Vuex 強調使用單一狀態樹,也就是在一個專案裡只有一個 store,這個 store 集中管理了專案中所有的資料以及對資料的操作行為。但這樣帶來的問題是 store 可能會非常臃腫龐大不易維護,所以就需要對狀態樹進行模組化的分割。
範例教學
範例是在vue-cli基礎上建構的,以下是src檔案下的內容目錄。
├── App.vue ├── components // 组件文件夹 │ ├── tab1.vue │ ├── tab2.vue │ ├── tab3.vue │ └── tab4.vue ├── main.js // vue的主文件入口 ├── router // vue-router文件 │ └── index.js └── store // vuex文件 ├── action.js // action ├── getter.js // getter ├── index.js // vuex的主文件 ├── module // 模块文件 │ ├── tab2.js │ └── tab3.js ├── mutation-type.js // mutation常量名文件 └── mutation.js // mutation
效果是這樣的(不要嫌棄簡陋啊啊啊)
#在這個例子裡,把文檔裡提到的vuex的相關知識都使用了一遍,包括模組相關的知識,基本上把一般的使用場景都覆蓋了吧。
那不廢話了,開始吧。
首先app.vue和router兩部分是和路由相關,就是很簡單的東西,看看文件就能了解。
vuex的模組化
在寫這個例子之前看了很多的開源專案的程式碼,一開始蠻新鮮的,畢竟之前專案中並沒有深度使用過vuex,基本上就是一個store.js把vuex的功能就都完成了,但是專案複雜肯定不能這麼寫,剛好現在有這個需求,我就想寫個例子理一理這方面的思路。結果還是蠻簡單的。
store檔案裡的內容就是按照vuex五個核心概念建立的,這麼做的好處對於梳理業務邏輯和後期維護都是極大的方便,例如mutation.js和mutation-type.js這兩個檔案:
// mutation-type.js const CHANGE_COUNT = 'CHANGE_COUNT'; export default { CHANGE_COUNT }
// mutation.js import type from './mutation-type' let mutations = { [type.CHANGE_COUNT](state) { state.count++ } } export default mutations
將mutation中的方法名稱單獨作為常數提取出來,放在單獨的檔案中,用的時候引用相關的內容,這樣非常便於管理和了解有哪些方法存在,很直觀。另一方面,有時候可能需要用到action,可以使用相同的方法名,只要再引入常數的檔案就行。
// action.js import type from './mutation-type' let actions = { [type.CHANGE_COUNT]({ commit }) { commit(type.CHANGE_COUNT) } } export default actions
怎麼樣,這樣是不是看起來就沒有寫在一個檔案裡那麼亂了。
...mapGetters和...mapActions
tab1.vue裡:
// tab1.vue <template> <p> </p> <p>这是tab1的内容</p> <em>{{count}}</em> <p>getter:{{NewArr}}</p> </template> <script> import { mapActions, mapGetters } from "vuex"; import type from "../store/mutation-type"; export default { computed: { ...mapGetters([ 'NewArr' ]), count: function() { return this.$store.state.count; }, }, methods: { ...mapActions({ CHANGE_COUNT: type.CHANGE_COUNT }), add: function() { this.CHANGE_COUNT(type.CHANGE_COUNT); } } }; </script> <style> </style>
index.js檔案裡:
import Vuex from 'vuex' import Vue from 'vue' import actions from './action' import mutations from './mutation' import getters from './getter' import tab2 from './module/tab2' import tab3 from './module/tab3' Vue.use(Vuex) let state = { count: 1, arr:[] } let store = new Vuex.Store({ state, getters, mutations, actions, modules:{ tab2,tab3 } }) export default store
vuex提供了一種叫做輔助函數的東西,他的好處能讓你在一個頁面集中展示一些需要用到的東西,並且在使用的時候也可以少寫一些內容,不過這個不是必須,根據自己需要取用。
要說明的是,他們兩個生效的地方可不一樣。
...mapGetters寫在本頁面的計算屬性中,之後就可以像使用計算屬性一樣使用getters裡的內容了。
...mapActions寫在本頁面的methods裡面,既可以在其他方法裡調用,甚至可以直接寫在@click裡面,像這樣:
<em>{{count}}</em>
醬紫,tab1裡面的數字每次點擊都會自增1。
mudule
vuex的文檔裡對於模組這部分寫的比較模糊,還是得自己實際使用才能行。
在這個例子中,我設定了兩個模組:tab2和tab3,分別對應著同名的兩個元件,當然,我這樣只是為了測試,實際看tab2就可以。
// module/tab2.js const tab2 = { state: { name:`这是tab2模块的内容` }, mutations:{ change2(state){ state.name = `我修改了tab2模块的内容` } }, getters:{ name(state,getters,rootState){ console.log(rootState) return state.name + ',使用getters修改' } } } export default tab2;
// tab2.vue <template> <p> </p> <p>这是tab2的内容</p> <strong>点击使用muttion修改模块tab2的内容:{{info}}</strong> <h4 id="newInfo">{{newInfo}}</h4> </template> <script> export default { mounted() { // console.log(this.$store.commit('change2')) }, computed: { info: function() { return this.$store.state.tab2.name; }, newInfo(){ return this.$store.getters.name; } }, methods: { change() { this.$store.commit('change2') } } }; </script> <style> </style>
這個例子裡主要是看怎麼在頁面中呼叫模組中的stated等。
首先說state,這個很簡單,在頁面中這樣寫就行:
this.$store.steta.tab2(模块名).name
在本頁面的mounted中console一下$store,可以看到模組中,stete加了一層嵌套在state的。
至於action,mutation,getter,和一般的使用方法一樣,沒有任何差別。
還有就是,在getter和action中,可以透過rootState取得根結構的state,mutation中沒有此方法。
相關推薦:
以上是學會簡單的vuex與模組化的詳細內容。更多資訊請關注PHP中文網其他相關文章!
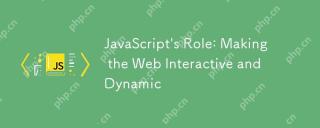
JavaScript是現代網站的核心,因為它增強了網頁的交互性和動態性。 1)它允許在不刷新頁面的情況下改變內容,2)通過DOMAPI操作網頁,3)支持複雜的交互效果如動畫和拖放,4)優化性能和最佳實踐提高用戶體驗。
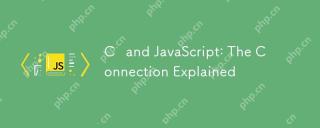
C 和JavaScript通過WebAssembly實現互操作性。 1)C 代碼編譯成WebAssembly模塊,引入到JavaScript環境中,增強計算能力。 2)在遊戲開發中,C 處理物理引擎和圖形渲染,JavaScript負責遊戲邏輯和用戶界面。
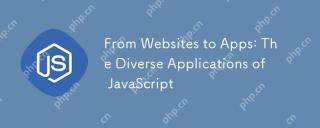
JavaScript在網站、移動應用、桌面應用和服務器端編程中均有廣泛應用。 1)在網站開發中,JavaScript與HTML、CSS一起操作DOM,實現動態效果,並支持如jQuery、React等框架。 2)通過ReactNative和Ionic,JavaScript用於開發跨平台移動應用。 3)Electron框架使JavaScript能構建桌面應用。 4)Node.js讓JavaScript在服務器端運行,支持高並發請求。
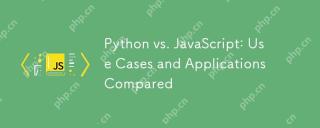
Python更適合數據科學和自動化,JavaScript更適合前端和全棧開發。 1.Python在數據科學和機器學習中表現出色,使用NumPy、Pandas等庫進行數據處理和建模。 2.Python在自動化和腳本編寫方面簡潔高效。 3.JavaScript在前端開發中不可或缺,用於構建動態網頁和單頁面應用。 4.JavaScript通過Node.js在後端開發中發揮作用,支持全棧開發。
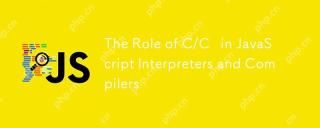
C和C 在JavaScript引擎中扮演了至关重要的角色,主要用于实现解释器和JIT编译器。1)C 用于解析JavaScript源码并生成抽象语法树。2)C 负责生成和执行字节码。3)C 实现JIT编译器,在运行时优化和编译热点代码,显著提高JavaScript的执行效率。
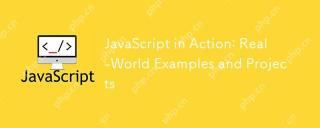
JavaScript在現實世界中的應用包括前端和後端開發。 1)通過構建TODO列表應用展示前端應用,涉及DOM操作和事件處理。 2)通過Node.js和Express構建RESTfulAPI展示後端應用。
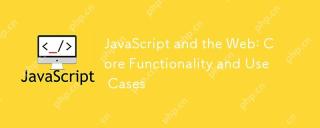
JavaScript在Web開發中的主要用途包括客戶端交互、表單驗證和異步通信。 1)通過DOM操作實現動態內容更新和用戶交互;2)在用戶提交數據前進行客戶端驗證,提高用戶體驗;3)通過AJAX技術實現與服務器的無刷新通信。
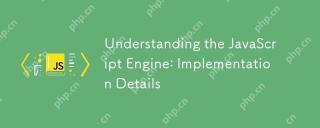
理解JavaScript引擎內部工作原理對開發者重要,因為它能幫助編寫更高效的代碼並理解性能瓶頸和優化策略。 1)引擎的工作流程包括解析、編譯和執行三個階段;2)執行過程中,引擎會進行動態優化,如內聯緩存和隱藏類;3)最佳實踐包括避免全局變量、優化循環、使用const和let,以及避免過度使用閉包。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
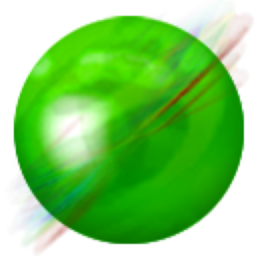
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境
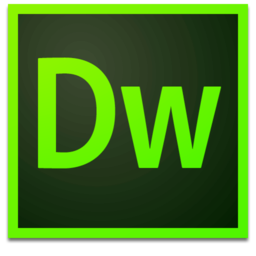
Dreamweaver Mac版
視覺化網頁開發工具
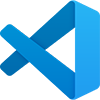
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

Atom編輯器mac版下載
最受歡迎的的開源編輯器

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。