最初發布:souvikinator.xyz
以下是如何為 API 端點設定單元測試。
我們將使用以下 NPM 套件:
- 伺服器的Express(可以使用任何其他框架)
- 開玩笑
- 超級測驗
VS Code 擴充使用:
- 開玩笑
- 玩笑跑者
安裝依賴項
npm install express
npm install -D jest supertest
目錄結構如下:
並且不要忘記 package.json
{ "name": "api-unit-testing", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "jest api.test.js", "start":"node server.js" }, "keywords": [], "author": "", "license": "ISC", "dependencies": { "express": "^4.18.1" }, "devDependencies": { "jest": "^28.1.2", "supertest": "^6.2.3" } }
創建一個簡單的 Express 伺服器
一個快速伺服器,在 /post 端點上接受帶有正文和標題的 POST 請求。如果其中一個缺失,則會傳回狀態 400 Bad Request。
// app.js const express = require("express"); const app = express(); app.use(express.json()); app.post("/post", (req, res) => { const { title, body } = req.body; if (!title || !body) return res.sendStatus(400).json({ error: "title and body is required" }); return res.sendStatus(200); }); module.exports = app;
const app = require("./app"); const port = 3000; app.listen(port, () => { console.log(`http://localhost:${port} ?`); });
express 應用程式已匯出以與 supertest 一起使用。使用其他 HTTP 伺服器框架也可以完成相同的操作。
建立測試文件
// api.test.js const supertest = require("supertest"); const app = require("./app"); // express app describe("Creating post", () => { // test cases goes here });
Supertest 負責運行伺服器並為我們發出請求,而我們則專注於測試。
express 應用程式被傳遞給超級測試代理,並將express 綁定到隨機可用連接埠。然後我們可以向所需的 API 端點發出 HTTP 請求,並將其回應狀態與我們的期望進行比較:
const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", });
我們將為 3 個案例建立測試:
- 當標題和正文都提供時
test("creating new post when both title and body are provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", }); // comparing response status code with expected status code expect(response.statusCode).toBe(200); });
- 當其中任何一個失蹤時
test("creating new post when either of the data is not provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", }); expect(response.statusCode).toBe(400); });
- 當他們兩個都失蹤時
test("creating new post when no data is not provided", async () => { const response = await supertest.agent(app).post("/post").send(); expect(response.statusCode).toBe(400); });
最終程式碼應如下圖所示:
const supertest = require("supertest"); const app = require("./app"); // express app describe("Creating post", () => { test("creating new post when both title and body are provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", body: "Awesome post body", }); expect(response.statusCode).toBe(200); }); test("creating new post when either of the data is not provided", async () => { const response = await supertest.agent(app).post("/post").send({ title: "Awesome post", }); expect(response.statusCode).toBe(400); }); test("creating new post when no data is not provided", async () => { const response = await supertest.agent(app).post("/post").send(); expect(response.statusCode).toBe(400); }); });
運行測試
使用以下命令執行測試:
npm run test
如果使用 VS Code 擴充功能(例如 jest 和 jest runner),那麼它會為您完成工作。
這就是我們如何輕鬆測試 API 端點的方法。 ?
以上是使用 Jest 和 Supertest 測試 REST API ✅的詳細內容。更多資訊請關注PHP中文網其他相關文章!
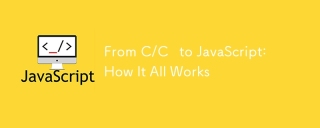
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
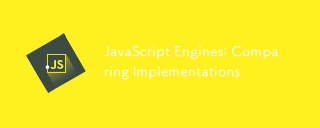
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
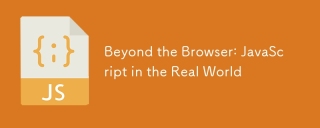
JavaScript在現實世界中的應用包括服務器端編程、移動應用開發和物聯網控制:1.通過Node.js實現服務器端編程,適用於高並發請求處理。 2.通過ReactNative進行移動應用開發,支持跨平台部署。 3.通過Johnny-Five庫用於物聯網設備控制,適用於硬件交互。
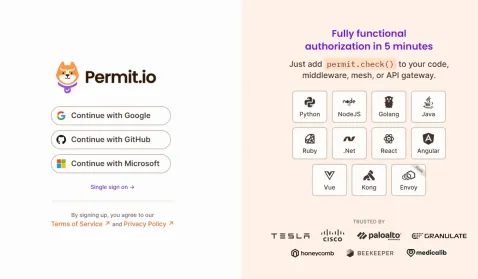
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
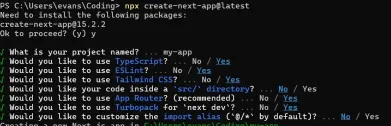
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
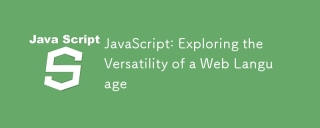
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
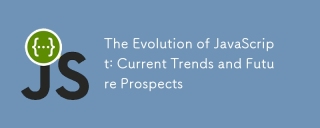
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
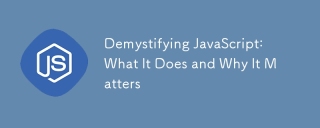
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

SublimeText3 Linux新版
SublimeText3 Linux最新版

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

SublimeText3漢化版
中文版,非常好用
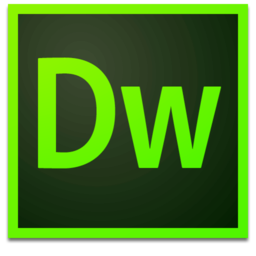
Dreamweaver Mac版
視覺化網頁開發工具

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中