本文主要跟大家介紹Vuex 模組化(module),小編覺得蠻不錯的,現在分享給大家,也給大家做個參考,希望能幫助到大家。
一、為什麼需要模組化
前面我們講到的例子都在一個狀態樹裡進行,當一個項目比較大時,所有的狀態都集中在一起會得到一個比較大的對象,進而顯得臃腫,難以維持。為了解決這個問題,Vuex允許我們將store分割成模組(module),每個module都有自己的state,mutation,action,getter,甚至可以往下嵌套模組,下面我們看一個典型的模組化範例
const moduleA = { state: {....}, mutations: {....}, actions: {....}, getters: {....} } const moduleB = { state: {....}, mutations: {....}, actions: {....}, getters: {....} } const store = new Vuex.Store({ modules: { a: moduleA, b: moduleB } }) store.state.a // moduleA的状态 store.state.b // moduleB的状态
二、模組的局部狀態
模組內部的mutation和getter,接收的第一參數(state)是模組的局部狀態物件,rootState
const moduleA = { state: { count: 0}, mutations: { increment (state) { // state是模块的局部状态,也就是上面的state state.count++ } }, getters: { doubleCount (state, getters, rootState) { // 参数 state为当前局部状态,rootState为根节点状态 return state.count * 2 } }, actions: { incremtnIfOddRootSum ( { state, commit, rootState } ) { // 参数 state为当前局部状态,rootState为根节点状态 if ((state.cont + rootState.count) % 2 === 1) { commit('increment') } } } }
三、命名空間(這裡一定要看,不然有些時候會被坑)
上面所有的例子中,模組內部的action、mutation、getter是註冊在全域命名空間的,如果你在moduleA和moduleB裡分別宣告了命名相同的action或mutation或getter(叫some),當你使用store.commit('some'),A和B模組會同時回應。所以,如果你希望你的模組更加自包含和提高可重複使用性,你可以添加namespaced: true的方式,使其成為命名空間模組。當模組註冊後,它的所有getter,action,mutation都會自動根據模組註冊的路徑調用整個命名,例如:
const store = new Vuex.Store({ modules: { account: { namespaced: true, state: {...}, // 模块内的状态已经是嵌套的,namespaced不会有影响 getters: { // 每一条注释为调用方法 isAdmin () { ... } // getters['account/isAdmin'] }, actions: { login () {...} // dispatch('account/login') }, mutations: { login () {...} // commit('account/login') }, modules: { // 继承父模块的命名空间 myPage : { state: {...}, getters: { profile () {...} // getters['account/profile'] } }, posts: { // 进一步嵌套命名空间 namespaced: true, getters: { popular () {...} // getters['account/posts/popular'] } } } } } })
啟用了命名空間的getter和action會收到局部化的getter, dispatch和commit。你在使用模組內容時不需要再在同一模組內加入空間名稱前綴,更改namespaced屬性後不需要修改模組內的程式碼。
四、在命名空間模組內存取全域內容(Global Assets)
如果你希望使用全域state和getter,roorState和rootGetter會作為第三和第四參數傳入getter,也會透過context物件的屬性傳入action若需要在全域命名空間內分發action或提交mutation,將{ root: true }作為第三參數傳給dispatch或commit即可。
modules: { foo: { namespaced: true, getters: { // 在这个被命名的模块里,getters被局部化了 // 你可以使用getter的第四个参数来调用 'rootGetters' someGetter (state, getters, rootSate, rootGetters) { getters.someOtherGetter // -> 局部的getter, ‘foo/someOtherGetter' rootGetters.someOtherGetter // -> 全局getter, 'someOtherGetter' } }, actions: { // 在这个模块里,dispatch和commit也被局部化了 // 他们可以接受root属性以访问跟dispatch和commit smoeActino ({dispatch, commit, getters, rootGetters }) { getters.someGetter // 'foo/someGetter' rootGetters.someGetter // 'someGetter' dispatch('someOtherAction') // 'foo/someOtherAction' dispatch('someOtherAction', null, {root: true}) // => ‘someOtherAction' commit('someMutation') // 'foo/someMutation' commit('someMutation', null, { root: true }) // someMutation } } } }
五、有命名空間的綁定函數
前面說過,帶了命名空間後,呼叫時必須要寫上命名空間,但是這樣就比較繁瑣,尤其涉及到多層嵌套時(當然開發中別嵌套太多,會暈。。)
下面我們看下一般寫法
computed: { ...mapState({ a: state => state.some.nested.module.a, b: state => state.some.nested.module.b }), methods: { ...mapActions([ 'some/nested/module/foo', 'some/nested/module/bar' ]) } }
對於這種情況,你可以將模組的命名空間作為第一個參數傳遞給上述函數,這樣所有的綁定會自動將該模組作為上下文。簡化寫入就是
computed: { ...mapStates('some/nested/module', { a: state => state.a, b: state => state.b }) }, methods: { ...mapActions('some/nested/module',[ 'foo', 'bar' ]) }
六、模組重複使用
#有時我們可能會建立一個模組的多個實例,例如:
- ##建立多個store,他們共用一個模組
- 在一個store中多次註冊同一個模組
實際上Vue元件內data是同樣的問題,因此解決辦法也是一樣的,使用一個函數來宣告模組狀態(2.3.0+支援)
const MyModule = { state () { return { foo: 'far' } } }七、總結到這裡模組化(module)的內容就已經講完了,本次主要講解了module出現的原因,使用方法,全局和局部namespaced模組命名空間,局部訪問全局內容,map函數帶有命名空間的綁定函數和模組的重用。 引用https://vuex.vuejs.org Vuex官方文件#相關推薦:
以上是Vuex模組化(module)實例詳解的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

記事本++7.3.1
好用且免費的程式碼編輯器
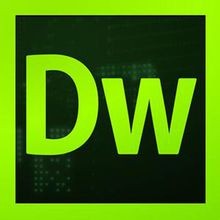
Dreamweaver CS6
視覺化網頁開發工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具