本文詳細介紹了在YII中實施數據庫交易的,並強調了使用DBTransaction的原子性。它涵蓋了最佳實踐,例如短交易,適當的隔離水平,細緻的例外處理(包括回滾)和避開
在YII中實施數據庫交易
Yii provides a straightforward way to implement database transactions using its Transaction
object.該對像管理事務生命週期,確保原子能 - 交易中的所有操作要么完全成功或完全失敗,因此數據庫處於一致的狀態。 The most common approach involves using a try-catch
block within a DbTransaction
object.您可以做到這一點:
<code class="php">use yii\db\Transaction; $transaction = Yii::$app->db->beginTransaction(); try { // Your database operations here. For example: $user = new User(); $user->username = 'testuser'; $user->email = 'test@example.com'; $user->save(); $profile = new Profile(); $profile->user_id = $user->id; $profile->bio = 'This is a test profile.'; $profile->save(); $transaction->commit(); } catch (\Exception $e) { $transaction->rollBack(); // Handle the exception appropriately, eg, log the error, display a user-friendly message. Yii::error($e, __METHOD__); throw $e; // Re-throw the exception for higher-level handling if needed. }</code>
該代碼首先開始交易。 If all save()
operations succeed, $transaction->commit()
is called, permanently saving the changes. If any operation throws an exception, $transaction->rollBack()
is called, reverting all changes made within the transaction, maintaining data integrity.錯誤處理至關重要; the catch
block ensures that even if errors occur, the database remains consistent.
Best Practices for Handling Database Transactions in Yii
在使用YII中的數據庫交易時,幾種最佳實踐可以提高數據完整性和效率:
- Keep transactions short and focused: Long-running transactions hold database locks for extended periods, potentially impacting concurrency.旨在進行單個交易中的原子操作。
- Use appropriate isolation levels: Choosing the right isolation level (discussed later) balances data consistency and concurrency.默認級別通常足夠,但是特定的應用需求可能需要調整。
- Handle exceptions meticulously: Always wrap transaction code in a
try-catch
block.徹底調試和監視的日誌異常。考慮針對特定方案的自定義異常處理,以向用戶提供信息性錯誤消息。 - Avoid nested transactions: While Yii supports nested transactions, they can lead to complexity and potential deadlocks.努力為邏輯單位的單一交易進行單一的定義交易。
- Test thoroughly: Thorough testing is essential to verify that transactions behave as expected under various conditions, including error scenarios.
回滾YII中的數據庫事務
As demonstrated in the first section, rolling back a transaction is handled automatically by the catch
block of a try-catch
statement. If an exception is thrown during the transaction, $transaction->rollBack()
is automatically called, undoing any changes made within the transaction.至關重要的是要確保您的異常處理機制始終包括此回滾,以確保數據一致性。 No explicit rollback is necessary beyond calling $transaction->rollBack()
within the catch
block.
使用YII中的不同數據庫事務級別
YII支持不同的數據庫交易隔離水平,該水平控制並發交易之間的隔離程度。 These levels are set using the isolationLevel
property of the DbTransaction
object.共同級別包括:
- READ UNCOMMITTED: Allows reading uncommitted data from other transactions.這可能會導致骯髒的讀取(讀取已修改但尚未承諾的數據)。
- READ COMMITTED: Prevents dirty reads but allows non-repeatable reads (reading different data for the same query multiple times within a transaction) and phantom reads (seeing new rows inserted by another transaction).
- REPEATABLE READ: Prevents dirty reads and non-repeatable reads, but may allow phantom reads.
- SERIALIZABLE: The strictest level, preventing all concurrency issues (dirty reads, non-repeatable reads, and phantom reads).這是最限制的,可能會嚴重影響性能。
隔離級別的選擇取決於您的應用程序要求。 If data consistency is paramount and concurrency is less critical, SERIALIZABLE
might be appropriate. For most applications, READ COMMITTED
offers a good balance between consistency and performance.您可以在開始交易時指定隔離級別:
<code class="php">$transaction = Yii::$app->db->beginTransaction(Transaction::SERIALIZABLE); // Or another level // ... your transaction code ...</code>
切記在選擇隔離水平時仔細考慮數據一致性和性能之間的權衡。默認級別通常為許多應用程序提供足夠的隔離。
以上是如何在YII中實現數據庫交易?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
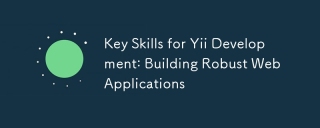
tobuildRobustWebapplicationswithyii,MasterTheSeskills:1)MvCarchitectureForstructuringApplications,2)ActivereCordForefifficdataBaseOperations,3)widgetsystemporreusableReusableSueuiComponents,4)驗證和驗證和驗證,5)cachingforpermificatization cachingforpermifications
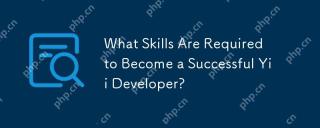
TobecomeasuccessfulYiideveloper,youneed:1)PHPmastery,2)understandingofMVCarchitecture,3)Yiiframeworkproficiency,4)databasemanagementskills,5)front-endknowledge,6)APIdevelopmentexpertise,7)testinganddebuggingcapabilities,8)versioncontrolproficiency,9)
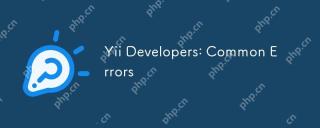
theSostCommonErrorsinyiiframeWorkare“ unknownproperty”,“無效configuration”,“ classNotfound”和“ valianationerationerrors” .1。 “ Unknownerproperty” errorSoccurWhenAccessingNon-existentSistentProperties; SusePropertiesexi; Susepropertiesexi;
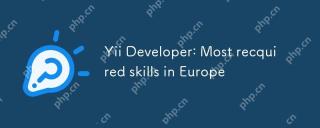
歐洲Yii開發者需具備的關鍵技能包括:1.Yii框架精通,2.PHP熟練度,3.數據庫管理,4.前端技能,5.RESTfulAPI開發,6.版本控制系統,7.測試與調試,8.安全知識,9.敏捷方法論,10.軟技能,11.本地化與國際化,12.持續學習,這些技能使開發者在歐洲市場中脫穎而出。
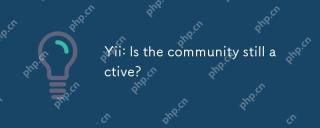
Yes,theYiicommunityisstillactiveandvibrant.1)TheofficialYiiforumremainsaresourcefordiscussionsandsupport.2)TheGitHubrepositoryshowsregularcommitsandpullrequests,indicatingongoingdevelopment.3)StackOverflowcontinuestohostYii-relatedquestionsandhigh-qu
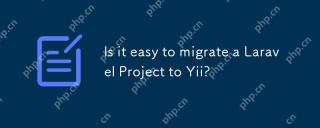
crigatingalaravel projectToyiiishallingButachieffable withiefleflant.1)mapoutlaravel組件likeoutes,控制器和模型。 2)Translatelaravel's sartisancancancommandeloequorentoottooyii的giiandeteverecordeba
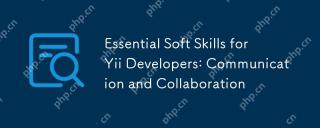
軟技能對Yii開發者至關重要,因為它們促進團隊溝通和協作。 1)有效溝通確保項目進展順利,如通過清晰的API文檔和定期會議。 2)協作通過Yii的工具如Gii增強團隊互動,提高開發效率。
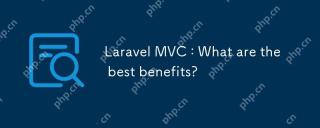
laravel'smvCarchitectureOfferSenhancedCodeorganization,改善確定性,andarobustseParefofConcerns.1)ItkeepscodeOdeOrganized,makenavigationNavigationAnvigationAndTeamWorkeer.2)itcompartmentalizestHeaplication,簡化了tompertalizestHeaplication,簡化了tlubloublyingttrublyingtimpertinging和maintenance.3)itse.3)itse


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
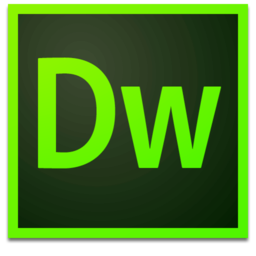
Dreamweaver Mac版
視覺化網頁開發工具
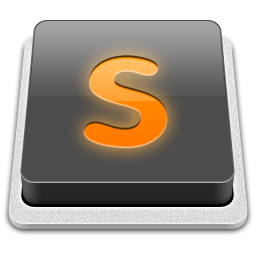
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
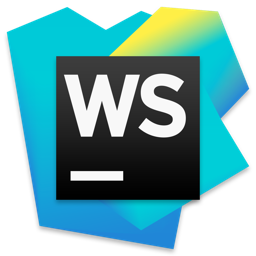
WebStorm Mac版
好用的JavaScript開發工具

Atom編輯器mac版下載
最受歡迎的的開源編輯器

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中