身為暢銷書作家,我鼓勵您在亞馬遜上探索我的書。 請記得在 Medium 上關注我以獲取更新和支持!您的參與意義重大。
高效的 goroutine 池管理對於創建高效能、可擴展的並發 Go 應用程式至關重要。 結構良好的池可以有效管理資源、提高效能並增強程式穩定性。
核心原則是維護一定數量的可重複使用的worker goroutine。這限制了活躍的 goroutine,防止資源耗盡並優化系統效能。
讓我們來看看在 Go 中創建強大的 goroutine 池的實現和最佳實踐。
我們先定義池的結構:
type Pool struct { tasks chan Task workers int wg sync.WaitGroup } type Task func() error
Pool
結構體包含任務通道、工作執行緒數和用於同步的 WaitGroup
。 Task
表示執行工作並傳回錯誤的函數。
接下來,我們將實現池的核心功能:
func NewPool(workers int) *Pool { return &Pool{ tasks: make(chan Task), workers: workers, } } func (p *Pool) Start() { for i := 0; i < p.workers; i++ { p.wg.Add(1) go p.worker() } } func (p *Pool) Submit(task Task) { p.tasks <- task } func (p *Pool) Stop() { close(p.tasks) p.wg.Wait() } func (p *Pool) worker() { defer p.wg.Done() for task := range p.tasks { task() } }
Start
方法啟動工作 goroutine,每個 goroutine 不斷檢索和執行任務。 Submit
新增任務,Stop
優雅地關閉池子。
使用泳池:
func main() { pool := NewPool(5) pool.Start() for i := 0; i < 10; i++ { pool.Submit(func() error { // ... task execution ... return nil }) } pool.Stop() }
這提供了一個基本的、功能性的 goroutine 池。 然而,一些改進可以提高其效率和穩健性。
一個關鍵的改進是處理工作人員內部的恐慌,以防止級聯故障:
func (p *Pool) worker() { defer p.wg.Done() defer func() { if r := recover(); r != nil { fmt.Printf("Recovered from panic: %v\n", r) } }() // ... rest of worker function ... }
增加等待所有提交任務完成的機制是另一個有價值的增強:
type Pool struct { // ... existing fields ... taskWg sync.WaitGroup } func (p *Pool) Submit(task Task) { p.taskWg.Add(1) p.tasks <- task defer p.taskWg.Done() } func (p *Pool) Wait() { p.taskWg.Wait() }
現在,pool.Wait()
確保在繼續之前完成所有任務。
動態大小調整讓池適應不同的工作負載:
type DynamicPool struct { tasks chan Task workerCount int32 maxWorkers int32 minWorkers int32 // ... other methods ... }
這涉及監控待處理的任務並在定義的限制內調整工作人員數量。 動態調整的實作細節比較複雜,為了簡潔就省略了。
錯誤處理至關重要;我們可以收集並報告錯誤:
type Pool struct { // ... existing fields ... errors chan error } func (p *Pool) Start() { // ... existing code ... p.errors = make(chan error, p.workers) } func (p *Pool) worker() { // ... existing code ... if err := task(); err != nil { p.errors <- err } }
這允許集中錯誤管理。
監控池性能在生產中至關重要。 新增指標集合可提供有價值的見解:
type PoolMetrics struct { // ... metrics ... } type Pool struct { // ... existing fields ... metrics PoolMetrics } func (p *Pool) Metrics() PoolMetrics { // ... metric retrieval ... }
這些指標可用於監控和效能分析。
工作竊取、動態調整大小、超時正常關閉以及其他先進技術可以進一步優化池性能。 具體實現很大程度上取決於應用程式的需求。 始終進行分析和基準測試,以確保池提供預期的性能提升。 精心設計的 goroutine 池可以顯著提高 Go 應用程式的可擴展性和效率。
101本書
101 Books是一家人工智慧出版社,由作家Aarav Joshi共同創立。 我們的人工智慧驅動方法使出版成本保持較低——一些書籍僅需4 美元——讓每個人都能獲得高品質的知識。
在亞馬遜上找到我們的書Golang Clean Code。
保持更新!在亞馬遜上搜尋 Aarav Joshi 了解更多書籍和特別優惠!
我們的出版品
探索我們的其他出版品:
投資者中心 | 投資者中心(西班牙語) | 投資者中心(德語) | 智能生活 | 時代與迴響 | 令人費解的謎團 | 印度教 | 菁英發展 | JS學校
在 Medium 上找到我們
科技無尾熊洞察 | 時代與迴響世界 | 投資者中心(中) | 令人費解的謎團(中) | 科學與時代(中) | 現代印度教
以上是掌握 Go 中的 Goroutine 池管理:提高效能和可擴充性的詳細內容。更多資訊請關注PHP中文網其他相關文章!
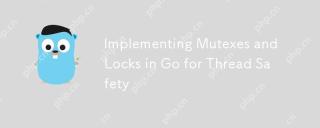
在Go中,使用互斥鎖和鎖是確保線程安全的關鍵。 1)使用sync.Mutex進行互斥訪問,2)使用sync.RWMutex處理讀寫操作,3)使用原子操作進行性能優化。掌握這些工具及其使用技巧對於編寫高效、可靠的並發程序至關重要。
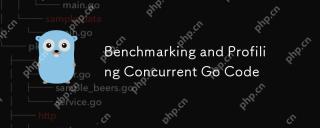
如何優化並發Go代碼的性能?使用Go的內置工具如gotest、gobench和pprof進行基準測試和性能分析。 1)使用testing包編寫基準測試,評估並發函數的執行速度。 2)通過pprof工具進行性能分析,識別程序中的瓶頸。 3)調整垃圾收集設置以減少其對性能的影響。 4)優化通道操作和限制goroutine數量以提高效率。通過持續的基準測試和性能分析,可以有效提升並發Go代碼的性能。
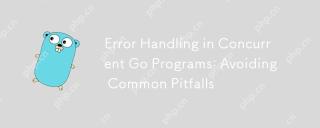
避免並發Go程序中錯誤處理的常見陷阱的方法包括:1.確保錯誤傳播,2.處理超時,3.聚合錯誤,4.使用上下文管理,5.錯誤包裝,6.日誌記錄,7.測試。這些策略有助於有效處理並發環境中的錯誤。
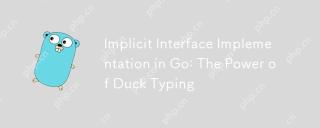
IndimitInterfaceImplementationingingoembodiesducktybybyallowingTypestoSatoSatiSatiSatiSatiSatiSatsatSatiSatplicesWithouTexpliclIctDeclaration.1)itpromotesflemotesflexibility andmodularitybybyfocusingion.2)挑戰挑戰InclocteSincludeUpdatingMethodSignateSignatiSantTrackingImplections.3)工具li
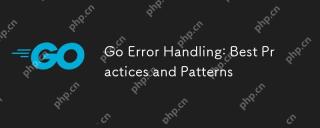
在Go編程中,有效管理錯誤的方法包括:1)使用錯誤值而非異常,2)採用錯誤包裝技術,3)定義自定義錯誤類型,4)復用錯誤值以提高性能,5)謹慎使用panic和recover,6)確保錯誤消息清晰且一致,7)記錄錯誤處理策略,8)將錯誤視為一等公民,9)使用錯誤通道處理異步錯誤。這些做法和模式有助於編寫更健壯、可維護和高效的代碼。
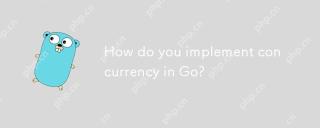
在Go中實現並發可以通過使用goroutines和channels來實現。 1)使用goroutines來並行執行任務,如示例中同時享受音樂和觀察朋友。 2)通過channels在goroutines之間安全傳遞數據,如生產者和消費者模式。 3)避免過度使用goroutines和死鎖,合理設計系統以優化並發程序。
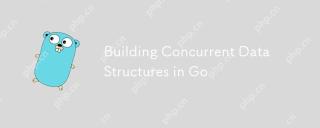
Gooffersmultipleapproachesforbuildingconcurrentdatastructures,includingmutexes,channels,andatomicoperations.1)Mutexesprovidesimplethreadsafetybutcancauseperformancebottlenecks.2)Channelsofferscalabilitybutmayblockiffullorempty.3)Atomicoperationsareef
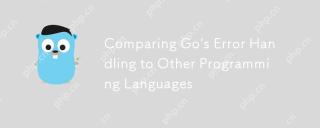
go'serrorhandlingisexplicit,治療eRROSASRETRATERTHANEXCEPTIONS,與pythonandjava.1)go'sapphifeensuresererrawaresserrorawarenessbutcanleadtoverbosecode.2)pythonandjavauseexeexceptionseforforforforforcleanerCodebutmaymobisserrors.3)


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

Atom編輯器mac版下載
最受歡迎的的開源編輯器

記事本++7.3.1
好用且免費的程式碼編輯器

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

SublimeText3漢化版
中文版,非常好用

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),