動態指定LINQ OrderBy參數
在C#中,LINQ的OrderBy方法允許根據指定的屬性或表達式對資料進行排序。但是,我們如何動態地指定要排序的屬性或表達式呢?
問題:
給定一個參數,我們希望根據指定的屬性對學生清單進行排序,但目前,OrderBy呼叫中的屬性名稱是硬編碼的。
範例:
List<Student> existingStudents = new List<Student> { new Student { ... }, new Student { ... } }; List<Student> orderByAddress = existingStudents.OrderBy(c => c.Address).ToList();
目標:
我們希望將屬性名稱作為參數,而不是在OrderBy中使用靜態屬性名稱。
範例:
string param = "City"; List<Student> orderByCity = existingStudents.OrderBy(c => c.City).ToList(); //仍然需要明确指定属性
解:
使用反射,我們可以動態建立OrderBy表達式:
public static IQueryable<T> OrderBy<T>(this IQueryable<T> source, string orderByProperty, bool desc) { // ... (反射代码构建表达式树) ... return source.Provider.CreateQuery<T>(resultExpression); }
然後,您可以依照指定的屬性動態排序:
existingStudents.OrderBy("City", true); // 降序 existingStudents.OrderBy("City", false); // 升序
(注意:完整的反射程式碼實作較為複雜,此處省略。需要使用 System.Linq.Expressions
命名空間來建立表達式樹,並處理可能出現的異常,例如屬性不存在的情況。) 這個解決方案提供了動態排序的思路,完整的實作需要根據實際情況編寫更健壯的程式碼。 直接使用c => param
是錯誤的,因為這只會對參數字串本身排序,而不是對學生物件的屬性排序。 必須使用反射來動態取得屬性並建立正確的表達式樹。
以上是如何在 LINQ 中動態指定 OrderBy 屬性?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
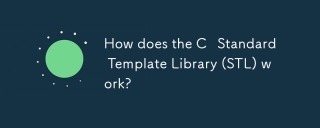
本文解釋了C標準模板庫(STL),重點關注其核心組件:容器,迭代器,算法和函子。 它詳細介紹了這些如何交互以啟用通用編程,提高代碼效率和可讀性t
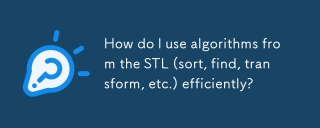
本文詳細介紹了c中有效的STL算法用法。 它強調了數據結構選擇(向量與列表),算法複雜性分析(例如,std :: sort vs. std vs. std :: partial_sort),迭代器用法和並行執行。 常見的陷阱
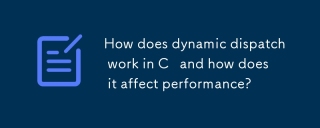
本文討論了C中的動態調度,其性能成本和優化策略。它突出了動態調度會影響性能並將其與靜態調度進行比較的場景,強調性能和之間的權衡
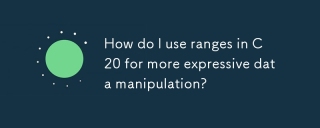
C 20範圍通過表現力,合成性和效率增強數據操作。它們簡化了複雜的轉換並集成到現有代碼庫中,以提高性能和可維護性。
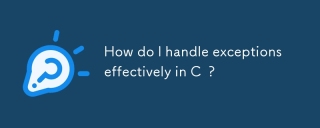
本文詳細介紹了C中的有效異常處理,涵蓋了嘗試,捕捉和投擲機制。 它強調了諸如RAII之類的最佳實踐,避免了不必要的捕獲塊,並為強大的代碼登錄例外。 該文章還解決了Perf
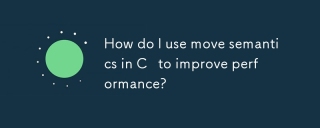
本文討論了使用C中的移動語義來通過避免不必要的複制來提高性能。它涵蓋了使用std :: Move的實施移動構造函數和任務運算符,並確定了關鍵方案和陷阱以有效
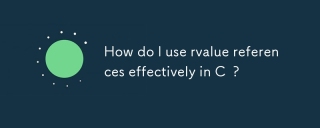
文章討論了在C中有效使用RVALUE參考,以進行移動語義,完美的轉發和資源管理,重點介紹最佳實踐和性能改進。(159個字符)


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
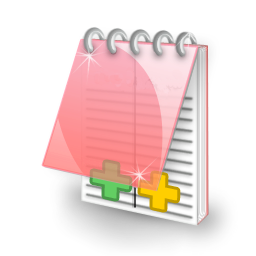
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
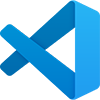
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器
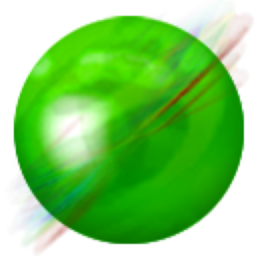
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

SublimeText3漢化版
中文版,非常好用