舉例
有了 ini 和 yaml,相信 toml 學習來也很簡單,先直接看一個例子吧。
import toml config = """ title = "toml 小栗子" [owner] name = "古明地觉" age = 17 place = "东方地灵殿" nickname = ["小五", "少女觉", "觉大人"] [database] host = "127.0.0.1" port = 5432 username = "satori" password = "123456" echo = true [server] [server.v1] api = "1.1" enable = false [server.v2] api = "1.2" enable = true [client] client = [ ["socket", "webservice"], [5555] ] address = [ "xxxx", "yyyy" ] """ # loads:从字符串加载 # load:从文件加载 # dumps:生成 toml 格式字符串 # dump:生成 toml 格式字符串并写入文件中 data = toml.loads(config) print(data) """ { 'title': 'toml 小栗子', 'owner': {'name': '古明地觉', 'age': 17, 'place': '东方地灵殿', 'nickname': ['小五', '少女觉', '觉大人']}, 'database': {'host': '127.0.0.1', 'port': 5432, 'username': 'satori', 'password': '123456', 'echo': True}, 'server': {'v1': {'api': '1.1', 'enable': False}, 'v2': {'api': '1.2', 'enable': True}}, 'client': {'client': [['socket', 'webservice'], [5555]], 'address': ['xxxx', 'yyyy']} } """
toml 是採用 var = value 的形式來配置,然後也有類似 ini 裡面的 section,每個 section 都是字典中的一個 key,然後該 key 也對應一個字典。由於缺乏section,我們需要注意到最初的標題,因此它是一個獨立的鍵。
而且還有一點就是 toml 支援巢狀,我們看到 server.v1,表示 v1 是 server 對應的字典裡面的一個 key,然後 v1 對應的值還是一個字典。
toml 變得更簡單了,而且寫來也非常像Python,它有以下特點:
toml 檔案是大小寫敏感的;
toml 檔案必須是有效的UTF-8 編碼的Unicode 文件;
toml 檔案的空白符應該是Tab 或空格;
toml 檔案的換行是LF 或CRLF;
#然後我們來介紹一下toml 的資料結構。
註釋
toml 採用# 表示註釋,舉個例子:
# 这是注释 key = "value" # 也是注释
可以解析一下看看會得到什麼,劇透:會得到只包含一個鍵值對的字典。
鍵值對
基本上構成區塊為鍵值對,在等號左側為鍵名,在右側為值,同時忽略鍵名和鍵值周圍的空白的TOML文件.此外鍵、等號和值必須在同一行(不過有些值可以跨多行)。
key = "value"
鍵名稱可以以裸鍵、引號鍵或點分隔鍵的形式出現。裸鍵只允許包含ASCII字元、數字、底線和連字號。
import toml config = """ key = "value" bare_key = "value" bare-key = "value" # 1234 会被当成字符串 1234 = "value" """ data = toml.loads(config) print(data) """ {'key': 'value', 'bare_key': 'value', 'bare-key': 'value', '1234': 'value'} """
如果不是裸鍵,那麼就必須使用引號括起來,但是此時也支援我們使用更廣泛的鍵名,但除了特殊場景,否則使用裸鍵是最佳實踐。
import toml config = """ "127.0.0.1" = "value" "character encoding" = "value" "ʎǝʞ" = "value" 'key2' = "value" 'quoted "value"' = "value" """ data = toml.loads(config) print(data) """ {'127.0.0.1': 'value', 'character encoding': 'value', 'ʎǝʞ': 'value', 'key2': 'value', 'quoted "value"': 'value'} """
注意:裸鍵不能為空,但空引號鍵是允許的(雖然不建議如此)。
= "没有键名" # 错误 "" = "空" # 正确但不鼓励 '' = '空' # 正确但不鼓励
然後是點分隔鍵,它是一系列透過點相連的裸鍵或引號鍵,這允許我們將相近屬性放在一起:
import toml config = """ name = "橙子" physical.color = "橙色" physical.shape = "圆形" site."google.com" = true site.google.com = true a.b.c.d = 123 """ data = toml.loads(config) print(data) """ { 'name': '橙子', 'physical': {'color': '橙色', 'shape': '圆形'}, 'site': {'google.com': True, 'google': {'com': True}}, 'a': {'b': {'c': {'d': 123}}} } """
我們看到這個點分隔符不錯喲,自動實作了巢狀結構,並且點分隔符號周圍的空白會被忽略。
fruit.name = "香蕉" # 这是最佳实践 fruit. color = "黄色" # 等同于 fruit.color fruit . flavor = "香蕉" # 等同于 fruit.flavor
注意:多次定義同一個鍵是不行的。
import toml config = """ # name 和 "name" 是等价的 name = "古明地觉" "name" = "古明地恋" """ try: data = toml.loads(config) except toml.decoder.TomlDecodeError as e: print(e) """ Duplicate keys! (line 4 column 1 char 36) """
對於點分隔鍵也是如此,只要一個鍵還沒有被直接定義過,我們就仍可以對它和它下屬的鍵名賦值。
import toml config = """ fruit.apple.smooth = true# 此时可以继续操作 fruit、fruit.apple,它们都是字典 # 给 fruit 这个字典加一个 key fruit.orange = 2 # 给 fruit.apple 加一个 key fruit.apple.color = "red" """ data = toml.loads(config) print(data) """ { 'fruit': {'apple': {'smooth': True, 'color': 'red'}, 'orange': 2} } """
但下面這個操作是不行的:
# 将 fruit.apple 的值定义为一个整数 fruit.apple = 1 # 但接下来就不合法了,因为整数不能变成字典 fruit.apple.smooth = true # 如果我们设置 fruit.apple = {},那么第二个赋值是可以的 # 没错,我们可以通过 {} 直接创建一个字典
可以看到,真的很像 Python。然後再來一個特例:
import toml config = """ 3.14 = "pi" "3.14" = "pi" """ data = toml.loads(config) print(data) """ {'3': {'14': 'pi'}, '3.14': 'pi'} """
如果鍵是浮點數,那麼需要使用引號括起來,否則會被解釋為點分隔鍵。
看完了鍵,再來看看值(value),其實對於 toml 來說,值比鍵要簡單的多得多。
字串
字串共有四種方式來表示:基礎式的,多行基礎式的,字面量式的,和多行字面量式的。
1)基礎字串由引號包裹,任何 Unicode 字元都可以使用,除了那些必須轉義的。
import toml config = """ str = '我是一个字符串,"你可以把我引起来"' """ data = toml.loads(config) print(data) """ {'str': '我是一个字符串,"你可以把我引起来"'} """
多行字串可以用三個引號括起來,可以包含換行。但是要注意,開頭引號之後的第一個換行會被移除,其他的空格和換行符會被保留。
import toml config = """ str = ''' 玫瑰是红色的 紫罗兰是蓝色的 ''' """ data = toml.loads(config) print(data) """ {'str': '玫瑰是红色的\n紫罗兰是蓝色的\n'} """
這裡的引號可以是雙引號、也可以是單引號。
整數
整數是純數字,正數可以有加號前綴,負數的前綴是減號。
import toml config = """ int1 = +99 int2 = 42 int3 = 0 int4 = -17 # 对于大数,可以在数字之间用下划线来增强可读性 # 每个下划线两侧必须至少有一个数字。 int5 = 1_000 int6 = 5_349_221 int7 = 53_49_221 # 印度记数体系分组 int8 = 1_2_3_4_5 # 无误但不鼓励 """ data = toml.loads(config) print(data) """ {'int1': 99, 'int2': 42, 'int3': 0, 'int4': -17, 'int5': 1000, 'int6': 5349221, 'int7': 5349221, 'int8': 12345} """
但是注意:數字不能以零開頭,除了 0 本身。無前綴的零、-0和 0是等效的。非負整數值也可以用十六進位、八進位或二進位來表示。
# 带有 `0x` 前缀的十六进制,大小写均可 hex1 = 0xDEADBEEF hex2 = 0xdeadbeef hex3 = 0xdead_beef # 带有 `0o` 前缀的八进制 oct1 = 0o01234567 oct2 = 0o755 # 对于表示 Unix 文件权限很有用 # 带有 `0b` 前缀的二进制 bin1 = 0b11010110
浮點數
一個浮點數可以由整數部分和小數部分組成,也可以由指數部分組成。整數部分和小數部分遵從與十進制整數值相同的規則。如果小數部分和指數部分兼有,那麼小數部分必須在指數部分前面。
import toml config = """ # 小数 flt1 = +1.0 flt2 = 3.1415 flt3 = -0.01 # 指数 flt4 = 5e+22 flt5 = 1e06 flt6 = -2E-2 flt7 = 6.626e-34 """ data = toml.loads(config) print(data) """ {'flt1': 1.0, 'flt2': 3.1415, 'flt3': -0.01, 'flt4': 5e+22, 'flt5': 1000000.0, 'flt6': -0.02, 'flt7': 6.626e-34} """
小數部分是一個小數點後面跟著一個或多個數字,一個指數部分是一個E(大小寫均可)後面跟著一個整數部分(遵從與十進制整數值相同的規則,但可以包含前導零)。小數點,如果有用到的話,每側必須緊鄰至少一個數字。
# 非法的浮点数 invalid_float_1 = .7 invalid_float_2 = 7. invalid_float_3 = 3.e+20
與整數相似,可以使用底線來增強可讀性,每個底線必須被至少一個數字圍繞。
flt8 = 224_617.445_991_228
浮點數值 -0.0 與 0.0 是有效的,且應遵循 IEEE 754。特殊浮點值也能夠表示:
# 无穷 sf1 = inf # 正无穷 sf2 = +inf # 正无穷 sf3 = -inf # 负无穷 # 非数 sf4 = nan # 是对应信号非数码还是静默非数码,取决于实现 sf5 = +nan # 等同于 `nan` sf6 = -nan # 正确,实际码取决于实现
布林值
布林值就是慣用的那樣,但要小寫。
bool1 = true bool2 = false
日期
可以是普通的 datetime,或是遵循 ISO-8859-1 格式的日期。
import toml config = """ dt1 = 2020-01-01T12:33:22+00:00 dt2 = 2020-11-12 12:11:33 dt3 = 2020-11-23 """ data = toml.loads(config) print(data) """ {'dt1': datetime.datetime(2020, 1, 1, 12, 33, 22, tzinfo=...), 'dt2': datetime.datetime(2020, 11, 12, 12, 11, 33), 'dt3': datetime.date(2020, 11, 23)} """
陣列
語法和 Python 的清單類似:
import toml config = """ # 每个数组里面的元素类型要一致 integers = [1, 2, 3] colors = ["红", "黄", "绿"] nested_array_of_ints = [[1, 2], [3, 4, 5]] nested_mixed_array = [[1, 2], ["a", "b", "c"]] numbers = [0.1, 0.2, 0.5] """ data = toml.loads(config) print(data) """ {'colors': ['红', '黄', '绿'], 'integers': [1, 2, 3], 'nested_array_of_ints': [[1, 2], [3, 4, 5]], 'nested_mixed_array': [[1, 2], ['a', 'b', 'c']], 'numbers': [0.1, 0.2, 0.5]} """
陣列可以跨行,陣列的最後一個值後面可以有終逗號(也稱為尾逗號)。
import toml config = """ integers2 = [ 1, 2, 3 ] integers3 = [ 1, 2, # 这是可以的 ] """ data = toml.loads(config) print(data) """ {'integers2': [1, 2, 3], 'integers3': [1, 2]} """
表
表,完全可以把它想像成 ini 的 section。
import toml config = """ # 表名的定义规则与键名相同 # 解析之后得到的大字典中就有 "table-1" 这个 key # 并且其 value 也是一个表,在它下方 # 直至下一个表头或文件结束,都是这个表内部的键值对 [table-1] key1 = "some string" key2 = 123 [table-2] key1 = "another string" key2 = 456 """ data = toml.loads(config) print(data) """ {'table-1': {'key1': 'some string', 'key2': 123}, 'table-2': {'key1': 'another string', 'key2': 456}} """
但是我們之前也實作過類似這種結構,沒錯,就是點分隔符號:
import toml config = """ # 所以 other-table-1 和 table-1 是等价的 # other-table-2 和 table-2 是等价的 other-table-1.key1 = "some string" other-table-1.key2 = 123 other-table-2.key1 = "another string" other-table-2.key2 = 456 [table-1] key1 = "some string" key2 = 123 [table-2] key1 = "another string" key2 = 456 """ data = toml.loads(config) print(data) """ {'other-table-1': {'key1': 'some string', 'key2': 123}, 'other-table-2': {'key1': 'another string', 'key2': 456}, 'table-1': {'key1': 'some string', 'key2': 123}, 'table-2': {'key1': 'another string', 'key2': 456}} """
不过注意:我们必须要把 other-table-1 和 other-table-2 定义在上面,如果我们定义在下面看看会有什么后果:
import toml config = """ [table-1] key1 = "some string" key2 = 123 [table-2] key1 = "another string" key2 = 456 other-table-1.key1 = "some string" other-table-1.key2 = 123 other-table-2.key1 = "another string" other-table-2.key2 = 456 """ data = toml.loads(config) print(data) """ { 'table-1': {'key1': 'some string', 'key2': 123}, 'table-2': {'key1': 'another string', 'key2': 456, 'other-table-1': {'key1': 'some string', 'key2': 123}, 'other-table-2': {'key1': 'another string', 'key2': 456}} } """
你可能已经猜到了,它们被视为了“table-2”对应的字典键。此外我们还可以将上面两种方式结合起来:
import toml config = """ # [] 里面的不再是一个普通的键,而是点分隔键 # 另外键名周围的空格会被忽略,但是最好不要有 [dog . "tater.man"] type.name = "哈巴狗" """ data = toml.loads(config) print(data) """ { 'dog': {'tater.man': {'type': {'name': '哈巴狗'}}} } """
表的里面也是可以没有键值对的:
import toml config = """ [x.y.z.w.a.n] [x.m] [x.n] [x] a.b.c = "xxx" """ data = toml.loads(config) print(data) """ {'x': { 'a': {'b': {'c': 'xxx'}}, 'm': {}, 'n': {}, 'y': {'z': {'w': {'a': {'n': {}}}}} } } """
总的来说还是蛮强大的,但是要注意:不能重复定义。
行内表
行内表提供了一种更为紧凑的语法来表示表,因为上面每一个键值对都需要单独写一行,比如:
[table1] a = 1 b = 2 c = 3 # 最终可以得到 # {'table1': {'a': 1, 'b': 2, 'c': 3}}
但是除了上面的表达方式之外,我们还可以采用行内表:
import toml config = """ # 和 Python 字典的表示方式略有不同 # 并且也支持多种 key table1 = {a = 1, b = "二", c.a = "3"} table2 = {c."b c".d = "4"} """ data = toml.loads(config) print(data) """ { 'table1': {'a': 1, 'b': '二', 'c': {'a': '3'}}, 'table2': {'c': {'b c': {'d': '4'}}} } """
表数组
然后来看看数组和表的结合:
import toml config = """ [name1] girl = "古明地觉" [[name2]] girl = "古明地恋" [name3] [[name4]] """ data = toml.loads(config) print(data) """ {'name1': {'girl': '古明地觉'}, 'name2': [{'girl': '古明地恋'}], 'name3': {}, 'name4': [{}]} """
当使用 [[]] 的时候,相当于在 [] 的基础上套上一层列表。任何对表数组的引用都会指向该数组中最近定义的表元素,这使得我们能够在最近的表内定义子表甚至子表数组。
我们再举个更复杂的例子:
import toml config = """ [[fruits]] name = "苹果" # 会操作 [] 里面最近定义的 {} [fruits.physical] color = "红色" shape = "圆形" [[fruits.varieties]] # 嵌套表数组 name = "蛇果" [[fruits.varieties]] name = "澳洲青苹" [[fruits]] name = "香蕉" [[fruits.varieties]] name = "车前草" """ data = toml.loads(config) print(data) """ { 'fruits': [ { 'name': '苹果', 'physical': {'color': '红色', 'shape': '圆形'}, 'varieties': [{'name': '蛇果'}, {'name': '澳洲青苹'}] }, { 'name': '香蕉', 'varieties': [{'name': '车前草'}] } ] } """
很明显这种定义不是很常用,配置文件应该要非常直观才对,但这已经不是很好理解了。
以上是怎麼用Python解析toml設定檔的詳細內容。更多資訊請關注PHP中文網其他相關文章!
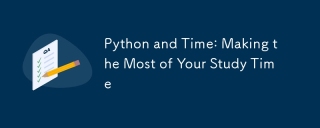
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
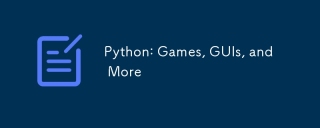
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
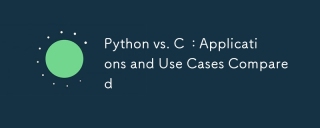
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
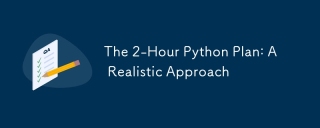
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
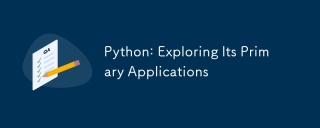
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
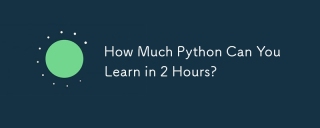
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
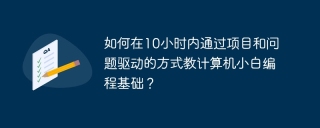
如何在10小時內教計算機小白編程基礎?如果你只有10個小時來教計算機小白一些編程知識,你會選擇教些什麼�...
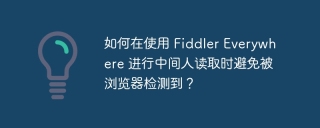
使用FiddlerEverywhere進行中間人讀取時如何避免被檢測到當你使用FiddlerEverywhere...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SublimeText3 Linux新版
SublimeText3 Linux最新版

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

SublimeText3漢化版
中文版,非常好用
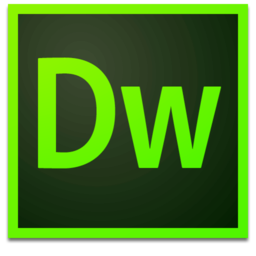
Dreamweaver Mac版
視覺化網頁開發工具

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中