<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Login.aspx.cs" Inherits="Login" %> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" > <head id="Head1" runat="server"> <title>无标题页</title> <script type="text/javascript"> function DoFresh() { document.getElementById("Image1").src = "VerifyCode.aspx"; } </script> </head> <body> <form id="form1" runat="server"> <div> <table> <tr> <td> 验证码:<asp:TextBox ID="txtValidateCode" runat="server"></asp:TextBox> </td> <td> <asp:Image ID="Image1" runat="server" /> <a href="javascript:DoFresh();">看不清?</a> </td> </tr> <tr> <td align="center" colspan="2"> <br /> <asp:Literal ID="litErrorMsg" runat="server"></asp:Literal> <asp:Button ID="btnSubmit" runat="server" Text="确定" onclick="btnSubmit_Click" /> </td> </tr> </table> </div> </form> </body> </html> using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class Login : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { if (!Page.IsPostBack) { Image1.ImageUrl = "VerifyCode.aspx"; } } protected void btnSubmit_Click(object sender, EventArgs e) { if (Session["ValidateCode"] != null) { string outputValidateCode = Session["ValidateCode"] as string; string inputValidateCode = txtValidateCode.Text.Trim(); if (string.Compare(outputValidateCode, inputValidateCode, true) != 0) { //Response.Write("<script>javascript:alert('输入的验证码错误!');</script>"); litErrorMsg.Text = "输入的验证码错误!"; } else { //Response.Write("<script>javascript:alert('输入的验证码正确!');</script>"); litErrorMsg.Text = "输入的验证码正确!"; } } } #region 调用下面的方法实现客户端保存Cookie验证模式 private void ValidateMethod() { if (Request.Cookies["CheckCode"] == null) { litErrorMsg.Text = "您的浏览器设置已被禁用 Cookies,您必须设置浏览器允许使用 Cookies 选项后才能使用本系统。"; litErrorMsg.Visible = true; return; } if (String.Compare(Request.Cookies["CheckCode"].Value, TextBox1.Text.ToString().Trim(), true) != 0) { litErrorMsg.Text = "<font color=red>对不起,验证码错误!</font>"; litErrorMsg.Visible = true; return; } else { litErrorMsg.Text = "<font color=green>恭喜,验证码输入正确!</font>"; litErrorMsg.Visible = true; } } #endregion }
//VerifyCode.aspx為預設產生的程式碼
using System; using System.Data; using System.Configuration; using System.Collections; using System.Web; using System.Web.Security; using System.Web.UI; using System.Web.UI.WebControls.WebParts; using System.Web.UI.HtmlControls; using System.Drawing; using System.Drawing.Imaging; using System.Drawing.Drawing2D; using System.IO; public partial class VerifyCode : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { //GenerateValidateCode(); GenerateVerifyImage(4);//GenerateVerifyImage(int length) } #region 【无刷新仿google波形扭曲彩色】验证码样式0___GenerateValidateCode() private void GenerateValidateCode() { this.Length = this.length; this.FontSize = this.fontSize; this.Chaos = this.chaos; this.BackgroundColor = this.backgroundColor; this.ChaosColor = this.chaosColor; this.CodeSerial = this.codeSerial; this.Colors = this.colors; this.Fonts = this.fonts; this.Padding = this.padding; string VNum = this.CreateVerifyCode(); //取随机码 Session["ValidateCode"] = VNum.ToUpper();//取得验证码,以便后来验证 this.CreateImageOnPage(VNum, this.Context); // 输出图片 //Cookie验证模式, 使用Cookies取验证码的值 //Response.Cookies.Add(new HttpCookie("CheckCode", code.ToUpper())); } #endregion #region 验证码长度(默认4个验证码的长度) int length = 4; public int Length { get { return length; } set { length = value; } } #endregion #region 验证码字体大小(为了显示扭曲效果,默认40像素,可以自行修改) int fontSize = 22; public int FontSize { get { return fontSize; } set { fontSize = value; } } #endregion #region 边框补(默认1像素) int padding = 2; public int Padding { get { return padding; } set { padding = value; } } #endregion #region 是否输出燥点(默认不输出) bool chaos = true; public bool Chaos { get { return chaos; } set { chaos = value; } } #endregion #region 输出燥点的颜色(默认灰色) Color chaosColor = Color.LightGray; public Color ChaosColor { get { return chaosColor; } set { chaosColor = value; } } #endregion #region 自定义背景色(默认白色) Color backgroundColor = Color.White; public Color BackgroundColor { get { return backgroundColor; } set { backgroundColor = value; } } #endregion #region 自定义随机颜色数组 Color[] colors = { Color.Black, Color.Red, Color.DarkBlue, Color.Green, Color.Orange, Color.Brown, Color.DarkCyan, Color.Purple }; public Color[] Colors { get { return colors; } set { colors = value; } } #endregion #region 自定义字体数组 string[] fonts = { "Arial", "Georgia" }; public string[] Fonts { get { return fonts; } set { fonts = value; } } #endregion #region 自定义随机码字符串序列(使用逗号分隔) string codeSerial = "0,1,2,3,4,5,6,7,8,9,a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z,A,B,C,D,E,F,G,H,I,J,K,L,M,N,O,P,Q,R,S,T,U,V,W,X,Y,Z"; public string CodeSerial { get { return codeSerial; } set { codeSerial = value; } } #endregion #region 产生波形滤镜效果 private const double PI = 3.1415926535897932384626433832795; private const double PI2 = 6.283185307179586476925286766559; /// <summary> /// 正弦曲线Wave扭曲图片 /// </summary> /// <param name="srcBmp">图片路径</param> /// <param name="bXDir">如果扭曲则选择为True</param> /// <param name="nMultValue">波形的幅度倍数,越大扭曲的程度越高,一般为3</param> /// <param name="dPhase">波形的起始相位,取值区间[0-2*PI)</param> /// <returns></returns> public Bitmap TwistImage(Bitmap srcBmp, bool bXDir, double dMultValue, double dPhase) { Bitmap destBmp = new Bitmap(srcBmp.Width, srcBmp.Height); // 将位图背景填充为白色 System.Drawing.Graphics graph = Graphics.FromImage(destBmp); graph.FillRectangle(new SolidBrush(Color.White), 0, 0, destBmp.Width, destBmp.Height); graph.Dispose(); double dBaseAxisLen = bXDir ? (double)destBmp.Height : (double)destBmp.Width; for (int i = 0; i < destBmp.Width; i++) { for (int j = 0; j < destBmp.Height; j++) { double dx = 0; dx = bXDir ? (PI2 * (double)j) / dBaseAxisLen : (PI2 * (double)i) / dBaseAxisLen; dx += dPhase; double dy = Math.Sin(dx); // 取得当前点的颜色 int nOldX = 0, nOldY = 0; nOldX = bXDir ? i + (int)(dy * dMultValue) : i; nOldY = bXDir ? j : j + (int)(dy * dMultValue); System.Drawing.Color color = srcBmp.GetPixel(i, j); if (nOldX >= 0 && nOldX < destBmp.Width && nOldY >= 0 && nOldY < destBmp.Height) { destBmp.SetPixel(nOldX, nOldY, color); } } } return destBmp; } #endregion #region 生成校验码图片 public Bitmap CreateImageCode(string code) { int fSize = FontSize; int fWidth = fSize + Padding; int imageWidth = (int)(code.Length * fWidth) + 4 + Padding * 2; int imageHeight = fSize * 2 + Padding; System.Drawing.Bitmap image = new System.Drawing.Bitmap(imageWidth, imageHeight); Graphics g = Graphics.FromImage(image); g.Clear(BackgroundColor); Random rand = new Random(); //给背景添加随机生成的燥点 if (this.Chaos) { Pen pen = new Pen(ChaosColor, 0); int c = Length * 10; for (int i = 0; i < c; i++) { int x = rand.Next(image.Width); int y = rand.Next(image.Height); g.DrawRectangle(pen, x, y, 1, 1); } } int left = 0, top = 0, top1 = 1, top2 = 1; int n1 = (imageHeight - FontSize - Padding * 2); int n2 = n1 / 4; top1 = n2; top2 = n2 * 2; Font f; Brush b; int cindex, findex; //随机字体和颜色的验证码字符 for (int i = 0; i < code.Length; i++) { cindex = rand.Next(Colors.Length - 1); findex = rand.Next(Fonts.Length - 1); f = new System.Drawing.Font(Fonts[findex], fSize, System.Drawing.FontStyle.Bold); b = new System.Drawing.SolidBrush(Colors[cindex]); if (i % 2 == 1) { top = top2; } else { top = top1; } left = i * fWidth; g.DrawString(code.Substring(i, 1), f, b, left, top); } //画一个边框 边框颜色为Color.Gainsboro g.DrawRectangle(new Pen(Color.Gainsboro, 0), 0, 0, image.Width - 1, image.Height - 1); g.Dispose(); //产生波形(Add By 51aspx.com) image = TwistImage(image, true, 4, 4); return image; } #endregion #region 将创建好的图片输出到页面 public void CreateImageOnPage(string code, HttpContext context) { Response.BufferOutput = true; //特别注意 Response.Cache.SetExpires(DateTime.Now.AddMilliseconds(-1));//特别注意 Response.Cache.SetCacheability(HttpCacheability.NoCache);//特别注意 Response.AppendHeader("Pragma", "No-Cache"); //特别注意 MemoryStream ms = new MemoryStream(); Bitmap image = this.CreateImageCode(code); image.Save(ms, ImageFormat.Jpeg); Response.ClearContent(); Response.ContentType = "image/JPEG"; Response.BinaryWrite(ms.ToArray()); Response.End(); ms.Close(); ms = null; image.Dispose(); image = null; } #endregion #region 生成随机字符码 public string CreateVerifyCode(int codeLen) { if (codeLen == 0) { codeLen = Length; } string[] arr = CodeSerial.Split(','); string code = ""; int randValue = -1; Random rand = new Random(unchecked((int)DateTime.Now.Ticks)); for (int i = 0; i < codeLen; i++) { randValue = rand.Next(0, arr.Length - 1); code += arr[randValue]; } return code; } public string CreateVerifyCode() { return CreateVerifyCode(0); } #endregion #region 另一种验证码样式 GenerateVerifyImage(int length) /// <summary> /// 将创建好的图片输出到页面 /// </summary> public void GenerateVerifyImage(int nLen) { string validateCode = "";//生成的验证码 int nBmpWidth = GetImagewidth(nLen); int nBmpHeight = GetImageHeight(); System.Drawing.Bitmap bmp = new System.Drawing.Bitmap(nBmpWidth, nBmpHeight); //对图像进行弯曲 TwistImage(bmp, true, 12, 2); // 1. 生成随机背景颜色 int nRed, nGreen, nBlue; // 背景的三元色 System.Random rd = new Random((int)System.DateTime.Now.Ticks); nRed = rd.Next(255) % 128 + 128; nGreen = rd.Next(255) % 128 + 128; nBlue = rd.Next(255) % 128 + 128; // 2. 填充位图背景 System.Drawing.Graphics graph = System.Drawing.Graphics.FromImage(bmp); graph.FillRectangle(new SolidBrush(System.Drawing.Color.FromArgb(nRed, nGreen, nBlue)) , 0 , 0 , nBmpWidth , nBmpHeight); // 3. 绘制干扰线条,采用比背景略深一些的颜色 int nLines = 3; System.Drawing.Pen pen = new System.Drawing.Pen(System.Drawing.Color.FromArgb(nRed - 17, nGreen - 17, nBlue - 17), 2); for (int a = 0; a < nLines; a++) { int x1 = rd.Next() % nBmpWidth; int y1 = rd.Next() % nBmpHeight; int x2 = rd.Next() % nBmpWidth; int y2 = rd.Next() % nBmpHeight; graph.DrawLine(pen, x1, y1, x2, y2); } // 采用的字符集,可以随即拓展,并可以控制字符出现的几率 string strCode = "0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"; // 4. 循环取得字符,并绘制 for (int i = 0; i < nLen; i++) { int x = (i * 13 + rd.Next(3)); int y = rd.Next(4) + 1; // 确定字体 System.Drawing.Font font = new System.Drawing.Font("Courier New",//文字字体类型 12 + rd.Next() % 4,//文字字体大小 System.Drawing.FontStyle.Bold);//文字字体样式 char c = strCode[rd.Next(strCode.Length)]; // 随机获取字符 validateCode += c.ToString(); // 绘制字符 graph.DrawString(c.ToString(), font, new SolidBrush(System.Drawing.Color.FromArgb(nRed - 60 + y * 3, nGreen - 60 + y * 3, nBlue - 40 + y * 3)), x, y); } Session["ValidateCode"] = validateCode; //对图像进行弯曲 TwistImage(bmp, true, 4, 4); Response.BufferOutput = true; //特别注意 Response.Cache.SetExpires(DateTime.Now.AddMilliseconds(-1));//特别注意 Response.Cache.SetCacheability(HttpCacheability.NoCache);//特别注意 Response.AppendHeader("Pragma", "No-Cache"); //特别注意 // 5. 输出字节流 MemoryStream bstream = new MemoryStream(); bmp.Save(bstream, ImageFormat.Jpeg); Response.ClearContent(); Response.ContentType = "image/JPEG"; Response.BinaryWrite(bstream.ToArray()); Response.End(); bstream.Close(); bstream = null; bmp.Dispose(); bmp = null; graph.Dispose(); } ///<summary> ///得到验证码图片的宽度 ///</summary> ///<paramname="validateNumLength">验证码的长度</param> ///<returns></returns> public static int GetImagewidth(int validateNumLength) { return (int)(13 * validateNumLength + 5); } ///<summary> ///得到验证码的高度 ///</summary> ///<returns></returns> public static int GetImageHeight() { return 25; } #endregion }
更多ASP.NET中的無刷新驗證碼的開發相關文章請關注PHP中文網!
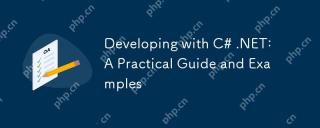
C#和.NET提供了強大的功能和高效的開發環境。 1)C#是一種現代、面向對象的編程語言,結合了C 的強大和Java的簡潔性。 2).NET框架是一個用於構建和運行應用程序的平台,支持多種編程語言。 3)C#中的類和對像是面向對象編程的核心,類定義數據和行為,對像是類的實例。 4).NET的垃圾回收機制自動管理內存,簡化開發者的工作。 5)C#和.NET提供了強大的文件操作功能,支持同步和異步編程。 6)常見錯誤可以通過調試器、日誌記錄和異常處理來解決。 7)性能優化和最佳實踐包括使用StringBuild
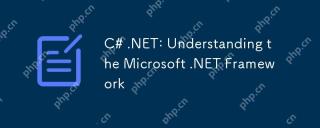
.NETFramework是一個跨語言、跨平台的開發平台,提供一致的編程模型和強大的運行時環境。 1)它由CLR和FCL組成,CLR管理內存和線程,FCL提供預構建功能。 2)使用示例包括讀取文件和LINQ查詢。 3)常見錯誤涉及未處理異常和內存洩漏,需使用調試工具解決。 4)性能優化可通過異步編程和緩存實現,保持代碼可讀性和可維護性是關鍵。
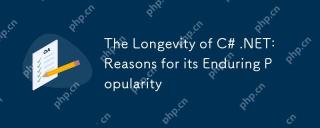
C#.NET保持持久吸引力的原因包括其出色的性能、豐富的生態系統、強大的社區支持和跨平台開發能力。 1)性能表現優異,適用於企業級應用和遊戲開發;2).NET框架提供了廣泛的類庫和工具,支持多種開發領域;3)擁有活躍的開發者社區和豐富的學習資源;4).NETCore實現了跨平台開發,擴展了應用場景。
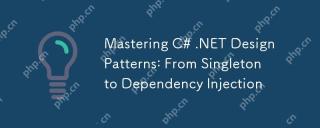
C#.NET中的設計模式包括Singleton模式和依賴注入。 1.Singleton模式確保類只有一個實例,適用於需要全局訪問點的場景,但需注意線程安全和濫用問題。 2.依賴注入通過注入依賴提高代碼靈活性和可測試性,常用於構造函數注入,但需避免過度使用導致複雜度增加。
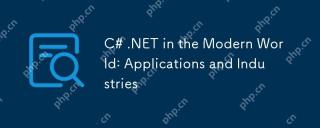
C#.NET在現代世界中廣泛應用於遊戲開發、金融服務、物聯網和雲計算等領域。 1)在遊戲開發中,通過Unity引擎使用C#進行編程。 2)金融服務領域,C#.NET用於開發高性能的交易系統和數據分析工具。 3)物聯網和雲計算方面,C#.NET通過Azure服務提供支持,開發設備控制邏輯和數據處理。
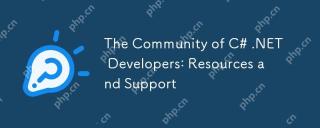
C#.NET開發者社區提供了豐富的資源和支持,包括:1.微軟的官方文檔,2.社區論壇如StackOverflow和Reddit,3.GitHub上的開源項目,這些資源幫助開發者從基礎學習到高級應用,提升編程技能。
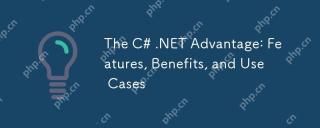
C#.NET的優勢包括:1)語言特性,如異步編程簡化了開發;2)性能與可靠性,通過JIT編譯和垃圾回收機制提升效率;3)跨平台支持,.NETCore擴展了應用場景;4)實際應用廣泛,從Web到桌面和遊戲開發都有出色表現。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具
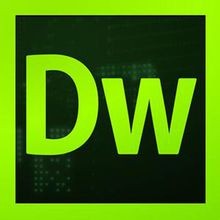
Dreamweaver CS6
視覺化網頁開發工具
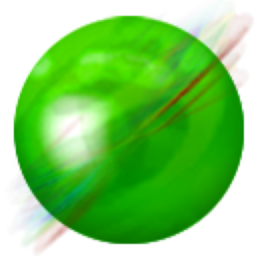
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境
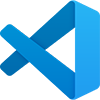
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器
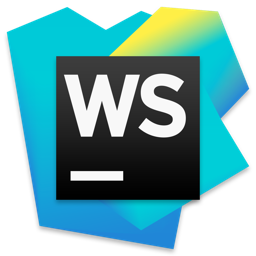
WebStorm Mac版
好用的JavaScript開發工具