為什麼選擇Python套件?
Python支援所有類型的執行;您可以直接在 shell 中運行 Python 程式碼,或將程式碼放入檔案中並稍後運行。
有時候開始一個新的Python專案是非常困難的;寫劇本?寫一個模組?寫個包嗎?
最好的選擇是微片模式:編寫一個腳本,然後在模組中重寫它,然後在套件中重寫。
這種模式可以讓您不必每天重新發明輪子,並且可以在將來重複使用程式碼。
Python包結構
Python 套件具有以下結構:
pkg ├── __init__.py ├── module1.py └── subpkg ├── __init__.py ├── __main__.py └── module2.py
資料夾pkg是一個包,因為包含__init__.py模組。 subpkg 資料夾也是一個包;是pkg的子包。
module1.py 和 module2.py 是其套件的模組。
__main__.py 模組允許執行套件。
只有這裡嗎?其他的事情?
如果你要成為 Python 開發人員,那麼你會使用其他常用工具。
依照順序,您所寫的每一段程式碼都遵循以下步驟:
- 將Python程式碼寫入你的套件
- 追蹤您的修改
- 測試您所寫的所有程式碼
- 將您的程式碼放入您測試的環境中
- 將程式碼推送到遠端儲存庫
- 建立您的分送包
- 將你的包上傳到 PyPi
管道
程式碼中的每一個變更都可能會引入可能的錯誤。為了放棄這個,每次我們需要在正確的環境中測試自己的包時。
要做到這一點,除了 Python 本身之外還需要一些工具,像是 git、docker 和 make。
文件、許可證和其他通用文件
僅僅創建一個 Python 套件並使其立即可供所有人使用是不夠的。您還必須考慮如何記錄它、向其他人簡要解釋它、許可它並解釋如何整合到專案中。
這需要發展諸如 README、LICENSE、CODE_OF_CONDUCT 和 CONTRIBUTING 等文件。
也許添加一個 CHANGELOG 來保存並讓其他人追蹤對每個版本所做的更改。
幾分鐘內建立項目
要實作 Python 專案的所有部分,需要幾個小時或幾天的時間。
但有一個用於此目的的工具:psp。
依照安裝說明進行操作後:
[test@ubuntu ~] sudo apt install -y python3 python3-pip git curl [test@ubuntu ~] curl -L https://github.com/MatteoGuadrini/psp/releases/download/v0.1.0/psp.deb -o psp.deb [test@ubuntu ~] sudo dpkg -i psp.deb
運行它:
[test@ubuntu ~] psp Welcome to PSP (Python Scaffolding Projects): 0.1.0 > Name of Python project: app > Do you want to create a virtual environment? Yes > Do you want to start git repository? Yes > Select git remote provider: Gitlab > Username of Gitlab: test_user > Do you want unit test files? Yes > Install dependencies: flask > Select documention generator: MKDocs > Do you want to configure tox? Yes > Select remote CI provider: CircleCI > Do you want create common files? Yes > Select license: Gnu Public License > Do you want to install dependencies to publish on pypi? Yes > Do you want to create a Dockerfile and Containerfile? Yes Python project `app` created at app
現在,檢查已建立的Python專案:
[test@ubuntu ~] ls -lah app total 88K drwxrwxr-x 9 test test 440 Dec 20 14:48 . drwxrwxrwt 29 root root 680 Dec 20 14:49 .. drwxrwxr-x 2 test test 60 Dec 20 14:47 .circleci drwxrwxr-x 7 test test 200 Dec 20 14:47 .git -rw-rw-r-- 1 test test 381 Dec 20 14:47 .gitignore drwxrwxr-x 4 test test 80 Dec 20 14:47 .gitlab -rw-rw-r-- 1 test test 127 Dec 20 14:48 CHANGES.md -rw-rw-r-- 1 test test 5.4K Dec 20 14:48 CODE_OF_CONDUCT.md -rw-rw-r-- 1 test test 1.1K Dec 20 14:48 CONTRIBUTING.md -rw-rw-r-- 1 test test 190 Dec 20 14:48 Containerfile -rw-rw-r-- 1 test test 190 Dec 20 14:48 Dockerfile -rw-rw-r-- 1 test test 35K Dec 20 14:48 LICENSE.md -rw-rw-r-- 1 test test 697 Dec 20 14:48 Makefile -rw-rw-r-- 1 test test 177 Dec 20 14:48 README.md drwxrwxr-x 2 test test 60 Dec 20 14:47 docs -rw-rw-r-- 1 test test 19 Dec 20 14:47 mkdocs.yml -rw-rw-r-- 1 test test 819 Dec 20 14:48 pyproject.toml -rw-rw-r-- 1 test test 66 Dec 20 14:47 requirements.txt drwxrwxr-x 2 test test 80 Dec 20 14:47 tests -rw-rw-r-- 1 test test 213 Dec 20 14:47 tox.ini drwxrwxr-x 2 test test 80 Dec 20 14:46 app drwxrwxr-x 5 test test 140 Dec 20 14:46 venv
開始開發包
開始開發 psp 指令為我們的專案所建立的套件。
[test@ubuntu ~] cd app/ && ls -lh app/ total 8.0K -rw-rw-r-- 1 test test 162 Dec 20 14:46 __init__.py -rw-rw-r-- 1 test test 204 Dec 20 14:46 __main__.py [test@ubuntu ~] vim app/core.py
from flask import Flask app = Flask(__name__) @app.route("/") def hello_world(): return "<p>Wow, this is my app!</p>"
現在,將我們的 hello_world 函數匯入到 __main__.py 檔案中:
pkg ├── __init__.py ├── module1.py └── subpkg ├── __init__.py ├── __main__.py └── module2.py
[test@ubuntu ~] sudo apt install -y python3 python3-pip git curl [test@ubuntu ~] curl -L https://github.com/MatteoGuadrini/psp/releases/download/v0.1.0/psp.deb -o psp.deb [test@ubuntu ~] sudo dpkg -i psp.deb
運行我們的包
您已經編寫了一個簡單但有組織且功能強大的包,可以用於生產和分發。
測試我們的包。
[test@ubuntu ~] psp Welcome to PSP (Python Scaffolding Projects): 0.1.0 > Name of Python project: app > Do you want to create a virtual environment? Yes > Do you want to start git repository? Yes > Select git remote provider: Gitlab > Username of Gitlab: test_user > Do you want unit test files? Yes > Install dependencies: flask > Select documention generator: MKDocs > Do you want to configure tox? Yes > Select remote CI provider: CircleCI > Do you want create common files? Yes > Select license: Gnu Public License > Do you want to install dependencies to publish on pypi? Yes > Do you want to create a Dockerfile and Containerfile? Yes Python project `app` created at app
結果是:
對包執行單元測試
現在也透過測試資料夾測試套件上的 Python 程式碼:
[test@ubuntu ~] ls -lah app total 88K drwxrwxr-x 9 test test 440 Dec 20 14:48 . drwxrwxrwt 29 root root 680 Dec 20 14:49 .. drwxrwxr-x 2 test test 60 Dec 20 14:47 .circleci drwxrwxr-x 7 test test 200 Dec 20 14:47 .git -rw-rw-r-- 1 test test 381 Dec 20 14:47 .gitignore drwxrwxr-x 4 test test 80 Dec 20 14:47 .gitlab -rw-rw-r-- 1 test test 127 Dec 20 14:48 CHANGES.md -rw-rw-r-- 1 test test 5.4K Dec 20 14:48 CODE_OF_CONDUCT.md -rw-rw-r-- 1 test test 1.1K Dec 20 14:48 CONTRIBUTING.md -rw-rw-r-- 1 test test 190 Dec 20 14:48 Containerfile -rw-rw-r-- 1 test test 190 Dec 20 14:48 Dockerfile -rw-rw-r-- 1 test test 35K Dec 20 14:48 LICENSE.md -rw-rw-r-- 1 test test 697 Dec 20 14:48 Makefile -rw-rw-r-- 1 test test 177 Dec 20 14:48 README.md drwxrwxr-x 2 test test 60 Dec 20 14:47 docs -rw-rw-r-- 1 test test 19 Dec 20 14:47 mkdocs.yml -rw-rw-r-- 1 test test 819 Dec 20 14:48 pyproject.toml -rw-rw-r-- 1 test test 66 Dec 20 14:47 requirements.txt drwxrwxr-x 2 test test 80 Dec 20 14:47 tests -rw-rw-r-- 1 test test 213 Dec 20 14:47 tox.ini drwxrwxr-x 2 test test 80 Dec 20 14:46 app drwxrwxr-x 5 test test 140 Dec 20 14:46 venv
保存我們的作品
現在您可以節省網頁應用程式的開發。
[test@ubuntu ~] cd app/ && ls -lh app/ total 8.0K -rw-rw-r-- 1 test test 162 Dec 20 14:46 __init__.py -rw-rw-r-- 1 test test 204 Dec 20 14:46 __main__.py [test@ubuntu ~] vim app/core.py
測試環境
用docker模擬你的生產環境:
from flask import Flask app = Flask(__name__) @app.route("/") def hello_world(): return "<p>Wow, this is my app!</p>"
結果是一樣的:
使用 make 建構管道
現在,下次開發後,您可以透過Makefile使用管道:
[test@ubuntu app] vim app/__main__.py
在 PyPi 上發布包
現在,如果您願意,您就可以在 PyPi 上發布 Python 套件了:
#! /usr/bin/env python3 # -*- encoding: utf-8 -*- # vim: se ts=4 et syn=python: # Generated by psp (https://github.com/MatteoGuadrini/psp) from .__init__ import __version__ print(f'app {__version__}') from .core import app app.run(debug=True)
結論
不到五分鐘,您就創建了一個 Python 項目,其中套件本身的開發是您唯一需要擔心的事情。
本文使用的工具:
psp:儲存庫 -- 文件
git: 儲存庫 -- 文件
docker: 儲存庫 -- 文件
make: 儲存庫 -- 文件
python: 儲存庫 -- 文件
以上是如何在 inutes 中建立自己的 Python 項目的詳細內容。更多資訊請關注PHP中文網其他相關文章!
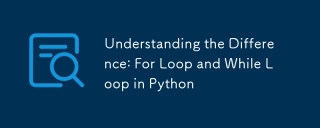
theDifferenceBetweewneaforoopandawhileLoopInpythonisthataThataThataThataThataThataThataNumberoFiterationSiskNownInAdvance,而leleawhileLoopisusedWhenaconDitionNeedneedneedneedNeedStobeCheckedStobeCheckedStobeCheckedStobeCheckedStobeceDrepeTysepectients.peatsiveSectlyStheStobeCeptellyWithnumberofiterations.1)forloopsareAceareIdealForitoringercortersence
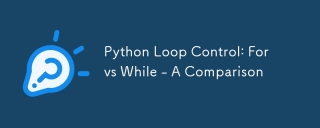
在Python中,for循環適用於已知迭代次數的情況,而while循環適合未知迭代次數且需要更多控制的情況。 1)for循環適用於遍歷序列,如列表、字符串等,代碼簡潔且Pythonic。 2)while循環在需要根據條件控制循環或等待用戶輸入時更合適,但需注意避免無限循環。 3)性能上,for循環略快,但差異通常不大。選擇合適的循環類型可以提高代碼的效率和可讀性。
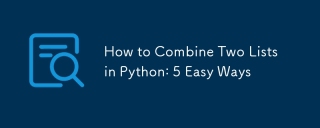
在Python中,可以通過五種方法合併列表:1)使用 運算符,簡單直觀,適用於小列表;2)使用extend()方法,直接修改原列表,適用於需要頻繁更新的列表;3)使用列表解析式,簡潔且可對元素進行操作;4)使用itertools.chain()函數,內存高效,適合大數據集;5)使用*運算符和zip()函數,適用於需要配對元素的場景。每種方法都有其特定用途和優缺點,選擇時應考慮項目需求和性能。
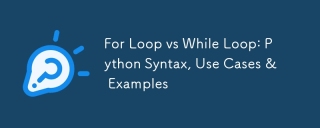
foroopsare whenthenemberofiterationsisknown,而whileLoopsareUseduntilacTitionismet.1)ForloopSareIdealForeSequencesLikeLists,UsingSyntaxLike'forfruitinFruitinFruitinFruitIts:print(fruit)'。 2)'
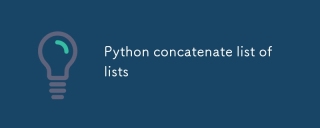
toConcateNateAlistofListsInpython,useextend,listComprehensions,itertools.Chain,orrecursiveFunctions.1)ExtendMethodStraightForwardButverBose.2)listComprechencomprechensionsareconconconciseandemandeconeandefforlargerdatasets.3)
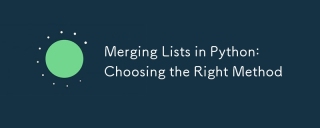
Tomergelistsinpython,YouCanusethe操作員,estextMethod,ListComprehension,Oritertools
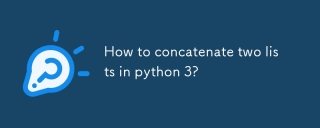
在Python3中,可以通過多種方法連接兩個列表:1)使用 運算符,適用於小列表,但對大列表效率低;2)使用extend方法,適用於大列表,內存效率高,但會修改原列表;3)使用*運算符,適用於合併多個列表,不修改原列表;4)使用itertools.chain,適用於大數據集,內存效率高。
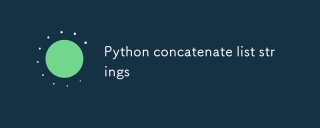
使用join()方法是Python中從列表連接字符串最有效的方法。 1)使用join()方法高效且易讀。 2)循環使用 運算符對大列表效率低。 3)列表推導式與join()結合適用於需要轉換的場景。 4)reduce()方法適用於其他類型歸約,但對字符串連接效率低。完整句子結束。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),
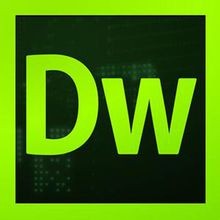
Dreamweaver CS6
視覺化網頁開發工具