隨著資料庫安全問題的日益凸顯,對資料進行加密處理已成為必要的措施。而Go語言的高效性和簡潔性,使其備受關注,特別是在Web開發領域中被廣泛應用。本文將介紹如何使用Go語言實作MySQL資料庫中資料欄位的加密處理。
一、MySQL資料庫欄位加密的意義
在現代資訊化的時代背景下,資料庫系統已變得越來越重要。然而,由於威脅不斷增加,資料庫安全成為企業和組織面臨的主要挑戰。一些研究表明,資料庫攻擊和資料外洩已成為業務最大的安全風險之一。因此,資料加密成為解決這個問題的必要途徑之一。
資料庫欄位加密的意義在於,保護資料庫中的敏感訊息,如使用者的姓名、電話號碼、電子郵件地址、密碼等,防範駭客攻擊和資料外洩。透過加密這些敏感數據,可以在資料被取得時抵禦駭客的資料遍歷和資訊竊取。
二、Go語言實作MySQL資料庫資料欄位加密的方法
Go語言是一種高效率、輕量、編譯型、開源的程式語言,廣泛用於Web開發。我們可以使用Go語言中的函式庫來加密和解密MySQL資料庫中的資料欄位。這裡我們使用Go語言的GORM函式庫。
GORM是一款優秀的Go語言ORM函式庫,它提供了程式碼優先的資料庫存取方式,支援多種資料庫,包括MySQL、SQLite、PostgreSQL、SQL Server等。我們可以透過對GORM函式庫的使用輕鬆實現MySQL資料庫的加密處理。
- 匯入依賴套件
開啟Go語言的開發環境,匯入需要使用的依賴套件:
import ( "crypto/aes" "crypto/cipher" "encoding/base64" "fmt" "gorm.io/driver/mysql" "gorm.io/gorm" )
- 資料庫連線
使用GORM的Open函數來連接資料庫,程式碼如下:
dsn := "user:password@tcp(127.0.0.1:3306)/database_name?charset=utf8mb4&parseTime=True&loc=Local" db, err := gorm.Open(mysql.Open(dsn), &gorm.Config{})
- #記錄加密和解密的金鑰
var key = []byte("the-key-has-to-be-32-bytes-long!")
- 定義加密和解密函數
func encrypt(data []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } plaintext := padData(data) // The IV needs to be unique, but not secure. Therefore it's common to // include it at the beginning of the ciphertext. ciphertext := make([]byte, aes.BlockSize+len(plaintext)) iv := ciphertext[:aes.BlockSize] if _, err := rand.Read(iv); err != nil { return nil, err } mode := cipher.NewCBCEncrypter(block, iv) mode.CryptBlocks(ciphertext[aes.BlockSize:], plaintext) return []byte(base64.StdEncoding.EncodeToString(ciphertext)), nil }解密函數程式碼如下:
func decrypt(data []byte) ([]byte, error) { block, err := aes.NewCipher(key) if err != nil { return nil, err } ciphertext, err := base64.StdEncoding.DecodeString(string(data)) if err != nil { return nil, err } if len(ciphertext) < aes.BlockSize { return nil, fmt.Errorf("ciphertext too short") } iv := ciphertext[:aes.BlockSize] ciphertext = ciphertext[aes.BlockSize:] // CBC mode always works in whole blocks. if len(ciphertext)%aes.BlockSize != 0 { return nil, fmt.Errorf("ciphertext is not a multiple of the block size") } mode := cipher.NewCBCDecrypter(block, iv) mode.CryptBlocks(ciphertext, ciphertext) return unpadData(ciphertext), nil }
- #範例:在MySQL資料庫中新增一個加密欄位
type User struct { ID uint Name string Email string Passwd []byte `gorm:"column:passwd"` }接下來,在模型中重寫表名和加密欄位的寫入和讀取方法,程式碼如下:
func (u *User) TableName() string { return "users" } func (u *User) BeforeSave(tx *gorm.DB) (err error) { pData, err := encrypt(u.Passwd) if err != nil { return } u.Passwd = pData return } func (u *User) AfterFind(tx *gorm.DB) (err error) { pData, err := decrypt(u.Passwd) if err != nil { return } u.Passwd = pData return }在BeforeSave()方法中,將用戶密碼加密並儲存。在AfterFind()方法中,將儲存的加密密碼解密並傳回。這樣我們就可以在MySQL資料庫中儲存加密的密碼欄位了。
- 範例:查詢MySQL資料庫中的加密欄位
users := []User{} result := db.Find(&users) if result.Error != nil { panic(result.Error) } for _, user := range users { fmt.Println(user) }在上面的範例中,我們查詢所有的使用者記錄並將傳回的結果印到控制台。當呼叫Find()函數時,GORM會自動執行AfterFind()方法對結果進行解密。 三、總結在本文中,我們介紹了使用Go語言和GORM函式庫實作MySQL資料庫中欄位加密的方法,主要步驟包括連接資料庫、定義加密和解密函數、將加密的欄位寫入表中以及查詢資料時的解密操作。透過這些操作,我們可以輕鬆地加密並保護MySQL資料庫中的敏感資訊。
以上是使用Go語言進行MySQL資料庫的資料欄位加密的方法的詳細內容。更多資訊請關注PHP中文網其他相關文章!
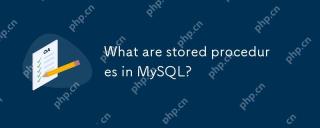
存儲過程是MySQL中的預編譯SQL語句集合,用於提高性能和簡化複雜操作。 1.提高性能:首次編譯後,後續調用無需重新編譯。 2.提高安全性:通過權限控制限制數據表訪問。 3.簡化複雜操作:將多條SQL語句組合,簡化應用層邏輯。
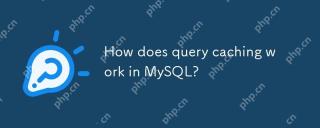
MySQL查詢緩存的工作原理是通過存儲SELECT查詢的結果,當相同查詢再次執行時,直接返回緩存結果。 1)查詢緩存提高數據庫讀取性能,通過哈希值查找緩存結果。 2)配置簡單,在MySQL配置文件中設置query_cache_type和query_cache_size。 3)使用SQL_NO_CACHE關鍵字可以禁用特定查詢的緩存。 4)在高頻更新環境中,查詢緩存可能導致性能瓶頸,需通過監控和調整參數優化使用。
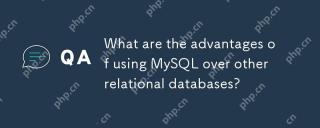
MySQL被廣泛應用於各種項目中的原因包括:1.高性能與可擴展性,支持多種存儲引擎;2.易於使用和維護,配置簡單且工具豐富;3.豐富的生態系統,吸引大量社區和第三方工具支持;4.跨平台支持,適用於多種操作系統。
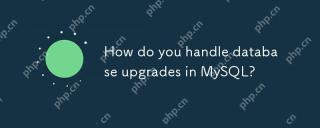
MySQL數據庫升級的步驟包括:1.備份數據庫,2.停止當前MySQL服務,3.安裝新版本MySQL,4.啟動新版本MySQL服務,5.恢復數據庫。升級過程需注意兼容性問題,並可使用高級工具如PerconaToolkit進行測試和優化。
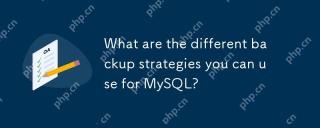
MySQL備份策略包括邏輯備份、物理備份、增量備份、基於復制的備份和雲備份。 1.邏輯備份使用mysqldump導出數據庫結構和數據,適合小型數據庫和版本遷移。 2.物理備份通過複製數據文件,速度快且全面,但需數據庫一致性。 3.增量備份利用二進制日誌記錄變化,適用於大型數據庫。 4.基於復制的備份通過從服務器備份,減少對生產系統的影響。 5.雲備份如AmazonRDS提供自動化解決方案,但成本和控制需考慮。選擇策略時應考慮數據庫大小、停機容忍度、恢復時間和恢復點目標。
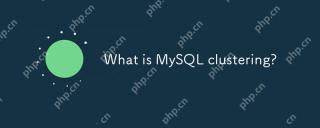
MySQLclusteringenhancesdatabaserobustnessandscalabilitybydistributingdataacrossmultiplenodes.ItusestheNDBenginefordatareplicationandfaulttolerance,ensuringhighavailability.Setupinvolvesconfiguringmanagement,data,andSQLnodes,withcarefulmonitoringandpe
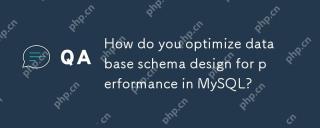
在MySQL中優化數據庫模式設計可通過以下步驟提升性能:1.索引優化:在常用查詢列上創建索引,平衡查詢和插入更新的開銷。 2.表結構優化:通過規範化或反規範化減少數據冗餘,提高訪問效率。 3.數據類型選擇:使用合適的數據類型,如INT替代VARCHAR,減少存儲空間。 4.分區和分錶:對於大數據量,使用分區和分錶分散數據,提升查詢和維護效率。
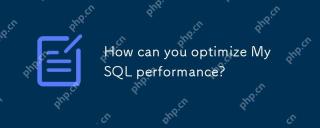
tooptimizemysqlperformance,lofterTheSeSteps:1)inasemproperIndexingTospeedUpqueries,2)使用ExplaintplaintoAnalyzeandoptimizequeryPerformance,3)ActiveServerConfigurationStersLikeTlikeTlikeTlikeIkeLikeIkeIkeLikeIkeLikeIkeLikeIkeLikeNodb_buffer_pool_sizizeandmax_connections,4)


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

記事本++7.3.1
好用且免費的程式碼編輯器
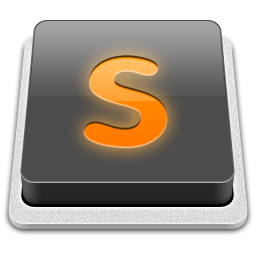
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。