這篇文章主要介紹了React組件中的this的具體使用,現在分享給大家,也給大家做個參考。
React元件的this是什麼
透過寫一個簡單元件,並且渲染出來,分別列印出自訂函數和render中的this:
import React from 'react'; const STR = '被调用,this指向:'; class App extends React.Component{ constructor(){ super() } //测试函数 handler() { console.log(`handler ${STR}`,this); } render(){ console.log(`render ${STR}`,this); return( <p> <h1 id="hello-nbsp-World">hello World</h1> <label htmlFor = 'btn'>单击打印函数handler中this的指向</label> <input id = "btn" type="button" value = '单击' onClick = {this.handler}/> </p> ) } } export default App
結果如圖:
可以看到,render函數中的this指向了元件實例,而handler()函數中的this則為undefined,這是為何?
JavaScript函數中的this
我們都知道JavaScript函數中的this不是在函數宣告的時候定義的,而是在函數呼叫(即執行)的時候定義的
var student = { func: function() { console.log(this); }; }; student.func(); var studentFunc = student.func; studentFunc();
這段程式碼運行,可以看到student.func()列印了student對象,因為此時this指向student物件;而studentFunc()列印了window,因為此時由window調用的,this指向window。
這段程式碼形象的驗證了,JavaScript函數中的this不是在函數宣告的時候,而是在函數運行的時候定義的;
#同樣,React元件也遵循JavaScript的這個特性,所以元件方法的'呼叫者'不同會導致this的不同(這裡的「呼叫者」 指的是函數執行時的目前物件)
「呼叫者」不同導致this不同
測試:分別在元件自帶的生命週期函數以及自訂函數中列印this,並在render()方法中分別使用this.handler(),window.handler() ,onCilck={this.handler}這三種方法呼叫handler():
/App.jsx
//测试函数 handler() { console.log(`handler ${STR}`,this); } render(){ console.log(`render ${STR}`,this); this.handler(); window.handler = this.handler; window.handler(); return( <p> <h1 id="hello-nbsp-World">hello World</h1> <label htmlFor = 'btn'>单击打印函数handler中this的指向</label> <input id = "btn" type="button" value = '单击' onClick = {this.handler}/> </p> ) } } export default App
- render中this -> 元件實例App物件;
- #render中this.handler() -> 元件實例App物件;
- render中window.handler() -> window物件;
- onClick ={this.handler} -> undefined
import React from 'react' import {render,unmountComponentAtNode} from 'react-dom' import App from './App.jsx' const root=document.getElementById('root') console.log("首次挂载"); let instance = render(<App />,root); window.renderComponent = () => { console.log("挂载"); instance = render(<App />,root); } window.setState = () => { console.log("更新"); instance.setState({foo: 'bar'}); } window.unmountComponentAtNode = () => { console.log('卸载'); unmountComponentAtNode(root); }使用三個按鈕觸發元件的裝載、更新和卸載過程:/index.html
<!DOCTYPE html> <html> <head> <title>react-this</title> </head> <body> <button onclick="window.renderComponent()">挂载</button> <button onclick="window.setState()">更新</button> <button onclick="window.unmountComponentAtNode()">卸载</button> <p id="root"> <!-- app --> </p> </body> </html>運行程序,依序點擊“掛載”,綁定onClick={this.handler}“點擊”按鈕,“更新”和“卸載」按鈕結果如下:
自動綁定和手動綁定
- React.createClass有一個內建的魔法,可以自動綁定所使用的方法,使得其this指向元件的實例化對象,但是其他JavaScript類別並沒有這種特性;
- 所以React團隊決定不再React元件類別中實作自動綁定,把上下文轉換的自由權交給開發者;
- 所以我們通常在建構子中綁定方法的this指向:##
import React from 'react'; const STR = '被调用,this指向:'; class App extends React.Component{ constructor(){ super(); this.handler = this.handler.bind(this); } //测试函数 handler() { console.log(`handler ${STR}`,this); } render(){ console.log(`render ${STR}`,this); this.handler(); window.handler = this.handler; window.handler(); return( <p> <h1 id="hello-nbsp-World">hello World</h1> <label htmlFor = 'btn'>单击打印函数handler中this的指向</label> <input id = "btn" type="button" value = '单击' onClick = {this.handler}/> </p> ) } } export default App
將this.handler()綁定在設定為元件實例後,this.handler()中的this就指向群組將實例,即onClick={this.handler}列印出來的為元件實例;
React元件生命週期函數中的this指向元件實例;
自訂元件方法的this會因呼叫者不同而不同;
為了在元件的自訂方法中取得元件實例,需要手動綁定this到群組將實例。
上面是我整理給大家的,希望今後對大家有幫助。
相關文章:
vue取得目前啟動路由的方法vue中實作先請求資料再渲染dom分享解決vue頁面DOM操作不生效的問題以上是在React元件中詳細講解this的使用方法。的詳細內容。更多資訊請關注PHP中文網其他相關文章!
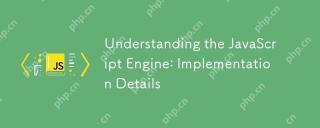
理解JavaScript引擎內部工作原理對開發者重要,因為它能幫助編寫更高效的代碼並理解性能瓶頸和優化策略。 1)引擎的工作流程包括解析、編譯和執行三個階段;2)執行過程中,引擎會進行動態優化,如內聯緩存和隱藏類;3)最佳實踐包括避免全局變量、優化循環、使用const和let,以及避免過度使用閉包。

Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
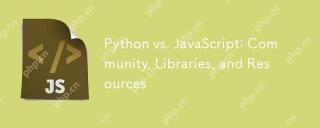
Python和JavaScript在社區、庫和資源方面的對比各有優劣。 1)Python社區友好,適合初學者,但前端開發資源不如JavaScript豐富。 2)Python在數據科學和機器學習庫方面強大,JavaScript則在前端開發庫和框架上更勝一籌。 3)兩者的學習資源都豐富,但Python適合從官方文檔開始,JavaScript則以MDNWebDocs為佳。選擇應基於項目需求和個人興趣。
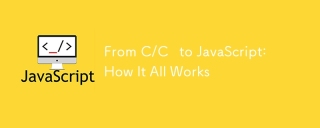
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
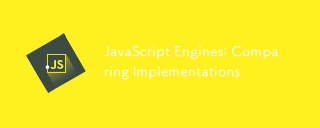
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
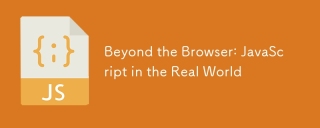
JavaScript在現實世界中的應用包括服務器端編程、移動應用開發和物聯網控制:1.通過Node.js實現服務器端編程,適用於高並發請求處理。 2.通過ReactNative進行移動應用開發,支持跨平台部署。 3.通過Johnny-Five庫用於物聯網設備控制,適用於硬件交互。
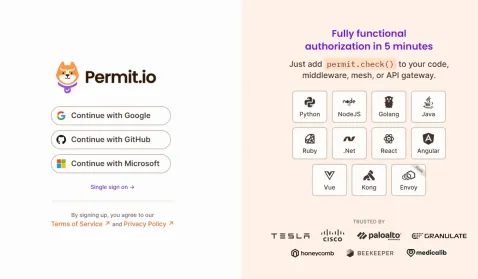
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
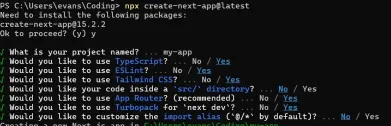
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

禪工作室 13.0.1
強大的PHP整合開發環境

SublimeText3 Linux新版
SublimeText3 Linux最新版

Atom編輯器mac版下載
最受歡迎的的開源編輯器
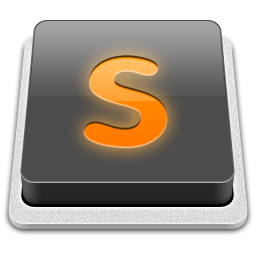
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
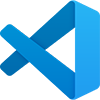
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器