在Java 中模擬Unix“tail -f”
在Java 中實現“tail -f”實用程式的功能需要找到一個有效的方法能夠監視檔案是否有新新增並允許程式連續讀取這些新行的技術或程式庫。要實現此目標,請考慮以下方法:
Apache Commons Tailer 類別
一個值得注意的選項是 Apache Commons IO 中的 Tailer 類別。此類提供了用於監視文件及其修改的簡單解決方案。它允許連續讀取添加到文件中的新行,類似於“tail -f”的行為。可以使用以下程式碼實現與Tailer 類別的整合:
Tailer tailer = Tailer.create(new File("application.log"), new TailerListener() { @Override public void handle(String line) { // Perform desired operations on the newly added line } }); tailer.run();
自訂檔案監控實作
或者,可以建立定期的自訂檔案監控實作檢查檔案大小的變化並相應地更新其內部緩衝區。這種方法需要更深入地了解 Java 中的檔案系統行為和事件偵聽機制。這是一個簡化的範例:
import java.io.File; import java.io.IOException; import java.nio.file.*; import java.nio.file.attribute.BasicFileAttributes; public class TailFileMonitor implements Runnable { private File file; private long lastFileSize; public TailFileMonitor(File file) { this.file = file; this.lastFileSize = file.length(); } @Override public void run() { Path filePath = file.toPath(); try { WatchKey watchKey = filePath.getParent().register(new WatchService(), StandardWatchEventKinds.ENTRY_MODIFY); while (true) { WatchKey key = watchKey.poll(); if (key == watchKey) { for (WatchEvent> event : key.pollEvents()) { WatchEvent.Kind> kind = event.kind(); if (kind == StandardWatchEventKinds.ENTRY_MODIFY && event.context().toString().equals(file.getName())) { tailFile(); } } } key.reset(); } } catch (IOException e) { // Handle IO exceptions } } private void tailFile() { long currentFileSize = file.length(); if (currentFileSize != lastFileSize) { try (BufferedReader br = new BufferedReader(new FileReader(file))) { br.skip(lastFileSize); String line; while ((line = br.readLine()) != null) { // Process newly added lines } lastFileSize = currentFileSize; } catch (IOException e) { // Handle IO exceptions } } } }
要使用自訂監視器,請建立一個實例並將其作為執行緒執行。它將不斷監視檔案的變更並相應地更新緩衝區。
以上是如何用Java實作Unix'tail -f”功能?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
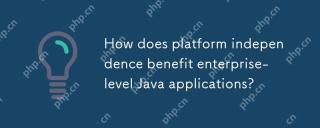
Java在企業級應用中被廣泛使用是因為其平台獨立性。 1)平台獨立性通過Java虛擬機(JVM)實現,使代碼可在任何支持Java的平台上運行。 2)它簡化了跨平台部署和開發流程,提供了更大的靈活性和擴展性。 3)然而,需注意性能差異和第三方庫兼容性,並採用最佳實踐如使用純Java代碼和跨平台測試。
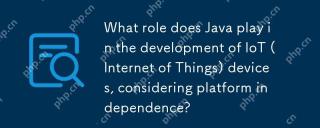
JavaplaysigantroleiniotduetoitsplatFormentence.1)itallowscodeTobewrittenOnCeandrunonVariousDevices.2)Java'secosystemprovidesuseusefidesusefidesulylibrariesforiot.3)
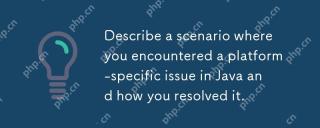
ThesolutiontohandlefilepathsacrossWindowsandLinuxinJavaistousePaths.get()fromthejava.nio.filepackage.1)UsePaths.get()withSystem.getProperty("user.dir")andtherelativepathtoconstructthefilepath.2)ConverttheresultingPathobjecttoaFileobjectifne
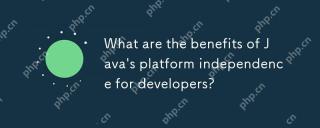
Java'splatFormIndenceistificantBecapeitAllowSitallowsDevelostWriTecoDeonCeandRunitonAnyPlatFormwithAjvm.this“ writeonce,runanywhere”(era)櫥櫃櫥櫃:1)交叉plat formcomplibility cross-platformcombiblesible,enablingDeploymentMentMentMentMentAcrAptAprospOspOspOssCrossDifferentoSswithOssuse; 2)
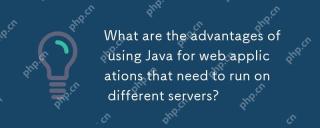
Java適合開發跨服務器web應用。 1)Java的“一次編寫,到處運行”哲學使其代碼可在任何支持JVM的平台上運行。 2)Java擁有豐富的生態系統,包括Spring和Hibernate等工具,簡化開發過程。 3)Java在性能和安全性方面表現出色,提供高效的內存管理和強大的安全保障。
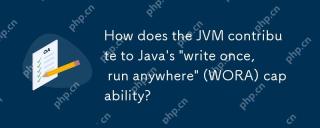
JVM通過字節碼解釋、平台無關的API和動態類加載實現Java的WORA特性:1.字節碼被解釋為機器碼,確保跨平台運行;2.標準API抽像操作系統差異;3.類在運行時動態加載,保證一致性。
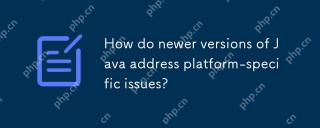
Java的最新版本通過JVM優化、標準庫改進和第三方庫支持有效解決平台特定問題。 1)JVM優化,如Java11的ZGC提升了垃圾回收性能。 2)標準庫改進,如Java9的模塊系統減少平台相關問題。 3)第三方庫提供平台優化版本,如OpenCV。
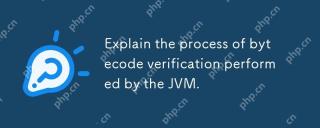
JVM的字節碼驗證過程包括四個關鍵步驟:1)檢查類文件格式是否符合規範,2)驗證字節碼指令的有效性和正確性,3)進行數據流分析確保類型安全,4)平衡驗證的徹底性與性能。通過這些步驟,JVM確保只有安全、正確的字節碼被執行,從而保護程序的完整性和安全性。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SublimeText3漢化版
中文版,非常好用

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

Atom編輯器mac版下載
最受歡迎的的開源編輯器