在 C# 中使用非同步鎖定解決間歇性檔案存取錯誤
非同步鎖定,特別是在使用雜湊 URL 和類似 AsyncDuplicateLock
的類別時,有時會導致間歇性檔案存取錯誤。 這通常源自於並發字典中信號量處理不當。 最初有缺陷的方法可能如下:
SemaphoreSlim locker; if (SemaphoreSlims.TryRemove(s, out locker)) { locker.Release(); locker.Dispose(); }
這裡的問題是在釋放信號量之前刪除它。這會造成過多的信號量流失,導致即使從字典中刪除信號量後仍會持續使用信號量。
強大的解決方案採用引用計數來管理信號量生命週期:
public sealed class AsyncDuplicateLock { private sealed class RefCounted<T> { public RefCounted(T value) { RefCount = 1; Value = value; } public int RefCount { get; set; } public T Value { get; private set; } } private static readonly Dictionary<object, RefCounted<SemaphoreSlim>> SemaphoreSlims = new Dictionary<object, RefCounted<SemaphoreSlim>>(); private SemaphoreSlim GetOrCreate(object key) { RefCounted<SemaphoreSlim> item; lock (SemaphoreSlims) { if (SemaphoreSlims.TryGetValue(key, out item)) { ++item.RefCount; } else { item = new RefCounted<SemaphoreSlim>(new SemaphoreSlim(1, 1)); SemaphoreSlims[key] = item; } } return item.Value; } public IDisposable Lock(object key) { GetOrCreate(key).Wait(); return new Releaser { Key = key }; } public async Task<IDisposable> LockAsync(object key) { await GetOrCreate(key).WaitAsync().ConfigureAwait(false); return new Releaser { Key = key }; } private sealed class Releaser : IDisposable { public object Key { get; set; } public void Dispose() { RefCounted<SemaphoreSlim> item; lock (SemaphoreSlims) { item = SemaphoreSlims[Key]; --item.RefCount; if (item.RefCount == 0) SemaphoreSlims.Remove(Key); } item.Value.Release(); } } }
此修訂後的程式碼使用 RefCounted<t></t>
包裝器來追蹤信號量參考。 信號量只有在引用計數為零時才會從字典中刪除,確保正確釋放並防止過早處置,從而消除間歇性文件存取錯誤。
以上是如何在 C# 中使用非同步鎖定避免間歇性檔案存取錯誤?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
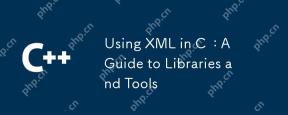
在C 中使用XML是因為它提供了結構化數據的便捷方式,尤其在配置文件、數據存儲和網絡通信中不可或缺。 1)選擇合適的庫,如TinyXML、pugixml、RapidXML,根據項目需求決定。 2)了解XML解析和生成的兩種方式:DOM適合頻繁訪問和修改,SAX適用於大文件或流數據。 3)優化性能時,TinyXML適合小文件,pugixml在內存和速度上表現好,RapidXML處理大文件優異。
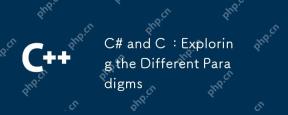
C#和C 的主要區別在於內存管理、多態性實現和性能優化。 1)C#使用垃圾回收器自動管理內存,C 則需要手動管理。 2)C#通過接口和虛方法實現多態性,C 使用虛函數和純虛函數。 3)C#的性能優化依賴於結構體和並行編程,C 則通過內聯函數和多線程實現。
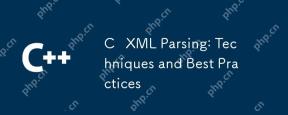
C 中解析XML數據可以使用DOM和SAX方法。 1)DOM解析將XML加載到內存,適合小文件,但可能佔用大量內存。 2)SAX解析基於事件驅動,適用於大文件,但無法隨機訪問。選擇合適的方法並優化代碼可提高效率。
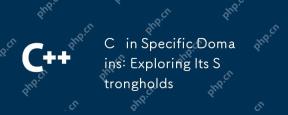
C 在遊戲開發、嵌入式系統、金融交易和科學計算等領域中的應用廣泛,原因在於其高性能和靈活性。 1)在遊戲開發中,C 用於高效圖形渲染和實時計算。 2)嵌入式系統中,C 的內存管理和硬件控制能力使其成為首選。 3)金融交易領域,C 的高性能滿足實時計算需求。 4)科學計算中,C 的高效算法實現和數據處理能力得到充分體現。
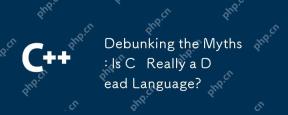
C 沒有死,反而在許多關鍵領域蓬勃發展:1)遊戲開發,2)系統編程,3)高性能計算,4)瀏覽器和網絡應用,C 依然是主流選擇,展現了其強大的生命力和應用場景。
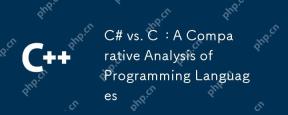
C#和C 的主要區別在於語法、內存管理和性能:1)C#語法現代,支持lambda和LINQ,C 保留C特性並支持模板。 2)C#自動內存管理,C 需要手動管理。 3)C 性能優於C#,但C#性能也在優化中。
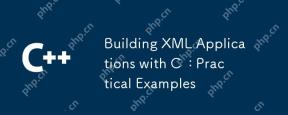
在C 中處理XML數據可以使用TinyXML、Pugixml或libxml2庫。 1)解析XML文件:使用DOM或SAX方法,DOM適合小文件,SAX適合大文件。 2)生成XML文件:將數據結構轉換為XML格式並寫入文件。通過這些步驟,可以有效地管理和操作XML數據。
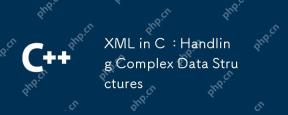
在C 中處理XML數據結構可以使用TinyXML或pugixml庫。 1)使用pugixml庫解析和生成XML文件。 2)處理複雜的嵌套XML元素,如書籍信息。 3)優化XML處理代碼,建議使用高效庫和流式解析。通過這些步驟,可以高效處理XML數據。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。
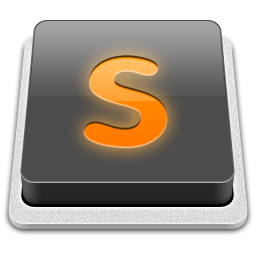
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

記事本++7.3.1
好用且免費的程式碼編輯器
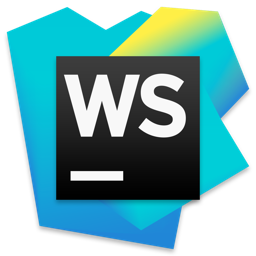
WebStorm Mac版
好用的JavaScript開發工具