SpringBoot啟動過程簡介
SpringBoot應用程式的啟動過程可以分為以下步驟:
載入應用程式上下文
掃描應用程式中的所有元件
自動配置應用程式環境
啟動嵌入式網路伺服器
#載入應用程式上下文
一個包含SpringBoot 應用程式所有元件的容器就是它的上下文。在啟動過程中,SpringBoot 會載入並初始化這個容器。
這個步驟的原始碼在SpringApplication
類別中。具體來說,SpringApplication
類別的run
#方法是這個過程的入口點。在這個方法中,Spring Boot會透過呼叫createApplicationContext
方法來建立應用程式上下文。
下面是createApplicationContext
方法的原始程式碼:
protected ConfigurableApplicationContext createApplicationContext() { Class<?> contextClass = this.applicationContextClass; if (contextClass == null) { try { switch (this.webApplicationType) { case SERVLET: contextClass = Class.forName(DEFAULT_SERVLET_WEB_CONTEXT_CLASS); break; case REACTIVE: contextClass = Class.forName(DEFAULT_REACTIVE_WEB_CONTEXT_CLASS); break; default: contextClass = Class.forName(DEFAULT_CONTEXT_CLASS); } } catch (ClassNotFoundException ex) { throw new IllegalStateException( "Unable to create a default ApplicationContext, " + "please specify an ApplicationContextClass", ex); } } return (ConfigurableApplicationContext) BeanUtils.instantiateClass(contextClass); }
在這個方法中,SpringBoot 會根據應用程式類型(Servlet或Reactive)選擇合適的上下文類別。接著,使用 Java 反射機制來實例化該類別並傳回一個可配置的應用程式上下文物件。
掃描應用程式中的所有元件
在上一個步驟中,SpringBoot創建了應用程式上下文。在此階段,SpringBoot會掃描應用程式中的所有元件並將它們註冊到應用程式上下文中。
這個步驟的原始碼在SpringApplication
類別中的scan
方法中。具體來說,在這個方法中,SpringBoot 會建立一個SpringBootBeanDefinitionScanner
對象,並使用它來掃描應用程式中的所有元件。
下面是scan
方法的原始程式碼:
private void scan(String... basePackages) { if (ObjectUtils.isEmpty(basePackages)) { return; } ClassPathScanningCandidateComponentProvider scanner = new ClassPathScanningCandidateComponentProvider( this.includeFilters, this.excludeFilters, this.resourceLoader); scanner.setResourceLoader(this.resourceLoader); scanner.setEnvironment(this.environment); scanner.setIncludeAnnotationConfig(this.useAnnotatedConfig); scanner.addExcludeFilter(new AbstractTypeHierarchyTraversingFilter(false, false) { @Override protected boolean matchClassName(String className) { return getExcludeClassNames().contains(className); } }); for (String basePackage : basePackages) { scanner.findCandidateComponents(basePackage).forEach(this.componentDefinitions::add); } }
在這個方法中,SpringBoot 會建立一個ClassPathScanningCandidateComponentProvider
對象,並使用它來掃描應用程式中的所有元件。這個物件會掃描指定套件路徑下的所有類別,並將它們轉換為 Spring 的 Bean 定義。這些 Bean 定義將會註冊到應用程式上下文中。
自動配置應用程式環境
SpringBoot在前一步將應用程式中的所有元件註冊到應用程式上下文中。 SpringBoot會自動配置應用程式環境,其中包括資料來源、事務管理器和JPA配置。
這個步驟的原始碼在SpringApplication
類別中的configureEnvironment
方法中。在這個方法中,Spring Boot會建立一個SpringApplicationRunListeners
對象,並使用它來配置應用程式環境。
下面是configureEnvironment
方法的原始程式碼:
private void configureEnvironment(ConfigurableEnvironment environment, String[] args) { if (this.addCommandLineProperties) { ApplicationArguments applicationArguments = new DefaultApplicationArguments(args); environment.getPropertySources().addLast(new CommandLinePropertySource(applicationArguments)); } this.listeners.environmentPrepared(environment); if (this.logStartupInfo) { this.logStartupInfo(environment); } ConfigurationPropertySources.attach(environment); Binder.get(environment).bind(ConfigurationPropertyName.EMPTY, Bindable.ofInstance(this.sources)); if (!this.isCustomEnvironment) { EnvironmentConverter.configureEnvironment(environment, this.deduceEnvironmentClass()); } this.listeners.environmentPrepared(environment); }
在這個方法中,SpringBoot 會建立一個ApplicationArguments
對象,並將其轉換為一個命令列屬性來源。然後,它會呼叫listeners
中的environmentPrepared
方法來通知應用程式環境已經準備好了。隨後,SpringBoot 會綁定屬性來源到應用程式環境中,並呼叫listeners
中的environmentPrepared
方法來通知應用程式環境已經準備好了。
啟動嵌入式Web伺服器
在前一步驟中,SpringBoot已自動完成了應用程式環境的設定。在這一步驟,SpringBoot將啟動內嵌式Web伺服器,以便應用程式提供Web服務。
這個步驟的原始碼在SpringApplication
類別中的run
方法中。具體來說,在這個方法中,SpringBoot 會根據應用程式類型(Servlet或Reactive)選擇合適的嵌入式Web伺服器,並使用它來啟動應用程式。
下面是run
方法的原始程式碼:
public ConfigurableApplicationContext run(String... args) { StopWatch stopWatch = new StopWatch(); stopWatch.start(); ConfigurableApplicationContext context = null; Collection<SpringBootExceptionReporter> exceptionReporters = new ArrayList<>(); configureHeadlessProperty(); SpringApplicationRunListeners listeners = getRunListeners(args); listeners.starting(); try { ApplicationArguments applicationArguments = new DefaultApplicationArguments(args); ConfigurableEnvironment environment = prepareEnvironment(listeners, applicationArguments); configureIgnoreBeanInfo(environment); Banner printedBanner = printBanner(environment); context = createApplicationContext(); exceptionReporters = getSpringFactoriesInstances( SpringBootExceptionReporter.class, new Class[] { ConfigurableApplicationContext.class }, context); prepareContext(context, environment, listeners, applicationArguments, printedBanner); refreshContext(context); afterRefresh(context, applicationArguments); stopWatch.stop(); if (this.logStartupInfo) { new StartupInfoLogger(this.mainApplicationClass).logStarted(getApplicationLog(), stopWatch); } listeners.started(context); callRunners(context, applicationArguments); } catch (Throwable ex) { handleRunFailure(context, ex, exceptionReporters, listeners); throw new IllegalStateException(ex); } try { listeners.running(context); } catch (Throwable ex) { handleRunFailure(context, ex, exceptionReporters, null); throw new IllegalStateException(ex); } return context; }
在這個方法中,SpringBoot 會使用一個StopWatch
物件來計算應用程式啟動時間。然後,它會呼叫listeners
中的starting
方法來通知應用程式即將啟動。接著,SpringBoot 會準備應用程式環境,並使用它來建立應用程式上下文。隨後,SpringBoot 會呼叫listeners
中的started
方法來通知應用程式已經啟動。最後,SpringBoot 會呼叫callRunners
方法來運行所有的CommandLineRunner
和ApplicationRunner
元件。
以上是SpringBoot啟動流程是什麼的詳細內容。更多資訊請關注PHP中文網其他相關文章!
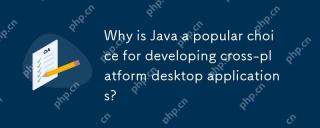
javaispopularforcross-platformdesktopapplicationsduetoits“ writeonce,runany where”哲學。 1)itusesbytiesebyTecodeThatrunsonAnyJvm-備用Platform.2)librarieslikeslikeslikeswingingandjavafxhelpcreatenative-lookingenative-lookinguisis.3)
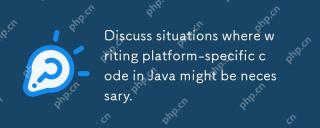
在Java中編寫平台特定代碼的原因包括訪問特定操作系統功能、與特定硬件交互和優化性能。 1)使用JNA或JNI訪問Windows註冊表;2)通過JNI與Linux特定硬件驅動程序交互;3)通過JNI使用Metal優化macOS上的遊戲性能。儘管如此,編寫平台特定代碼會影響代碼的可移植性、增加複雜性、可能帶來性能開銷和安全風險。
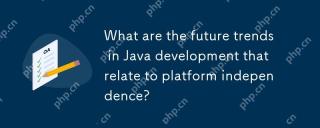
Java將通過雲原生應用、多平台部署和跨語言互操作進一步提昇平台獨立性。 1)雲原生應用將使用GraalVM和Quarkus提升啟動速度。 2)Java將擴展到嵌入式設備、移動設備和量子計算機。 3)通過GraalVM,Java將與Python、JavaScript等語言無縫集成,增強跨語言互操作性。
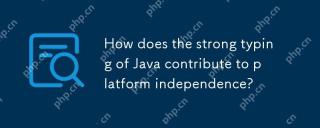
Java的強類型系統通過類型安全、統一的類型轉換和多態性確保了平台獨立性。 1)類型安全在編譯時進行類型檢查,避免運行時錯誤;2)統一的類型轉換規則在所有平台上一致;3)多態性和接口機制使代碼在不同平台上行為一致。
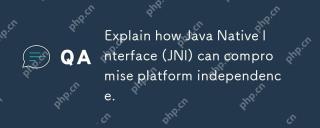
JNI會破壞Java的平台獨立性。 1)JNI需要特定平台的本地庫,2)本地代碼需在目標平台編譯和鏈接,3)不同版本的操作系統或JVM可能需要不同的本地庫版本,4)本地代碼可能引入安全漏洞或導致程序崩潰。
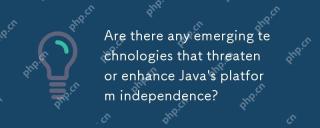
新興技術對Java的平台獨立性既有威脅也有增強。 1)雲計算和容器化技術如Docker增強了Java的平台獨立性,但需要優化以適應不同雲環境。 2)WebAssembly通過GraalVM編譯Java代碼,擴展了其平台獨立性,但需與其他語言競爭性能。
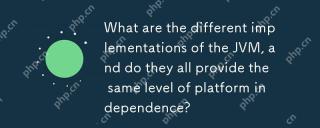
不同JVM實現都能提供平台獨立性,但表現略有不同。 1.OracleHotSpot和OpenJDKJVM在平台獨立性上表現相似,但OpenJDK可能需額外配置。 2.IBMJ9JVM在特定操作系統上表現優化。 3.GraalVM支持多語言,需額外配置。 4.AzulZingJVM需特定平台調整。
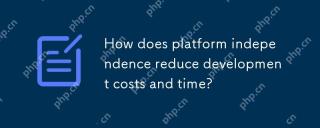
平台獨立性通過在多種操作系統上運行同一套代碼,降低開發成本和縮短開發時間。具體表現為:1.減少開發時間,只需維護一套代碼;2.降低維護成本,統一測試流程;3.快速迭代和團隊協作,簡化部署過程。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3 Linux新版
SublimeText3 Linux最新版

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。