用過反射的人都知道,呼叫一個方法很簡單,但如何給一個【不知簽名】的方法準備傳入參數呢?
下面就來回答這個問題,請接著看GetActionCallParameters的實現過程:
private static object[] GetActionCallParameters(HttpContext context, ActionDescription action) { if( action.Parameters == null || action.Parameters.Length == 0 ) return null; object[] parameters = new object[action.Parameters.Length]; for( int i = 0; i < action.Parameters.Length; i++ ) { ParameterInfo p = action.Parameters[i]; if( p.IsOut ) continue; if( p.ParameterType == typeof(NameValueCollection) ) { if( string.Compare(p.Name, "Form", StringComparison.OrdinalIgnoreCase) == 0 ) parameters[i] = context.Request.Form; else if( string.Compare(p.Name, "QueryString", StringComparison.OrdinalIgnoreCase) == 0 ) parameters[i] = context.Request.QueryString; else if( string.Compare(p.Name, "Headers", StringComparison.OrdinalIgnoreCase) == 0 ) parameters[i] = context.Request.Headers; else if( string.Compare(p.Name, "ServerVariables", StringComparison.OrdinalIgnoreCase) == 0 ) parameters[i] = context.Request.ServerVariables; } else{ Type paramterType = p.ParameterType.GetRealType(); // 如果参数是简单类型,则直接从HttpRequest中读取并赋值 if( paramterType.IsSimpleType() ) { object val = ModelHelper.GetValueByKeyAndTypeFrommRequest( context.Request, p.Name, paramterType, null); if( val != null ) parameters[i] = val; } else { // 自定义的类型。首先创建实例,然后给所有成员赋值。 // 注意:这里不支持嵌套类型的自定义类型。 object item = Activator.CreateInstance(paramterType); ModelHelper.FillModel(context.Request, item, p.Name); parameters[i] = item; } } } return parameters; }
要理解這段程式碼還要從前面的【查找Action的過程】說起,在那個階段,可以獲取一個Action的描述,具體在框架內部表示為ActionDescription類型:
internal sealed class ActionDescription : BaseDescription{ public ControllerDescription PageController; //为PageAction保留 public MethodInfo MethodInfo { get; private set; } public ActionAttribute Attr { get; private set; } public ParameterInfo[] Parameters { get; private set; } public bool HasReturn { get; private set; } public ActionDescription(MethodInfo m, ActionAttribute atrr) : base(m) { this.MethodInfo = m; this.Attr = atrr; this.Parameters = m.GetParameters(); this.HasReturn = m.ReturnType != ReflectionHelper.VoidType; } }
在建構子的第三行程式碼中,我就可以得到這個方法的所有參數情況。
然後,我在就可以在GetActionCallParameters方法中,循環每個參數的定義,為它們賦值。
這段程式碼也解釋了前面所說的只支援4種NameValueCollection集合的原因。
注意了,我在取得每個參數的類型時,是使用了下面的語句:
Type paramterType = p.ParameterType.GetRealType();
實際上,ParameterType就已經反映了參數的類型,為什麼不直接使用它呢?
答:因為【可空泛型】的原因。這個類型我們需要特殊的處理。
例如:如果某個參數是這樣宣告的: int? id
那麼,即使在QueryString中包含id這樣一個參數,我也不能直接轉成int? 使用這種類型,必須得到它的【實際類型】。
GetRealType()是個擴充方法,它就專門完成這個功能:
/// <summary> /// 得到一个实际的类型(排除Nullable类型的影响)。比如:int? 最后将得到int/// </summary> /// <param name="type"></param> /// <returns></returns>public static Type GetRealType(this Type type) { if( type.IsGenericType ) return Nullable.GetUnderlyingType(type) ?? type; else return type; }
如果某個參數的類型是一個自訂的類型,框架會先建立實例(呼叫無參的建構子),然後給它的Property, Field賦值。
注意了:自訂的型別,一定要提供一個無參的建構子。
為自訂類型的實例填入資料成員的程式碼如下:
internal static class ModelHelper{ public static readonly bool IsDebugMode; static ModelHelper() { CompilationSection configSection = ConfigurationManager.GetSection("system.web/compilation") as CompilationSection; if( configSection != null ) IsDebugMode = configSection.Debug; } /// <summary> /// 根据HttpRequest填充一个数据实体。 /// 这里不支持嵌套类型的数据实体,且要求各数据成员都是简单的数据类型。 /// </summary> /// <param name="request"></param> /// <param name="model"></param> public static void FillModel(HttpRequest request, object model, string paramName) { ModelDescripton descripton = ReflectionHelper.GetModelDescripton(model.GetType()); object val = null; foreach( DataMember field in descripton.Fields ) { // 这里的实现方式不支持嵌套类型的数据实体。 // 如果有这方面的需求,可以将这里改成递归的嵌套调用。 val = GetValueByKeyAndTypeFrommRequest( request, field.Name, field.Type.GetRealType(), paramName); if( val != null ) field.SetValue(model, val); } } /// <summary> /// 读取一个HTTP参数值。这里只读取QueryString以及Form /// </summary> /// <param name="request"></param> /// <param name="key"></param> /// <returns></returns> public static string GetHttpValue(HttpRequest request, string key) { string val = request.QueryString[key]; if( val == null ) val = request.Form[key]; return val; } public static object GetValueByKeyAndTypeFrommRequest( HttpRequest request, string key, Type type, string paramName) { // 不支持复杂类型 if( type.IsSimpleType() == false ) return null; string val = GetHttpValue(request, key); if( val == null ) { // 再试一次。有可能是多个自定义类型,Form表单元素采用变量名做为前缀。 if( string.IsNullOrEmpty(paramName) == false ) { val = GetHttpValue(request, paramName + "." + key); } if( val == null ) return null; } return SafeChangeType(val.Trim(), type); } public static object SafeChangeType(string value, Type conversionType) { if( conversionType == typeof(string) ) return value; if( value == null || value.Length == 0 ) // 空字符串根本不能做任何转换,所以直接返回null return null; try { // 为了简单,直接调用 .net framework中的方法。 // 如果转换失败,则会抛出异常。 return Convert.ChangeType(value, conversionType); } catch { if( IsDebugMode ) throw; // Debug 模式下抛异常 else return null; // Release模式下忽略异常(防止恶意用户错误输入) } } }
在載入資料給自訂的資料類型實例之前,需要先知道這個實例物件有哪些屬性以及字段,這個過程的程式碼如下:
/// <summary> /// 返回一个实体类型的描述信息(全部属性及字段)。/// </summary> /// <param name="type"></param> /// <returns></returns>public static ModelDescripton GetModelDescripton(Type type) { if( type == null ) throw new ArgumentNullException("type"); string key = type.FullName; ModelDescripton mm = (ModelDescripton)s_modelTable[key]; if( mm == null ) { List<DataMember> list = new List<DataMember>(); (from p in type.GetProperties(BindingFlags.Instance | BindingFlags.Public) select new PropertyMember(p)).ToList().ForEach(x=>list.Add(x)); (from f in type.GetFields(BindingFlags.Instance | BindingFlags.Public) select new FieldMember(f)).ToList().ForEach(x => list.Add(x)); mm = new ModelDescripton { Fields = list.ToArray() }; s_modelTable[key] = mm; } return mm; }
在拿到一個類型的所有屬性以及字段的描述資訊後,就可以通過循環的方式,根據這些數據成員的名字去QueryString,Form讀取所需的資料了。
【相關推薦】
1. 特別推薦#:「php程式設計師工具箱」V0.1版本下載
2. ASP免費影片教學
以上是.NET MyMVC框架如何為方法賦值的教學課程的詳細內容。更多資訊請關注PHP中文網其他相關文章!
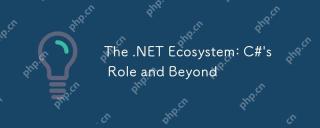
C#在.NET生態系統中扮演核心角色,是開發者的首選語言。 1)C#提供高效、易用的編程方式,結合C、C 和Java的優點。 2)通過.NET運行時(CLR)執行,確保跨平台高效運行。 3)C#支持從基本到高級的用法,如LINQ和異步編程。 4)優化和最佳實踐包括使用StringBuilder和異步編程,提高性能和可維護性。
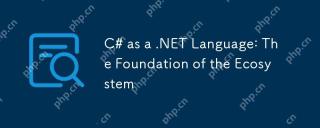
C#是微軟在2000年發布的編程語言,旨在結合C 的強大功能和Java的簡潔性。 1.C#是一種類型安全、面向對象的編程語言,支持封裝、繼承和多態。 2.C#的編譯過程將代碼轉化為中間語言(IL),然後在.NET運行時環境(CLR)中即時編譯成機器碼執行。 3.C#的基本用法包括變量聲明、控制流和函數定義,而高級用法涵蓋異步編程、LINQ和委託等。 4.常見錯誤包括類型不匹配和空引用異常,可通過調試器、異常處理和日誌記錄來調試。 5.性能優化建議包括使用LINQ、異步編程和提高代碼可讀性。
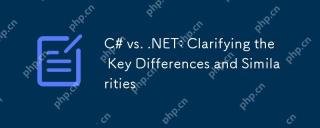
C#是一種編程語言,而.NET是一個軟件框架。 1.C#由微軟開發,適用於多平台開發。 2..NET提供類庫和運行時環境,支持多語言。兩者協同工作,構建現代應用。
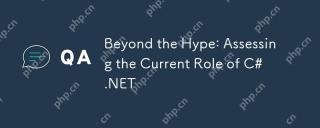
C#.NET是一個強大的開發平台,結合了C#語言和.NET框架的優勢。 1)它廣泛應用於企業應用、Web開發、遊戲開發和移動應用開發。 2)C#代碼編譯成中間語言後由.NET運行時環境執行,支持垃圾回收、類型安全和LINQ查詢。 3)使用示例包括基本控制台輸出和高級LINQ查詢。 4)常見錯誤如空引用和類型轉換錯誤可以通過調試器和日誌記錄解決。 5)性能優化建議包括異步編程和優化LINQ查詢。 6)儘管面臨競爭,C#.NET通過不斷創新保持其重要地位。
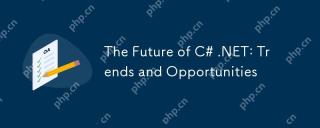
C#.NET的未來趨勢主要集中在雲計算、微服務、AI和機器學習集成以及跨平台開發三個方面。 1)雲計算和微服務:C#.NET通過Azure平台優化雲環境表現,支持構建高效微服務架構。 2)AI和機器學習集成:借助ML.NET庫,C#開發者可在應用中嵌入機器學習模型,推動智能化應用發展。 3)跨平台開發:通過.NETCore和.NET5 ,C#應用可在Windows、Linux和macOS上運行,擴展部署範圍。
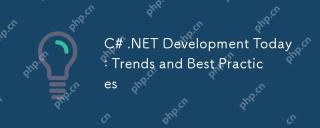
C#.NET開發的最新動態和最佳實踐包括:1.異步編程提高應用響應性,使用async和await關鍵字簡化非阻塞代碼;2.LINQ提供強大查詢功能,通過延遲執行和表達式樹高效操作數據;3.性能優化建議包括使用異步編程、優化LINQ查詢、合理管理內存、提升代碼可讀性和維護性、以及編寫單元測試。
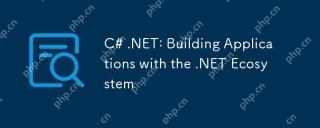
如何利用.NET構建應用?使用.NET構建應用可以通過以下步驟實現:1)了解.NET基礎知識,包括C#語言和跨平台開發支持;2)學習核心概念,如.NET生態系統的組件和工作原理;3)掌握基本和高級用法,從簡單控制台應用到復雜的WebAPI和數據庫操作;4)熟悉常見錯誤與調試技巧,如配置和數據庫連接問題;5)應用性能優化與最佳實踐,如異步編程和緩存。
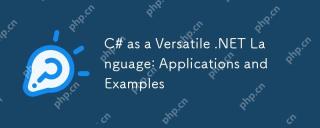
C#在企業級應用、遊戲開發、移動應用和Web開發中均有廣泛應用。 1)在企業級應用中,C#常用於ASP.NETCore開發WebAPI。 2)在遊戲開發中,C#與Unity引擎結合,實現角色控制等功能。 3)C#支持多態性和異步編程,提高代碼靈活性和應用性能。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!
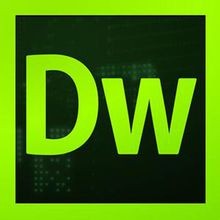
Dreamweaver CS6
視覺化網頁開發工具
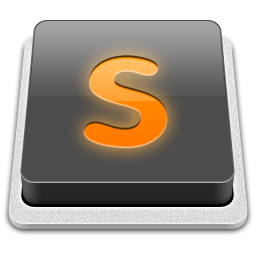
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

SublimeText3 Linux新版
SublimeText3 Linux最新版
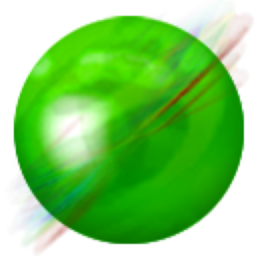
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境