官方文件:http://vuejs.github.io/vue-validator/zh-cn/index.html
github專案網址:https:// github.com/vuejs/vue-validator
單獨使用vue-validator的方法見官方文檔,本文結合vue-router使用。
安裝驗證器
不加入自訂驗證器或無需全域使用的公用驗證器,在main.js中安裝驗證器,使用CommonJS 模組規格, 需要明確的使用 Vue.use() 安裝驗證器元件。
import Validator from 'vue-validator' Vue.use(Validator)
與 vue-router 同時使用,必須在呼叫 router#map, router#start 等實例方法前安裝驗證。
若要自訂驗證器,請建立一個js文件,在該文件中安裝驗證器元件。例如:validation.js
import Vue from 'vue' import Validator from 'vue-validator' Vue.use(Validator) //自定义验证器
自訂驗證器
官方提供的api如下
input[type="text"] input[type="radio"] input[type="checkbox"] input[type="number"] input[type="password"] input[type="email"] input[type="tel"] input[type="url"] select textarea
但是以上的不一定要滿足我們的需求,這時就需要用到另一個全域api,用來註冊和取得全域驗證器。
Vue.validator( id, [definition] )
範例 定義validation.js 內容如下
import Vue from 'vue' import Validator from 'vue-validator' Vue.use(Validator) //自定义验证器 //添加一个简单的手机号验证 //匹配0-9之间的数字,并且长度是11位 Vue.validator('tel', function (val) { return /^[0-9]{11}$/.test(val) }); //添加一个密码验证 //匹配6-20位的任何字类字符,包括下划线。与“[A-Za-z0-9_]”等效。 Vue.validator('passw', function (val) { return /^(\w){6,20}$/.test(val) });
使用驗證器
驗證器語法
<validator name="validation"> <input type="text" v-model='comment' id='comment' v-validate:comment="{ minlength: 3, maxlength: 15 }"> <div> <span v-show="$validation.comment.minlength">不得少于3个字符</span> <span v-show="$validation.comment.maxlength">不得大于15个字符</span> </div> </validator>
預設情況下, vue-validator 會依照 validator 和 v-validate 指令自動進行驗證。然而有時我們需要關閉自動驗證,在有需要時手動觸發驗證。如果你不需要自動驗證,可以透過 initial 屬性或 v-validate 驗證規則來關閉自動驗證。
如下:
<validator name="validation"> <input type="text" v-model='comment' id='comment' v-validate:comment="{ minlength: 3, maxlength: 15 }" detect-change="off" initial='off'> <div> <span v-show="$validation.comment.minlength">不得少于3个字符</span> <span v-show="$validation.comment.maxlength">不得大于15个字符</span> </div> </validator>
Terminal 指令問題
<validator name="test_validator"> <!-- @invalid:valid的逆 ,表示验证不通过 --> <input @invalid="passwInvalid" @valid="passwok" type="password" v-model='passw' id='passw' v-validate:passw="['passw']" detect-change="off" initial='off' placeholder='请输入密码'> <input @invalid="passwInvalid" @valid="passwok" type="password" v-model='passw2' id='passw2' v-validate:passw2="['passw']" detect-change="off" initial='off' placeholder='请输入密码'> </validator> <script> //若是在main.js中导入 无需再次导入 //此处导入的是上面代码的validation.js import validator from '../validator/validation' export default{ data(){ return{ comment:'', passw:'', passw2:'' } }, methods:{ passwInvalid(){ alert('只能输入6-20个字母、数字、下划线'); }, passwok(){ //alert('验证码符合规范') } } } </script>
範例:使用者註冊驗證
用了一個元件來顯示提示訊息
toast.vue
<template> <div v-show="toastshow" transition="toast" class="toast font-normal"> {{toasttext}} </div> </template> <script> export default{ props:{ //是否显示提示 toastshow:{ type:Boolean, required: false, default:function(){ return false; } }, //提示的内容 toasttext:{ type:String, required: false, default:function(){ return 'no message'; } }, //显示的时间 duration: { type: Number, default:3000,//默认3秒 required:false } }, ready() { }, watch:{ toastshow(val){ if (this._timeout) clearTimeout(this._timeout) if (val && !!this.duration) { this._timeout = setTimeout(()=> this.toastshow = false, this.duration) } } } } </script> <style> .toast{ position:absolute; left:50%; margin-left:-25%; bottom:30px; display:block; width:200px; height:auto; text-align:center; color:white; background-color:rgba(0,0,0,0.5); border-radius:10px; z-index:10; transform:scale(1); padding:5px; } .toast-transition{ transition: all .3s ease; } .toast-enter{ opacity:0; transform:scale(0.1); } .toast-leave{ opacity:0; transform:scale(0.1); } </style>
註冊用戶:假如我們需要填寫手機號碼和輸入兩次密碼
<template> <div class='register-box'> <!-- 组件:用于显示提示信息 --> <Toast :toastshow.sync="toastshow" :toasttext="toasttext"></Toast> <validator name="validation_register1"> <div class='register1'> <div class='pd05'> <input @invalid="telonInvalid" initial="off" detect-change="off" v-model="telphone" id="telphone" type="tel" class='phone-number' v-validate:telphone="['tel']" placeholder='请输入手机号码'> </div> <div class='pd05'> <input @invalid="passwInvalid" v-model="passw1" initial="off" detect-change="off" id="passw1" type="password" v-validate:passw1="['passw']"class='password-number'placeholder='请输入密码'> </div> <div class='pd05'> <input @invalid="passwInvalid" v-model="passw2" initial="off" detect-change="off" id="passw2" type="password" v-validate:passw2="['passw']" class='password-number' placeholder='请输入密码'> </div> <a class='greenBtn' v-on:click='register_user()'>下一步</a> </div> </validator> </div> </template> <script> //导入validation.js 此处的validation.js就是上文中validation.js的内容 import validator from '../validator/validation'; //导入显示提示信息的组件 import Toast from '../components/toast.vue'; export default{ components: { //注册组件 Toast }, data(){ return{ telphone:'',//电话号码 toastshow:false,//默认不现实提示信息 toasttext:'',//提示信息内容 passw1:'',//首次输入密码 passw2:''//再次输入密码 } }, methods:{ //手机号验证失败时执行的方法 telonInvalid(){ //设置提示信息内容 this.$set('toasttext','手机不正确'); //显示提示信息组件 this.$set('toastshow',true); }, //密码验证失败时执行的方法 passwInvalid(){ this.$set('toasttext','只能输入6-20个字母、数字、下划线'); this.$set('toastshow',true); }, register_user(){ var that = this; var telephones = that.$get('telphone'); var pw1 = that.$get('passw1'); var pw2 = that.$get('passw2') that.$validate(true, function () { if (that.$validation_register1.invalid) { //验证无效 that.$set('toasttext','请完善表单'); that.$set('toastshow',true); }else{ that.$set('toasttext','验证通过'); that.$set('toastshow',true); //验证通过做注册请求 /*that.$http.post('http://192.168.30.235:9999/rest/user/register', 'account':telephones,'pwd':pw1,'pwd2':pw2}).then(function(data){ if(data.data.code == '0'){ that.$set('toasttext','注册成功'); that.$set('toastshow',true); }else{ that.$set('toasttext','注册失败'); that.$set('toastshow',true); } },function(error){ //显示返回的错误信息 that.$set('toasttext',String(error.status)); that.$set('toastshow',true); })*/ } }) } } } </script> <style> .register-box{ padding: 10px; } .pd05{ margin-top: 5px; } .greenBtn{ width: 173px; height: 30px; text-align: center; line-height: 30px; background: red; color: #fff; margin-top: 5px; } </style>
如果點擊下一步,會提示“請完善表單”,因為驗證不通過;若是文字方塊獲得焦點後失去焦點則會提示相應的錯誤訊息;若內容填寫正確,則會提示驗證通過並發送相應的請求。
效果如圖
相關推薦:
更多程式相關知識,請訪問:程式設計入門! !
以上是詳解vue驗證器(vue-validator)的使用的詳細內容。更多資訊請關注PHP中文網其他相關文章!

Vue.js通過多種功能提升用戶體驗:1.響應式系統實現數據即時反饋;2.組件化開發提高代碼復用性;3.VueRouter提供平滑導航;4.動態數據綁定和過渡動畫增強交互效果;5.錯誤處理機制確保用戶反饋;6.性能優化和最佳實踐提升應用性能。

Vue.js在Web開發中的角色是作為一個漸進式JavaScript框架,簡化開發過程並提高效率。 1)它通過響應式數據綁定和組件化開發,使開發者能專注於業務邏輯。 2)Vue.js的工作原理依賴於響應式系統和虛擬DOM,優化性能。 3)實際項目中,使用Vuex管理全局狀態和優化數據響應性是常見實踐。

Vue.js是由尤雨溪在2014年發布的漸進式JavaScript框架,用於構建用戶界面。它的核心優勢包括:1.響應式數據綁定,數據變化自動更新視圖;2.組件化開發,UI可拆分為獨立、可複用的組件。

Netflix使用React作為其前端框架。 1)React的組件化開發模式和強大生態系統是Netflix選擇它的主要原因。 2)通過組件化,Netflix將復雜界面拆分成可管理的小塊,如視頻播放器、推薦列表和用戶評論。 3)React的虛擬DOM和組件生命週期優化了渲染效率和用戶交互管理。

Netflix在前端技術上的選擇主要集中在性能優化、可擴展性和用戶體驗三個方面。 1.性能優化:Netflix選擇React作為主要框架,並開發了SpeedCurve和Boomerang等工具來監控和優化用戶體驗。 2.可擴展性:他們採用微前端架構,將應用拆分為獨立模塊,提高開發效率和系統擴展性。 3.用戶體驗:Netflix使用Material-UI組件庫,通過A/B測試和用戶反饋不斷優化界面,確保一致性和美觀性。

NetflixusesAcustomFrameworkcalled“ Gibbon” BuiltonReact,notReactorVuedIrectly.1)TeamSperience:selectBasedonFamiliarity.2)ProjectComplexity:vueforsimplerprojects:reactforforforproproject,reactforforforcompleplexones.3)cocatizationneedneeds:reactoffipicatizationneedneedneedneedneedneeds:reactoffersizationneedneedneedneedneeds:reactoffersizatization needefersmoreflexibleise.4)

Netflix在框架選擇上主要考慮性能、可擴展性、開發效率、生態系統、技術債務和維護成本。 1.性能與可擴展性:選擇Java和SpringBoot以高效處理海量數據和高並發請求。 2.開發效率與生態系統:使用React提升前端開發效率,利用其豐富的生態系統。 3.技術債務與維護成本:選擇Node.js構建微服務,降低維護成本和技術債務。

Netflix主要使用React作為前端框架,輔以Vue用於特定功能。 1)React的組件化和虛擬DOM提升了Netflix應用的性能和開發效率。 2)Vue在Netflix的內部工具和小型項目中應用,其靈活性和易用性是關鍵。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SublimeText3漢化版
中文版,非常好用

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。
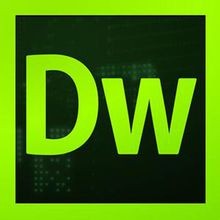
Dreamweaver CS6
視覺化網頁開發工具

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

禪工作室 13.0.1
強大的PHP整合開發環境