這是該系列的第 7 部分,我將在其中記錄我使用 Django 學習 HTMX 的過程,其中我們將按照 HTMX 的文檔來實現待辦事項的無限滾動功能。
如果您想查看該系列的其餘部分,請查看 dev.to/rodbv 以獲得完整清單。
更新部分模板以載入多個項目
當我們實作無限滾動時,我們將必須傳回幾個待辦事項(專案的下一個「頁面」)並將它們載入到我們目前擁有的部分範本中。這意味著稍微改變我們的部分範本的組成方式;目前的設定如下圖所示,其中部分範本負責渲染單一待辦事項:
我們想要反轉順序,讓部分圍繞 for 迴圈:
讓我們在範本 core/templates/index.html 中執行交換:
Soon we will get back to the template to add the hx-get ... hx-trigger="revealed" bit that performs the infinite scroll, but first let's just change the view to return several items instead of one on the toggle and create operations:
... previous code def _create_todo(request): title = request.POST.get("title") if not title: raise ValueError("Title is required") todo = Todo.objects.create(title=title, user=request.user) return render( request, "tasks.html#todo-items-partial", # <p>檢查內容的測試仍然通過,而且頁面看起來相同,因此我們很好地實現無限滾動本身。 </p> <h2> 實現無限滾動 </h2> <p>在模板上,我們需要向/tasks 設定一個hx-get 請求,其中hx-trigger="revealed" ,這意味著只有當元素即將進入螢幕上可見時才會觸發GET 請求;這意味著我們希望將其設定在清單中最後一個元素之後,並且我們還需要指示要載入哪個「頁面」資料。在我們的例子中,我們將一次顯示 20 個項目。 </p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/173613850692024.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="Creating a To-Do app with HTMX and Django, part infinite scroll"></p> <p>讓我們相應地更改模板:<br> </p> <pre class="brush:php;toolbar:false">
- s we get from the server, which is great because not only we show the new data we got, but we also get rid of the loading icon.
We can now move to the views file.
As a recap, here's how the GET /tasks view looks like by now; it's always returning the full template.
@require_http_methods(["GET", "POST"]) @login_required def tasks(request): if request.method == "POST": return _create_todo(request) # GET /tasks context = { "todos": request.user.todos.all().order_by("-created_at"), "fullname": request.user.get_full_name() or request.user.username, } return render(request, "tasks.html", context)
上面的程式碼已經做了改動,就是按照最新的待辦事項優先排序;既然我們期望有一個很長的列表,那麼在底部添加新項目並將其與無限滾動混合是沒有意義的- 新項目最終將混合在清單的中間。
我們現在需要區分常規 GET 請求和 HTMX 請求,為此我們將只傳回待辦事項清單和部分範本。有一個名為django-htmx 的函式庫,它非常方便,因為它使用request.htmx 等屬性和所有hx-* 屬性的值擴展了請求參數,但目前這有點過分了;現在讓我們檢查HTMX 標頭,並使用Django 分頁器處理分頁。
# core/views.py ... previous code PAGE_SIZE = 20 ...previous code @require_http_methods(["GET", "POST"]) @login_required def tasks(request): if request.method == "POST": return _create_todo(request) page_number = int(request.GET.get("page", 1)) all_todos = request.user.todos.all().order_by("-created_at") paginator = Paginator(all_todos, PAGE_SIZE) curr_page = paginator.get_page(page_number) context = { "todos": curr_page.object_list, "fullname": request.user.get_full_name() or request.user.username, "next_page_number": page_number + 1 if curr_page.has_next() else None, } template_name = "tasks.html" if "HX-Request" in request.headers: template_name += "#todo-items-partial" return render(request, template_name, context)
我們做的第一件事是檢查頁面參數,如果不存在則將其設為 1。
我們檢查請求中的 HX-Request 標頭,這將告知我們傳入的請求是否來自 HTMX,並讓我們相應地返回部分模板或完整模板。
這段程式碼肯定需要一些測試,但在此之前讓我們先嘗試一下。看看網路工具,當頁面滾動時如何觸發請求,直到到達最後一頁。您還可以看到動畫「正在載入」圖示短暫顯示;我已將網路速度限制為 4g,以使其可見時間更長。
添加測試
最後,我們可以新增一個測驗來確保分頁能如預期運作
Soon we will get back to the template to add the hx-get ... hx-trigger="revealed" bit that performs the infinite scroll, but first let's just change the view to return several items instead of one on the toggle and create operations:
... previous code def _create_todo(request): title = request.POST.get("title") if not title: raise ValueError("Title is required") todo = Todo.objects.create(title=title, user=request.user) return render( request, "tasks.html#todo-items-partial", # <p>現在就這樣了!這是迄今為止我使用 HTMX 遇到的最有趣的事情。這篇文章的完整程式碼在這裡。 </p> <p>對於下一篇文章,我正在考慮使用 AlpineJS 新增一些客戶端狀態管理,或新增「截止日期」功能。再見! </p>
There's an if next_page_number check around the "loading" icon at the bottom of the list, it will have two purposes: one is to indicate when we're loading more data, but more importantly, when the loader is revealed (it appears on the visible part of the page), it will trigger the hx-get call to /tasks, passing the page number to be retrieved. The attribute next_page_number will also be provided by the context
The directive hx-swap:outerHTML indicates that we will replace the outerHTML of this element with the set of
以上是使用 HTMX 和 Django 建立待辦事項應用程序,部分無限滾動的詳細內容。更多資訊請關注PHP中文網其他相關文章!
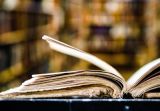
本教程演示如何使用Python處理Zipf定律這一統計概念,並展示Python在處理該定律時讀取和排序大型文本文件的效率。 您可能想知道Zipf分佈這個術語是什麼意思。要理解這個術語,我們首先需要定義Zipf定律。別擔心,我會盡量簡化說明。 Zipf定律 Zipf定律簡單來說就是:在一個大型自然語言語料庫中,最頻繁出現的詞的出現頻率大約是第二頻繁詞的兩倍,是第三頻繁詞的三倍,是第四頻繁詞的四倍,以此類推。 讓我們來看一個例子。如果您查看美國英語的Brown語料庫,您會注意到最頻繁出現的詞是“th
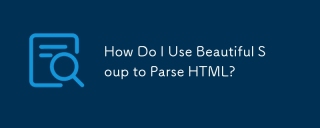
本文解釋瞭如何使用美麗的湯庫來解析html。 它詳細介紹了常見方法,例如find(),find_all(),select()和get_text(),以用於數據提取,處理不同的HTML結構和錯誤以及替代方案(SEL)
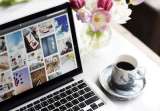
處理嘈雜的圖像是一個常見的問題,尤其是手機或低分辨率攝像頭照片。 本教程使用OpenCV探索Python中的圖像過濾技術來解決此問題。 圖像過濾:功能強大的工具圖像過濾器
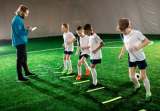
Python是數據科學和處理的最愛,為高性能計算提供了豐富的生態系統。但是,Python中的並行編程提出了獨特的挑戰。本教程探討了這些挑戰,重點是全球解釋
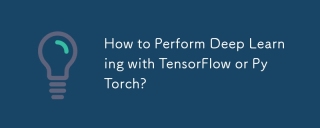
本文比較了Tensorflow和Pytorch的深度學習。 它詳細介紹了所涉及的步驟:數據準備,模型構建,培訓,評估和部署。 框架之間的關鍵差異,特別是關於計算刻度的
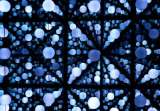
本教程演示了在Python 3中創建自定義管道數據結構,利用類和操作員超載以增強功能。 管道的靈活性在於它能夠將一系列函數應用於數據集的能力,GE
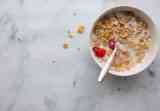
Python 對象的序列化和反序列化是任何非平凡程序的關鍵方面。如果您將某些內容保存到 Python 文件中,如果您讀取配置文件,或者如果您響應 HTTP 請求,您都會進行對象序列化和反序列化。 從某種意義上說,序列化和反序列化是世界上最無聊的事情。誰會在乎所有這些格式和協議?您想持久化或流式傳輸一些 Python 對象,並在以後完整地取回它們。 這是一種在概念層面上看待世界的好方法。但是,在實際層面上,您選擇的序列化方案、格式或協議可能會決定程序運行的速度、安全性、維護狀態的自由度以及與其他系
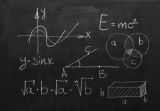
Python的statistics模塊提供強大的數據統計分析功能,幫助我們快速理解數據整體特徵,例如生物統計學和商業分析等領域。無需逐個查看數據點,只需查看均值或方差等統計量,即可發現原始數據中可能被忽略的趨勢和特徵,並更輕鬆、有效地比較大型數據集。 本教程將介紹如何計算平均值和衡量數據集的離散程度。除非另有說明,本模塊中的所有函數都支持使用mean()函數計算平均值,而非簡單的求和平均。 也可使用浮點數。 import random import statistics from fracti


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具
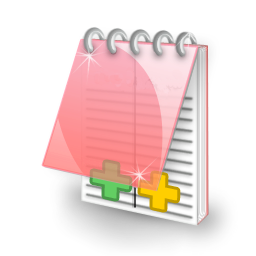
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!
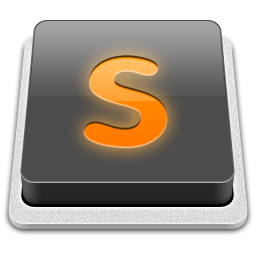
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)