ThreadLocal翻譯成中文比較準確的叫法應該是:線程局部變數。
這個玩意有什麼用處,或者說為什麼要有這麼一個東東?先解釋一下,在並發程式設計的時候,成員變數如果不做任何處理其實是線程不安全的,各個線程都在操作同一個變量,顯然是不行的,並且我們也知道volatile這個關鍵字也是不能保證線程安全的。那麼在有一種情況之下,我們需要滿足這樣一個條件:變數是同一個,但是每個執行緒都使用同一個初始值,也就是使用同一個變數的新的副本。這種情況之下ThreadLocal就非常使用,比如說DAO的資料庫連接,我們知道DAO是單例的,那麼他的屬性Connection就不是線程安全的變數。而我們每個線程都需要使用他,並且各自使用各自的。這種情況,ThreadLocal就比較好的解決了這個問題。
我們從原始碼的角度來分析這個問題。
先定義一個ThreadLocal:
ThreadLocal<connection> tl = ThreadLocal<connection> Connection initConn = = DriverManager.getConnection("url, name and password"=( ==</connection></connection>
package java.lang;import java.lang.ref.*;import java.util.concurrent.atomic.AtomicInteger;public class ThreadLocal<t> { private final int threadLocalHashCode = nextHashCode(); private static AtomicInteger nextHashCode =new AtomicInteger();private static final int HASH_INCREMENT = 0x61c88647;private static int nextHashCode() {return nextHashCode.getAndAdd(HASH_INCREMENT); }protected T initialValue() {return null; }public ThreadLocal() { }public T get() { Thread t = Thread.currentThread(); ThreadLocalMap map = getMap(t);if (map != null) { ThreadLocalMap.Entry e = map.getEntry(this);if (e != null)return (T)e.value; }return setInitialValue(); }private T setInitialValue() { T value = initialValue(); Thread t = Thread.currentThread(); ThreadLocalMap map = getMap(t);if (map != null) map.set(this, value);elsecreateMap(t, value);return value; }public void set(T value) { Thread t = Thread.currentThread(); ThreadLocalMap map = getMap(t);if (map != null) map.set(this, value);elsecreateMap(t, value); } public void remove() { ThreadLocalMap m = getMap(Thread.currentThread()); if (m != null) m.remove(this); }ThreadLocalMap getMap(Thread t) {return t.threadLocals; }void createMap(Thread t, T firstValue) { t.threadLocals = new ThreadLocalMap(this, firstValue); }static ThreadLocalMap createInheritedMap(ThreadLocalMap parentMap) {return new ThreadLocalMap(parentMap); }T childValue(T parentValue) {throw new UnsupportedOperationException(); }static class ThreadLocalMap {static class Entry extends WeakReference<threadlocal> {/** The value associated with this ThreadLocal. */Object value; Entry(ThreadLocal k, Object v) {super(k); value = v; } }private static final int INITIAL_CAPACITY = 16;private Entry[] table;private int size = 0;private int threshold; // Default to 0private void setThreshold(int len) { threshold = len * 2 / 3; }private static int nextIndex(int i, int len) {return ((i + 1 = 0) ? i - 1 : len - 1); }ThreadLocalMap(ThreadLocal firstKey, Object firstValue) { table = new Entry[INITIAL_CAPACITY];int i = firstKey.threadLocalHashCode & (INITIAL_CAPACITY - 1); table[i] = new Entry(firstKey, firstValue); size = 1; setThreshold(INITIAL_CAPACITY); }private ThreadLocalMap(ThreadLocalMap parentMap) { Entry[] parentTable = parentMap.table;int len = parentTable.length; setThreshold(len); table = new Entry[len];for (int j = 0; j = threshold) rehash(); }private void remove(ThreadLocal key) { Entry[] tab = table;int len = tab.length;int i = key.threadLocalHashCode & (len-1);for (Entry e = tab[i]; e != null; e = tab[i = nextIndex(i, len)]) {if (e.get() == key) { e.clear(); expungeStaleEntry(i);return; } } }private void replaceStaleEntry(ThreadLocal key, Object value, int staleSlot) { Entry[] tab = table;int len = tab.length; Entry e;int slotToExpunge = staleSlot;for (int i = prevIndex(staleSlot, len); (e = tab[i]) != null; i = prevIndex(i, len))if (e.get() == null) slotToExpunge = i;for (int i = nextIndex(staleSlot, len); (e = tab[i]) != null; i = nextIndex(i, len)) { ThreadLocal k = e.get();if (k == key) { e.value = value; tab[i] = tab[staleSlot]; tab[staleSlot] = e;if (slotToExpunge == staleSlot) slotToExpunge = i; cleanSomeSlots(expungeStaleEntry(slotToExpunge), len);return; }if (k == null && slotToExpunge == staleSlot) slotToExpunge = i; }tab[staleSlot].value = null; tab[staleSlot] = new Entry(key, value);if (slotToExpunge != staleSlot) cleanSomeSlots(expungeStaleEntry(slotToExpunge), len); }private int expungeStaleEntry(int staleSlot) { Entry[] tab = table;int len = tab.length;tab[staleSlot].value = null; tab[staleSlot] = null; size--; Entry e;int i;for (i = nextIndex(staleSlot, len); (e = tab[i]) != null; i = nextIndex(i, len)) { ThreadLocal k = e.get();if (k == null) { e.value = null; tab[i] = null; size--; } else {int h = k.threadLocalHashCode & (len - 1);if (h != i) { tab[i] = null;while (tab[h] != null) h = nextIndex(h, len); tab[h] = e; } } }return i; }private boolean cleanSomeSlots(int i, int n) {boolean removed = false; Entry[] tab = table;int len = tab.length;do { i = nextIndex(i, len); Entry e = tab[i];if (e != null && e.get() == null) { n = len; removed = true; i = expungeStaleEntry(i); } } while ( (n >>>= 1) != 0);return removed; }private void rehash() { expungeStaleEntries();// Use lower threshold for doubling to avoid hysteresisif (size >= threshold - threshold / 4) resize(); }private void resize() { Entry[] oldTab = table;int oldLen = oldTab.length;int newLen = oldLen * 2; Entry[] newTab = new Entry[newLen];int count = 0;for (int j = 0; j </threadlocal></t>
這樣子,都是用同一個連接,但是每個連接都是新的,是同一個連接的副本。
那麼實現機制是如何的呢?
1、每個Thread物件內部都維護了一個ThreadLocalMap這樣一個ThreadLocal的Map,可以存放若干個ThreadLocal
/* ThreadLocal values pertaining to this thread. This map is maintained * by the ThreadLocal class. */ThreadLocal.ThreadLocalMap threadLocals = null;
2、當我們在呼叫get( )方法的時候,先取得當前線程,然後取得到當前線程的ThreadLocalMap對象,如果非空,那麼取出ThreadLocal的value,否則進行初始化,初始化就是將initialValue的值set到ThreadLocal。
public T get() { Thread t = Thread.currentThread(); ThreadLocalMap map = getMap(t);if (map != null) { ThreadLocalMap.Entry e = map.getEntry(this);if (e != null)return (T)e.value; }return setInitialValue(); }
3、當我們呼叫set()方法的時候,很常規,就是將值設為ThreadLocal。
4、總結:當我們呼叫get方法的時候,其實每個目前執行緒都有一個ThreadLocal。每次取得或設定都是對該ThreadLocal進行的操作,是與其他執行緒分開的。
5、應用程式場景:當很多執行緒需要多次使用同一個對象,並且需要該物件具有相同初始化值的時候最適合使用ThreadLocal。
6、其實說再多也不如看一下原始碼來得清晰。如果要看源碼,其中涉及到一個WeakReference和一個Map,這兩個地方需要了解下,這兩個東西分別是a.Java的弱引用,也就是GC的時候會銷毀該引用所包裹(引用)的對象,這個threadLocal作為key可能被銷毀,但是只要我們定義成他的類不卸載,tl這個強引用就始終引用著這個ThreadLocal的,永遠不會被gc掉。 b.和HashMap差不多。
以上是什麼是線程局部變數?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

本文討論了使用Maven和Gradle進行Java項目管理,構建自動化和依賴性解決方案,以比較其方法和優化策略。
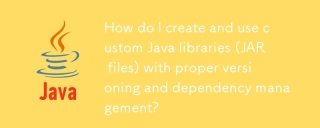
本文使用Maven和Gradle之類的工具討論了具有適當的版本控制和依賴關係管理的自定義Java庫(JAR文件)的創建和使用。
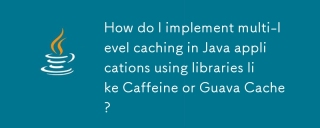
本文討論了使用咖啡因和Guava緩存在Java中實施多層緩存以提高應用程序性能。它涵蓋設置,集成和績效優勢,以及配置和驅逐政策管理最佳PRA

本文討論了使用JPA進行對象相關映射,並具有高級功能,例如緩存和懶惰加載。它涵蓋了設置,實體映射和優化性能的最佳實踐,同時突出潛在的陷阱。[159個字符]
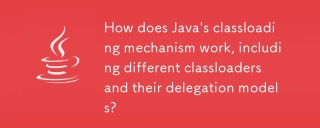
Java的類上載涉及使用帶有引導,擴展程序和應用程序類負載器的分層系統加載,鏈接和初始化類。父代授權模型確保首先加載核心類別,從而影響自定義類LOA


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。
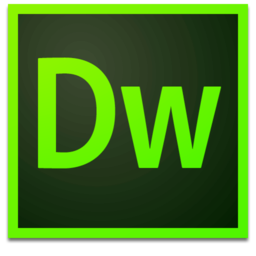
Dreamweaver Mac版
視覺化網頁開發工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。