在Java 中編寫和發送HTTP 請求
要利用Java 中HTTP 請求的強大功能,您可以使用java.net.HttpUrlopConnection班級。它提供了一個全面的 API,用於建立 HTTP 請求並將其分派到任何 Web 伺服器。
建立連接
要建立連接,您將建立一個 URL 物件並使用它來建立 HttpUrlConnection 連接。指定請求方法(例如 POST、GET)並設定必要的標頭,例如 Content-Type 和 Content-Length。
準備 HTTP 請求
對於POST 請求,將請求參數格式化為查詢字串(例如「name=John&age=30」)。使用 DataOutputStream 將參數寫入輸出流。
發送請求
請求準備好後,使用 DataOutputStream.writeBytes() 發送請求,以傳輸請求正文。 Web 伺服器將回應請求的資源。
接收回應
InputStream 用於從伺服器擷取回應。使用 BufferedReader 逐行讀取回應並建立最終回應正文。
範例程式碼
以下程式碼片段展示如何使用以下指令執行HTTP POST 要求上述技術:
import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.io.OutputStreamWriter; import java.io.IOException; public static void sendHttpRequest() throws IOException { // Create the connection URL url = new URL("http://example.com/"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("POST"); connection.setDoOutput(true); connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded"); // Build the request parameters String postData = URLEncoder.encode("name", "UTF-8") + "=" + URLEncoder.encode("John", "UTF-8"); // Send the request OutputStreamWriter writer = new OutputStreamWriter(connection.getOutputStream()); writer.write(postData); writer.flush(); // Get the response int responseCode = connection.getResponseCode(); if (responseCode == HttpURLConnection.HTTP_OK) { BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream())); String line; while ((line = reader.readLine()) != null) { System.out.println(line); } reader.close(); } connection.disconnect(); }
透過執行下列步驟並要求上述技術:
透過執行下列步驟並要求上述技術:透過執行下列步驟並利用HttpUrlConnection 類,您可以在您的Java 應用程式中輕鬆編寫和傳輸HTTP 請求。以上是如何在 Java 中使用「HttpUrlConnection」發送 HTTP 請求?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
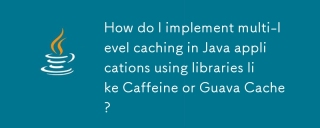
本文討論了使用咖啡因和Guava緩存在Java中實施多層緩存以提高應用程序性能。它涵蓋設置,集成和績效優勢,以及配置和驅逐政策管理最佳PRA
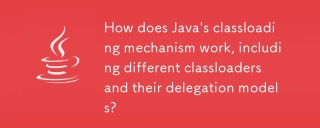
Java的類上載涉及使用帶有引導,擴展程序和應用程序類負載器的分層系統加載,鏈接和初始化類。父代授權模型確保首先加載核心類別,從而影響自定義類LOA
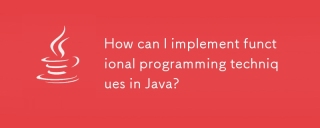
本文使用lambda表達式,流API,方法參考和可選探索將功能編程集成到Java中。 它突出顯示了通過簡潔性和不變性改善代碼可讀性和可維護性等好處

本文討論了使用JPA進行對象相關映射,並具有高級功能,例如緩存和懶惰加載。它涵蓋了設置,實體映射和優化性能的最佳實踐,同時突出潛在的陷阱。[159個字符]

本文討論了使用Maven和Gradle進行Java項目管理,構建自動化和依賴性解決方案,以比較其方法和優化策略。

本文使用選擇器和頻道使用單個線程有效地處理多個連接的Java的NIO API,用於非阻滯I/O。 它詳細介紹了過程,好處(可伸縮性,性能)和潛在的陷阱(複雜性,
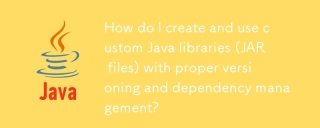
本文使用Maven和Gradle之類的工具討論了具有適當的版本控制和依賴關係管理的自定義Java庫(JAR文件)的創建和使用。
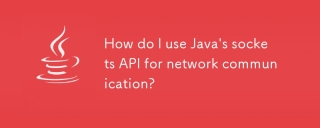
本文詳細介紹了用於網絡通信的Java的套接字API,涵蓋了客戶服務器設置,數據處理和關鍵考慮因素,例如資源管理,錯誤處理和安全性。 它還探索了性能優化技術,我


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。
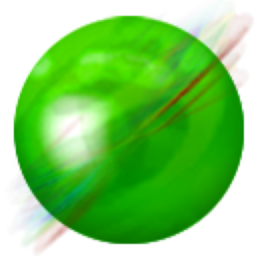
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SublimeText3漢化版
中文版,非常好用
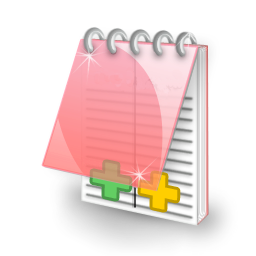
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能