網頁抓取是為檢索增強生成 (RAG) 應用程式收集內容的常用方法。然而,解析網頁內容可能具有挑戰性。
Mozilla 的開源 Readability.js 函式庫提供了一個方便的解決方案,用於僅提取網頁的基本部分。 讓我們探討一下它將其整合到 RAG 應用程式的資料攝取管道中。
從網頁擷取非結構化資料
網頁是非結構化資料的豐富來源,非常適合 RAG 應用程式。 然而,網頁通常包含不相關的訊息,例如頁首、側邊欄和頁尾。雖然這些額外內容對於瀏覽很有用,但會分散頁面的主要主題。
為了獲得最佳的 RAG 數據,必須刪除不相關的內容。 雖然像 Cheerio 這樣的工具可以根據網站已知的結構解析 HTML,但這種方法對於抓取不同的網站佈局效率很低。需要一種強大的方法來僅提取相關內容。
利用閱讀器視圖功能
大多數瀏覽器都包含一個閱讀器視圖,該視圖會刪除除文章標題和內容之外的所有內容。下圖說明了應用於 DataStax 部落格文章的標準瀏覽模式和閱讀器模式之間的差異:
Mozilla 提供 Readability.js(Firefox 閱讀器模式背後的函式庫)作為獨立的開源模組。這使我們能夠將 Readability.js 整合到資料管道中,以刪除不相關的內容並改善抓取結果。
使用 Node.js 和 Readability.js 抓取資料
讓我們舉例說明如何從先前關於在 Node.js 中建立向量嵌入的部落格文章中抓取文章內容。 以下 JavaScript 程式碼擷取頁面的 HTML:
const html = await fetch( "https://www.datastax.com/blog/how-to-create-vector-embeddings-in-node-js" ).then((res) => res.text()); console.log(html);
這包括所有 HTML,包括導覽、頁腳和網站上常見的其他元素。
或者,您可以使用 Cheerio 來選擇特定元素:
npm install cheerio
import * as cheerio from "cheerio"; const html = await fetch( "https://www.datastax.com/blog/how-to-create-vector-embeddings-in-node-js" ).then((res) => res.text()); const $ = cheerio.load(html); console.log($("h1").text(), "\n"); console.log($("section#blog-content > div:first-child").text());
這會產生標題和文章文字。 然而,這種方法依賴於了解 HTML 結構,這並不總是可行。
更好的方法是安裝 Readability.js 和 jsdom:
npm install @mozilla/readability jsdom
Readability.js 在瀏覽器環境中運行,需要 jsdom 在 Node.js 中模擬它。 我們可以將載入的 HTML 轉換為文件並使用 Readability.js 解析內容:
import { Readability } from "@mozilla/readability"; import { JSDOM } from "jsdom"; const url = "https://www.datastax.com/blog/how-to-create-vector-embeddings-in-node-js"; const html = await fetch(url).then((res) => res.text()); const doc = new JSDOM(html, { url }); const reader = new Readability(doc.window.document); const article = reader.parse(); console.log(article);
article
物件包含各種解析元素:
這包括標題、作者、摘錄、出版時間以及 HTML (content
) 和純文字 (textContent
)。 textContent
已準備好進行分塊、嵌入和存儲,而 content
保留連結和圖像以供進一步處理。
isProbablyReaderable
函數有助於確定文件是否適合 Readability.js:
const html = await fetch( "https://www.datastax.com/blog/how-to-create-vector-embeddings-in-node-js" ).then((res) => res.text()); console.log(html);
不合適的頁面應被標記以供審核。
將可讀性與 LangChain.js 整合
Readability.js 與 LangChain.js 無縫整合。以下範例使用 LangChain.js 載入頁面,使用 MozillaReadabilityTransformer
擷取內容,使用 RecursiveCharacterTextSplitter
分割文本,使用 OpenAI 建立嵌入,並將資料儲存在 Astra DB 中。
所需的依賴項:
npm install cheerio
您需要 Astra DB 憑證(ASTRA_DB_APPLICATION_TOKEN
、ASTRA_DB_API_ENDPOINT
)和 OpenAI API 金鑰 (OPENAI_API_KEY
) 作為環境變數。
導入必要的模組:
import * as cheerio from "cheerio"; const html = await fetch( "https://www.datastax.com/blog/how-to-create-vector-embeddings-in-node-js" ).then((res) => res.text()); const $ = cheerio.load(html); console.log($("h1").text(), "\n"); console.log($("section#blog-content > div:first-child").text());
初始化組件:
npm install @mozilla/readability jsdom
載入、轉換、分割、嵌入和儲存文件:
import { Readability } from "@mozilla/readability"; import { JSDOM } from "jsdom"; const url = "https://www.datastax.com/blog/how-to-create-vector-embeddings-in-node-js"; const html = await fetch(url).then((res) => res.text()); const doc = new JSDOM(html, { url }); const reader = new Readability(doc.window.document); const article = reader.parse(); console.log(article);
透過 Readability.js 提高網頁抓取準確性
Readability.js 是一個為 Firefox 閱讀器模式提供支援的強大函式庫,可以有效地從網頁中提取相關數據,從而提高 RAG 資料品質。 可以直接使用,也可以透過LangChain.js的MozillaReadabilityTransformer
.
這只是攝取管道的初始階段。 分塊、嵌入和 Astra DB 儲存是建立 RAG 應用程式的後續步驟。
您是否使用其他方法來清理 RAG 應用程式中的網頁內容? 分享你的技巧!
以上是使用 Readability.js 清理 HTML 內容以進行檢索增強生成的詳細內容。更多資訊請關注PHP中文網其他相關文章!
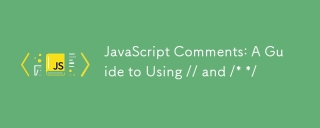
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
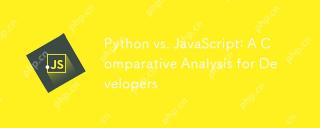
Python和JavaScript的主要區別在於類型系統和應用場景。 1.Python使用動態類型,適合科學計算和數據分析。 2.JavaScript採用弱類型,廣泛用於前端和全棧開發。兩者在異步編程和性能優化上各有優勢,選擇時應根據項目需求決定。
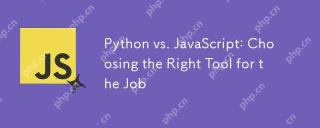
選擇Python還是JavaScript取決於項目類型:1)數據科學和自動化任務選擇Python;2)前端和全棧開發選擇JavaScript。 Python因其在數據處理和自動化方面的強大庫而備受青睞,而JavaScript則因其在網頁交互和全棧開發中的優勢而不可或缺。
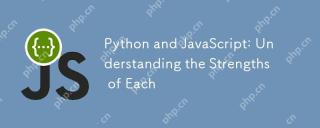
Python和JavaScript各有優勢,選擇取決於項目需求和個人偏好。 1.Python易學,語法簡潔,適用於數據科學和後端開發,但執行速度較慢。 2.JavaScript在前端開發中無處不在,異步編程能力強,Node.js使其適用於全棧開發,但語法可能複雜且易出錯。
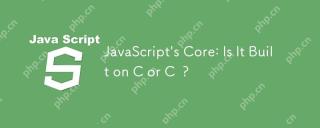
javascriptisnotbuiltoncorc; sanInterpretedlanguagethatrunsonenginesoftenwritteninc.1)JavascriptwasdesignedAsignedAsalightWeight,drackendedlanguageforwebbrowsers.2)Enginesevolvedfromsimpleterterpretpretpretpretpreterterpretpretpretpretpretpretpretpretpretcompilerers,典型地,替代品。
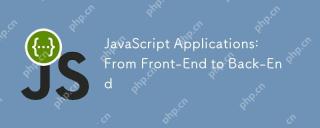
JavaScript可用於前端和後端開發。前端通過DOM操作增強用戶體驗,後端通過Node.js處理服務器任務。 1.前端示例:改變網頁文本內容。 2.後端示例:創建Node.js服務器。
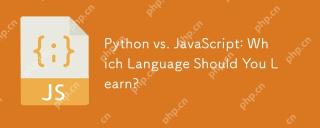
選擇Python還是JavaScript應基於職業發展、學習曲線和生態系統:1)職業發展:Python適合數據科學和後端開發,JavaScript適合前端和全棧開發。 2)學習曲線:Python語法簡潔,適合初學者;JavaScript語法靈活。 3)生態系統:Python有豐富的科學計算庫,JavaScript有強大的前端框架。
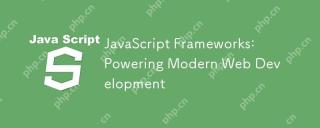
JavaScript框架的強大之處在於簡化開發、提升用戶體驗和應用性能。選擇框架時應考慮:1.項目規模和復雜度,2.團隊經驗,3.生態系統和社區支持。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。
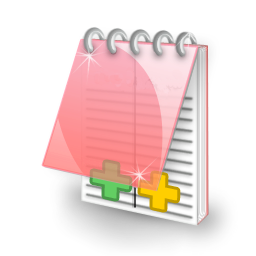
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
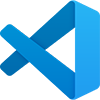
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器
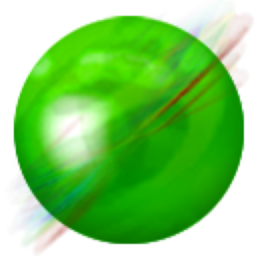
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具