<?php // Database connection (replace with your credentials) $host = 'localhost'; $dbname = 'your_database'; $user = 'your_user'; $password = 'your_password'; try { $dbh = new PDO("mysql:host=$host;dbname=$dbname", $user, $password); $dbh->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); } catch (PDOException $e) { die("Database connection failed: " . $e->getMessage()); } // Get total number of records $stmt = $dbh->query('SELECT COUNT(*) FROM your_table'); $totalRecords = $stmt->fetchColumn(); // Pagination settings $recordsPerPage = 20; $totalPages = ceil($totalRecords / $recordsPerPage); $currentPage = isset($_GET['page']) ? (int)$_GET['page'] : 1; $currentPage = max(1, min($currentPage, $totalPages)); // Ensure page number is within valid range $offset = ($currentPage - 1) * $recordsPerPage; // Fetch data for current page $stmt = $dbh->prepare("SELECT * FROM your_table LIMIT :limit OFFSET :offset"); $stmt->bindParam(':limit', $recordsPerPage, PDO::PARAM_INT); $stmt->bindParam(':offset', $offset, PDO::PARAM_INT); $stmt->execute(); $data = $stmt->fetchAll(PDO::FETCH_ASSOC); // Display data echo "<h2 id="Data-Page-currentPage-of-totalPages">Data (Page " . $currentPage . " of " . $totalPages . ")</h2>"; if ($data) { echo "<ul>"; foreach ($data as $row) { echo "<li>" . implode(", ", $row) . "</li>"; // Adjust as needed for your table structure } echo "</ul>"; } else { echo "<p>No data found for this page.</p>"; } // Display pagination links echo "<div class='pagination'>"; for ($i = 1; $i <= $totalPages; $i++) { $activeClass = ($i == $currentPage) ? 'active' : ''; echo "<a href='?page=" . $i . "' class='" . $activeClass . "'>" . $i . "</a>"; } echo "</div>"; ?> <!DOCTYPE html> <html> <head> <title>Simple Pagination</title> <style> .pagination a { display: inline-block; padding: 8px 12px; margin: 0 4px; text-decoration: none; border: 1px solid #ccc; } .pagination a.active { background-color: #4CAF50; color: white; } </style> </head> <body> </body> </html>
這個改進的範例包括:
-
錯誤處理:
try-catch
區塊處理潛在的資料庫連線錯誤。 - 準備語句: 使用準備語句來防止 SQL 注入漏洞。 這對於安全至關重要。
-
輸入驗證:雖然原始範例使用
filter_input
,但它缺乏全面的驗證。 此版本確保頁碼為正整數且在有效範圍內。 -
更清晰的資料顯示:資料以更使用者友善的格式(無序列表)顯示。 您需要調整
implode
部分以符合資料庫表的列名稱。 - 完整的 HTML: 提供完整的 HTML 結構以實現更好的渲染。
- CSS 樣式: 包含基本 CSS,用於設定分頁連結的樣式。
-
佔位符值: 請記得將
"your_database"
、"your_user"
、"your_password"
和"your_table"
替換為您的實際資料庫憑證和表格名稱。
請記住在運行此程式碼之前建立必要的資料庫和表。 這個增強的範例為 PHP 分頁提供了更強大、更安全的解決方案。
以上是如何用PHP實作簡單的資料庫分頁?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
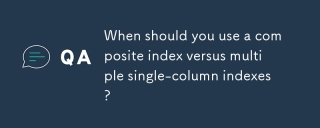
在數據庫優化中,應根據查詢需求選擇索引策略:1.當查詢涉及多個列且條件順序固定時,使用複合索引;2.當查詢涉及多個列但條件順序不固定時,使用多個單列索引。複合索引適用於優化多列查詢,單列索引則適合單列查詢。
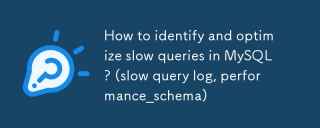
要優化MySQL慢查詢,需使用slowquerylog和performance_schema:1.啟用slowquerylog並設置閾值,記錄慢查詢;2.利用performance_schema分析查詢執行細節,找出性能瓶頸並優化。
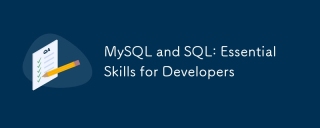
MySQL和SQL是開發者必備技能。 1.MySQL是開源的關係型數據庫管理系統,SQL是用於管理和操作數據庫的標準語言。 2.MySQL通過高效的數據存儲和檢索功能支持多種存儲引擎,SQL通過簡單語句完成複雜數據操作。 3.使用示例包括基本查詢和高級查詢,如按條件過濾和排序。 4.常見錯誤包括語法錯誤和性能問題,可通過檢查SQL語句和使用EXPLAIN命令優化。 5.性能優化技巧包括使用索引、避免全表掃描、優化JOIN操作和提升代碼可讀性。
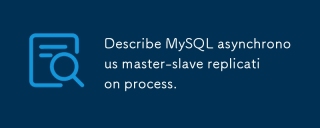
MySQL異步主從復制通過binlog實現數據同步,提升讀性能和高可用性。 1)主服務器記錄變更到binlog;2)從服務器通過I/O線程讀取binlog;3)從服務器的SQL線程應用binlog同步數據。
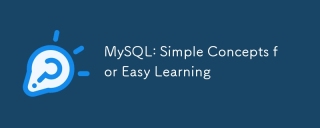
MySQL是一個開源的關係型數據庫管理系統。 1)創建數據庫和表:使用CREATEDATABASE和CREATETABLE命令。 2)基本操作:INSERT、UPDATE、DELETE和SELECT。 3)高級操作:JOIN、子查詢和事務處理。 4)調試技巧:檢查語法、數據類型和權限。 5)優化建議:使用索引、避免SELECT*和使用事務。
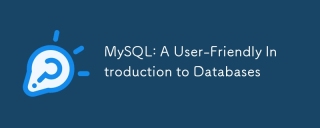
MySQL的安裝和基本操作包括:1.下載並安裝MySQL,設置根用戶密碼;2.使用SQL命令創建數據庫和表,如CREATEDATABASE和CREATETABLE;3.執行CRUD操作,使用INSERT,SELECT,UPDATE,DELETE命令;4.創建索引和存儲過程以優化性能和實現複雜邏輯。通過這些步驟,你可以從零開始構建和管理MySQL數據庫。
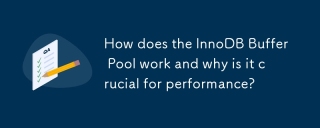
InnoDBBufferPool通過將數據和索引頁加載到內存中來提升MySQL數據庫的性能。 1)數據頁加載到BufferPool中,減少磁盤I/O。 2)臟頁被標記並定期刷新到磁盤。 3)LRU算法管理數據頁淘汰。 4)預讀機制提前加載可能需要的數據頁。
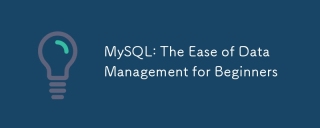
MySQL適合初學者使用,因為它安裝簡單、功能強大且易於管理數據。 1.安裝和配置簡單,適用於多種操作系統。 2.支持基本操作如創建數據庫和表、插入、查詢、更新和刪除數據。 3.提供高級功能如JOIN操作和子查詢。 4.可以通過索引、查詢優化和分錶分區來提升性能。 5.支持備份、恢復和安全措施,確保數據的安全和一致性。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

PhpStorm Mac 版本
最新(2018.2.1 )專業的PHP整合開發工具

SublimeText3漢化版
中文版,非常好用

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

禪工作室 13.0.1
強大的PHP整合開發環境