首先看下我們的目標:向mysql資料庫中批次插入10000條資料
操作環境:Mysql和Java程式碼都運行在我本機Windows電腦(i7處理器,4核,16G運行內存,64位元作業系統
1、JPA單執行緒執行
程式碼省略,大概需要39S左右
2、JPA多執行緒執行
大概要37S左右,並沒有想像中的快很多
(免費學習影片分享:java影片教學)
原因: 多執行緒只是大幅提高了程式處理資料的時間,並不會提高插入資料庫的時間,相反地在我這邊JPA的框架下,多執行緒也意味著多連接,反而更消耗資料庫效能
package com.example.demo.controller; import com.example.demo.entity.Student; import com.example.demo.service.StudentServiceInterface; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import javax.xml.bind.ValidationException; import java.util.ArrayList; import java.util.Date; import java.util.List; import java.util.concurrent.CountDownLatch; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; @RestController @RequestMapping("/student") public class StudentController { @Autowired private StudentServiceInterface studentServiceInterface; // 来使主线程等待线程池中的线程执行完毕 private CountDownLatch threadsSignal; // 每个线程处理的数据量 private static final int count = 1000; // 我的电脑为4核 线程池大小设置为2N+1 private static ExecutorService execPool = Executors.newFixedThreadPool(9); /** * 多线程保存 * * @return * @throws ValidationException */ @GetMapping() public String saveStudentEnableThread() throws ValidationException { Long begin = new Date().getTime(); // 需要插入数据库的数据 List<Student> list = new ArrayList<>(); for (int i = 0; i < 10000; i++) { Student student = new Student(); student.setName("张三"); student.setAge(10); list.add(student); } try { if (list.size() <= count) { threadsSignal = new CountDownLatch(1); execPool.submit(new InsertDate(list)); } else { List<List<Student>> lists = dealData(list, count); threadsSignal = new CountDownLatch(lists.size()); for (List<Student> students : lists) { execPool.submit(new InsertDate(students)); } } threadsSignal.await(); } catch (Exception e) { System.out.println(e.toString() + " 错误所在行数:" + e.getStackTrace()[0].getLineNumber()); } // 结束时间 Long end = new Date().getTime(); return "10000条数据插入花费时间 : " + (end - begin) / 1000 + " s"; } /** * 数据组装 * 把每个线程要处理的数据 再组成一个List * 我这边就是把10000条数据 组成 10个1000条的集合 * * @param target 数据源 * @param size 每个线程处理的数量 * @return */ public static List<List<Student>> dealData(List<Student> target, int size) { List<List<Student>> threadList = new ArrayList<List<Student>>(); // 获取被拆分的数组个数 int arrSize = target.size() % size == 0 ? target.size() / size : target.size() / size + 1; for (int i = 0; i < arrSize; i++) { List<Student> students = new ArrayList<Student>(); //把指定索引数据放入到list中 for (int j = i * size; j <= size * (i + 1) - 1; j++) { if (j <= target.size() - 1) { students.add(target.get(j)); } } threadList.add(students); } return threadList; } /** * 内部类,开启线程批量保存数据 */ class InsertDate extends Thread { List<Student> list = new ArrayList<Student>(); public InsertDate(List<Student> students) { list = students; } public void run() { try { // 与数据库交互 studentServiceInterface.save(list); threadsSignal.countDown(); } catch (ValidationException e) { e.printStackTrace(); } } } }
3、傳統JDBC插入
大概要8S左右,相較於前兩種方式已經快很多了,程式碼如下:
package com.example.demo.controller; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import javax.xml.bind.ValidationException; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.util.Date; @RestController @RequestMapping("/student1") public class StudentController1 { @GetMapping() public String saveStudentEnableThread() throws ValidationException { // 开始时间 Long begin = new Date().getTime(); Connection connection = null; try { connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/db01?characterEncoding=utf8&useUnicode=true&useSSL=false&serverTimezone=UTC&allowPublicKeyRetrieval=true", "admin", "123456");//获取连接 if (connection != null) { System.out.println("获取连接成功"); } else { System.out.println("获取连接失败"); } //这里必须设置为false,我们手动批量提交 connection.setAutoCommit(false); //这里需要注意,SQL语句的格式必须是预处理的这种,就是values(?,?,...,?),否则批处理不起作用 PreparedStatement statement = connection.prepareStatement("insert into student(id,`name`,age) values(?,?,?)"); // 塞数据 for (int i = 0; i < 10000; i++) { statement.setInt(1, i+1); statement.setString(2, "张三"); statement.setInt(3, 10); //将要执行的SQL语句先添加进去,不执行 statement.addBatch(); } // 提交要执行的批处理,防止 JDBC 执行事务处理 statement.executeBatch(); connection.commit(); // 关闭相关连接 statement.close(); connection.close(); } catch (Exception e) { e.printStackTrace(); } // 结束时间 Long end = new Date().getTime(); // 耗时 System.out.println("10000条数据插入花费时间 : " + (end - begin) / 1000 + " s"); return "10000条数据插入花费时间 : " + (end - begin) / 1000 + " s"; } }
4、最後檢查一下資料是否成功儲存庫,一共30000條,沒有丟資料
完成!
相關推薦:java入門教學
#以上是java向mysql資料庫批量插入大量數據的詳細內容。更多資訊請關注PHP中文網其他相關文章!
陳述
本文轉載於:csdn。如有侵權,請聯絡admin@php.cn刪除

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章
R.E.P.O.能量晶體解釋及其做什麼(黃色晶體)
4 週前By尊渡假赌尊渡假赌尊渡假赌
R.E.P.O.最佳圖形設置
3 週前By尊渡假赌尊渡假赌尊渡假赌
刺客信條陰影:貝殼謎語解決方案
2 週前ByDDD
R.E.P.O.如果您聽不到任何人,如何修復音頻
4 週前By尊渡假赌尊渡假赌尊渡假赌
WWE 2K25:如何解鎖Myrise中的所有內容
1 個月前By尊渡假赌尊渡假赌尊渡假赌

熱工具

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。
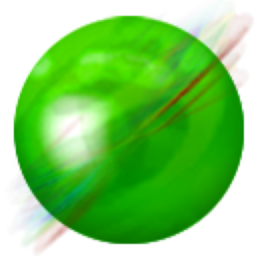
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

Atom編輯器mac版下載
最受歡迎的的開源編輯器

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具
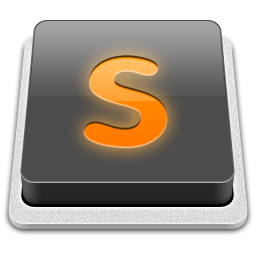
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)