React Context 是一個很棒的工具——就像一個神奇的管道,可以跨組件傳遞共享數據,而不會出現道具鑽取的混亂。但這種便利性帶來了一個問題:未經檢查的使用可能會導致效能瓶頸,從而削弱您的應用程式。
在這篇部落格中,我們將探索如何掌握 React Context,同時避開常見的陷阱。最後,您將成為 Context 專業人士,擁有優化的高效能應用程式。
1.什麼是 React Context 以及為什麼你應該關心?
React Context 是將應用程式的元件編織在一起的無形執行緒。它可以實現資料共享,而無需在元件樹的每一層傳遞 props。
這是一個簡單的範例:
const ThemeContext = React.createContext('light'); // Default: light theme function App() { return ( <themecontext.provider value="dark"> <toolbar></toolbar> </themecontext.provider> ); } function ThemedButton() { const theme = React.useContext(ThemeContext); return <button> <hr> <h2> <strong>2. The Hidden Dangers of React Context</strong> </h2> <h3> <strong>Context Change = Full Re-render</strong> </h3> <p>Whenever a context value updates, all consumers re-render. Even if the specific value a consumer uses hasn’t changed, React doesn’t know, and it re-renders anyway.</p> <p>For example, in a responsive app using AdaptivityContext:<br> </p> <pre class="brush:php;toolbar:false">const AdaptivityContext = React.createContext({ width: 0, isMobile: false }); function App() { const [width, setWidth] = React.useState(window.innerWidth); const isMobile = width <header></header> <footer></footer> ); }
在這裡,AdaptivityContext 的每個用戶都會在任何寬度變化時重新渲染 - 即使他們只關心 isMobile。
3.透過最佳實務增強環境
規則 1:縮小上下文
將上下文分解為邏輯單元,以防止不必要的重新渲染。
const SizeContext = React.createContext(0); const MobileContext = React.createContext(false); function App() { const [width, setWidth] = React.useState(window.innerWidth); const isMobile = width <mobilecontext.provider value="{isMobile}"> <header></header> <footer></footer> </mobilecontext.provider> ); }
規則 2:穩定上下文值
使用 useMemo 避免在每次渲染時為上下文值建立新物件。
const memoizedValue = React.useMemo(() => ({ isMobile }), [isMobile]); <mobilecontext.provider value="{memoizedValue}"> <header></header> </mobilecontext.provider>;
規則 3:使用較小的上下文消費者
將上下文相關的程式碼移到更小的、獨立的元件中以限制重新渲染。
function ModalClose() { const isMobile = React.useContext(MobileContext); return !isMobile ? <button>Close</button> : null; } function Modal() { return ( <div> <h1 id="Modal-Content">Modal Content</h1> <modalclose></modalclose> </div> ); }
4.當背景不夠時:了解你的極限
上下文適合全域、輕量級數據,例如主題、區域設定或使用者身份驗證。對於複雜的狀態管理,請考慮 Redux、Zustand 或 Jotai 等函式庫。
5.備忘單:React 上下文概覽
Concept | Description | Example |
---|---|---|
Create Context | Creates a context with a default value. | const ThemeContext = React.createContext('light'); |
Provider | Makes context available to child components. | |
useContext Hook | Accesses the current context value. | const theme = React.useContext(ThemeContext); |
Split Contexts | Separate context values with different update patterns. | const SizeContext = React.createContext(); const MobileContext = React.createContext(); |
Stabilize Values | Use useMemo to stabilize context objects. | const memoValue = useMemo(() => ({ key }), [key]); |
Avoid Full Re-renders | Isolate context usage in smaller components or use libraries like use-context-selector. | |
When Not to Use Context | Avoid for complex state; use dedicated state management libraries. | Use Redux or Zustand for large-scale state management. |
描述
useContext 鉤子
- 穩定價值觀
6. React Context 的未來
以上是揭開 React Context 的秘密:力量、陷阱和性能的詳細內容。更多資訊請關注PHP中文網其他相關文章!
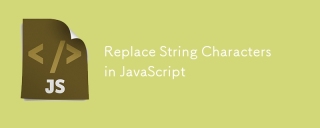
JavaScript字符串替換方法詳解及常見問題解答 本文將探討兩種在JavaScript中替換字符串字符的方法:在JavaScript代碼內部替換和在網頁HTML內部替換。 在JavaScript代碼內部替換字符串 最直接的方法是使用replace()方法: str = str.replace("find","replace"); 該方法僅替換第一個匹配項。要替換所有匹配項,需使用正則表達式並添加全局標誌g: str = str.replace(/fi
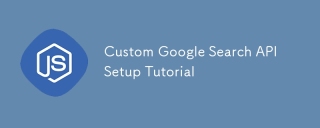
本教程向您展示瞭如何將自定義的Google搜索API集成到您的博客或網站中,提供了比標準WordPress主題搜索功能更精緻的搜索體驗。 令人驚訝的是簡單!您將能夠將搜索限制為Y
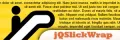
利用輕鬆的網頁佈局:8 ESTISSEL插件jQuery大大簡化了網頁佈局。 本文重點介紹了簡化該過程的八個功能強大的JQuery插件,對於手動網站創建特別有用
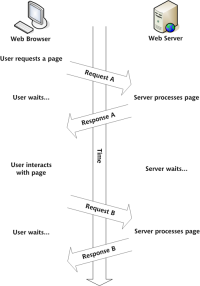
因此,在這裡,您準備好了解所有稱為Ajax的東西。但是,到底是什麼? AJAX一詞是指用於創建動態,交互式Web內容的一系列寬鬆的技術。 Ajax一詞,最初由Jesse J創造
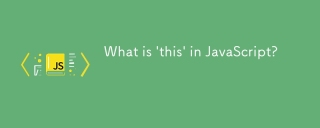
核心要點 JavaScript 中的 this 通常指代“擁有”該方法的對象,但具體取決於函數的調用方式。 沒有當前對象時,this 指代全局對象。在 Web 瀏覽器中,它由 window 表示。 調用函數時,this 保持全局對象;但調用對象構造函數或其任何方法時,this 指代對象的實例。 可以使用 call()、apply() 和 bind() 等方法更改 this 的上下文。這些方法使用給定的 this 值和參數調用函數。 JavaScript 是一門優秀的編程語言。幾年前,這句話可

jQuery是一個很棒的JavaScript框架。但是,與任何圖書館一樣,有時有必要在引擎蓋下發現發生了什麼。也許是因為您正在追踪一個錯誤,或者只是對jQuery如何實現特定UI感到好奇
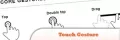
該帖子編寫了有用的作弊表,參考指南,快速食譜以及用於Android,BlackBerry和iPhone應用程序開發的代碼片段。 沒有開發人員應該沒有他們! 觸摸手勢參考指南(PDF)是Desig的寶貴資源


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Atom編輯器mac版下載
最受歡迎的的開源編輯器
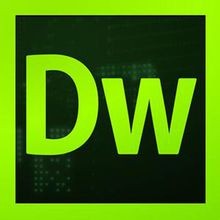
Dreamweaver CS6
視覺化網頁開發工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

禪工作室 13.0.1
強大的PHP整合開發環境