本文詳細介紹了YII框架中創建和使用自定義驗證器。它涵蓋了擴展驗證者類,效率的最佳實踐(簡潔,利用內置驗證器,輸入消毒),整合第三方庫,
在yii中創建和使用自定義驗證器
在YII中創建和使用自定義驗證器可以使您可以執行內置的特定驗證規則。這對於實施業務邏輯或處理獨特的驗證要求至關重要。 The process generally involves extending the yii\validators\Validator
class and overriding the validateAttribute()
method.
假設您需要一個驗證器來檢查字符串是否僅包含字母數字字符和下劃線。這是您創建和使用它的方式:
<code class="php">// Custom validator class namespace app\validators; use yii\validators\Validator; class AlphanumericUnderscoreValidator extends Validator { public function validateAttribute($model, $attribute) { $value = $model->$attribute; if (!preg_match('/^[a-zA-Z0-9_] $/', $value)) { $this->addError($model, $attribute, 'Only alphanumeric characters and underscores are allowed.'); } } }</code>
現在,在您的模型中:
<code class="php">use app\validators\AlphanumericUnderscoreValidator; class MyModel extends \yii\db\ActiveRecord { public function rules() { return [ [['username'], 'required'], [['username'], AlphanumericUnderscoreValidator::class], ]; } }</code>
This code defines a AlphanumericUnderscoreValidator
that uses a regular expression to check the input. The rules()
method in your model then uses this custom validator for the username
attribute.如果驗證失敗,將顯示指定的錯誤消息。
在YII中編寫有效自定義驗證器的最佳實踐
編寫有效的自定義驗證器對於性能和可維護性至關重要。這是一些關鍵最佳實踐:
- Keep it concise: Avoid unnecessary complexity within your validator.專注於一個定義明確的驗證規則。如果您需要多個檢查,請考慮將它們分解為單獨的驗證器。
- Use built-in validators where possible: Don't reinvent the wheel.只要有優化的性能,就可以使用YII的內置驗證器。
- Input sanitization: Before performing validation, sanitize the input to prevent vulnerabilities like SQL injection or cross-site scripting (XSS). This should be handled before the validation itself.
- Error messages: Provide clear and informative error messages to the user.避免神秘的技術術語。 Use placeholders like
{attribute}
to dynamically insert the attribute name. - Testing: Thoroughly test your custom validators with various inputs, including edge cases and invalid data, to ensure they function correctly and handle errors gracefully.強烈建議進行單元測試。
- Code readability and maintainability: Use descriptive variable names and comments to improve code understanding and ease future modifications.遵循一致的編碼樣式準則。
- Performance optimization: For computationally intensive validations, consider optimizing your code.分析您的代碼可以幫助識別瓶頸。
將第三方庫與YII中的自定義驗證器集成
對於專業驗證需求,通常需要將第三方庫與自定義驗證器集成在一起。 This usually involves incorporating the library's functionality within your custom validator's validateAttribute()
method.
例如,如果您正在使用庫來驗證電子郵件地址的嚴格性比YII的內置驗證器更嚴格,則可以這樣將其合併:
<code class="php">use yii\validators\Validator; use SomeThirdPartyEmailValidator; // Replace with your library's class class StrictEmailValidator extends Validator { public function validateAttribute($model, $attribute) { $value = $model->$attribute; $validator = new SomeThirdPartyEmailValidator(); // Instantiate the third-party validator if (!$validator->isValid($value)) { $this->addError($model, $attribute, 'Invalid email address.'); } } }</code>
切記在項目的依賴項中包括必要的庫(例如,使用作曲家)。第三方庫中的正確處理和文檔對於成功集成至關重要。
在YII中創建自定義驗證器時處理不同的數據類型
在自定義驗證器中處理不同的數據類型對於靈活性和正確性至關重要。您的驗證器應優雅處理各種輸入類型,並為類型不匹配提供適當的錯誤消息。
You can achieve this using type checking within your validateAttribute()
method.例如:
<code class="php">use yii\validators\Validator; class MyCustomValidator extends Validator { public function validateAttribute($model, $attribute) { $value = $model->$attribute; if (is_string($value)) { // String-specific validation logic if (strlen($value) addError($model, $attribute, 'String must be at least 5 characters long.'); } } elseif (is_integer($value)) { // Integer-specific validation logic if ($value addError($model, $attribute, 'Integer must be non-negative.'); } } else { $this->addError($model, $attribute, 'Invalid data type.'); } } }</code>
這個示例演示了處理字符串和整數。 Adding more elseif
blocks allows you to support additional data types.請記住處理輸入為null或意外類型以防止意外錯誤的情況。明確的錯誤消息對於向用戶告知數據類型問題至關重要。
以上是如何在YII中創建和使用自定義驗證器?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
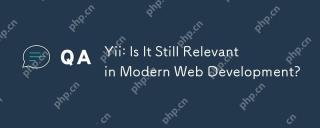
Yiiremainsrelevantinmodernwebdevelopmentforprojectsneedingspeedandflexibility.1)Itoffershighperformance,idealforapplicationswherespeediscritical.2)Itsflexibilityallowsfortailoredapplicationstructures.However,ithasasmallercommunityandsteeperlearningcu
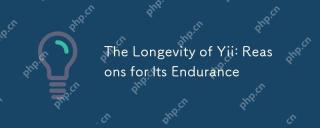
Yii框架在眾多PHP框架中依然保持強大生命力是因為其高效、簡潔和可擴展的設計理念。 1)Yii通過“約定優於配置”提高開發效率;2)基於組件的架構和強大的ORM系統Gii增強了靈活性和開發速度;3)性能優化和不斷的更新迭代確保其持續競爭力。
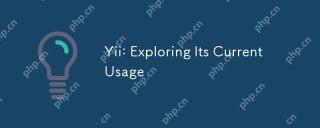
Yii在現代Web開發中仍適用於需要高性能和靈活性的項目。 1)Yii基於PHP的高性能框架,遵循MVC架構。 2)它的優勢在於高效、簡潔和組件化設計。 3)性能優化主要通過緩存和ORM實現。 4)隨著新框架的出現,Yii的使用情況有所變化。
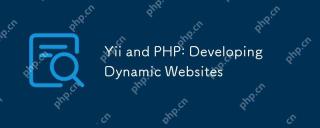
Yii和PHP可以打造動態網站。 1)Yii是一個高性能的PHP框架,簡化Web應用開發。 2)Yii提供MVC架構、ORM、緩存等功能,適合大型應用開發。 3)使用Yii的基本和高級功能可以快速構建網站。 4)注意配置、命名空間和數據庫連接問題,使用日誌和調試工具進行調試。 5)通過緩存和優化查詢提升性能,遵循最佳實踐提高代碼質量。
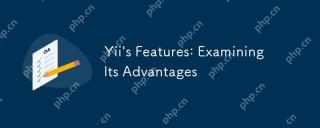
Yii框架在PHP框架中脫穎而出,其優勢包括:1.MVC架構和組件化設計,提升代碼組織和復用性;2.Gii代碼生成器和ActiveRecord,提高開發效率;3.多種緩存機制,優化性能;4.靈活的RBAC系統,簡化權限管理。
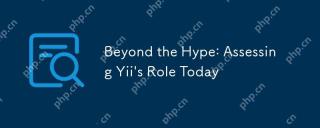
Yii仍然是開發者的一個強有力的選擇。 1)Yii是一個高性能的PHP框架,基於MVC架構,提供ActiveRecord、Gii和緩存系統等工具。 2)它的優點包括高效性和靈活性,但學習曲線較陡,社區支持相對有限。 3)適合需要高性能和靈活性的項目,但需考慮團隊技術棧和學習成本。
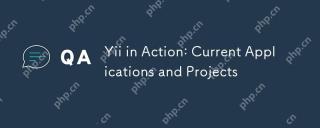
Yii框架適用於企業級應用、中小型項目和個人項目。 1)在企業級應用中,Yii的高性能和可擴展性使其在電商平台等大型項目中表現出色。 2)中小型項目中,Yii的Gii工具幫助快速搭建原型和MVP。 3)個人項目和開源項目中,Yii的輕量級特性使其適合小型網站和博客。
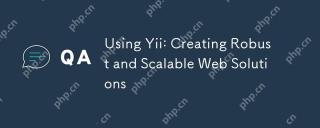
Yii框架適合構建高效、安全和可擴展的Web應用。 1)Yii基於MVC架構,提供組件化設計和安全特性。 2)它支持基本CRUD操作和高級RESTfulAPI開發。 3)提供日誌記錄和調試工具欄等調試技巧。 4)建議使用緩存和延遲加載進行性能優化。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
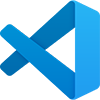
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中
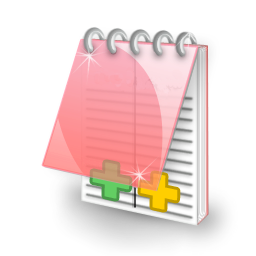
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

禪工作室 13.0.1
強大的PHP整合開發環境