進行學生資訊管理,其中key為學生ID,value為學生物件。 2.透過鍵盤輸入學生資訊3.對集合中的學生資訊進行增刪該查操作先建立一個StuMap類別來測試Map的使用方法。如下:
1 /** 2 * 学生类的Map集合类 3 *
4 * @author acer 5 * 6 */ 7 public class StuMap { 8 // 用来承装学生类型对象 9 private Map<String, Student> students;10 private static Scanner in;11 {12 in = new Scanner(System.in);13 }14 15 public StuMap() {16 students = new HashMap<String, Student>();17 18 }19 //省略方法,下面的方法会逐个列出20 }
>>>>>>>>>>>>>>>>>>>>>>>> ;>>>>>>>>>>>>>>>>>>>>>>>>> ;>
和List介面不同,向Map新增物件使用的是put(key,value)方法。以下是使用範例:
1 /* 2 * 添加学生类 输入学生id, 3 * 判断是否被占用 若未被占用,则输入姓名,创建新的学生对象,并且把该对象添加到Map中 4 * 否则,则提示已有该id 5 */ 6 public void AddStu() { 7 System.out.println("请输入要添加的学生id:"); 8 String Id = in.next();// 接受输入的id 9 Student st=students.get(Id);10 if(st==null){11 12 System.out.println("请输入要添加的学生姓名:");13 String name = in.next();// 接受输入的name14 this.students.put(Id, new Student(Id, name));15 }else{16 System.out.println("此Id已被占用!");17 }18 19 }
再寫一個列印輸出的測試函數,如:
1 /* 2 * 打印学生类 3 *
4 */ 5 public void PrintStu() { 6 System.out.println("总共有"+this.students.size()+"名学生:"); 7 //遍历keySet 8 for (String s : this.students.keySet()) { 9 Student st=students.get(s);10 if(st!=null){11 System.out.println("学生:" + students.get(s).getId() + "," + students.get(s).getName());12 }13 }14 }
上面的範例是使用Map的keySet()傳回Map中的key的Set集合再用if來判斷輸出,在Map還可以用entrySet()的方法傳回Map中的鍵值對Entry,如:
1 /* 2 * 通过entrySet方法遍历Map 3 */ 4 public void EntrySet(){ 5 Set<Entry<String, Student>> entrySet =students.entrySet(); 6 for(Entry<String, Student> entry:entrySet){ 7 System.out.println("取得建:"+entry.getKey()); 8 System.out.println("对应的值:"+entry.getValue().getName()); 9 10 }11 }
最後我們用主函數呼叫這些函數來看看效果如何
1 public static void main(String[] args) {2 StuMap stu = new StuMap();3 for (int i = 0; i < 3; i++) {4 stu.AddStu();5 }6 stu.PrintStu();7 stu.EntrySet();8 }
程式碼分析:1.student.get(ID)是採用Map的get()方法,偵測是否存在值為ID的學生,如果沒有,則傳回null。 這裡的案例是把Map中的key值設為學生的ID值,所以可以用這樣的方式來偵測,如果key值是學生其他屬則性另當別論! !
2.keySet()方法,傳回所有鍵的Set集合。 3.keyset()傳回Map中所有的key以集合的形式可用Set集合接收,HashMap當中的映射是無序的。 3.Map也可以用entrySet()的方法回傳Map中的鍵值對Entry,Entry也是Set集合,它可以呼叫getKey()和getValue()方法來分別得到鍵值對的“鍵”和“值”。
運行結果: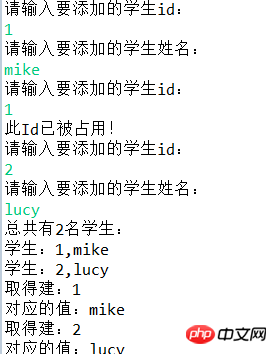
三、學生選課-刪除Map中的學生刪除Map中的鍵值對是呼叫remove(object key)方法,下面是它的使用範例: 1 /* 2 * 删除map中映射 3 */ 4 public void RemoveStu(){ 5 do{ 6 System.out.println("请输入要删除的学生id:"); 7 String Id = in.next();// 接受输入的id 8 Student st=students.get(Id); 9 if(st==null){10 System.out.println("此id不存在!");11 12 }else{13 this.students.remove(Id);14 System.out.println("成功删除"+st.getId()+","+st.getName()+"同学");15 break;16 }17 }while(true);18 }
運行結果: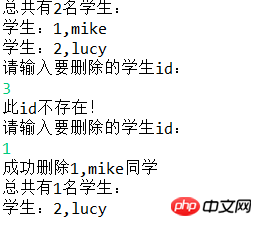
四、學生選課-修改Map中的學生修改Map中的鍵值對有兩種方法,第一種就是用put方法。其實就是添加方法中的put,使用方法跟添加無異,這裡的本質就是利用put把原來的資料給覆蓋掉,也就是修改。
1 /* 2 * 利用put方法修改Map中的value值 3 */ 4 public void ModifyStu(){ 5 do{ 6 System.out.println("请输入要修改的学生id:"); 7 String Id = in.next();// 接受输入的id 8 Student st=students.get(Id); 9 if(st==null){10 System.out.println("此id不存在!");11 12 }else{13 System.out.println("学生原来的姓名:"+st.getName()+",请输入修改后的姓名:");14 String name = in.next();// 接受输入的name15 st=new Student(Id,name);16 this.students.put(Id,st);17 System.out.println("成功修改!修改后的学生为:"+st.getId()+","+st.getName()+"同学");18 break;19 }20 }while(true);21 22 }
除了用put方法外,Map中提供一個叫做replace的方法,知名知其意,就是「替換」的意思。 replace的方法使用和put方法一樣,這是因為它的內部原始碼如下:1 if (map.containsKey(key)) {2 return map.put(key, value);3 } else4 return null;5
可以看出replace方法就是调用put方法来完成修改操作的,但是我们为了和添加put进行区分,最好在使用修改的时候用replace方法进行修改。这样的代码可读性和维护性就增强了。
那么使用replace修改Map中的value值如下:(推荐使用replace方法)
1 /* 2 * 利用replace方法修改Map中的value值 3 */ 4 public void Modify(){ 5 do{ 6 System.out.println("请输入要修改的学生id:"); 7 String Id = in.next();// 接受输入的id 8 Student st=students.get(Id); 9 if(st==null){10 System.out.println("此id不存在!");11 12 }else{13 System.out.println("学生原来的姓名:"+st.getName()+",请输入修改后的姓名:");14 String name = in.next();// 接受输入的name15 st=new Student(Id,name);16 this.students.replace(Id, st);17 System.out.println("成功修改!修改后的学生为:"+st.getId()+","+st.getName()+"同学");18 break;19 }20 }while(true);21 }
运行结果:
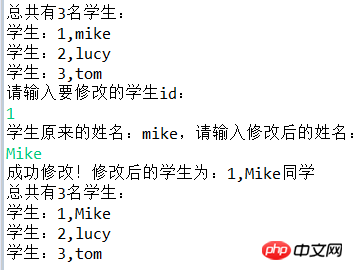
五、总结
Map -特点:元素成对出现,key-value,是映射关系,key不能重复,但value可以重复,也就是说,可以多key对一个value。支持泛型如Map。-实现类:HashMap是最常用的,HashMap中是无序排列,其元素中key或value可为null(但只能有一个为null)。
-声明(泛型)举例: 在类中声明 public Map<类型1, 类型2> xxx; 然后再构造方法中this.xxx = new HashMap<类型1, 类型2();
-获取:yy temp = xxx.get(key)
-添加:xxx.put( key(xx型), zz(yy型) );
-返回map中所有key(返回是set型集合形式) set xxxxx = xxx.keyset(); 用于遍历。
-返回map中所有entry对(key-value对)(返回是set型集合形式) set> xxxxx = xxx.entrySet(); 同样用于遍历。 遍历时:for(Entry 元素: xxxxx)
-删除:xxx.remove(key);
-修改:可以用put,当put方法传入的key存在就相当于是修改(覆盖);但是推荐使用replace方法!
以上是Map&HashMapde的簡單介紹的詳細內容。更多資訊請關注PHP中文網其他相關文章!
陳述:本文內容由網友自願投稿,版權歸原作者所有。本站不承擔相應的法律責任。如發現涉嫌抄襲或侵權的內容,請聯絡admin@php.cn