LINQ - 全外部聯接
問題:
給定兩個包含ID以及名字或姓氏的人員列表,執行全外部聯接以生成包含名字和姓氏的個人列表。
解答:
全外部聯接與其他聯接的不同之處在於,它包含來自兩個輸入列表的項,即使在另一個列表中沒有匹配的元素。 為此,我們可以利用FullOuterJoin
擴展方法,其工作原理如下:
- 使用指定的鍵選擇器函數為兩個輸入列表創建查找表。
- 識別兩個查找表中的唯一鍵並將它們組合成一個集合。
- 遍歷鍵集,並為每個鍵從查找表中檢索相應的值。
- 利用投影函數生成每次迭代的所需結果。
更新後的代碼實現:
internal static class MyExtensions { internal static IEnumerable<TResult> FullOuterJoin<TA, TB, TKey, TResult>( this IEnumerable<TA> a, IEnumerable<TB> b, Func<TA, TKey> selectKeyA, Func<TB, TKey> selectKeyB, Func<TA, TB, TKey, TResult> projection, IEqualityComparer<TKey> cmp = null) { cmp = cmp ?? EqualityComparer<TKey>.Default; var alookup = a.ToLookup(selectKeyA, cmp); var blookup = b.ToLookup(selectKeyB, cmp); var keys = new HashSet<TKey>(alookup.Select(p => p.Key), cmp); keys.UnionWith(blookup.Select(p => p.Key)); var join = from key in keys let xa = alookup[key].DefaultIfEmpty(default(TA)) let xb = blookup[key].DefaultIfEmpty(default(TB)) select projection(xa.FirstOrDefault(), xb.FirstOrDefault(), key); return join; } }
示例用法:
var ax = new[] { new { id = 1, name = "John" }, new { id = 2, name = "Sue" } }; var bx = new[] { new { id = 1, surname = "Doe" }, new { id = 3, surname = "Smith" } }; ax.FullOuterJoin(bx, a => a.id, b => b.id, (a, b, id) => new { a?.name, b?.surname, id }) .ToList().ForEach(Console.WriteLine);
這段代碼將輸出以下結果:
<code>{ name = John, surname = Doe, id = 1 } { name = Sue, surname = , id = 2 } { name = , surname = Smith, id = 3 }</code>
This revised answer uses FirstOrDefault()
to handle the potential null values from DefaultIfEmpty()
more gracefully and directly outputs the name and surname, along with the ID, making the result clearer. The ?.
null-conditional operator prevents exceptions if a
or b
are null.
以上是如何在LINQ中執行完整的外部加入以根據ID合併兩個人列表?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
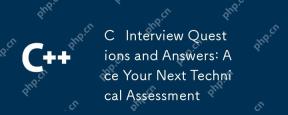
C 面試中,智能指針是關鍵工具,幫助管理內存並減少內存洩漏。 1)std::unique_ptr提供獨占所有權,確保資源自動釋放。 2)std::shared_ptr用於共享所有權,適用於多引用場景。 3)std::weak_ptr可避免循環引用,確保安全資源管理。
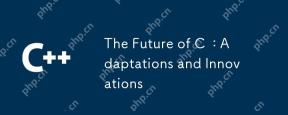
C 的未來將專注於並行計算、安全性、模塊化和AI/機器學習領域:1)並行計算將通過協程等特性得到增強;2)安全性將通過更嚴格的類型檢查和內存管理機制提升;3)模塊化將簡化代碼組織和編譯;4)AI和機器學習將促使C 適應新需求,如數值計算和GPU編程支持。
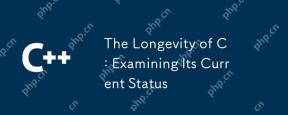
C 在現代編程中依然重要,因其高效、靈活和強大的特性。 1)C 支持面向對象編程,適用於系統編程、遊戲開發和嵌入式系統。 2)多態性是C 的亮點,允許通過基類指針或引用調用派生類方法,增強代碼的靈活性和可擴展性。
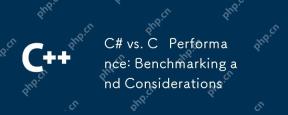
C#和C 在性能上的差異主要體現在執行速度和資源管理上:1)C 在數值計算和字符串操作上通常表現更好,因為它更接近硬件,沒有垃圾回收等額外開銷;2)C#在多線程編程上更為簡潔,但性能略遜於C ;3)選擇哪種語言應根據項目需求和團隊技術棧決定。
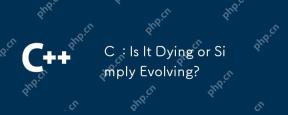
1)c relevantduetoItsAverity and效率和效果臨界。 2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
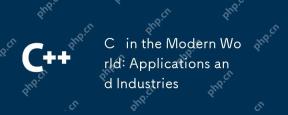
C 在現代世界中的應用廣泛且重要。 1)在遊戲開發中,C 因其高性能和多態性被廣泛使用,如UnrealEngine和Unity。 2)在金融交易系統中,C 的低延遲和高吞吐量使其成為首選,適用於高頻交易和實時數據分析。
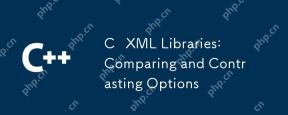
C 中有四種常用的XML庫:TinyXML-2、PugiXML、Xerces-C 和RapidXML。 1.TinyXML-2適合資源有限的環境,輕量但功能有限。 2.PugiXML快速且支持XPath查詢,適用於復雜XML結構。 3.Xerces-C 功能強大,支持DOM和SAX解析,適用於復雜處理。 4.RapidXML專注於性能,解析速度極快,但不支持XPath查詢。
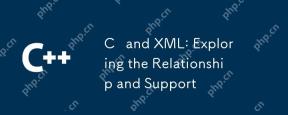
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
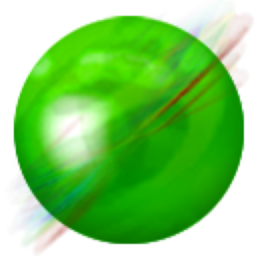
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

記事本++7.3.1
好用且免費的程式碼編輯器

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中
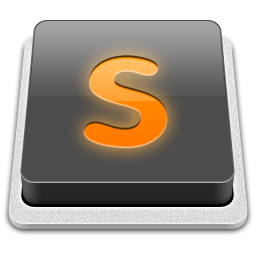
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!