繼續我的編碼之旅(第一天仍然沒有寫下來,也許永遠!),我正在處理實時用戶驗證程序以阻止未經授權的訪問。 這是一個看似簡單的想法,但請聽我說完。
預期功能:
程式在系統啟動時謹慎運行,並定期(例如每小時)提示輸入密碼。 至關重要的是,它保持高優先級,防止關閉或最小化。密碼輸入錯誤會導致系統關閉。
from tkinter import * import subprocess import threading import time import getpass # Added for secure password input window = Tk() window.title("User Verification") window.config(background="black") # Initialize password (should be replaced with a more secure method) q = getpass.getpass("Set initial password: ") entry = Entry(window, fg='#00FF00', bg='black', font=('Arial',30), show='*') # Mask password input entry.pack(side=RIGHT) def verify_user(): global q while True: y = entry.get() if y != q: subprocess.run('shutdown /s', shell=True) break # Exit the loop after shutdown else: print('Verification successful.') # Replace password here (securely!) q = getpass.getpass("Set new password: ") entry.delete(0, END) # Clear entry field time.sleep(3600) # Check every hour def start_verification(): verification_thread = threading.Thread(target=verify_user) verification_thread.daemon = True # Allow program to exit even if thread is running verification_thread.start() u = Button(window, text='Start Verification', # Changed button text fg='#00FF00', bg='black', command=start_verification) u.pack(side=BOTTOM) t = Label(window, text='Enter Password:', # Simplified label text font=('Arial',15), fg='#00FF00', bg='black') t.pack(side=LEFT) window.mainloop()
計畫的增強功能:
這是一個初級版本。 未來的改進包括:
-
動態密碼變更:實作一種安全方法,在每次成功驗證後變更密碼。 目前的
getpass
模組提供了一個開始,但需要更強大的密碼管理。 - 後台執行緒:程式碼現在使用守護執行緒在後台運行驗證過程,允許主視窗保持回應(儘管是最低限度的回應)。
免責聲明:這是一個基本範例,缺乏強大的安全功能。 使用風險自負。 對於生產級安全性,請使用已建立的身份驗證系統。 歡迎提出建議(但不保證一定會得到答案!)。
以上是日間編碼之旅)的詳細內容。更多資訊請關注PHP中文網其他相關文章!
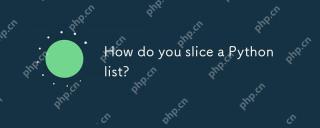
SlicingaPythonlistisdoneusingthesyntaxlist[start:stop:step].Here'showitworks:1)Startistheindexofthefirstelementtoinclude.2)Stopistheindexofthefirstelementtoexclude.3)Stepistheincrementbetweenelements.It'susefulforextractingportionsoflistsandcanuseneg

numpyallowsforvariousoperationsonArrays:1)basicarithmeticlikeaddition,減法,乘法和division; 2)evationAperationssuchasmatrixmultiplication; 3)element-wiseOperations wiseOperationswithOutexpliitloops; 4)
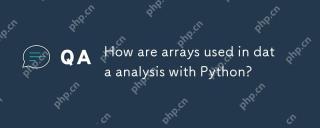
Arresinpython,尤其是Throughnumpyandpandas,weessentialFordataAnalysis,offeringSpeedAndeffied.1)NumpyArseNable efflaysenable efficefliceHandlingAtaSetSetSetSetSetSetSetSetSetSetSetsetSetSetSetSetsopplexoperationslikemovingaverages.2)
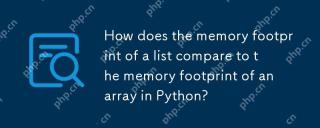
列表sandnumpyArraysInpythonHavedIfferentMemoryfootprints:listSaremoreFlexibleButlessMemory-效率,而alenumpyArraySareSareOptimizedFornumericalData.1)listsStorReereReereReereReereFerenceStoObjects,with withOverHeadeBheadaroundAroundaround64byty64-bitsysysysysysysysysyssyssyssyssysssyssys2)
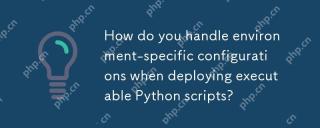
toensurepythonscriptsbehavecorrectlyacrycrosdevelvermations,分期和生產,USETHESTERTATE:1)Environment varriablesForsimplesettings,2)configurationfilesfilesForcomPlexSetups,3)dynamiCofforComplexSetups,dynamiqualloadingForaptaptibality.eachmethodoffersuniquebeneiquebeneqeniquebenefitsandrefitsandrequiresandrequiresandrequiresca
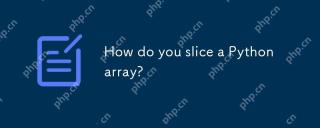
Python列表切片的基本語法是list[start:stop:step]。 1.start是包含的第一個元素索引,2.stop是排除的第一個元素索引,3.step決定元素之間的步長。切片不僅用於提取數據,還可以修改和反轉列表。
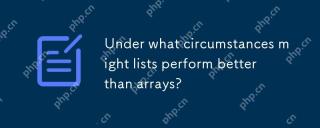
ListSoutPerformarRaysin:1)DynamicsizicsizingandFrequentInsertions/刪除,2)儲存的二聚體和3)MemoryFeliceFiceForceforseforsparsedata,butmayhaveslightperformancecostsinclentoperations。
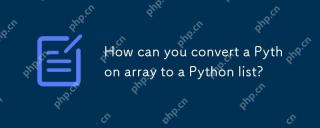
toConvertapythonarraytoalist,usEthelist()constructororageneratorexpression.1)intimpthearraymoduleandcreateanArray.2)USELIST(ARR)或[XFORXINARR] to ConconverTittoalist,請考慮performorefformanceandmemoryfformanceandmemoryfformienceforlargedAtasetset。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3 Linux新版
SublimeText3 Linux最新版

SublimeText3漢化版
中文版,非常好用
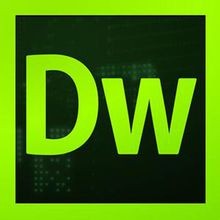
Dreamweaver CS6
視覺化網頁開發工具
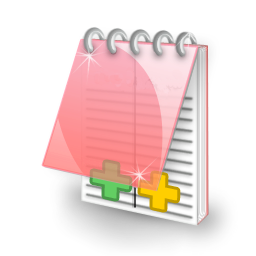
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能
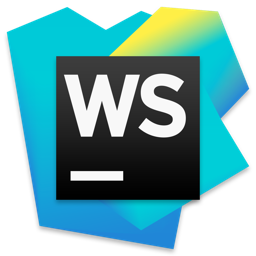
WebStorm Mac版
好用的JavaScript開發工具