,程式碼混淆是一種常見的技術,其主要目的是使程式碼難以被破解、保護程式碼智慧財產權。
程式碼混淆是一種將程式碼進行加工,以使人類難以讀懂的技術。這種加工可以包括新增冗餘程式碼、重新命名變數和函數名稱、刪除註解和空格等。程式碼混淆雖然並不能真正加強程式碼的安全性,但它會使攻擊者難以查看程式碼邏輯和逆向工程製定攻擊計劃。
在PHP開發中,程式碼混淆可以使用第三方工具,例如Zend Guard和Ioncube。但是,這些工具的使用通常需要付費,而且不一定適用於所有的PHP項目。因此,本文將介紹如何使用PHP原生功能來實現程式碼混淆。
- 重命名變數和函數名稱
在PHP中,變數和函數名稱是在執行時間解析的。因此,可以編寫一個腳本來自動將所有變數和函數名稱進行重新命名,使它們變得更加難以理解。這可以透過PHP的反射機制來實現。反射是一種在運行時檢查類別、方法和屬性的能力。以下是一個簡單的例子:
<?php function myFunction($parameter1, $parameter2) { return $parameter1 + $parameter2; } $reflectionFunc = new ReflectionFunction('myFunction'); $reflectionParams = $reflectionFunc->getParameters(); foreach ($reflectionParams as $param) { $newName = generateRandomString(); renameParameter($reflectionFunc, $param->getName(), $newName); } renameFunction($reflectionFunc, 'myFunction', generateRandomString()); function renameParameter($reflectionFunc, $currentName, $newName) { $definition = $reflectionFunc->getFileName() . ':' . $reflectionFunc->getStartLine(); $contents = file_get_contents($definition); $contents = str_replace('$' . $currentName, '$' . $newName, $contents); file_put_contents($definition, $contents); } function renameFunction($reflectionFunc, $currentName, $newName) { $definition = $reflectionFunc->getFileName() . ':' . $reflectionFunc->getStartLine(); $contents = file_get_contents($definition); $contents = str_replace('function ' . $currentName, 'function ' . $newName, $contents); file_put_contents($definition, $contents); } function generateRandomString($length = 10) { $characters = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; $charactersLength = strlen($characters); $randomString = ''; for ($i = 0; $i < $length; $i++) { $randomString .= $characters[rand(0, $charactersLength - 1)]; } return $randomString; } ?>
- 添加冗餘程式碼
為了讓程式碼變得難以理解,可以添加一些冗餘的程式碼區塊。這些程式碼區塊通常與程式碼的主要功能無關,但在限制攻擊者對程式碼的理解方面卻十分有用。以下是一個簡單的範例:
<?php $randomInt1 = rand(1, 10); $randomInt2 = rand(10, 100); $randomInt3 = rand(100, 1000); if ($randomInt1 > 3) { if ($randomInt2 > 50) { $tempString = "abcdefghijklmnopqrstuvwxyz1234567890"; for ($i = 0; $i < 5; $i++) { $randNum = rand(0, strlen($tempString) - 1); } } else { $tempString = "ABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890"; for ($i = 0; $i < 10; $i++) { $randNum = rand(0, strlen($tempString) - 1); } } } else { if ($randomInt3 > 500) { $tempString = "$&/\+%()?!""; for ($i = 0; $i < 5; $i++) { $randNum = rand(0, strlen($tempString) - 1); } } else { $tempString = " "; for ($i = 0; $i < 10; $i++) { $randNum = rand(0, strlen($tempString) - 1); } } } ?>
- 刪除註解和空格
#最後,在混淆程式碼之前,可以刪除所有註解和空格。這可以透過使用PHP的語法分析器來實現。以下是一個簡單的例子:
<?php // Define the input to the script $input = "<?php /** * Display user comments */ function displayComments($postId) { // Connect to the database $connection = new mysqli($host, $username, $password, $dbName); // Get the comments for the post $query = "SELECT * FROM comments WHERE post_id = {$postId}"; $results = $connection->query($query); // Display the comments while ($row = $results->fetch_assoc()) { echo "<p>{$row['comment']}</p>"; } } ?>"; // Use the PHP syntax parser to remove comments and whitespace $tokens = token_get_all($input); $output = ""; foreach ($tokens as $token) { if (is_array($token)) { if ($token[0] == T_COMMENT || $token[0] == T_DOC_COMMENT) { continue; } else { $output .= $token[1]; } } else { $output .= $token; } } echo $output; ?>
綜上所述,在PHP開發中實現程式碼混淆的過程可以分為三個步驟:重新命名變數和函數名稱、新增冗餘程式碼、刪除註解和空格。透過這些步驟,我們可以有效地保護我們的PHP程式碼,使它難以被破解和逆向工程。
以上是如何利用PHP實作程式碼混淆功能的詳細內容。更多資訊請關注PHP中文網其他相關文章!
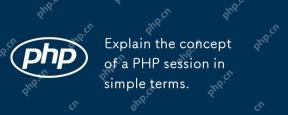
phpsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIdStoredInAcookie.here'showtomanageThemeffectionaly:1)startAsessionWithSessionWwithSession_start()和stordoredAtain $ _session.2)
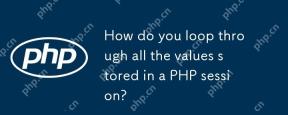
在PHP中,遍歷會話數據可以通過以下步驟實現:1.使用session_start()啟動會話。 2.通過foreach循環遍歷$_SESSION數組中的所有鍵值對。 3.處理複雜數據結構時,使用is_array()或is_object()函數,並用print_r()輸出詳細信息。 4.優化遍歷時,可採用分頁處理,避免一次性處理大量數據。這將幫助你在實際項目中更有效地管理和使用PHP會話數據。
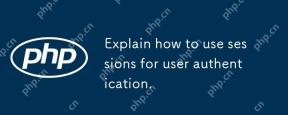
會話通過服務器端的狀態管理機制實現用戶認證。 1)會話創建並生成唯一ID,2)ID通過cookies傳遞,3)服務器存儲並通過ID訪問會話數據,4)實現用戶認證和狀態管理,提升應用安全性和用戶體驗。
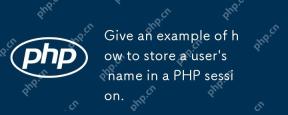
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
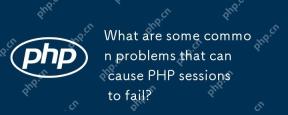
PHPSession失效的原因包括配置錯誤、Cookie問題和Session過期。 1.配置錯誤:檢查並設置正確的session.save_path。 2.Cookie問題:確保Cookie設置正確。 3.Session過期:調整session.gc_maxlifetime值以延長會話時間。
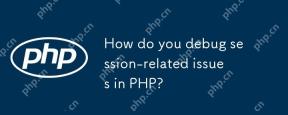
在PHP中調試會話問題的方法包括:1.檢查會話是否正確啟動;2.驗證會話ID的傳遞;3.檢查會話數據的存儲和讀取;4.查看服務器配置。通過輸出會話ID和數據、查看會話文件內容等方法,可以有效診斷和解決會話相關的問題。
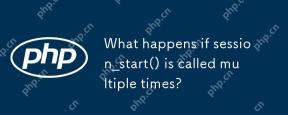
多次調用session_start()會導致警告信息和可能的數據覆蓋。 1)PHP會發出警告,提示session已啟動。 2)可能導致session數據意外覆蓋。 3)使用session_status()檢查session狀態,避免重複調用。
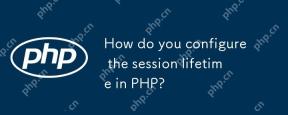
在PHP中配置會話生命週期可以通過設置session.gc_maxlifetime和session.cookie_lifetime來實現。 1)session.gc_maxlifetime控制服務器端會話數據的存活時間,2)session.cookie_lifetime控制客戶端cookie的生命週期,設置為0時cookie在瀏覽器關閉時過期。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
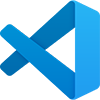
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

Atom編輯器mac版下載
最受歡迎的的開源編輯器
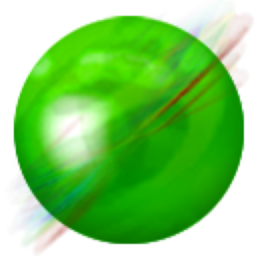
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中