使用openai而無需函數
>在本節中,我們將使用GPT-3.5-Turbo模型生成響應,而無需呼叫,以查看我們是否獲得一致的輸出。
>在安裝OpenAI Python API之前,您必須獲得一個API鍵並將其設置在本地系統上。通過Python教程中的OpenAI API遵循GPT-3.5和GPT-4,以了解如何獲取API鍵並進行設置。該教程還包括在DataCamp的DataCamp的AI啟用數據筆記本中設置環境變量的示例。以獲取進一步的幫助,請查看Datalab上的OpenAI函數撥打工作簿中的代碼。
>使用以下方式將OpenAi Python API升級到V1
之後,使用API鍵啟動OpenAI客戶端。
pip install --upgrade openai -q>
>
import os from openai import OpenAI client = OpenAI( api_key=os.environ['OPENAI_API_KEY'], )注:OpenAI不再向新用戶提供免費的積分,因此您必須購買它們才能使用API。
我們將編寫一個隨機的學生描述。您可以提出自己的文字,或者使用chatgpt為您生成一個。 >
>在下一部分中,我們將編寫一個提示,以從文本中提取學生信息並將輸出返回為JSON對象。我們將在學生描述中提取名稱,專業,學校,成績和俱樂部。
>student_1_description = "David Nguyen is a sophomore majoring in computer science at Stanford University. He is Asian American and has a 3.8 GPA. David is known for his programming skills and is an active member of the university's Robotics Club. He hopes to pursue a career in artificial intelligence after graduating."
>將提示添加到OpenAI API聊天完成模塊中以生成響應。
# A simple prompt to extract information from "student_description" in a JSON format. prompt1 = f''' Please extract the following information from the given text and return it as a JSON object: name major school grades club This is the body of text to extract the information from: {student_1_description} '''響應非常好。讓我們將其轉換為JSON,以更好地理解它。
>
# Generating response back from gpt-3.5-turbo openai_response = client.chat.completions.create( model = 'gpt-3.5-turbo', messages = [{'role': 'user', 'content': prompt_1}] ) openai_response.choices[0].message.content我們將使用“ JSON”庫將文本轉換為JSON對象。
最終結果非常完美。那麼,為什麼我們需要調用函數?
'{\n "name": "David Nguyen",\n "major": "computer science",\n "school": "Stanford University",\n "grades": "3.8 GPA",\n "club": "Robotics Club"\n}'>
>讓我們嘗試相同的提示,但使用其他學生描述。
import json # Loading the response as a JSON object json_response = json.loads(openai_response.choices[0].message.content) json_response
>我們將在提示中更改學生描述文本。
{'name': 'David Nguyen', 'major': 'computer science', 'school': 'Stanford University', 'grades': '3.8 GPA', 'club': 'Robotics Club'}
,並使用第二個提示來運行聊天完成功能。
student_2_description="Ravi Patel is a sophomore majoring in computer science at the University of Michigan. He is South Asian Indian American and has a 3.7 GPA. Ravi is an active member of the university's Chess Club and the South Asian Student Association. He hopes to pursue a career in software engineering after graduating."如您所見,這是不一致的。它沒有返回一個俱樂部,而是返回了拉維(Ravi)加入的俱樂部名單。這也與第一個學生不同。
>
prompt2 = f''' Please extract the following information from the given text and return it as a JSON object: name major school grades club This is the body of text to extract the information from: {student_2_description} '''openai函數調用示例
為了解決此問題,我們現在將使用最近引入的功能呼叫的功能。創建一個自定義功能以在字典列表中添加必要的信息是至關重要的,以便OpenAI API了解其功能。
- >名稱:寫您最近創建的python函數名稱。
- 描述:函數的功能。 >
- 參數:在“屬性”中,我們將寫入參數,類型和描述的名稱。它將幫助Openai API確定我們正在尋找的世界。
note:確保您遵循正確的模式。通過閱讀官方文檔來了解有關函數調用的更多信息。 >
接下來,我們將使用添加到“函數”參數中的自定義函數為兩個學生描述生成響應。之後,我們將將文本響應轉換為JSON對象並打印它。pip install --upgrade openai -q>
如我們所見,我們獲得了統一的輸出。我們甚至在數字而不是字符串中獲得成績。一致的輸出對於創建沒有錯誤的AI應用程序至關重要。
>import os from openai import OpenAI client = OpenAI( api_key=os.environ['OPENAI_API_KEY'], )
多個自定義功能
student_1_description = "David Nguyen is a sophomore majoring in computer science at Stanford University. He is Asian American and has a 3.8 GPA. David is known for his programming skills and is an active member of the university's Robotics Club. He hopes to pursue a career in artificial intelligence after graduating.">您可以在聊天完成功能中添加多個自定義功能。在本節中,我們將看到OpenAI API的神奇功能,以及它如何自動選擇正確的函數並返回正確的參數。
。 在字典的python列表中,我們將添加另一個稱為“ extract_school_info”的功能,該功能將幫助我們從文本中提取大學信息。 為了實現這一目標,您必須添加另一個具有名稱,描述和參數的函數的字典。
>我們將使用Chatgpt生成“ Stanford University”描述來測試我們的功能。
>創建學生和學校描述列表,並通過OpenAI聊天完成功能將其傳遞以生成響應。確保您提供了更新的自定義功能。
># A simple prompt to extract information from "student_description" in a JSON format. prompt1 = f''' Please extract the following information from the given text and return it as a JSON object: name major school grades club This is the body of text to extract the information from: {student_1_description} '''
> GPT-3.5-Turbo模型已自動為不同的描述類型選擇了正確的功能。我們為學生和學校提供了完美的JSON輸出。
># Generating response back from gpt-3.5-turbo openai_response = client.chat.completions.create( model = 'gpt-3.5-turbo', messages = [{'role': 'user', 'content': prompt_1}] ) openai_response.choices[0].message.content
我們甚至可以使用“ extract_school_info”函數生成休息的名稱。
'{\n "name": "David Nguyen",\n "major": "computer science",\n "school": "Stanford University",\n "grades": "3.8 GPA",\n "club": "Robotics Club"\n}'
import json # Loading the response as a JSON object json_response = json.loads(openai_response.choices[0].message.content) json_response
>函數調用的應用
在本節中,我們將構建一個穩定的文本摘要,該摘要將以某種方式匯總學校和學生信息。
首先,我們將創建兩個python函數,即extract_student_info和extract_school_info,從函數調用中獲取參數並返回匯總的字符串。
pip install --upgrade openai -q
- 創建Python列表,該列表由學生描述,隨機提示和學校描述組成。添加隨機提示以驗證自動函數調用機械師。
- 我們將使用“描述”列表中的每個文本生成響應。 >
- 如果使用了函數調用,我們將獲得函數的名稱,並基於它,將相關參數應用於函數。否則,返回正常響應。 >
- 打印所有三個樣本的輸出。
import os from openai import OpenAI client = OpenAI( api_key=os.environ['OPENAI_API_KEY'], )
- 示例#1 :GPT模型選擇了“ extract_student_info”,我們得到了有關學生的簡短摘要。 >
- >樣本#2 :GPT模型尚未選擇任何功能,並將提示視為常規問題,結果,我們得到了亞伯拉罕·林肯的傳記。
- >樣本#3 :GPT模型選擇了“ extract_school_info”,我們得到了有關斯坦福大學的簡短摘要。
student_1_description = "David Nguyen is a sophomore majoring in computer science at Stanford University. He is Asian American and has a 3.8 GPA. David is known for his programming skills and is an active member of the university's Robotics Club. He hopes to pursue a career in artificial intelligence after graduating."在本教程中,我們了解了Openai的功能調用。我們還學習瞭如何使用它來生成一致的輸出,創建多個功能並構建可靠的文本摘要。
>如果您想了解有關OpenAi API的更多信息,請考慮使用OpenAI API課程,並在Python備忘單中使用OpenAi API來創建您的第一個AI驅動項目。
>>
是否可以與外部API或數據庫一起使用OpenAI函數來使用?
>
>>如果模型的功能調用與任何定義的函數不匹配,會發生什麼?
>
如果模型的函數調用與已定義的函數或所提供的架構不匹配,則該函數調用未觸發,並且該模型將輸入視為基於標准文本的提示,返回基於文本的基於典型的基於文本的響應。這確保了處理各種輸入類型的靈活性。
賺取頂級AI認證證明您可以有效,負責任地使用AI。獲得認證,被錄用以上是OpenAI函數調用教程:生成結構化輸出的詳細內容。更多資訊請關注PHP中文網其他相關文章!
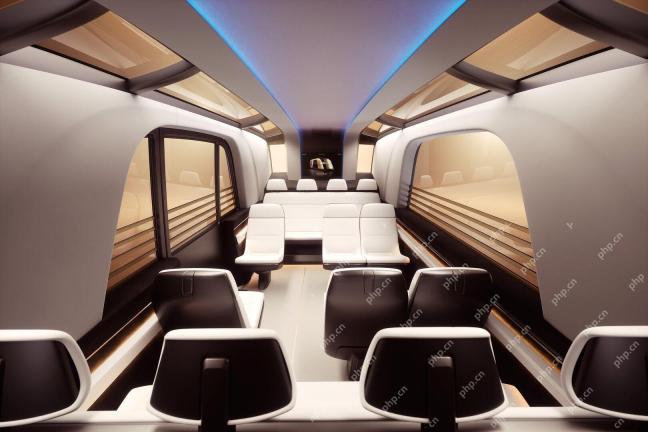
自2008年以來,我一直倡導這輛共享乘車麵包車,即後來被稱為“ Robotjitney”,後來是“ Vansit”,這是城市運輸的未來。 我預見這些車輛是21世紀的下一代過境解決方案Surpas
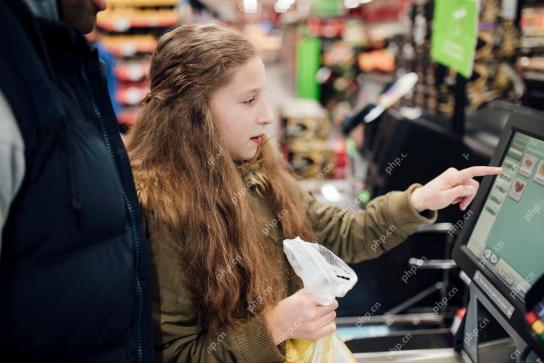
革新結帳體驗 Sam's Club的創新性“ Just Go”系統建立在其現有的AI驅動“掃描和GO”技術的基礎上,使會員可以在購物旅行期間通過Sam's Club應用程序進行掃描。
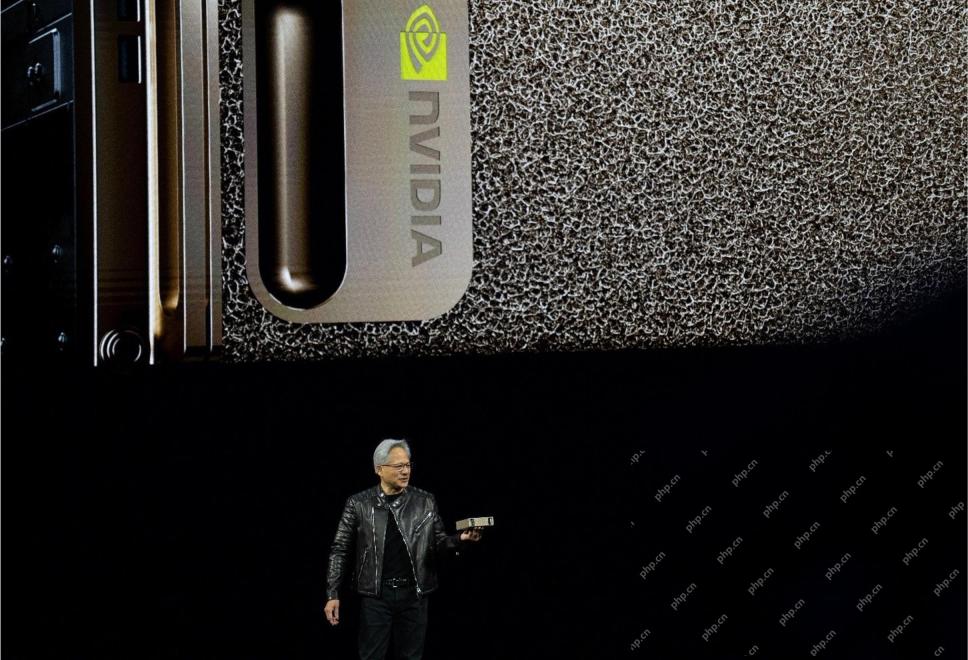
NVIDIA在GTC 2025上的增強可預測性和新產品陣容 NVIDIA是AI基礎架構的關鍵參與者,正在專注於提高其客戶的可預測性。 這涉及一致的產品交付,達到績效期望以及
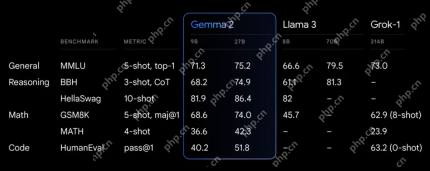
Google的Gemma 2:強大,高效的語言模型 Google的Gemma語言模型家族以效率和性能而慶祝,隨著Gemma 2的到來而擴展。此最新版本包括兩種模型:270億個參數VER
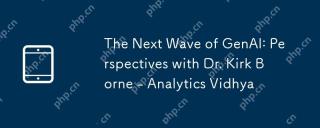
這一領先的數據劇集以數據科學家,天體物理學家和TEDX演講者Kirk Borne博士為特色。 Borne博士是大數據,AI和機器學習的著名專家,為當前狀態和未來的Traje提供了寶貴的見解
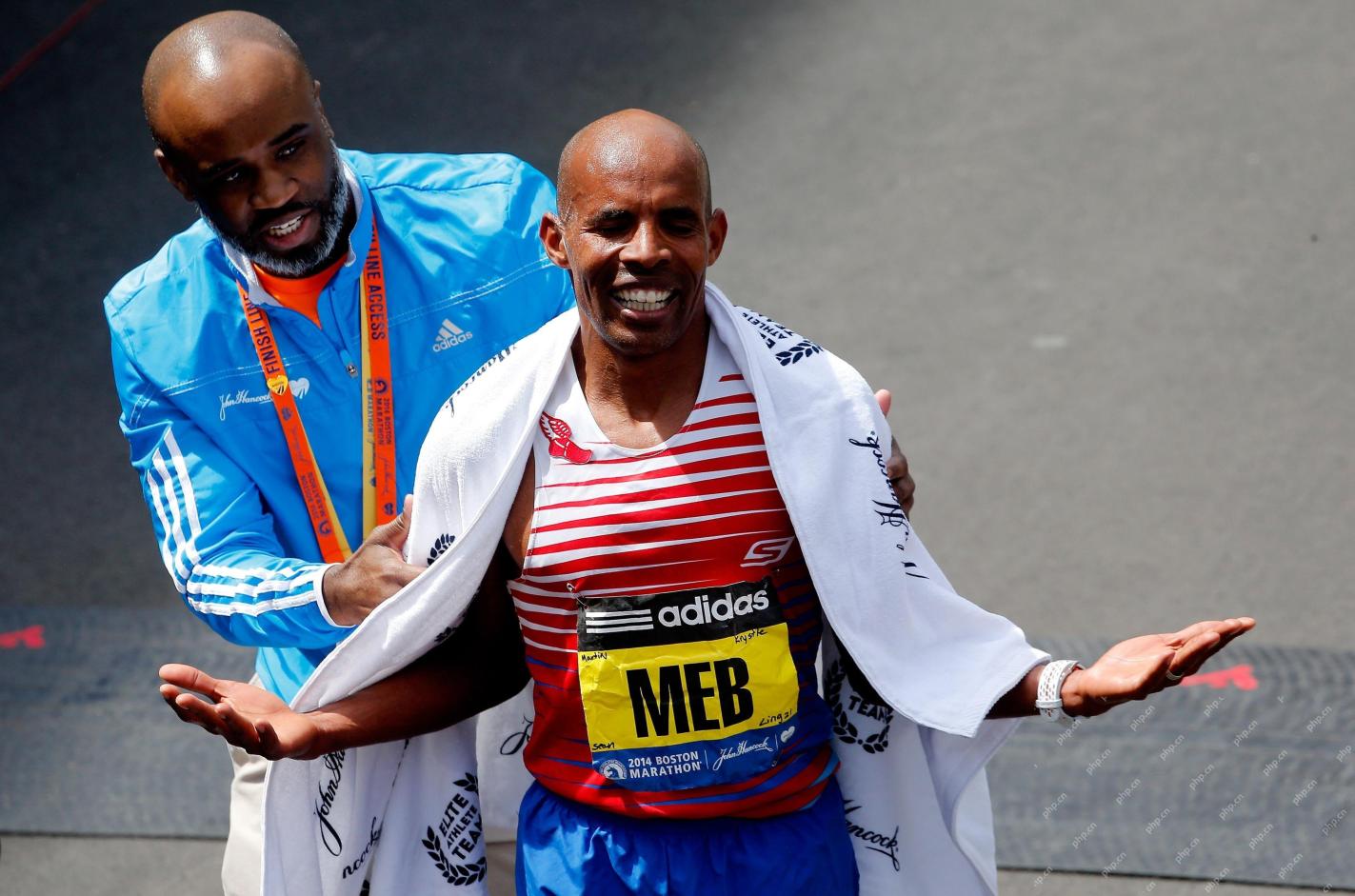
這次演講中出現了一些非常有見地的觀點——關於工程學的背景信息,這些信息向我們展示了為什麼人工智能如此擅長支持人們的體育鍛煉。 我將從每位貢獻者的觀點中概括出一個核心思想,以展示三個設計方面,這些方面是我們探索人工智能在體育運動中應用的重要組成部分。 邊緣設備和原始個人數據 關於人工智能的這個想法實際上包含兩個組成部分——一個與我們放置大型語言模型的位置有關,另一個與我們人類語言和我們的生命體徵在實時測量時“表達”的語言之間的差異有關。 Alexander Amini 對跑步和網球都很了解,但他還
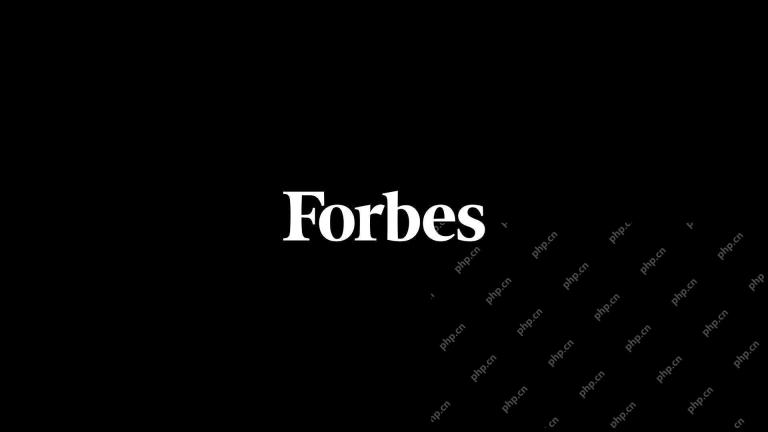
卡特彼勒(Caterpillar)的首席信息官兼高級副總裁傑米·恩格斯特(Jamie Engstrom)領導了一支由28個國家 /地區的2200多名IT專業人員組成的全球團隊。 在卡特彼勒(Caterpillar)工作了26年,其中包括她目前的四年半,Engst
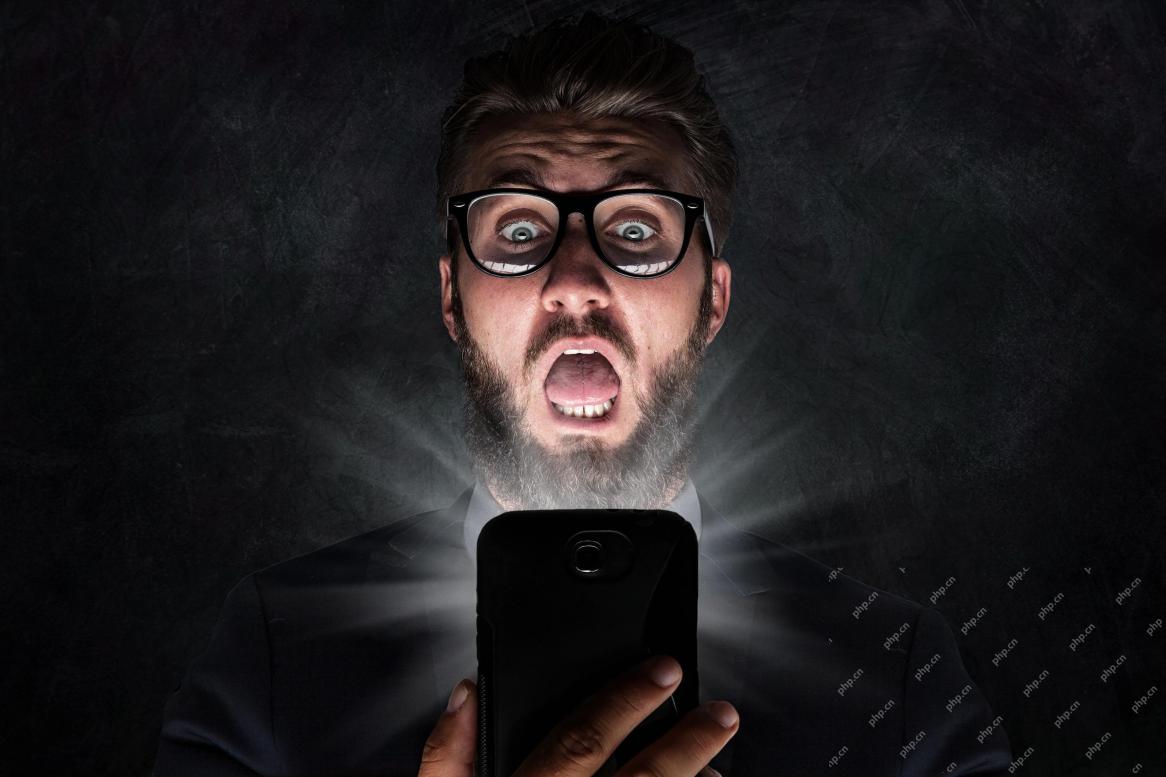
Google Photos的新Ultra HDR工具:快速指南 使用Google Photos的新型Ultra HDR工具增強照片,將標準圖像轉換為充滿活力的高動態範圍傑作。對於社交媒體而言,此工具可提高任何照片的影響,


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
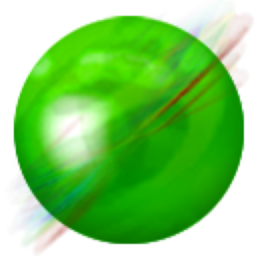
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),
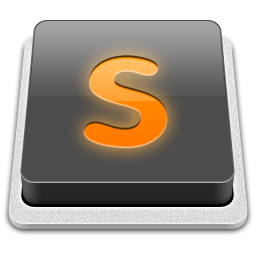
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具
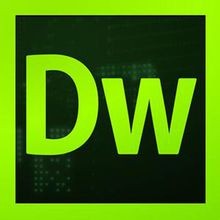
Dreamweaver CS6
視覺化網頁開發工具