分頁元件在web專案中是十分常見的元件,讓我們使用Vue建立可重複使用的分頁元件,關於基本架構和相關事件監聽大家參考下本文
Web應用程式中資源分頁不僅對效能很有幫助,而且從使用者體驗的角度來說也是非常有用的。在這篇文章中,將了解如何使用Vue建立動態和可用的分頁元件。
基本結構
分頁元件應該允許使用者訪問第一個和最後一個頁面,向前和向後移動,並直接切換到近距離的頁面。
大多數應用程式在使用者每次變更頁面時都會發出API請求。我們需要確保元件允許這樣做,但是我們不希望在元件內發出這樣的請求。這樣,我們將確保元件在整個應用程式中是可重複使用的,並且請求都是在操作或服務層中進行的。我們可以透過使用使用者點擊的頁面的數字觸發事件來實現此目的。
有幾種可能的方法來實作API端點上的分頁。對於這個例子,我們假設API告訴我們每個頁面的結果數量、頁面總數和目前頁面。這些將會是我們的動態 props 。
相反,如果API只告訴記錄的總數,那麼我們可以透過將結果的數量除以每一頁的結果數來計算頁數: totalResults / resultsPerPage 。
我們想要渲染一個按鈕到第一頁、 上一頁、 頁面數量範圍、 下一頁和最後一頁:
[first] [next] [1] [2 ] [3] [previous] [last]
例如像下圖這樣的一個效果:
儘管我們希望渲染一個系列的頁面,但並不希望渲染所有可用頁面。讓我們允許在我們的元件中設定一個最多可見按鈕的 props 。
既然我們知道了我們想要的元件要做成什麼,需要哪些數據,我們就可以設定HTML結構和所需的 props 。
<template id="pagination"> <ul class="pagination"> <li> <button type="button">« First</button> </li> <li> <button type="button">«</button> </li> <!-- 页数的范围 --> <li> <button type="button">Next »</button> </li> <li> <button type="button">»</button> </li> </ul> </template> Vue.component('pagination', { template: '#pagination', props: { maxVisibleButtons: { type: Number, required: false, default: 3 }, totalPages: { type: Number, required: true }, total: { type: Number, required: true }, currentPage: { type: Number, required: true } } })
上面的程式碼註冊了一個pagination 元件,如果呼叫這個元件:
<p id="app"> <pagination></pagination> </p>
這時候看到的效果如下:
注意,為了能讓元件看起來好看一點,給組件添加了一點樣式。
事件監聽
現在我們需要通知父元件,當使用者點擊按鈕時,使用者點擊了哪個按鈕。
我們需要為每個按鈕新增一個事件監聽器。 v-on 指令 允許偵聽DOM事件。在本例中,我將使用 v-on 的快捷鍵 來偵聽單擊事件。
為了通知父節點,我們將使用 $emit 方法 來發出一個帶有頁面點擊的事件。
我們也要確保分頁按鈕只有在頁面可用時才唯一一個目前狀態。為了這樣做,將使用 v-bind 將 disabled 屬性的值與目前頁面綁定。我們還是使用 :v-bind 的快捷鍵 : 。
為了保持我們的 template 乾淨,將使用 computed 屬性 來檢查按鈕是否已停用。使用 computed 也會被緩存,這意味著只要 currentPage 不會更改,對相同計算屬性的幾個訪問將返回先前計算的結果,而不必再次運行該函數。
<template id="pagination"> <ul class="pagination"> <li> <button type="button" @click="onClickFirstPage" :disabled="isInFirstPage">« First</button> </li> <li> <button type="button" @click="onClickPreviousPage" :disabled="isInFirstPage">«</button> </li> <li v-for="page in pages"> <button type="button" @click="onClickPage(page.name)" :disabled="page.isDisabled"> {{ page.name }}</button> </li> <li> <button type="button" @click="onClickNextPage" :disabled="isInLastPage">Next »</button> </li> <li> <button type="button" @click="onClickLastPage" :disabled="isInLastPage">»</button> </li> </ul> </template> Vue.component('pagination', { template: '#pagination', props: { maxVisibleButtons: { type: Number, required: false, default: 3 }, totalPages: { type: Number, required: true }, total: { type: Number, required: true }, currentPage: { type: Number, required: true } }, computed: { isInFirstPage: function () { return this.currentPage === 1 }, isInLastPage: function () { return this.currentPage === this.totalPages } }, methods: { onClickFirstPage: function () { this.$emit('pagechanged', 1) }, onClickPreviousPage: function () { this.$emit('pagechanged', this.currentPage - 1) }, onClickPage: function (page) { this.$emit('pagechanged', page) }, onClickNextPage: function () { this.$emit('pagechanged', this.currentPage + 1) }, onClickLastPage: function () { this.$emit('pagechanged', this.totalPages) } } })
在呼叫pagination 元件時,將totalPages 和total 以及currentPage 傳到元件中:
<p id="app"> <pagination :total-pages="11" :total="120" :current-page="currentPage"></pagination> </p> let app = new Vue({ el: '#app', data () { return { currentPage: 2 } } })
執行上面的程式碼,將會報錯:
不難發現,在pagination元件中,咱們還少了pages 。從前面介紹的內容,我們不難發現,需要計算 pages 的值。
Vue.component('pagination', { template: '#pagination', props: { maxVisibleButtons: { type: Number, required: false, default: 3 }, totalPages: { type: Number, required: true }, total: { type: Number, required: true }, currentPage: { type: Number, required: true } }, computed: { isInFirstPage: function () { return this.currentPage === 1 }, isInLastPage: function () { return this.currentPage === this.totalPages }, startPage: function () { if (this.currentPage === 1) { return 1 } if (this.currentPage === this.totalPages) { return this.totalPages - this.maxVisibleButtons + 1 } return this.currentPage - 1 }, endPage: function () { return Math.min(this.startPage + this.maxVisibleButtons - 1, this.totalPages) }, pages: function () { const range = [] for (let i = this.startPage; i <= this.endPage; i+=1) { range.push({ name: i, isDisabled: i === this.currentPage }) } return range } }, methods: { onClickFirstPage: function () { this.$emit('pagechanged', 1) }, onClickPreviousPage: function () { this.$emit('pagechanged', this.currentPage - 1) }, onClickPage: function (page) { this.$emit('pagechanged', page) }, onClickNextPage: function () { this.$emit('pagechanged', this.currentPage + 1) }, onClickLastPage: function () { this.$emit('pagechanged', this.totalPages) } } })
這個時候得到的結果不再報錯,你在瀏覽器中會看到下圖這樣的效果:
新增樣式
現在我們的元件實作了原本想要的所有功能,而且添加了一些樣式,讓它看起來更像一個分頁元件,而不僅像是一個清單。
我們也希望使用者能夠清楚地辨識他們所在的頁面。讓我們改變表示目前頁面的按鈕的顏色。
為此,我們可以使用物件語法將HTML類別綁定到目前頁面按鈕。當使用物件語法綁定類別名稱時,Vue將在值變更時自動切換類別。
虽然 v-for 中的每个块都可以访问父作用域范围,但是我们将使用 method 来检查页面是否处于 active 状态,以便保持我们的 templage 干净。
Vue.component('pagination', { template: '#pagination', props: { maxVisibleButtons: { type: Number, required: false, default: 3 }, totalPages: { type: Number, required: true }, total: { type: Number, required: true }, currentPage: { type: Number, required: true } }, computed: { isInFirstPage: function () { return this.currentPage === 1 }, isInLastPage: function () { return this.currentPage === this.totalPages }, startPage: function () { if (this.currentPage === 1) { return 1 } if (this.currentPage === this.totalPages) { return this.totalPages - this.maxVisibleButtons + 1 } return this.currentPage - 1 }, endPage: function () { return Math.min(this.startPage + this.maxVisibleButtons - 1, this.totalPages) }, pages: function () { const range = [] for (let i = this.startPage; i <= this.endPage; i+=1) { range.push({ name: i, isDisabled: i === this.currentPage }) } return range } }, methods: { onClickFirstPage: function () { this.$emit('pagechanged', 1) }, onClickPreviousPage: function () { this.$emit('pagechanged', this.currentPage - 1) }, onClickPage: function (page) { this.$emit('pagechanged', page) }, onClickNextPage: function () { this.$emit('pagechanged', this.currentPage + 1) }, onClickLastPage: function () { this.$emit('pagechanged', this.totalPages) }, isPageActive: function (page) { return this.currentPage === page; } } })
接下来,在 pages 中添加当前状态:
<li v-for="page in pages"> <button type="button" @click="onClickPage(page.name)" :disabled="page.isDisabled" :class="{active: isPageActive(page.name)}"> {{ page.name }}</button> </li>
这个时候你看到效果如下:
但依然还存在一点点小问题,当你在点击别的按钮时, active 状态并不会随着切换:
继续添加代码改变其中的效果:
let app = new Vue({ el: '#app', data () { return { currentPage: 2 } }, methods: { onPageChange: function (page) { console.log(page) this.currentPage = page; } } })
在调用组件时:
<p id="app"> <pagination :total-pages="11" :total="120" :current-page="currentPage" @pagechanged="onPageChange"></pagination> </p>
这个时候的效果如下了:
到这里,基本上实现了咱想要的分页组件效果。
无障碍化处理
熟悉Bootstrap的同学都应该知道,Bootstrap中的组件都做了无障碍化的处理,就是在组件中添加了WAI-ARIA相关的设计。比如在分页按钮上添加 aria-label 相关属性:
在我们这个组件中,也相应的添加有关于WAI-ARIA相关的处理:
<template id="pagination"> <ul class="pagination" aria-label="Page navigation"> <li> <button type="button" @click="onClickFirstPage" :disabled="isInFirstPage" aria-label="Go to the first page">« First</button> </li> <li> <button type="button" @click="onClickPreviousPage" :disabled="isInFirstPage" aria-label="Previous">«</button> </li> <li v-for="page in pages"> <button type="button" @click="onClickPage(page.name)" :disabled="page.isDisabled" :aria-label="`Go to page number ${page.name}`"> {{ page.name }}</button> </li> <li> <button type="button" @click="onClickNextPage" :disabled="isInLastPage" aria-label="Next">Next »</button> </li> <li> <button type="button" @click="onClickLastPage" :disabled="isInLastPage" aria-label="Go to the last page">»</button> </li> </ul> </template>
这样有关于 aria 相关的属性就加上了:
最终的效果如下,可以点击下面的连接访问:
相关推荐:
以上是使用Vue建立可重複使用的分頁元件的詳細內容。更多資訊請關注PHP中文網其他相關文章!
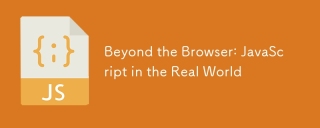
JavaScript在現實世界中的應用包括服務器端編程、移動應用開發和物聯網控制:1.通過Node.js實現服務器端編程,適用於高並發請求處理。 2.通過ReactNative進行移動應用開發,支持跨平台部署。 3.通過Johnny-Five庫用於物聯網設備控制,適用於硬件交互。
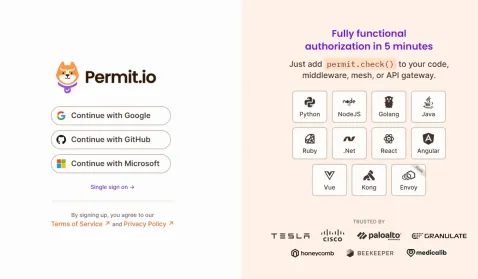
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
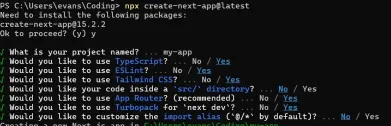
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
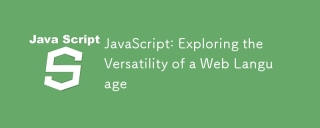
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
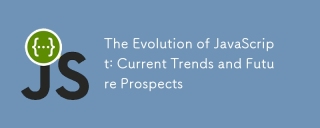
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
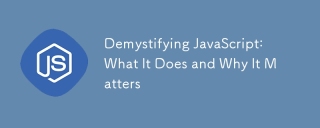
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
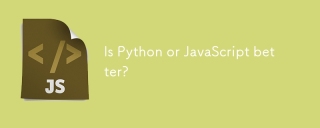
Python更适合数据科学和机器学习,JavaScript更适合前端和全栈开发。1.Python以简洁语法和丰富库生态著称,适用于数据分析和Web开发。2.JavaScript是前端开发核心,Node.js支持服务器端编程,适用于全栈开发。
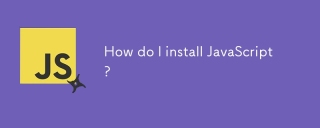
JavaScript不需要安裝,因為它已內置於現代瀏覽器中。你只需文本編輯器和瀏覽器即可開始使用。 1)在瀏覽器環境中,通過標籤嵌入HTML文件中運行。 2)在Node.js環境中,下載並安裝Node.js後,通過命令行運行JavaScript文件。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

記事本++7.3.1
好用且免費的程式碼編輯器

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。