如何使用Uni-App的生命週期鉤?
要使用Uni-App的生命週期鉤,您需要了解它們已集成到應用程序的生命週期中,從而使您可以在應用程序生命週期的不同階段執行特定功能。您可以使用它們:
-
應用程序生命週期鉤:這些是在
onLaunch
,onShow
,onHide
和onError
方法中的App.vue
文件中定義的。例如,您可能需要在應用程序啟動時初始化數據:<code class="javascript">export default { onLaunch: function() { console.log('App Launch') }, onShow: function() { console.log('App Show') }, onHide: function() { console.log('App Hide') } }</code>
-
頁面生命週期掛鉤:這些是在頁面的
.vue
文件中定義的,並包含諸如onLoad
,onShow
,Onshow,onReady
,Ready,onHide
,onUnload
等的掛鉤。例如,您可能需要在頁面加載時加載數據:<code class="javascript">export default { data() { return { title: 'Hello' } }, onLoad: function(options) { console.log('Page Load') // You can use options to get the parameters passed when the page is opened. } }</code>
-
組件生命週期鉤:這些類似於頁面生命週期鉤,但在組件中使用,包括
beforeCreate
,created
,beforeMount
,mounted
,beforeDestroy
和destroyed
。它們是在組件的腳本標籤中定義的:<code class="javascript">export default { data() { return { count: 0 } }, mounted() { console.log('Component Mounted') } }</code>
通過適當地使用這些生命週期鉤,您可以在整個生命週期內管理應用程序的狀態和行為。
Uni-App中有哪些不同的生命週期掛鉤?
Uni-App提供了各種生命週期鉤子來管理應用程序,頁面或組件的不同階段。這是可用的不同類型的生命週期鉤子:
應用生命週期鉤:
-
onLaunch
:初始化應用程序時觸發。 -
onShow
:當應用顯示在前景中時觸發。 -
onHide
:當應用進入背景時觸發。 -
onError
:當應用程序中發生錯誤時觸發。
頁面生命週期掛鉤:
-
onLoad
:加載頁面時觸發。傳遞參數options
,其中包含打開頁面時傳遞的數據。 -
onShow
:顯示頁面時觸發。 -
onReady
:當頁面完全渲染時觸發。 -
onHide
:隱藏頁面時觸發。 -
onUnload
:卸載頁面時觸發。 -
onPullDownRefresh
:用戶拉下以刷新頁面時觸發。 -
onReachBottom
:頁面滾動到底部時觸發。 -
onShareAppMessage
:用戶單擊共享按鈕時觸發。 -
onPageScroll
:滾動頁面時觸發。 -
onResize
:當頁面大小更改時觸發。 -
onTabItemTap
:單擊選項卡時觸發。
組件生命週期鉤:
-
beforeCreate
:在創建組件之前調用。 -
created
:創建組件後調用。 -
beforeMount
:在安裝組件之前調用。 -
mounted
:將組件安裝後打電話。 -
beforeUpdate
:當數據更新之前的數據更改時調用。 -
updated
:在更新DOM後打電話。 -
beforeDestroy
:在銷毀組件之前打電話。 -
destroyed
:在銷毀組件後打電話。
如何使用Uni-App LifeCycle鉤子優化應用程序的性能?
使用Uni-App生命週期掛鉤優化應用程序的性能涉及在不同的生命週期階段仔細管理資源和有效的數據處理。以下是一些策略:
-
有效地初始化數據:使用
onLaunch
掛鉤來初始化整個應用程序生命週期中需要可用的數據。這樣可以防止在多個頁面上獲取冗餘數據。<code class="javascript">onLaunch: function() { // Fetch initial data here }</code>
-
懶惰加載:使用頁面上的
onLoad
和onShow
Hook僅在必要時加載數據,從而減少初始加載時間和內存使用量。<code class="javascript">onLoad: function() { // Load page-specific data here }</code>
-
清理資源:使用
onHide
和onUnload
掛鉤清理隱藏或卸載頁面時不再需要的資源。這可以幫助減少內存使用量。<code class="javascript">onUnload: function() { // Clear timers, event listeners, etc. }</code>
-
避免冗餘計算:如果需要,請使用
onShow
刷新數據,但請嘗試通過在可能的情況下緩存結果來避免冗餘計算。<code class="javascript">onShow: function() { if (!this.cachedData) { // Fetch data only if not already cached this.fetchData(); } }</code>
-
優化性能:使用
onPageScroll
和onReachBottom
處理與捲軸相關的性能優化,例如圖像的懶惰加載或其他數據。<code class="javascript">onReachBottom: function() { // Load more data when the user scrolls to the bottom }</code>
通過策略性地使用這些生命週期鉤,您可以更有效地管理應用程序的性能,減少加載時間並改善用戶體驗。
如何處理Uni-App生命週期鉤中的錯誤和例外?
在Uni-App生命週期掛鉤中處理錯誤和例外,對於保持穩定且用戶友好的應用程序至關重要。這是您可以管理它們的方法:
-
全局錯誤處理:使用
App.vue
中的onError
Hook在整個應用程序中捕獲任何未熟悉的錯誤。這使您可以記錄錯誤並為用戶提供後備。<code class="javascript">export default { onError: function(error) { console.error('App Error:', error); // Show a user-friendly message or redirect to an error page } }</code>
-
特定於頁面的錯誤處理:對於特定於頁面的錯誤,您可以使用
onLoad
,onShow
或其他頁面生命週期掛鉤來捕獲和處理錯誤。<code class="javascript">export default { onLoad: function(options) { try { // Attempt to load data this.loadData(); } catch (error) { console.error('Page Load Error:', error); // Handle the error, eg, show an error message to the user } } }</code>
-
組件特定的錯誤處理:使用組件生命週期掛鉤(如
mounted
或updated
來處理組件中的錯誤。<code class="javascript">export default { mounted: function() { try { // Attempt to initialize the component this.initComponent(); } catch (error) { console.error('Component Initialization Error:', error); // Handle the error, eg, show an error state in the component } } }</code>
-
集中式錯誤處理:您可能需要通過創建可以從任何生命週期掛鉤調用的實用程序函數來集中錯誤處理,以統一處理錯誤。
<code class="javascript">// utils/errorHandler.js export function handleError(error) { console.error('Error:', error); // Implement global error handling logic here } // In any lifecycle hook import { handleError } from './utils/errorHandler'; export default { onLoad: function(options) { try { // Attempt to load data this.loadData(); } catch (error) { handleError(error); } } }</code>
通過實施這些策略,您可以有效地管理Uni-App生命週期鉤中的錯誤和異常,從而提高應用程序的可靠性和魯棒性。
以上是如何使用Uni-App的生命週期鉤?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3 Linux新版
SublimeText3 Linux最新版

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。
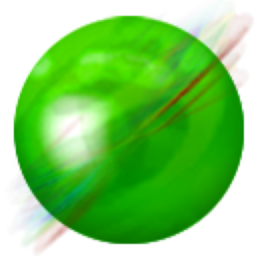
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

記事本++7.3.1
好用且免費的程式碼編輯器