隨著網路的快速發展,HTTP請求已成為各種Web應用程式的重要組成部分。對於PHP開發者而言,Guzzle是一個非常值得推薦的HTTP客戶端程式庫,可以幫助我們發起HTTP請求、處理回應、管理會話等。
本文將介紹如何使用PHP和Guzzle發起HTTP請求,幫助讀者更能理解Guzzle的基本原理和使用方法。
一、Guzzle簡介
Guzzle 是一個 PHP 函式庫,用於發起 HTTP 請求並處理回應。它的設計目標是提供簡單、優雅的 API,並且是可擴展的,可以輕鬆適應各種需求。 Guzzle 支援 HTTP 1.1 協議,協助 PHP 開發者快速建立 API 用戶端和 Web 服務。
二、安裝Guzzle
在專案目錄下使用Composer 安裝Guzzle,可以使用以下指令:
composer require guzzlehttp/guzzle
三、使用Guzzle 發起HTTP 要求
- 發起GET 請求
以下是使用Guzzle 發起GET 請求的簡單範例:
use GuzzleHttpClient; $client = new Client(); $response = $client->get('http://httpbin.org/get'); $body = (string) $response->getBody(); print_r(json_decode($body));
上述程式碼中,我們首先建立了Guzzle 的Client 實例,然後使用get
方法發起GET 請求,請求位址為http://httpbin.org/get
,並將回應結果解析為JSON 格式輸出。
- 發起POST 請求
以下是使用Guzzle 發起POST 請求的範例:
use GuzzleHttpClient; $client = new Client(); $response = $client->post('http://httpbin.org/post', [ 'form_params' => [ 'username' => 'testuser', 'password' => 'testpassword', ] ]); $body = (string) $response->getBody(); print_r(json_decode($body));
上述程式碼中,我們使用post
方法發起POST 請求,請求位址為http://httpbin.org/post
,並在請求體中加入了username
和password
兩個參數,最後將響應結果解析為JSON 格式輸出。
- 發起PUT 請求
以下是使用Guzzle 發起PUT 請求的範例:
use GuzzleHttpClient; $client = new Client(); $response = $client->put('http://httpbin.org/put', [ 'json' => [ 'name' => 'testuser', 'age' => 18, ] ]); $body = (string) $response->getBody(); print_r(json_decode($body));
上述程式碼中,我們使用put
方法啟動PUT 請求,請求位址為http://httpbin.org/put
,並在請求體中加入了一個JSON 物件{ "name": "testuser", "age" : 18 }
,最後將回應結果解析為JSON 格式輸出。
- 發起DELETE 請求
以下是使用Guzzle 發起DELETE 請求的範例:
use GuzzleHttpClient; $client = new Client(); $response = $client->delete('http://httpbin.org/delete'); $body = (string) $response->getBody(); print_r(json_decode($body));
上述程式碼中,我們使用delete
方法發起DELETE 請求,請求位址為http://httpbin.org/delete
,最後將回應結果解析為JSON 格式輸出。
五、處理 Guzzle 回應
Guzzle 的 Response 物件提供了一些方法來取得回應的資料、狀態碼、回應頭等資訊。以下是部分範例:
use GuzzleHttpClient; $client = new Client(); $response = $client->get('http://httpbin.org/get'); // 获取响应体 $body = (string) $response->getBody(); // 获取响应状态码 $statusCode = $response->getStatusCode(); // 获取响应原因短语 $reasonPhrase = $response->getReasonPhrase(); // 获取响应头 $headers = $response->getHeaders(); // 获取响应内容类型 $contentType = $response->getHeaderLine('Content-Type');
六、使用Guzzle 管理會話
Guzzle 提供了一個CookieJar 類,可以對會話中的Cookie 進行管理,以下是範例:
use GuzzleHttpClient; use GuzzleHttpCookieCookieJar; $client = new Client([ 'cookies' => true, ]); $cookieJar = new CookieJar(); $client->get('http://httpbin.org/cookies/set', [ 'query' => [ 'name' => 'testcookie', 'value' => 'testvalue', ], 'cookies' => $cookieJar, ]); $client->get('http://httpbin.org/cookies', [ 'cookies' => $cookieJar, ]); print_r($cookieJar->toArray());
在上述程式碼中,我們使用$client
建立了一個Guzzle 的Client 實例,並在建構函式中啟用了Cookie 自動管理功能,然後透過建立一個CookieJar 實例來管理Cookie。接下來,我們使用$client
發起兩次GET 請求,第一次請求將Cookie testcookie
的值設定為testvalue
,第二次請求取得Cookie資訊並將其輸出。
七、總結
Guzzle 是一個非常強大的 HTTP 用戶端程式庫,可以幫助 PHP 開發者快速且方便地發起 HTTP 請求、處理回應和管理會話。透過學習本文介紹的方法,相信讀者已經對 Guzzle 的基本原理和使用方法有了初步的了解,可以在實際專案中應用和擴展。
以上是使用PHP和Guzzle發起HTTP請求的詳細內容。更多資訊請關注PHP中文網其他相關文章!
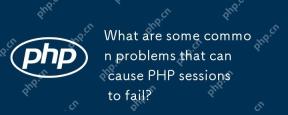
PHPSession失效的原因包括配置錯誤、Cookie問題和Session過期。 1.配置錯誤:檢查並設置正確的session.save_path。 2.Cookie問題:確保Cookie設置正確。 3.Session過期:調整session.gc_maxlifetime值以延長會話時間。
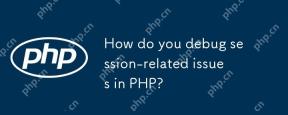
在PHP中調試會話問題的方法包括:1.檢查會話是否正確啟動;2.驗證會話ID的傳遞;3.檢查會話數據的存儲和讀取;4.查看服務器配置。通過輸出會話ID和數據、查看會話文件內容等方法,可以有效診斷和解決會話相關的問題。
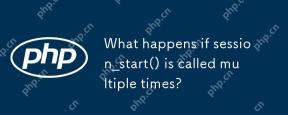
多次調用session_start()會導致警告信息和可能的數據覆蓋。 1)PHP會發出警告,提示session已啟動。 2)可能導致session數據意外覆蓋。 3)使用session_status()檢查session狀態,避免重複調用。
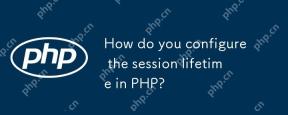
在PHP中配置會話生命週期可以通過設置session.gc_maxlifetime和session.cookie_lifetime來實現。 1)session.gc_maxlifetime控制服務器端會話數據的存活時間,2)session.cookie_lifetime控制客戶端cookie的生命週期,設置為0時cookie在瀏覽器關閉時過期。
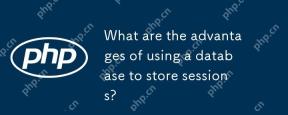
使用數據庫存儲會話的主要優勢包括持久性、可擴展性和安全性。 1.持久性:即使服務器重啟,會話數據也能保持不變。 2.可擴展性:適用於分佈式系統,確保會話數據在多服務器間同步。 3.安全性:數據庫提供加密存儲,保護敏感信息。
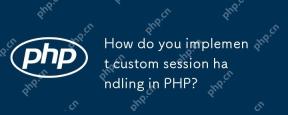
在PHP中實現自定義會話處理可以通過實現SessionHandlerInterface接口來完成。具體步驟包括:1)創建實現SessionHandlerInterface的類,如CustomSessionHandler;2)重寫接口中的方法(如open,close,read,write,destroy,gc)來定義會話數據的生命週期和存儲方式;3)在PHP腳本中註冊自定義會話處理器並啟動會話。這樣可以將數據存儲在MySQL、Redis等介質中,提升性能、安全性和可擴展性。
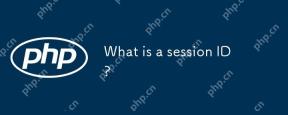
SessionID是網絡應用程序中用來跟踪用戶會話狀態的機制。 1.它是一個隨機生成的字符串,用於在用戶與服務器之間的多次交互中保持用戶的身份信息。 2.服務器生成並通過cookie或URL參數發送給客戶端,幫助在用戶的多次請求中識別和關聯這些請求。 3.生成通常使用隨機算法保證唯一性和不可預測性。 4.在實際開發中,可以使用內存數據庫如Redis來存儲session數據,提升性能和安全性。
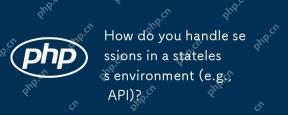
在無狀態環境如API中管理會話可以通過使用JWT或cookies來實現。 1.JWT適合無狀態和可擴展性,但大數據時體積大。 2.Cookies更傳統且易實現,但需謹慎配置以確保安全性。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中
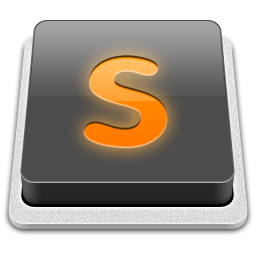
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

記事本++7.3.1
好用且免費的程式碼編輯器
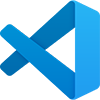
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器