這篇文章主要介紹了Python 統計字數的思路詳解,文中也給大家提供了不借助第三方模組的解決方法,有興趣的朋友一起看看吧
問題描述:
用Python 實作函數count_words(),該函數輸入字串s 和數字n,傳回s 中n 個出現頻率最高的單字。傳回值是元組列表,包含出現次數最高的n 個字及其次數,即[(, ), (, ), ... ],依出現次數降序排列。
您可以假設所有輸入都是小寫形式,且不含標點符號或其他字元(只包含字母和單個空格)。若出現次數相同,則依字母順序排列。
例如:
print count_words("betty bought a bit of butter but the butter was bitter",3)
輸出:
[('butter', 2), ('a ', 1), ('betty', 1)]
解決問題的想法:
1. 將字串s進行空白符號分割得到所有的單字清單split_s,如:['betty', 'bought', 'a', 'bit', 'of', 'butter', 'but', 'the', 'butter' , 'was', 'bitter']
2. 建立maplist,將split_s轉換為元素為元組的列表形式,如:[('betty', 1), ('bought', 1) , ('a', 1), ('bit', 1), ('of', 1), ('butter', 1), ('but', 1), ('the', 1), ( 'butter', 1), ('was', 1), ('bitter', 1)]
#3. 合併maplist中元素,元組的第一個索引值相同,則將其第二個索引值相加。
// 備註:準備採用defaultdict。得到的資料如下:{'betty': 1, 'bought': 1, 'a': 1, 'bit': 1, 'of': 1, 'butter': 2, 'but': 1, 'the ': 1, 'was': 1, 'bitter': 1}
4. 進行排序,依照key進行字母排序,得到如下:[('a', 1), ('betty', 1), ('bit', 1), ('bitter', 1), ('bought', 1), ('but', 1), ('butter', 2), ('of', 1) , ('the', 1), ('was', 1)]
5. 進行二次排序, 依照value進行排序,得到如下:[('butter', 2), ('a ', 1), ('betty', 1), ('bit', 1), ('bitter', 1), ('bought', 1), ('but', 1), ('of', 1), ('the', 1), ('was', 1)]
6. 使用切片取出頻率較高的*組資料
總結:在python3上不進行defaultdict進行排序結果也是正確的,python2上不正確。 defaultdict本身是沒有順序的,要區分列表,所以必須進行排序。
也可嘗試自己寫,不借助第三方模組
解決方案1(使用defaultdict):
from collections import defaultdict """Count words.""" def count_words(s, n): """Return the n most frequently occuring words in s.""" split_s = s.split() map_list = [(k,1) for k in split_s] output = defaultdict(int) for d in map_list: output[d[0]] += d[1] output1 = dict(output) top_n = sorted(output1.items(), key=lambda pair:pair[0], reverse=False) top_n = sorted(top_n, key=lambda pair:pair[1], reverse=True) return top_n[:n] def test_run(): """Test count_words() with some inputs.""" print(count_words("cat bat mat cat bat cat", 3)) print(count_words("betty bought a bit of butter but the butter was bitter", 4)) if __name__ == '__main__': test_run()
解決方案2(使用Counter)
from collections import Counter """Count words.""" def count_words(s, n): """Return the n most frequently occuring words in s.""" split_s = s.split() split_s = Counter(name for name in split_s) print(split_s) top_n = sorted(split_s.items(), key=lambda pair:pair[0], reverse=False) print(top_n) top_n = sorted(top_n, key=lambda pair:pair[1], reverse=True) print(top_n) return top_n[:n] def test_run(): """Test count_words() with some inputs.""" print(count_words("cat bat mat cat bat cat", 3)) print(count_words("betty bought a bit of butter but the butter was bitter", 4)) if __name__ == '__main__': test_run()
相關推薦:
以上是Python 統計字數的思路詳解的詳細內容。更多資訊請關注PHP中文網其他相關文章!
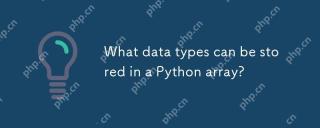
pythonlistscanStoryDatatepe,ArrayModulearRaysStoreOneType,and numpyArraySareSareAraysareSareAraysareSareComputations.1)列出sareversArversAtileButlessMemory-Felide.2)arraymoduleareareMogeMogeNareSaremogeNormogeNoreSoustAta.3)
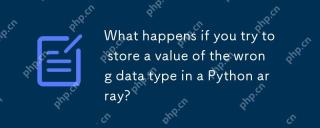
WhenyouattempttostoreavalueofthewrongdatatypeinaPythonarray,you'llencounteraTypeError.Thisisduetothearraymodule'sstricttypeenforcement,whichrequiresallelementstobeofthesametypeasspecifiedbythetypecode.Forperformancereasons,arraysaremoreefficientthanl
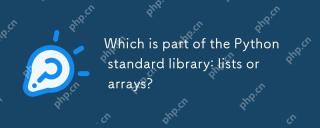
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
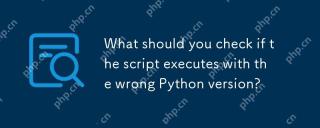
ThescriptisrunningwiththewrongPythonversionduetoincorrectdefaultinterpretersettings.Tofixthis:1)CheckthedefaultPythonversionusingpython--versionorpython3--version.2)Usevirtualenvironmentsbycreatingonewithpython3.9-mvenvmyenv,activatingit,andverifying
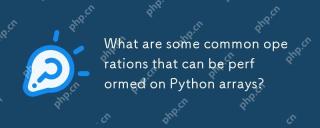
Pythonarrayssupportvariousoperations:1)Slicingextractssubsets,2)Appending/Extendingaddselements,3)Insertingplaceselementsatspecificpositions,4)Removingdeleteselements,5)Sorting/Reversingchangesorder,and6)Listcomprehensionscreatenewlistsbasedonexistin
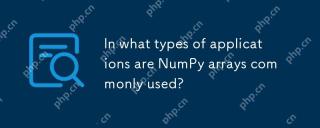
NumPyarraysareessentialforapplicationsrequiringefficientnumericalcomputationsanddatamanipulation.Theyarecrucialindatascience,machinelearning,physics,engineering,andfinanceduetotheirabilitytohandlelarge-scaledataefficiently.Forexample,infinancialanaly
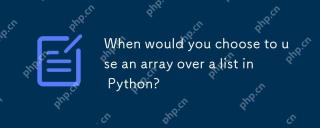
useanArray.ArarayoveralistinpythonwhendeAlingwithHomoGeneData,performance-Caliticalcode,orinterfacingwithccode.1)同質性data:arraysSaveMemorywithTypedElements.2)績效code-performance-calitialcode-calliginal-clitical-clitical-calligation-Critical-Code:Arraysofferferbetterperbetterperperformanceformanceformancefornallancefornalumericalical.3)
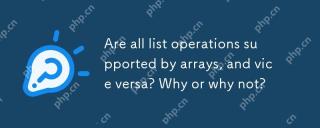
不,notalllistoperationsareSupportedByArrays,andviceversa.1)arraysdonotsupportdynamicoperationslikeappendorinsertwithoutresizing,wheremactsperformance.2)listssdonotguaranteeconecontanttanttanttanttanttanttanttanttanttimecomplecomecomplecomecomecomecomecomecomplecomectacccesslectaccesslecrectaccesslerikearraysodo。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

Atom編輯器mac版下載
最受歡迎的的開源編輯器
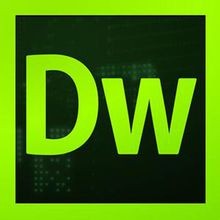
Dreamweaver CS6
視覺化網頁開發工具
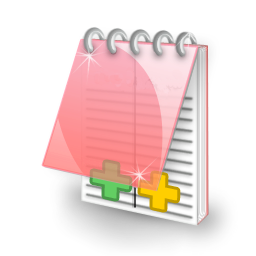
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
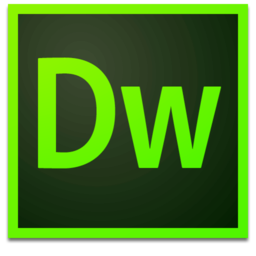
Dreamweaver Mac版
視覺化網頁開發工具

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!