JavaScript 取得 API
Fetch API 是 JavaScript 中基於承諾的現代接口,用於發出 HTTP 請求。它簡化了從伺服器取得資源的過程,取代了 XMLHttpRequest 等舊方法。 Fetch 提供了一種更清晰、更易讀的方法來處理網路請求和回應,支援 Promises、串流和非同步/等待等功能。
1. Fetch API 的主要特點
- 基於 Promise: 提供更優雅的方式來處理非同步操作。
- 簡化的語法:與 XMLHttpRequest 相比更具可讀性。
- 支援串流:有效處理大型回應。
- 可擴充: 輕鬆與現代 JavaScript 工具和函式庫整合。
2. Fetch 的基本文法
fetch(url, options) .then(response => { // Handle the response }) .catch(error => { // Handle errors });
3.發出 GET 請求
取得預設為 GET 方法。
範例:
fetch("https://jsonplaceholder.typicode.com/posts") .then(response => { if (!response.ok) { throw new Error(`HTTP error! Status: ${response.status}`); } return response.json(); }) .then(data => console.log("Data:", data)) .catch(error => console.error("Error:", error));
4.發出 POST 請求
要將資料傳送到伺服器,請使用具有選項物件中的 body 屬性的 POST 方法。
範例:
fetch("https://jsonplaceholder.typicode.com/posts", { method: "POST", headers: { "Content-Type": "application/json", }, body: JSON.stringify({ title: "foo", body: "bar", userId: 1, }), }) .then(response => response.json()) .then(data => console.log("Response:", data)) .catch(error => console.error("Error:", error));
5.常見的取得選項
fetch 函數接受一個選項物件來設定請求:
Option | Description |
---|---|
method | HTTP method (e.g., GET, POST, PUT, DELETE). |
headers | Object containing request headers. |
body | Data to send with the request (e.g., JSON, form data). |
credentials | Controls whether cookies are sent with the request (include, same-origin). |
描述
6.處理回應
Method | Description |
---|---|
response.text() | Returns response as plain text. |
response.json() | Parses the response as JSON. |
response.blob() | Returns response as a binary Blob. |
response.arrayBuffer() | Provides response as an ArrayBuffer. |
範例:取得 JSON
fetch(url, options) .then(response => { // Handle the response }) .catch(error => { // Handle errors });
7.將 Async/Await 與 Fetch 結合
Async/await 簡化了 Fetch 中 Promise 的處理。
範例:
fetch("https://jsonplaceholder.typicode.com/posts") .then(response => { if (!response.ok) { throw new Error(`HTTP error! Status: ${response.status}`); } return response.json(); }) .then(data => console.log("Data:", data)) .catch(error => console.error("Error:", error));
8.取得中的錯誤處理
與 XMLHttpRequest 不同,Fetch 不會因為 HTTP 錯誤而拒絕 Promise。您必須檢查回應的 ok 屬性或狀態代碼。
範例:
fetch("https://jsonplaceholder.typicode.com/posts", { method: "POST", headers: { "Content-Type": "application/json", }, body: JSON.stringify({ title: "foo", body: "bar", userId: 1, }), }) .then(response => response.json()) .then(data => console.log("Response:", data)) .catch(error => console.error("Error:", error));
9.超時取得
Fetch 本身不支援請求逾時。您可以使用 Promise.race() 實作逾時。
範例:
fetch("https://api.example.com/data") .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error("Error:", error));
10。比較:Fetch API 與 XMLHttpRequest
Feature | Fetch API | XMLHttpRequest |
---|---|---|
Syntax | Promise-based, simpler, cleaner. | Callback-based, verbose. |
Error Handling | Requires manual handling of HTTP errors. | Built-in HTTP error handling. |
Streaming | Supports streaming responses. | Limited streaming capabilities. |
Modern Features | Works with Promises, async/await. | No built-in Promise support. |
取得 API
11。何時使用 Fetch API
Fetch 是現代 Web 開發專案的理想選擇。
它與 Promises 和 async/await 無縫整合。
當您需要更乾淨且更易於維護的程式碼時使用它。
以上是掌握 Fetch API:在 JavaScript 中簡化 HTTP 請求的詳細內容。更多資訊請關注PHP中文網其他相關文章!
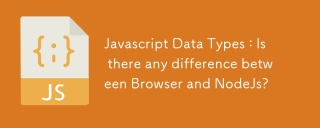
JavaScript核心數據類型在瀏覽器和Node.js中一致,但處理方式和額外類型有所不同。 1)全局對像在瀏覽器中為window,在Node.js中為global。 2)Node.js獨有Buffer對象,用於處理二進制數據。 3)性能和時間處理在兩者間也有差異,需根據環境調整代碼。
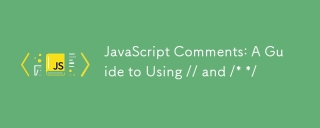
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
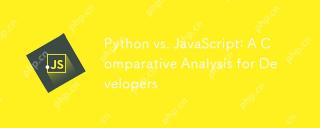
Python和JavaScript的主要區別在於類型系統和應用場景。 1.Python使用動態類型,適合科學計算和數據分析。 2.JavaScript採用弱類型,廣泛用於前端和全棧開發。兩者在異步編程和性能優化上各有優勢,選擇時應根據項目需求決定。
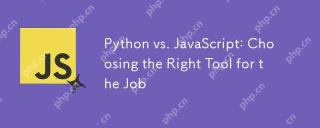
選擇Python還是JavaScript取決於項目類型:1)數據科學和自動化任務選擇Python;2)前端和全棧開發選擇JavaScript。 Python因其在數據處理和自動化方面的強大庫而備受青睞,而JavaScript則因其在網頁交互和全棧開發中的優勢而不可或缺。
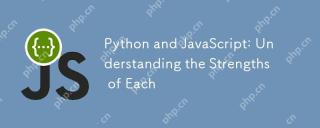
Python和JavaScript各有優勢,選擇取決於項目需求和個人偏好。 1.Python易學,語法簡潔,適用於數據科學和後端開發,但執行速度較慢。 2.JavaScript在前端開發中無處不在,異步編程能力強,Node.js使其適用於全棧開發,但語法可能複雜且易出錯。
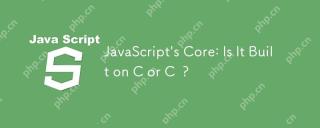
javascriptisnotbuiltoncorc; sanInterpretedlanguagethatrunsonenginesoftenwritteninc.1)JavascriptwasdesignedAsignedAsalightWeight,drackendedlanguageforwebbrowsers.2)Enginesevolvedfromsimpleterterpretpretpretpretpreterterpretpretpretpretpretpretpretpretpretcompilerers,典型地,替代品。
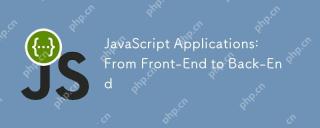
JavaScript可用於前端和後端開發。前端通過DOM操作增強用戶體驗,後端通過Node.js處理服務器任務。 1.前端示例:改變網頁文本內容。 2.後端示例:創建Node.js服務器。
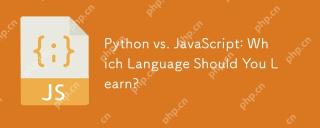
選擇Python還是JavaScript應基於職業發展、學習曲線和生態系統:1)職業發展:Python適合數據科學和後端開發,JavaScript適合前端和全棧開發。 2)學習曲線:Python語法簡潔,適合初學者;JavaScript語法靈活。 3)生態系統:Python有豐富的科學計算庫,JavaScript有強大的前端框架。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
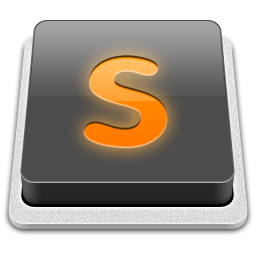
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中
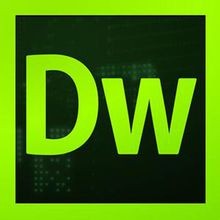
Dreamweaver CS6
視覺化網頁開發工具