Hello, 大家好,我是菜鳥哥。
是不是常常遇到這種窘境?當親友來家作客,問WiFi密碼,然後翻箱倒櫃、問了一圈也找不到。
今天,要跟大家介紹Python一些鮮為人知的操作。
這些操作,並非是炫技,而是真的實用!
1. 顯示WiFi密碼
我們常常忘記wifi的密碼,可是每當家裡來了親戚朋友問起WiFi密碼,卻又無從下手。
這裡有一個技巧,我們可以列出所有的裝置和它們的密碼。
import subprocess #import required library data = subprocess.check_output(['netsh', 'wlan', 'show', 'profiles']).decode('utf-8').split('n') #store profiles data in "data" variable profiles = [i.split(":")[1][1:-1] for i in data if"All User Profile"in i] #store the profile by converting them to list for i in profiles: # running the command to check passwords results = subprocess.check_output(['netsh', 'wlan', 'show', 'profile', i, 'key=clear']).decode('utf-8').split('n') # storing passwords after converting them to list results = [b.split(":")[1][1:-1] for b in results if"Key Content"in b] try: print ("{:<30}|{:<}".format(i, results[0])) except IndexError: print ("{:<30}|{:<}".format(i, ""))
2. 影片轉GIF
近年來,GIF出現了熱潮。大多數流行的社群媒體平台,都為用戶提供了各種GIF,以更有意義和更容易理解的方式表達他們的想法。
很多同學為了將影片轉成GIF可謂是煞費苦心,而且在這個過程中踩了不少坑。
而使用Python,簡短的幾行程式碼即可解決!
安裝
pip install moviepy
程式碼
from moviepy.editor import VideoFileClip clip = VideoFileClip("video_file.mp4") # Enter your video's path clip.write_gif("gif_file.gif", fps = 10)
3. 桌面提醒
當我們在做專案或其他事情的時候,我們可能會忘記某些重要的事情,我們可以透過在系統上看到一個簡單的通知來記住這些。
在python的幫助下,我們可以創建個人化的通知,並可以將其安排在特定的時間。
安裝
pip install win10toast, schedule
程式碼
import win10toast toaster = win10toast.ToastNotifier() import schedule import time def job(): toaster.show_toast('提醒', "到吃饭时间了!", duration = 15) schedule.every().hour.do(job)#scheduling for every hour; you can even change the scheduled time with schedule library whileTrue: schedule.run_pending() time.sleep(1)
4. 自訂快速鍵
有時,我們在工作中需要頻繁地輸入一些單字。如果我們能使我們的鍵盤自動化,只用縮寫就能寫出這些經常使用的單詞,這不是很有趣嗎?
沒錯,我們可以用Python使其成為可能。
安裝
pip install keyboard
程式碼
import keyboard #press sb and space immediately(otherwise the trick wont work) keyboard.add_abbreviation('ex', '我是一条测试数据!') #provide abbreviation and the original word here # Block forever, like `while True`. keyboard.wait()
然後,在任何位置輸入ex加空格就可以快速補全對應的語句!
5. 文字轉PDF
我們都知道,部分筆記和線上可用的書籍都是以pdf的形式存在。
這是因為pdf可以以相同的方式儲存內容,而不用考慮平台或裝置。
因此,如果我們有文字文件,我們可以在python庫fpdf的幫助下將它們轉換成PDF文件。
安裝
pip install fpdf
程式碼
from fpdf import FPDF pdf = FPDF() pdf.add_page()# Add a page pdf.set_font("Arial", size = 15) # set style and size of font f = open("game_notes.txt", "r")# open the text file in read mode # insert the texts in pdf for x in f: pdf.cell(50,5, txt = x, ln = 1, align = 'C') #pdf.output("path where you want to store pdf file\file_name.pdf") pdf.output("game_notes.pdf")
6. 產生二維碼
我們在日常生活中經常看到二維碼,QR碼節省了很多用戶的時間。
我們也可以用python庫qrcode為網站或個人資料建立獨特的QR碼。
安裝
pip install qrcode
程式碼
#import the library import qrcode #link to the website input_data = "https://car-price-prediction-project.herokuapp.com/" #Creating object #version: defines size of image from integer(1 to 40), box_size = size of each box in pixels, border = thickness of the border. qr = qrcode.QRCode(version=1,box_size=10,border=5) #add_date :pass the input text qr.add_data(input_data) #converting into image qr.make(fit=True) #specify the foreground and background color for the img img = qr.make_image(fill='black', back_color='white') #store the image img.save('qrcode_img.png')
7. 翻譯
我們生活在一個多語言的世界。
因此,為了理解不同的語言,我們需要一個語言翻譯器。
我們可以在python函式庫Translator的幫助下創建我們自己的語言翻譯器。
安裝
pip install translate
程式碼
#import the library from translate import Translator #specifying the language translator = Translator(to_lang="Hindi") #typing the message translation = translator.translate('Hello!!! Welcome to my class') #print the translated message print(translation)
8. Google搜尋
有時候程式設計太忙碌,以至於我們覺得懶得打開瀏覽器來搜尋我們想要的答案。
但有了google這個神奇的python函式庫,我們只需要寫3行程式碼就可以搜尋我們的查詢,而不需要手動開啟瀏覽器並在上面搜尋我們的查詢。
安裝
pip install google
代碼
#import library from googlesearch import search #write your query query = "best course for python" # displaying 10 results from the search for i in search(query, tld="co.in", num=10, stop=10, pause=2): print(i) #you will notice the 10 search results(website links) in the output.
9. 提取音頻
在某些情況下,我們有mp4文件,但我們只需要其中的音頻,例如用另一個視訊的音訊製作一個影片。
我們為獲得相同的音訊檔案做了足夠的努力,但我們失敗了。
這個問題用python庫moviepy可以輕易的解決。
安裝
pip install moviepy
程式碼
#import library import moviepy.editor as mp #specify the mp4 file here(mention the file path if it is in different directory) clip = mp.VideoFileClip('video.mp4') #specify the name for mp3 extracted clip.audio.write_audiofile('Audio.mp3') #you will notice mp3 file will be created at the specified location.
10. 產生短連結
經常和各種各樣的連結打交道,過長的URL讓思緒混亂不堪!
於是,就有了各式各樣的短連結產生工具。
不過,大多數使用都比較麻煩。
我們可以在python函式庫pyshorteners的幫助下創建我們自己的短連結產生器。
安裝
pip install pyshorteners
程式碼
#import library import pyshorteners #creating object s=pyshorteners.Shortener() #type the url url = "type the youtube link here" #print the shortend url print(s.tinyurl.short(url))
讀到這裡,會發現,Python除了完成工作中涉及到的機器學習、資料分析等專案開發,還可以完成很多非常有趣,且能大幅提高工作效率的操作。
本文就是拋磚引玉一下,希望大家能夠尋找到更多有趣的Python玩法!
以上是Python這些操作,逆天且實用!的詳細內容。更多資訊請關注PHP中文網其他相關文章!
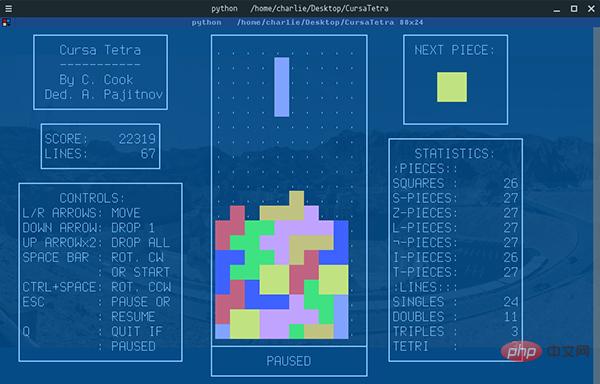
Curses首先出场的是 Curses[1]。CurseCurses 是一个能提供基于文本终端窗口功能的动态库,它可以: 使用整个屏幕 创建和管理一个窗口 使用 8 种不同的彩色 为程序提供鼠标支持 使用键盘上的功能键Curses 可以在任何遵循 ANSI/POSIX 标准的 Unix/Linux 系统上运行。Windows 上也可以运行,不过需要额外安装 windows-curses 库:pip install windows-curses 上面图片,就是一哥们用 Curses 写的 俄罗斯
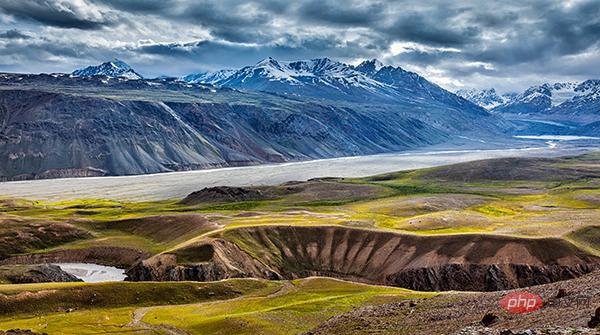
相比大家都听过自动化生产线、自动化办公等词汇,在没有人工干预的情况下,机器可以自己完成各项任务,这大大提升了工作效率。编程世界里有各种各样的自动化脚本,来完成不同的任务。尤其Python非常适合编写自动化脚本,因为它语法简洁易懂,而且有丰富的第三方工具库。这次我们使用Python来实现几个自动化场景,或许可以用到你的工作中。1、自动化阅读网页新闻这个脚本能够实现从网页中抓取文本,然后自动化语音朗读,当你想听新闻的时候,这是个不错的选择。代码分为两大部分,第一通过爬虫抓取网页文本呢,第二通过阅读工
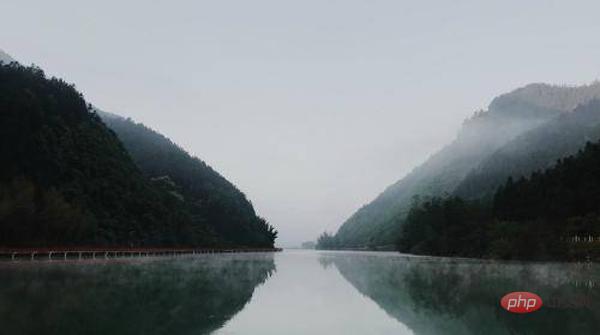
糟透了我承认我不是一个爱整理桌面的人,因为我觉得乱糟糟的桌面,反而容易找到文件。哈哈,可是最近桌面实在是太乱了,自己都看不下去了,几乎占满了整个屏幕。虽然一键整理桌面的软件很多,但是对于其他路径下的文件,我同样需要整理,于是我想到使用Python,完成这个需求。效果展示我一共为将文件分为9个大类,分别是图片、视频、音频、文档、压缩文件、常用格式、程序脚本、可执行程序和字体文件。# 不同文件组成的嵌套字典 file_dict = { '图片': ['jpg','png','gif','webp
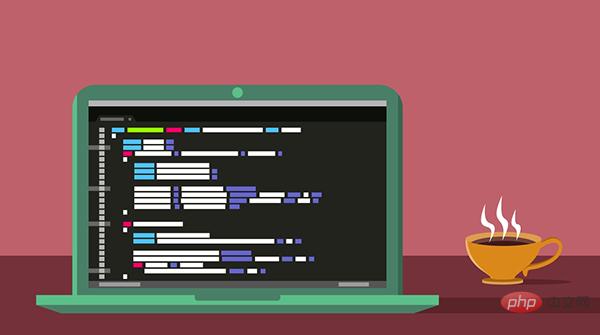
长期以来,Python 社区一直在讨论如何使 Python 成为网页浏览器中流行的编程语言。然而网络浏览器实际上只支持一种编程语言:JavaScript。随着网络技术的发展,我们已经把越来越多的程序应用在网络上,如游戏、数据科学可视化以及音频和视频编辑软件。这意味着我们已经把繁重的计算带到了网络上——这并不是JavaScript的设计初衷。所有这些挑战提出了对新编程语言的需求,这种语言可以提供快速、可移植、紧凑和安全的代码执行。因此,主要的浏览器供应商致力于实现这个想法,并在2017年向世界推出
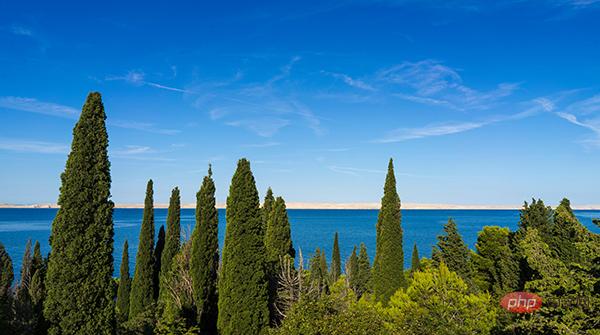
首先要说,聚类属于机器学习的无监督学习,而且也分很多种方法,比如大家熟知的有K-means。层次聚类也是聚类中的一种,也很常用。下面我先简单回顾一下K-means的基本原理,然后慢慢引出层次聚类的定义和分层步骤,这样更有助于大家理解。层次聚类和K-means有什么不同?K-means 工作原理可以简要概述为: 决定簇数(k) 从数据中随机选取 k 个点作为质心 将所有点分配到最近的聚类质心 计算新形成的簇的质心 重复步骤 3 和 4这是一个迭代过程,直到新形成的簇的质心不变,或者达到最大迭代次数
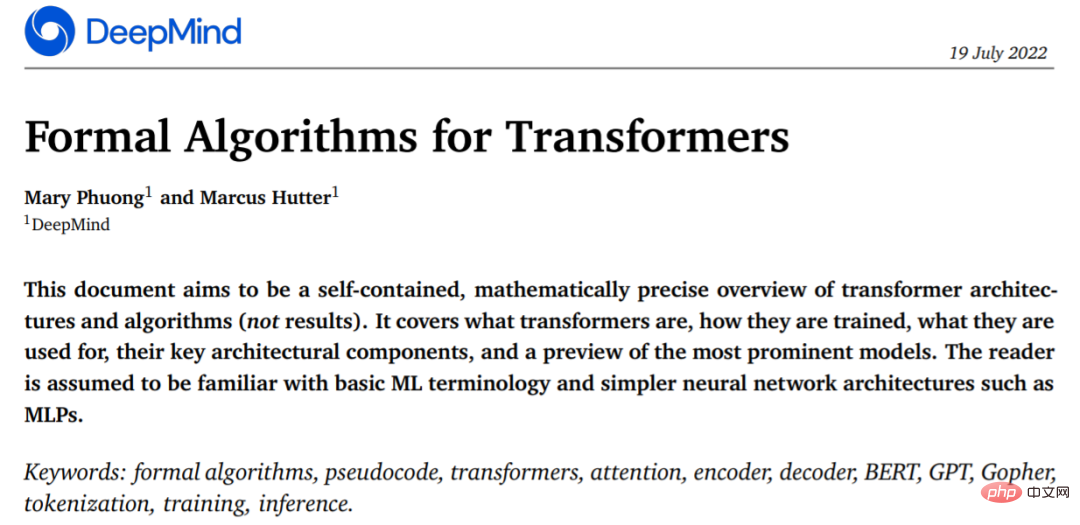
2017 年 Transformer 横空出世,由谷歌在论文《Attention is all you need》中引入。这篇论文抛弃了以往深度学习任务里面使用到的 CNN 和 RNN。这一开创性的研究颠覆了以往序列建模和 RNN 划等号的思路,如今被广泛用于 NLP。大热的 GPT、BERT 等都是基于 Transformer 构建的。Transformer 自推出以来,研究者已经提出了许多变体。但大家对 Transformer 的描述似乎都是以口头形式、图形解释等方式介绍该架构。关于 Tra
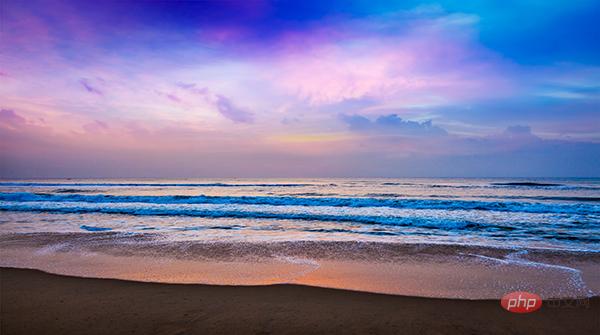
大家好,我是J哥。这个没有点数学基础是很难算出来的。但是我们有了计算机就不一样了,依靠计算机极快速的运算速度,我们利用微分的思想,加上一点简单的三角学知识,就可以实现它。好,话不多说,我们来看看它的算法原理,看图:由于待会要用pygame演示,它的坐标系是y轴向下,所以这里我们也用y向下的坐标系。算法总的思想就是根据上图,把时间t分割成足够小的片段(比如1/1000,这个时间片越小越精确),每一个片段分别构造如上三角形,计算出导弹下一个时间片走的方向(即∠a)和走的路程(即vt=|AC|),这时

集成GPT-4的Github Copilot X还在小范围内测中,而集成GPT-4的Cursor已公开发行。Cursor是一个集成GPT-4的IDE,可以用自然语言编写代码,让编写代码和聊天一样简单。 GPT-4和GPT-3.5在处理和编写代码的能力上差别还是很大的。官网的一份测试报告。前两个是GPT-4,一个采用文本输入,一个采用图像输入;第三个是GPT3.5,可以看出GPT-4的代码能力相较于GPT-3.5有较大能力的提升。集成GPT-4的Github Copilot X还在小范围内测中,而


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。
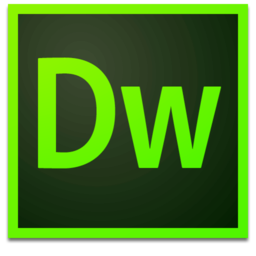
Dreamweaver Mac版
視覺化網頁開發工具