Node.js是一種基於事件驅動的JavaScript運行環境,常用來開發高效能、可擴展的網路應用程式。在許多場景下,我們需要向不同的API或資料來源發送多個請求,並且需要確保這些請求的順序。本文將介紹多個請求保持順序的三種方法。
1. 使用回呼函數
在Node.js中,回呼函數是非同步程式設計的核心。在多個請求中,我們可以將一個請求的回呼函數作為另一個請求的回呼函數,從而確保它們的順序。
例如,我們要傳送三個HTTP請求,分別是取得使用者資訊、取得使用者訂單、取得使用者地址。這三個請求需要按照順序執行,因為後面的請求需要依賴前面請求的資料。
getUserInfo(userId, function(err, userInfo) { if (err) throw err; getUserOrder(userId, function(err, userOrder) { if (err) throw err; getUserAddress(userId, function(err, userAddress) { if (err) throw err; // 处理获取到的用户信息、订单和地址 console.log(userInfo, userOrder, userAddress); }); }); });
在上面的程式碼中,getUserInfo、getUserOrder和getUserAddress都是非同步的HTTP請求,並且它們的回呼函數作為另一個請求的回呼函數。透過這種方式,我們可以保證請求的順序,並且在每個請求完成後處理對應的資料。
2. 使用async/await
在ES2017標準中,JavaScript引入了async/await語法,它是一種基於Promise的非同步程式設計方式。透過使用async/await,我們可以讓非同步程式碼看起來像同步程式碼,從而更容易管理多個非同步請求的順序。
async function getUserInfo(userId) { const response = await fetch(`/api/user/${userId}/info`); const userInfo = await response.json(); return userInfo; } async function getUserOrder(userId) { const response = await fetch(`/api/user/${userId}/order`); const userOrder = await response.json(); return userOrder; } async function getUserAddress(userId) { const response = await fetch(`/api/user/${userId}/address`); const userAddress = await response.json(); return userAddress; } async function getUserData(userId) { const userInfo = await getUserInfo(userId); const userOrder = await getUserOrder(userId); const userAddress = await getUserAddress(userId); return { userInfo, userOrder, userAddress }; } getUserData(userId) .then(data => { // 处理获取到的用户信息、订单和地址 console.log(data.userInfo, data.userOrder, data.userAddress); }) .catch(error => { console.error(error); });
在上面的程式碼中,getUserInfo、getUserOrder和getUserAddress都是傳回Promise的非同步請求。透過使用async/await,我們可以透過簡單的順序編寫程式碼來確保它們的順序。 getUserData函數是一個包含三個非同步請求的高層函數,它會取得使用者資料並傳回一個包含使用者資訊、訂單和地址的物件。 Promise物件的then方法用於處理傳回的數據,catch方法則用於處理錯誤。
3. 使用Promise.all和陣列解構語法
Promise.all方法是Promise API提供的一種方式,可用於並行地執行多個非同步請求,並在它們完成時返回結果。和async/await結合使用時,我們可以使用數組解構語法將傳回的結果解構為一個數組,從而更容易處理多個請求的結果。
const userInfoPromise = fetch(`/api/user/${userId}/info`).then(response => response.json()); const userOrderPromise = fetch(`/api/user/${userId}/order`).then(response => response.json()); const userAddressPromise = fetch(`/api/user/${userId}/address`).then(response => response.json()); Promise.all([userInfoPromise, userOrderPromise, userAddressPromise]) .then(([userInfo, userOrder, userAddress]) => { // 处理获取到的用户信息、订单和地址 console.log(userInfo, userOrder, userAddress); }) .catch(error => { console.error(error); });
在上面的程式碼中,我們使用fetch函數取得使用者資訊、訂單和地址,並將它們分別封裝成一個Promise物件。然後,我們使用Promise.all方法並行地執行這三個Promise,並將它們的結果解構為一個陣列。和上面的方法一樣,Promise物件的then方法用於處理傳回的數據,catch方法則用於處理錯誤。
透過使用回呼函數、async/await和Promise.all,我們可以輕鬆地管理多個非同步請求的順序,並處理它們的結果。根據不同的場景和需求,我們可以選擇最適合的方式來寫Node.js程式碼。
以上是nodejs多個請求保持順序的詳細內容。更多資訊請關注PHP中文網其他相關文章!

HTML和React的關係是前端開發的核心,它們共同構建現代Web應用的用戶界面。 1)HTML定義內容結構和語義,React通過組件化構建動態界面。 2)React組件使用JSX語法嵌入HTML,實現智能渲染。 3)組件生命週期管理HTML渲染,根據狀態和屬性動態更新。 4)使用組件優化HTML結構,提高可維護性。 5)性能優化包括避免不必要渲染,使用key屬性,保持組件單一職責。

React是構建交互式前端體驗的首選工具。 1)React通過組件化和虛擬DOM簡化UI開發。 2)組件分為函數組件和類組件,函數組件更簡潔,類組件提供更多生命週期方法。 3)React的工作原理依賴虛擬DOM和調和算法,提高性能。 4)狀態管理使用useState或this.state,生命週期方法如componentDidMount用於特定邏輯。 5)基本用法包括創建組件和管理狀態,高級用法涉及自定義鉤子和性能優化。 6)常見錯誤包括狀態更新不當和性能問題,調試技巧包括使用ReactDevTools和優

React是一個用於構建用戶界面的JavaScript庫,其核心是組件化和狀態管理。 1)通過組件化和狀態管理簡化UI開發。 2)工作原理包括調和和渲染,優化可通過React.memo和useMemo實現。 3)基本用法是創建並渲染組件,高級用法包括使用Hooks和ContextAPI。 4)常見錯誤如狀態更新不當,可使用ReactDevTools調試。 5)性能優化包括使用React.memo、虛擬化列表和CodeSplitting,保持代碼可讀性和可維護性是最佳實踐。

React通過JSX與HTML結合,提升用戶體驗。 1)JSX嵌入HTML,使開發更直觀。 2)虛擬DOM機制優化性能,減少DOM操作。 3)組件化管理UI,提高可維護性。 4)狀態管理和事件處理增強交互性。

React組件可以通過函數或類定義,封裝UI邏輯並通過props接受輸入數據。 1)定義組件:使用函數或類,返回React元素。 2)渲染組件:React調用render方法或執行函數組件。 3)復用組件:通過props傳遞數據,構建複雜UI。組件的生命週期方法允許在不同階段執行邏輯,提升開發效率和代碼可維護性。
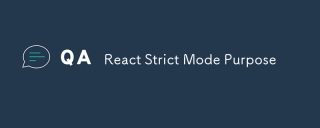
React嚴格模式是一種開發工具,可通過激活其他檢查和警告來突出反應應用中的潛在問題。它有助於識別遺產代碼,不安全的生命週期和副作用,鼓勵現代反應實踐。
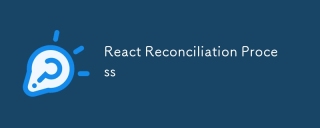
本文討論了React的對帳過程,詳細介紹了它如何有效地更新DOM。關鍵步驟包括觸發對帳,創建虛擬DOM,使用擴散算法以及應用最小的DOM更新。它還覆蓋了經家


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
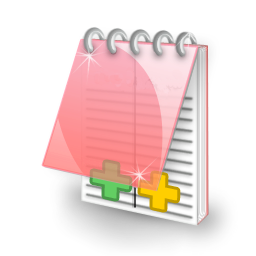
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

記事本++7.3.1
好用且免費的程式碼編輯器

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。
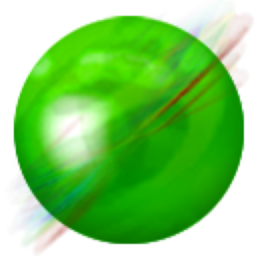
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境