如果您正在深入 React 的世界,您很可能會對其強大的功能和陡峭的學習曲線感到不知所措。相信我,我去過那裡。當我瀏覽 React 的功能和工具時,我發現了一些我希望早點知道的見解和技術。
在這篇部落格中,我將分享十個寶貴的經驗教訓,可以幫助您擺脫這些最初的陷阱,重點是整合像FAB Builder 這樣的平台,並有效地使用React 進行無縫應用程式開發。讓我們開始吧!
1.為什麼要使用 FAB Builder 在 React 中進行快速開發?
我早期的錯誤之一是花費無數時間設定樣板並維護重複的程式碼。輸入 FAB Builder - 一個消除這些低效率的平台。
使用 FAB Builder 的程式碼產生平台,您可以:
- 快速產生組件。
- 使用預製模板進行鷹架應用。
- 整合基於人工智慧的統計數據以優化工作流程。
範例:
jsx // Using the template generated by the FAB Builder import React from 'react'; import { FABButton } from 'fab-builder'; function App() { return <fabbutton label="Click Me" onclick="{()"> alert('Button Click!')} />; } export the default application; </fabbutton>
透過利用 FAB Builder 這樣的平台,您可以專注於解決業務問題而不是標準任務。
2.什麼是上下文和狀態?什麼時候應該使用它們?
最初,我過度使用了該條件,導致了不必要的重繪和效能瓶頸。理解上下文和狀態對於乾淨且可擴展的React應用程式至關重要。
- 狀態:非常適合管理單一元件中的動態資料。
- Context:最適合在沒有鑽孔支援的元件之間共用資料。
範例:
jsx // Use context for global state import React, { createContext, useContext, useState } from 'react'; const ThemeContext = createContext(); function App() { const [theme, setTheme] = useState('light'); return ( <themecontext.provider value="{{" theme settheme> <themedbutton></themedbutton> </themecontext.provider> ); } function ThemedButton() { const { theme, setTheme } = useContext(ThemeContext); return ( <button onclick="{()"> setTheme(theme === 'light' ? 'dark' : 'light')} > <h3> <strong>3. How FAB Builder Simplifies Omnichannel Marketing with React?</strong> </h3> <p>One thing I regret not taking advantage of earlier is integrating omnichannel marketing with platform like <strong>FAB Builder</strong>. This feature enables seamless communication across platforms, improving customer engagement and retention. </p> <p><strong>Such integrations are simple:</strong><br> </p> <pre class="brush:php;toolbar:false">jsx import { FABOmnichannel } from 'fab-builder'; function App() { return ( <fabomnichannel channels="{['WhatsApp'," onmessage="{(message)"> console.log(message)} /> ); } </fabomnichannel>
利用現成的組件,您可以輕鬆簡化全通路通訊。
4.優化 React 應用程式效能的最佳方法是什麼?
在我了解最佳化技術之前,效能問題一直是我的致命弱點。它在這裡工作:
- 延遲元件載入:僅在需要時使用 React.lazy() 載入元件。
- Memoization:使用 React.memo 和 useMemo 來避免不必要的渲染。
- 程式碼分割:將程式碼分割成更小的套件以加快載入速度。
範例:
jsx // Using the template generated by the FAB Builder import React from 'react'; import { FABButton } from 'fab-builder'; function App() { return <fabbutton label="Click Me" onclick="{()"> alert('Button Click!')} />; } export the default application; </fabbutton>
5. 為什麼你應該重新考慮如何在 React 中處理表單?
表單很快就會變得複雜,尤其是在沒有合適的平台的情況下。為了簡化表單的建立和管理,我建議使用FAB Builder’s Page Pilot。
FAB Builder 範例:
jsx // Use context for global state import React, { createContext, useContext, useState } from 'react'; const ThemeContext = createContext(); function App() { const [theme, setTheme] = useState('light'); return ( <themecontext.provider value="{{" theme settheme> <themedbutton></themedbutton> </themecontext.provider> ); } function ThemedButton() { const { theme, setTheme } = useContext(ThemeContext); return ( <button onclick="{()"> setTheme(theme === 'light' ? 'dark' : 'light')} > <h3> <strong>3. How FAB Builder Simplifies Omnichannel Marketing with React?</strong> </h3> <p>One thing I regret not taking advantage of earlier is integrating omnichannel marketing with platform like <strong>FAB Builder</strong>. This feature enables seamless communication across platforms, improving customer engagement and retention. </p> <p><strong>Such integrations are simple:</strong><br> </p> <pre class="brush:php;toolbar:false">jsx import { FABOmnichannel } from 'fab-builder'; function App() { return ( <fabomnichannel channels="{['WhatsApp'," onmessage="{(message)"> console.log(message)} /> ); } </fabomnichannel>
6.錯誤界線如何保護你的 React 應用程式免於崩潰?
錯誤界限是建立 React 應用程式時的救星。如果沒有它們,一個元件中的錯誤可能會導致整個應用程式崩潰。
範例:
jsx import React, { lazy, Suspense } from 'react'; const HeavyComponent = lazy(() => import('./HeavyComponent')); function App() { return ( <suspense fallback="{<div">Loading...}> <heavycomponent></heavycomponent> ); } </suspense>
7.即時分析在 React 應用程式中的作用是什麼?
即時追蹤使用者行為可以大幅提高應用程式的成功率。透過 FAB Analytics,您可以輕鬆追蹤和優化使用者旅程。
整合範例:
jsx import React from 'react'; import { FABForm, FABInput } from 'fab-builder'; function App() { return ( <fabform onsubmit="{(data)"> console.log('Form Data:', data)} field={[ { name: 'email', label: 'Email', type: 'email' }, { name: 'password', label: 'Password', type: 'password' }, ]} /> ); } </fabform>
8.為什麼應該使用命名導出而不是預設導出?
改進我的工作流程的最簡單的更改之一是切換到命名匯出。
- 預設導出很難重構。
- 命名導出提供更好的自動完成和重構支援。
範例:
jsx import React from 'react'; class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError() { return { hasError: true }; } render() { if (this.state.hasError) { return <h1 id="Something-went-wrong">Something went wrong.</h1>; } return this.props.children; } } function FaultyComponent() { throw new Error('Oops!'); } function App() { return ( <errorboundary> <faultycomponent></faultycomponent> </errorboundary> ); }
9.調試 React 應用程式最有效的方法是什麼?
React DevTools 是你最好的朋友。提供對元件、狀態和 prop 層次結構的深入了解。
- 使用Profiler選項卡來辨識效能瓶頸。
- 檢查組件以偵錯狀況問題。
10。 FAB Builder 的整合功能如何增強您的 React 專案?
整合是現代應用程式的關鍵。 FAB Builder 支持與Stripe、Zoom 和Google 等工具無縫集成床單。
範例:
jsx import { FABAnalytics } from 'fab-builder'; function App() { FABAnalytics.track('PageView', { page: 'Home' }); return <h1 id="Welcome-to-My-App">Welcome to My App</h1>; }
結論
React 是一個強大的工具,將其與 FAB Builder 等平台配對可以釋放其全部潛力。從快速開發到全通路行銷和分析,這些工具簡化了工作流程,使您能夠建立強大的應用程式。
您希望早點知道的 React 技巧是什麼?在評論中分享吧!不要忘記為您的下一個項目探索 FAB Builder——它會改變遊戲規則。 從今天開始建造更聰明、更快、更好!
以上是像專業人士一樣做出反應:我後悔不早點知道的事情的詳細內容。更多資訊請關注PHP中文網其他相關文章!
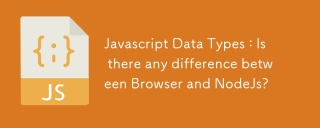
JavaScript核心數據類型在瀏覽器和Node.js中一致,但處理方式和額外類型有所不同。 1)全局對像在瀏覽器中為window,在Node.js中為global。 2)Node.js獨有Buffer對象,用於處理二進制數據。 3)性能和時間處理在兩者間也有差異,需根據環境調整代碼。
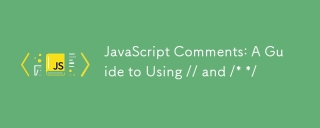
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
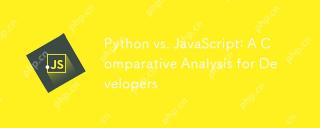
Python和JavaScript的主要區別在於類型系統和應用場景。 1.Python使用動態類型,適合科學計算和數據分析。 2.JavaScript採用弱類型,廣泛用於前端和全棧開發。兩者在異步編程和性能優化上各有優勢,選擇時應根據項目需求決定。
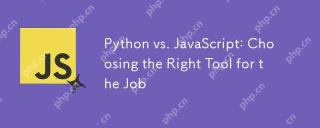
選擇Python還是JavaScript取決於項目類型:1)數據科學和自動化任務選擇Python;2)前端和全棧開發選擇JavaScript。 Python因其在數據處理和自動化方面的強大庫而備受青睞,而JavaScript則因其在網頁交互和全棧開發中的優勢而不可或缺。
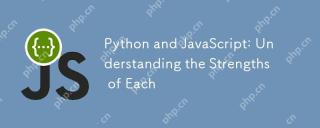
Python和JavaScript各有優勢,選擇取決於項目需求和個人偏好。 1.Python易學,語法簡潔,適用於數據科學和後端開發,但執行速度較慢。 2.JavaScript在前端開發中無處不在,異步編程能力強,Node.js使其適用於全棧開發,但語法可能複雜且易出錯。
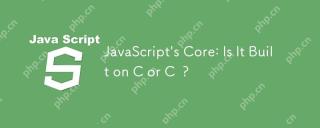
javascriptisnotbuiltoncorc; sanInterpretedlanguagethatrunsonenginesoftenwritteninc.1)JavascriptwasdesignedAsignedAsalightWeight,drackendedlanguageforwebbrowsers.2)Enginesevolvedfromsimpleterterpretpretpretpretpreterterpretpretpretpretpretpretpretpretpretcompilerers,典型地,替代品。
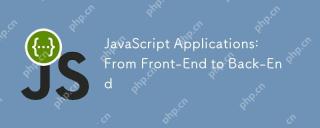
JavaScript可用於前端和後端開發。前端通過DOM操作增強用戶體驗,後端通過Node.js處理服務器任務。 1.前端示例:改變網頁文本內容。 2.後端示例:創建Node.js服務器。
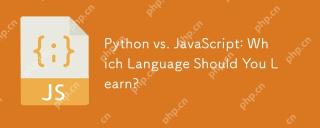
選擇Python還是JavaScript應基於職業發展、學習曲線和生態系統:1)職業發展:Python適合數據科學和後端開發,JavaScript適合前端和全棧開發。 2)學習曲線:Python語法簡潔,適合初學者;JavaScript語法靈活。 3)生態系統:Python有豐富的科學計算庫,JavaScript有強大的前端框架。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3漢化版
中文版,非常好用
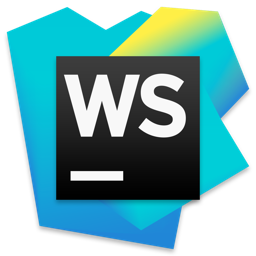
WebStorm Mac版
好用的JavaScript開發工具

禪工作室 13.0.1
強大的PHP整合開發環境

SublimeText3 Linux新版
SublimeText3 Linux最新版
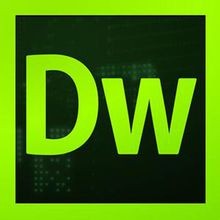
Dreamweaver CS6
視覺化網頁開發工具