Golang是一種高效能、快速且可靠的程式語言,經常被用於高效能的應用程式的開發。同時,Golang也內建了對IPC(Inter-process communication,進程間通訊)的支持,可以用於進程間通訊。在本文中,我們將會介紹Golang如何設定IPC的基本知識,並透過一些範例來幫助讀者更好地理解IPC。
IPC是什麼?
IPC是兩個或多個進程之間的通訊方法。 IPC是一種不同於在單一進程內運行的執行緒或進程之間的通訊方式。 IPC可以用於在本地或遠端,同步或非同步地共享資料。在作業系統中,IPC通常涉及共享記憶體、訊息傳遞、管道、訊號等。
Golang支援哪些IPC?
Golang提供了幾種IPC方法,包括記憶體共享(shared memory)、基於通道(channel-based)和進程間訊號(process signal)通訊。這些方法都有其自身的優缺點和適用範圍。
如何使用Golang設定IPC?
在Golang中,我們可以使用系統呼叫(syscall)來設定IPC。下面是一段範例程式碼,它使用syscall.Stat()函數來檢查一個檔案是否存在:
package main import ( "fmt" "syscall" ) func main() { var s syscall.Stat_t if err := syscall.Stat("/path/to/file", &s); err != nil { if err == syscall.ENOENT { fmt.Printf("File does not exist: %s\n", err) } else { fmt.Printf("Error: %s\n", err) } return } fmt.Printf("File information: %+v\n", s) }
利用syscall我們可以透過共享記憶體、訊息傳遞等方式在不同進程間進行資料傳輸。
共享記憶體
共享記憶體是IPC的一種形式,它允許多個進程共享相同的記憶體區域。如果在一個進程中更改了共享內存,更改將在所有使用共享內存的進程中生效。共享記憶體可用於高速資料傳輸、資料快取和共享資料結構。
Golang提供了一個sys/mman包,其提供了一個mmap()函數可以用於在多個進程間共享資料。下面是一個範例程式:
package main import ( "fmt" "os" "strconv" "syscall" ) func main() { //创建一个匿名内存映射 fd, _ := syscall.MemfdCreate("shared_mem_file", syscall.MFD_CLOEXEC) defer syscall.Close(fd) //分配共享内存 err := syscall.Ftruncate(fd, 1024*1024) // 1 MB if err != nil { fmt.Printf("Error: %s\n", err) return } // 使用mmap映射内存,通过sllice类型访问共享内存 mmap, err := syscall.Mmap(fd, 0, 1024*1024, syscall.PROT_READ|syscall.PROT_WRITE, syscall.MAP_SHARED) if err != nil { fmt.Printf("Error: %s\n", err) return } defer syscall.Munmap(mmap) pid := os.Getpid() strconv.Itoa(pid) // 在共享内存中写入当前进程号 copy(mmap, []byte("Process ID: "+strconv.Itoa(pid))) fmt.Printf("Data written to shared memory: %+v\n", mmap[:16]) // 等待共享内存被读取 fmt.Printf("Press enter to continue!\n") fmt.Scanln() }
訊息傳遞
訊息傳遞是IPC的另一種形式,它允許進程透過使用佇列或管道等通道來傳輸訊息。在Unix-like系統中,Golang可以使用sys/unix套件中的socketpair函數來建立一個雙向通訊管道,這樣每個進程都可以透過這個通道發送和接收訊息。
下面是一個使用管道通訊的範例程式:
package main import ( "fmt" "syscall" "unsafe" ) func main() { // 创建管道 var fds [2]int if err := syscall.Pipe(fds[:]); err != nil { fmt.Printf("Error creating pipe: %s\n", err) return } defer syscall.Close(fds[0]) defer syscall.Close(fds[1]) // 重定向stdin dupSTDIN, _ := syscall.Dup(0) defer syscall.Close(dupSTDIN) syscall.Dup2(fds[0], 0) // 写入到管道 fmt.Printf("Writing to pipe...\n") fmt.Printf("Data written to pipe: %s\n", "Hello, pipe!") // 关闭写管道,避免阻塞 syscall.Close(fds[1]) syscall.Dup2(dupSTDIN, 0) // 从管道中读取数据 data := make([]byte, 1000) bytesRead, _ := syscall.Read(fds[0], data) fmt.Printf("Data read from pipe: %s\n", string(data[:bytesRead])) }
進程間訊號
進程間訊號是一種IPC方法,它允許進程發送訊號給其他進程。在Unix-like系統中,訊號通常用於向進程發送警告或請求其關閉等。
Golang中,我們可以使用syscall套件中的Kill函數來傳送進程間訊號。下面是一個範例程式:
package main import ( "fmt" "os" "syscall" ) func main() { pid := os.Getpid() fmt.Printf("Current process ID: %d\n", pid) // 发送SIGUSR1信号 err := syscall.Kill(pid, syscall.SIGUSR1) if err != nil { fmt.Printf("Error sending signal: %s", err) } }
這裡我們使用了SIGUSR1訊號,並且給目前行程發送了一個SIGUSR1的訊號。
總結
在本文中,我們介紹了Golang的IPC通訊方法,包括共享記憶體、訊息傳遞和進程間訊號。 Golang內建了對IPC的支持,並透過syscall系統呼叫提供了存取底層作業系統IPC功能的介面。我們透過實例程式介紹如何使用這些IPC方法來在進程之間進行通訊。在實際應用中,我們應該根據特定的應用場景來選擇最適合的IPC方法。
以上是golang怎麼設定ipc的詳細內容。更多資訊請關注PHP中文網其他相關文章!
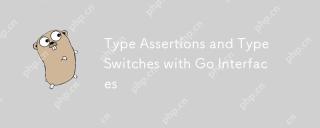
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
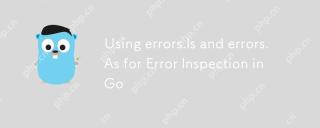
Go語言的錯誤處理通過errors.Is和errors.As函數變得更加靈活和可讀。 1.errors.Is用於檢查錯誤是否與指定錯誤相同,適用於錯誤鏈的處理。 2.errors.As不僅能檢查錯誤類型,還能將錯誤轉換為具體類型,方便提取錯誤信息。使用這些函數可以簡化錯誤處理邏輯,但需注意錯誤鏈的正確傳遞和避免過度依賴以防代碼複雜化。
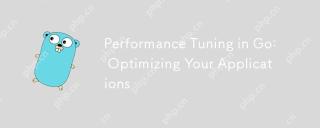
tomakegoapplicationsRunfasterandMorefly,useProflingTools,leverageConCurrency,andManageMoryfectily.1)usepprofforcpuorforcpuandmemoryproflingtoidentifybottlenecks.2)upitizegorizegoroutizegoroutinesandchannelstoparalletaparelalyizetasksandimproverperformance.3)
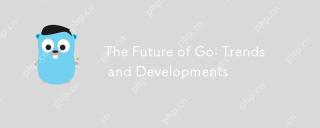
go'sfutureisbrightwithtrendslikeMprikeMprikeTooling,仿製藥,雲 - 納蒂維德象,performanceEnhancements,andwebassemblyIntegration,butchallengeSinclainSinClainSinClainSiNgeNingsImpliCityInsImplicityAndimimprovingingRornhandRornrorlling。
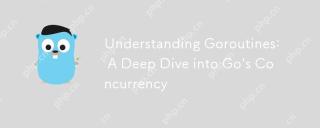
goroutinesarefunctionsormethodsthatruncurranceingo,啟用效率和燈威量。 1)shememanagedbodo'sruntimemultimusingmultiplexing,允許千sstorunonfewerosthreads.2)goroutinessimproverentimensImproutinesImproutinesImproveranceThroutinesImproveranceThrountinesimproveranceThroundinesImproveranceThroughEasySytaskParallowalizationAndeff
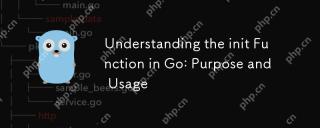
purposeoftheInitfunctionoIsistoInitializeVariables,setUpConfigurations,orperformneccesSetarySetupBeforEtheMainFunctionExeCutes.useInitby.UseInitby:1)placingitinyourcodetorunautoamenationally oneraty oneraty oneraty on inity in ofideShortAndAndAndAndForemain,2)keepitiTshortAntAndFocusedonSimImimpletasks,3)
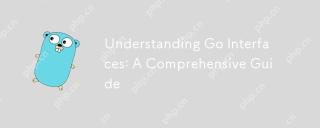
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
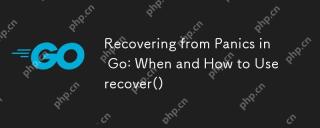
在Go中使用recover()函數可以從panic中恢復。具體方法是:1)在defer函數中使用recover()捕獲panic,避免程序崩潰;2)記錄詳細的錯誤信息以便調試;3)根據具體情況決定是否恢復程序執行;4)謹慎使用,以免影響性能。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3漢化版
中文版,非常好用
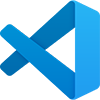
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器
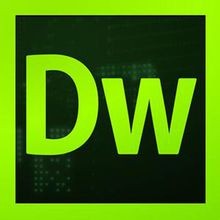
Dreamweaver CS6
視覺化網頁開發工具
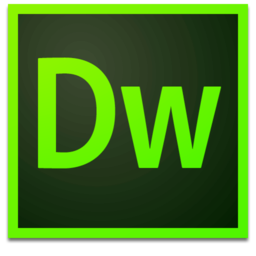
Dreamweaver Mac版
視覺化網頁開發工具

SublimeText3 Linux新版
SublimeText3 Linux最新版