在開發Angular 元件的過程中,我們習慣把元件的樣式寫在對應的css 檔案中,但一直不了解Angular 是怎麼做樣式隔離的,例如在A 元件中寫了h1 { color: red }
,這個樣式只會在A 元件中生效,而不會影響到其他的元件。為了探究原理,就有了這篇文章,以下內容是基於 Angular CLI 10.1.1 版本創建。
元件樣式工作原理
探索
#先用Angular CLI 建立一個新的Angular 項目,刪除 app.component.html
中的所有內容,替換成如下內容:
<h1 id="App-nbsp-Component">App Component</h1> <button class="red-button">Button</button>
在app.component.css
中新增以下內容:
.red-button { color: red; }
運行時有以下html 程式碼:
<app-root _nghost-ydo-c11="" ng-version="10.1.1"> <h2 id="App-nbsp-component">App component</h2> <button _ngcontent-ydo-c11="" class="red-button">Button</button> </app-root>
可以看到在在app-root
元素上有一個名為_nghost-ydo-c11
的屬性(property), app-root
裡面的兩個元素都有一個名為_ngcontent-ydo-c11
的屬性。 【相關教學推薦:《angular教學》】
那麼這些屬性是用來做什麼的呢?
為了更好的理解,我們先建立一個獨立的元件,新檔案blue-button.component.ts
,內容如下:
import { Component } from '@angular/core'; @Component({ selector: 'app-blue-button', template: ` <h2 id="Blue-nbsp-button-nbsp-component">Blue button component</h2> <button class="blue-button">Button</button> `, styles: [` .blue-button { background: blue; } `] }) export class BlueButtonComponent {}
放到app.component.html
中運行後,會看到如下html 程式碼:
#可以看到app-blue-button
中也有一個以_nghost-xxx
開頭的屬性,還有一個和app-root
中其他元素相同的屬性。而在元件裡面的兩個元素都有一個名為 _ngcontent-yke-c11
的屬性。
由於每次運行,Angular 產生的屬性字串都是隨機的,所以後面的程式碼如果出現了類似的屬性都是按照這個截圖對應的。
總結
透過觀察我們可以總結:
- 每個元件的宿主元素都會被指派一個唯一的屬性,取決於元件的處理順序,在例子中就是
_nghost_xxx
- 每個元件模板中的每個元素也會被指派一個該元件特有的屬性,在範例中就是
_ngcontent_xxx
那麼這些屬性是怎麼用來樣式隔離的呢?
這些屬性可以和CSS 結合起來,例如當我們查看範例中藍色按鈕的樣式時,會看到這樣的css:
.blue-button[_ngcontent-yke-c11] { background: blue; }
可以看出,Angular 透過這種方式使blue-button
類別只能套用在有這個屬性的元素上,而不會影響到其他元件中的元素。
知道了 Angular 對樣式隔離的行為,那麼 Angular 又是如何做到這些的呢?
在套用啟動時,Angular 將透過 styles 或 styleUrls 元件屬性來查看哪些樣式與哪些元件相關聯。之後Angular 會將這些樣式和該元件中元素特有的屬性套用在一起,將產生的 css 程式碼包裹在一個 style 標籤中並放到 header 裡。
以上就是 Angular 樣式封裝的原理。
:host, :host-context, ::ng-deep
在實際開發中,這種機制有時候並不能完全匹配我們的需求,針對這種情況, Angular 引入了幾種特殊的選擇器。
:host
使用 :host 偽類別選擇器,用來作用於元件(宿主元素)本身。
例如,當我們想要為app-blue-button
加個邊框時,可以在這個元件的樣式中加入如下程式碼:
:host { display: block; border: 1px solid red; }
透過檢視執行時的程式碼,可以看到如下程式碼區塊:
<style> [_nghost-yke-c11] { display: block; border: 1px solid red; } </style>
:host-context
有時候,基於某些來自元件檢視外部的條件應用樣式是很有用的。例如,在文件的 元素上可能有一個用於表示樣式主題 (theme) 的 CSS 類,你應該基於它來決定元件的樣式。
這時可以使用 :host-context()
偽類選擇器。它也以類似 :host()
形式使用。它在目前元件宿主元素的祖先節點中尋找 CSS 類, 直到文件的根節點為止。在與其它選擇器組合使用時,它非常有用。
在下面的範例中,會根據祖先元素的 CSS 類別是 blue-theme
還是 red-theme
來決定哪個 CSS 會生效。
import { Component } from '@angular/core'; @Component({ selector: 'app-btn-theme', template: ` <button class="btn-theme">Button</button> `, styles: [` :host-context(.blue-theme) .btn-theme { background: blue; } :host-context(.red-theme) .btn-theme { background: red; } `] }) export class BtnThemeComponent { }
然後在使用的地方:
<div class="blue-theme"> <app-btn-theme></app-btn-theme> </div>
在運行的時候按鈕背景是藍色的。
::ng-deep
我们经常会使用一些第三方 UI 库,有时候我们想改变第三方组件的一些样式,这时候可以使用 ::ng-deep,但是要注意,Angular 已经把这个特性标记为已废弃了,可能在未来的版本就被完全移除掉。
:host ::ng-deep h2 { color: yellow; }
通过查看运行时的代码:
[_nghost-yke-c12] h2 { color: yellow; }
可以看到,样式会作用在 app-root
里的所有元素上,包括 app-root
中使用的其他组件里的元素。
总结
在实际开发中,灵活使用以上几种方式,基本上可以满足大部分场景。
更多编程相关知识,请访问:编程教学!!
以上是淺談Angular中元件樣式的工作原理的詳細內容。更多資訊請關注PHP中文網其他相關文章!
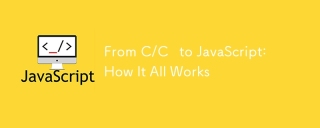
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
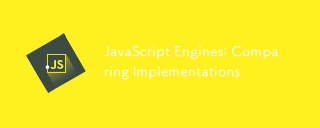
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
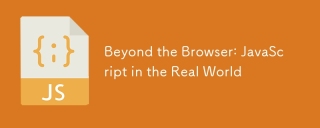
JavaScript在現實世界中的應用包括服務器端編程、移動應用開發和物聯網控制:1.通過Node.js實現服務器端編程,適用於高並發請求處理。 2.通過ReactNative進行移動應用開發,支持跨平台部署。 3.通過Johnny-Five庫用於物聯網設備控制,適用於硬件交互。
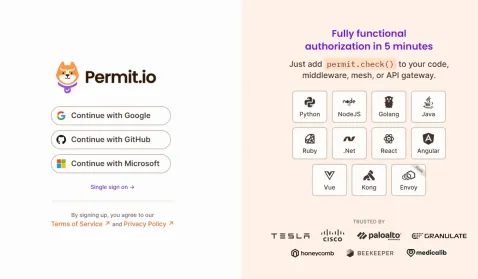
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
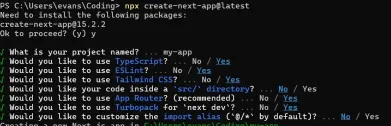
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
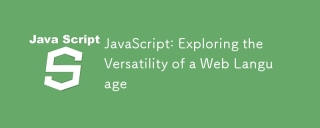
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
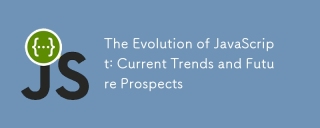
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
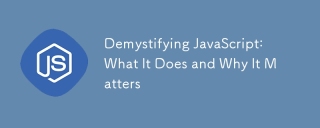
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SublimeText3 Linux新版
SublimeText3 Linux最新版

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

SublimeText3漢化版
中文版,非常好用
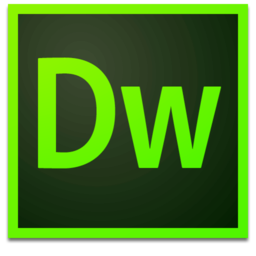
Dreamweaver Mac版
視覺化網頁開發工具

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中