Vue作為一種流行的前端框架,常常需要實作元件之間的值傳遞功能。其中,相鄰元件的值傳遞時,主要透過呼叫元件的方法來實現。本文將介紹Vue中的相鄰組件傳值函數實作方法。
1.父元件傳遞值向子元件
在Vue中,透過v-bind指令實作將父元件的值綁定到子元件中。具體實作程式碼如下:
在父元件中:
<template> <div> <child-component v-bind:data="parentData"></child-component> </div> </template> <script> import childComponent from './childComponent.vue'; export default { components: { childComponent }, data: { parentData: 'Hello, Vue!' } } </script>
在子元件中:
<template> <div> <p>{{data}}</p> </div> </template> <script> export default { props: ['data'] } </script>
上述程式碼將父元件的資料parentData
透過v-bind:data
綁定到子元件的data
屬性。
2.子元件向父元件傳遞值
在Vue中,子元件需要透過 $emit
方法向父元件發送事件。在父元件中註冊該事件,並在回呼函數中處理子元件發送的資料。具體實作程式碼如下:
在父元件中:
<template> <div> <child-component v-on:send-data="handleChildData"></child-component> </div> </template> <script> import childComponent from './childComponent.vue'; export default { components: { childComponent }, methods: { handleChildData(data) { console.log(data); } } } </script>
在子元件中:
<template> <div> <button v-on:click="sendDataToParent">向父组件传递数据</button> </div> </template> <script> export default { methods: { sendDataToParent() { this.$emit('send-data', 'Hello, Parent!'); } } } </script>
上述程式碼中,子元件透過v-on:click
綁定sendDataToParent
方法,在方法中透過$emit
方法向父元件發送事件send-data
並傳遞資料Hello, Parent !
。在父元件中,透過v-on:send-data
註冊事件send-data
的回呼函數handleChildData
,並在函數中處理子元件傳回的參數。
3.兄弟元件之間傳遞值
兄弟元件之間傳遞資料時,需要透過父元件作為中間橋樑。具體實作程式碼如下:
在父元件中:
<template> <div> <brother-component1 v-on:update-data="handleBrotherData"></brother-component1> <br> <brother-component2 v-bind:data="parentData"></brother-component2> </div> </template> <script> import brotherComponent1 from './brotherComponent1.vue'; import brotherComponent2 from './brotherComponent2.vue'; export default { components: { brotherComponent1, brotherComponent2 }, data: { parentData: '' }, methods: { handleBrotherData(data) { this.parentData = data; } } } </script>
在子元件1中:
<template> <div> <button v-on:click="sendDataToBrother">向兄弟组件2传递数据</button> </div> </template> <script> export default { methods: { sendDataToBrother() { this.$emit('update-data', 'Hello, Brother 2!'); } } } </script>
在子元件2中:
<template> <div> <p>{{data}}</p> </div> </template> <script> export default { props: ['data'] } </script>
上述程式碼中,子元件1向父元件發送事件update-data
並傳遞資料Hello, Brother 2!
;父元件中監聽該事件v-on:update- data
並在函數中處理資料handleBrotherData
,並將處理後的資料透過v-bind:data
綁定到子元件2的data
#屬性中。
綜上所述,Vue中的相鄰元件傳值函數實作方法主要是透過父子元件之間的值綁定和事件通訊來完成。而兄弟組件之間要透過父組件作為中間橋樑來實現。這種方法簡單易懂、靈活方便,是Vue中非常重要的組件通訊方式。
以上是Vue文檔中的相鄰元件傳值函數實作方法的詳細內容。更多資訊請關注PHP中文網其他相關文章!

Vue.js適合快速開發和小型項目,而React更適合大型和復雜的項目。 1.Vue.js簡單易學,適用於快速開發和小型項目。 2.React功能強大,適合大型和復雜的項目。 3.Vue.js的漸進式特性適合逐步引入功能。 4.React的組件化和虛擬DOM在處理複雜UI和數據密集型應用時表現出色。

Vue.js和React各有優缺點,選擇時需綜合考慮團隊技能、項目規模和性能需求。 1)Vue.js適合快速開發和小型項目,學習曲線低,但深層嵌套對象可能導致性能問題。 2)React適用於大型和復雜應用,生態系統豐富,但頻繁更新可能導致性能瓶頸。

Vue.js適合小型到中型項目,React適合大型項目和復雜應用場景。 1)Vue.js易於上手,適用於快速原型開發和小型應用。 2)React在處理複雜狀態管理和性能優化方面更有優勢,適合大型項目。

Vue.js和React各有優勢:Vue.js適用於小型應用和快速開發,React適合大型應用和復雜狀態管理。 1.Vue.js通過響應式系統實現自動更新,適用於小型應用。 2.React使用虛擬DOM和diff算法,適合大型和復雜應用。選擇框架時需考慮項目需求和團隊技術棧。

Vue.js和React各有優勢,選擇應基於項目需求和團隊技術棧。 1.Vue.js社區友好,提供豐富學習資源,生態系統包括VueRouter等官方工具,支持由官方團隊和社區提供。 2.React社區偏向企業應用,生態系統強大,支持由Facebook及其社區提供,更新頻繁。

Netflix使用React來提升用戶體驗。 1)React的組件化特性幫助Netflix將復雜UI拆分成可管理模塊。 2)虛擬DOM優化了UI更新,提高了性能。 3)結合Redux和GraphQL,Netflix高效管理應用狀態和數據流動。

Vue.js是前端框架,後端框架用於處理服務器端邏輯。 1)Vue.js專注於構建用戶界面,通過組件化和響應式數據綁定簡化開發。 2)後端框架如Express、Django處理HTTP請求、數據庫操作和業務邏輯,運行在服務器上。

Vue.js與前端技術棧緊密集成,提升開發效率和用戶體驗。 1)構建工具:與Webpack、Rollup集成,實現模塊化開發。 2)狀態管理:與Vuex集成,管理複雜應用狀態。 3)路由:與VueRouter集成,實現單頁面應用路由。 4)CSS預處理器:支持Sass、Less,提升樣式開發效率。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
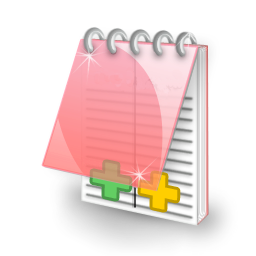
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

禪工作室 13.0.1
強大的PHP整合開發環境