一、什麼是字典樹
字典樹(Trie),也叫做前綴樹(Prefix Tree),是一種樹狀資料結構。字典樹可以對字串進行高效率的尋找、插入、刪除操作。其核心思想是利用字串的公共前綴來降低查詢時間的複雜度。
在字典樹中,每個節點都代表一個字串的前綴。從根節點到葉節點組成的路徑代表一個完整的字串。路徑上的每個節點都有一個標誌用來表示該節點所代表的字串是否存在於字典樹中。
二、字典樹的實作
在Python中,可以使用字典(dict)來實作字典樹。在字典樹中,每個節點都是一個字典,用來儲存下一個字元及其對應的節點。當需要遍歷字典樹時,只需要根據當前字元找到對應的節點,然後進入下一個字元對應的節點,如此遞歸下去直到字串結束或無法匹配為止。
以下是一個簡單的字典樹實作:
class TrieNode: def __init__(self): self.children = {} self.is_word = False class Trie: def __init__(self): self.root = TrieNode() def insert(self, word): curr = self.root for ch in word: if ch not in curr.children: curr.children[ch] = TrieNode() curr = curr.children[ch] curr.is_word = True def search(self, word): curr = self.root for ch in word: if ch not in curr.children: return False curr = curr.children[ch] return curr.is_word def starts_with(self, prefix): curr = self.root for ch in prefix: if ch not in curr.children: return False curr = curr.children[ch] return True
三、字典樹的應用
字典樹可以用於文字匹配,如單字拼字檢查、單字匹配等。以下是一個簡單的例子,用字典樹來實現單字拼字檢查:
import re word_list = ['hello', 'world', 'python', 'teacher', 'student'] def sanitize_word(word): return re.sub(r'[^a-z]', '', word.lower()) def spell_check(word): trie = Trie() for w in word_list: trie.insert(sanitize_word(w)) if trie.search(sanitize_word(word)): print('Correct spelling!') else: print('Did you mean one of the following words?') similar_words = get_similar_words(trie, sanitize_word(word)) for w in similar_words: print(w) def get_similar_words(trie, word, distance=1): similar_words = [] for i in range(len(word)): for ch in range(ord('a'), ord('z')+1): new_word = word[:i] + chr(ch) + word[i+1:] if trie.search(new_word): similar_words.append(new_word) return similar_words spell_check('helo')
在上面的程式碼中,我們可以透過字典樹來檢查單字是否存在於單字列表中。如果單字存在,則輸出「Correct spelling!」;否則輸出相似的單字。
四、總結
字典樹是一種非常實用的資料結構,可以用來有效率地進行文字比對。 Python中可以使用字典來實作字典樹,非常簡單易懂。在實際應用中,可以根據實際需求進行調整和擴展,以達到更好的效果。
以上是如何在Python中使用字典樹進行文字比對?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
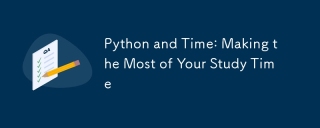
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
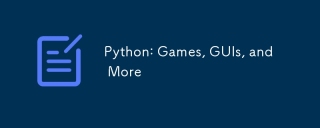
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
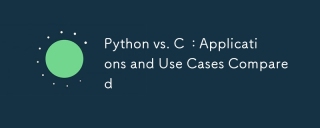
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
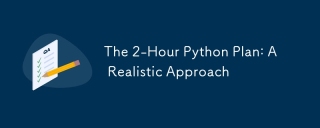
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
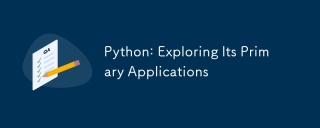
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
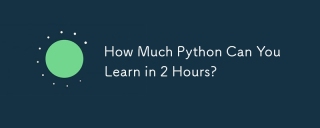
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
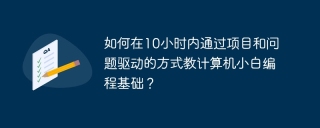
如何在10小時內教計算機小白編程基礎?如果你只有10個小時來教計算機小白一些編程知識,你會選擇教些什麼�...
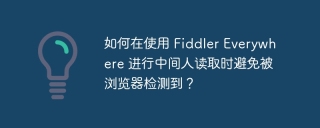
使用FiddlerEverywhere進行中間人讀取時如何避免被檢測到當你使用FiddlerEverywhere...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
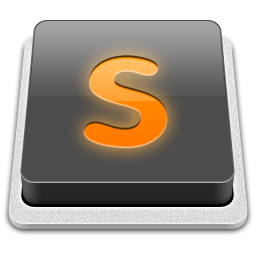
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具
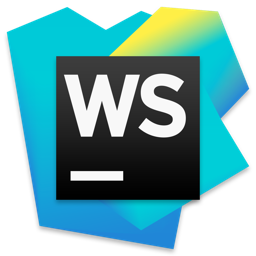
WebStorm Mac版
好用的JavaScript開發工具

Atom編輯器mac版下載
最受歡迎的的開源編輯器
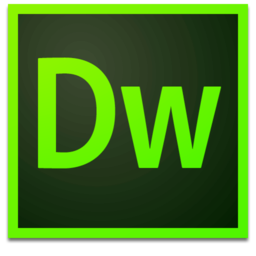
Dreamweaver Mac版
視覺化網頁開發工具