創建強大的軟體需要做出深思熟慮的設計選擇,以簡化程式碼維護和擴充功能。其中一個範例是在 C 應用程式中實作日誌記錄功能。日誌記錄不僅僅是列印錯誤訊息;它是關於建立一個支援調試、分析甚至跨平台相容性的結構化系統。
在本文中,我們將受現實場景的啟發,探索如何使用設計模式和最佳實踐逐步建立日誌系統。最後,您將對用 C 語言建立靈活且可擴展的日誌系統有深入的了解。
目錄
- 日誌記錄的需要
- 組織日誌檔案
- 建立中央日誌功能
- 實作軟體模組過濾器
- 新增條件日誌記錄
- 正確管理資源
- 確保線程安全
- 外部與動態配置
- 自訂日誌格式
- 內部錯誤處理
- 性能與效率
- 安全最佳實務
- 與日誌記錄工具整合
- 測試與驗證
- 跨平台文件記錄
- 把一切都包起來
- 額外
日誌記錄的需要
想像一下維護部署在遠端站點的軟體系統。每當出現問題時,您必須親自出差來調試問題。隨著部署在地理上的擴展,這種設定很快就會變得不切實際。記錄可以挽救局面。
日誌記錄提供了執行過程中關鍵點的系統內部狀態的詳細記錄。透過檢查日誌文件,開發人員可以診斷和解決問題,而無需直接重現問題。這對於難以在受控環境中重新建立的偶發錯誤特別有用。
日誌記錄的價值在多執行緒應用程式中變得更加明顯,其中錯誤可能取決於時間和競爭條件。在沒有日誌的情況下調試這些問題需要大量的努力和專門的工具,而這些工具可能並不總是可用。日誌提供了所發生事件的快照,有助於找出根本原因。
然而,日誌記錄不僅僅是一個簡單的功能——它是一個系統。實施不當的日誌記錄機制可能會導致效能問題、安全漏洞和不可維護的程式碼。因此,在設計日誌系統時,遵循結構化方法和模式至關重要。
組織記錄文件
正確的文件組織對於保持程式碼庫在成長時的可維護性至關重要。日誌記錄作為一項獨特的功能,應該隔離到自己的模組中,以便輕鬆定位和修改,而不影響程式碼的不相關部分。
頭檔(logger.h):
#ifndef LOGGER_H #define LOGGER_H #include <stdio.h> #include <time.h> // Function prototypes void log_message(const char* text); #endif // LOGGER_H </time.h></stdio.h>
實作檔案(logger.c):
#include "logger.h" void log_message(const char* text) { if (!text) { fprintf(stderr, "Invalid log message\n"); return; } time_t now = time(NULL); printf("[%s] %s\n", ctime(&now), text); }
用法(main.c):
#include "logger.h" int main() { log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); return 0; }
編譯並執行:
要編譯並執行此範例,請在終端機中使用以下命令:
gcc -o app main.c logger.c ./app
預期輸出:
[Mon Sep 27 14:00:00 2021 ] Application started [Mon Sep 27 14:00:00 2021 ] Performing operation... [Mon Sep 27 14:00:00 2021 ] Operation completed.
第一步是建立一個用於日誌記錄的專用目錄。該目錄應包含所有相關的實作檔案。例如,logger.c 可以包含日誌系統的核心邏輯,而 logger_test.c 可以保存單元測試。將相關文件放在一起可以提高開發團隊內的清晰度和協作性。
此外,日誌記錄介面應透過頭檔(例如 logger.h)公開,並放置在適當的目錄中,例如 include/ 或與原始檔案相同的目錄。這確保了需要日誌記錄功能的其他模組可以輕鬆存取它。將頭檔與實作檔分開也支援封裝,向日誌記錄 API 的使用者隱藏實作細節。
最後,對目錄和檔案採用一致的命名約定可以進一步增強可維護性。例如,使用 logger.h 和 logger.c 可以清楚地表明這些檔案屬於日誌記錄模組。避免將不相關的程式碼混合到日誌記錄模組中,因為這違背了模組化的目的。
建立中央日誌記錄功能
任何日誌系統的核心都有一個處理核心操作的核心功能:記錄日誌訊息。此功能的設計應考慮簡單性和可擴展性,以支援未來的增強功能,而無需進行重大更改。
實作(logger.c):
#include "logger.h" #include <stdio.h> #include <time.h> #include <assert.h> #define BUFFER_SIZE 256 static_assert(BUFFER_SIZE >= 64, "Buffer size is too small"); void log_message(const char* text) { char buffer[BUFFER_SIZE]; time_t now = time(NULL); if (!text) { fprintf(stderr, "Error: Null message passed to log_message\n"); return; } snprintf(buffer, BUFFER_SIZE, "[%s] %s", ctime(&now), text); printf("%s", buffer); } </assert.h></time.h></stdio.h>
注意:使用 static_assert 需要 C11 或更高版本。確保您的編譯器支援此標準。
基本的日誌記錄功能可以透過將訊息列印到標準輸出來啟動。在每個日誌條目中新增時間戳記可以透過提供時間上下文來提高其實用性。例如,日誌可以幫助識別特定錯誤何時發生或事件如何隨著時間的推移而展開。
為了保持日誌記錄模組無狀態,請避免在函數呼叫之間保留任何內部狀態。這種設計選擇簡化了實現,並確保模組在多線程環境中無縫工作。無狀態模組也更容易測試和調試,因為它們的行為不依賴先前的互動。
設計日誌記錄功能時考慮錯誤處理。例如,如果將 NULL 指標作為日誌訊息傳遞,會發生什麼情況?遵循“武士原則”,該函數應該要么優雅地處理這個問題,要么立即失敗,從而使調試更容易。
實作軟體模組過濾器
隨著應用程式變得越來越複雜,它們的日誌輸出可能會變得難以承受。如果沒有過濾器,來自不相關模組的日誌可能會淹沒控制台,從而難以關注相關資訊。實施過濾器可確保僅記錄所需的日誌。
為了實現這一點,引入一種機制來追蹤啟用的模組。這可以像全域列表一樣簡單,也可以像動態分配的雜湊表一樣複雜。此清單儲存模組名稱,並且僅處理來自這些模組的日誌。
過濾是透過在日誌記錄函數中新增模組參數來實現的。在寫入日誌之前,函數會檢查模組是否已啟用。如果不是,它會跳過日誌條目。這種方法使日誌記錄輸出簡潔並集中在感興趣的領域。這是過濾的範例實作:
頭檔(logger.h):
#ifndef LOGGER_H #define LOGGER_H #include <stdio.h> #include <time.h> // Function prototypes void log_message(const char* text); #endif // LOGGER_H </time.h></stdio.h>
實作檔案(logger.c):
#include "logger.h" void log_message(const char* text) { if (!text) { fprintf(stderr, "Invalid log message\n"); return; } time_t now = time(NULL); printf("[%s] %s\n", ctime(&now), text); }
此實作在簡單性和功能性之間取得了平衡,為特定於模組的日誌記錄提供了堅實的起點。
新增條件日誌記錄
條件日誌記錄對於建立適應不同環境或運行時條件的靈活系統至關重要。例如,在開發過程中,您可能需要詳細的偵錯日誌來追蹤應用程式行為。在生產中,您可能更願意僅記錄警告和錯誤,以最大限度地減少效能開銷。
實現此目的的一種方法是引入日誌等級。常見等級包括偵錯、資訊、警告和錯誤。日誌記錄功能可以為日誌等級新增一個附加參數,只有當日誌等級達到或超過目前閾值時才會記錄日誌。這種方法可確保過濾掉不相關的訊息,從而保持日誌簡潔且有用。
為了使其可配置,您可以使用全域變數來儲存日誌等級閾值。然後,應用程式可以動態調整此閾值,例如透過設定檔或運行時命令。
頭檔(logger.h):
#include "logger.h" int main() { log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); return 0; }
實作檔案(logger.c):
gcc -o app main.c logger.c ./app
此實作可以輕鬆控制日誌記錄的詳細程度。例如,您可以在故障排除工作階段期間將日誌等級設定為 DEBUG,並在生產中將其還原為 WARNING。
正確管理資源
適當的資源管理至關重要,尤其是在處理文件操作或多個日誌記錄目的地時。未能關閉檔案或釋放分配的記憶體可能會導致資源洩漏,隨著時間的推移會降低系統效能。
確保為記錄而開啟的任何檔案在不再需要時正確關閉。這可以透過實作初始化和關閉日誌系統的函數來實現。
實作(logger.c):
#ifndef LOGGER_H #define LOGGER_H #include <stdio.h> #include <time.h> // Function prototypes void log_message(const char* text); #endif // LOGGER_H </time.h></stdio.h>
用法(main.c):
#include "logger.h" void log_message(const char* text) { if (!text) { fprintf(stderr, "Invalid log message\n"); return; } time_t now = time(NULL); printf("[%s] %s\n", ctime(&now), text); }
編譯並執行:
#include "logger.h" int main() { log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); return 0; }
這會將日誌訊息寫入 application.log。透過提供 init_logging 和 close_logging 函數,您可以讓應用程式控制日誌記錄資源的生命週期,防止洩漏和存取問題。
確保線程安全
在多執行緒應用程式中,日誌記錄函數必須是執行緒安全的,以防止競爭條件並確保日誌訊息不會交錯或損壞。
實作執行緒安全的一種方法是使用互斥體或其他同步機制。
實作(logger.c):
gcc -o app main.c logger.c ./app
多執行緒環境中的使用(main.c):
[Mon Sep 27 14:00:00 2021 ] Application started [Mon Sep 27 14:00:00 2021 ] Performing operation... [Mon Sep 27 14:00:00 2021 ] Operation completed.
編譯並執行:
#include "logger.h" #include <stdio.h> #include <time.h> #include <assert.h> #define BUFFER_SIZE 256 static_assert(BUFFER_SIZE >= 64, "Buffer size is too small"); void log_message(const char* text) { char buffer[BUFFER_SIZE]; time_t now = time(NULL); if (!text) { fprintf(stderr, "Error: Null message passed to log_message\n"); return; } snprintf(buffer, BUFFER_SIZE, "[%s] %s", ctime(&now), text); printf("%s", buffer); } </assert.h></time.h></stdio.h>
這可以確保來自不同執行緒的日誌不會相互幹擾,從而保持日誌訊息的完整性。
外部和動態配置
允許在外部設定日誌配置增強了靈活性。日誌等級、啟用的模組和目標等配置可以從設定檔載入或透過命令列參數設定。
設定檔(config.cfg):
#ifndef LOGGER_H #define LOGGER_H #include <stdbool.h> void enable_module(const char* module); void disable_module(const char* module); void log_message(const char* module, const char* text); #endif // LOGGER_H </stdbool.h>
實作(logger.c):
#include "logger.h" #include <stdio.h> #include <string.h> #define MAX_MODULES 10 #define MODULE_NAME_LENGTH 20 static char enabled_modules[MAX_MODULES][MODULE_NAME_LENGTH]; void enable_module(const char* module) { for (int i = 0; i #ifndef LOGGER_H #define LOGGER_H typedef enum { DEBUG, INFO, WARNING, ERROR } LogLevel; void set_log_level(LogLevel level); void log_message(LogLevel level, const char* module, const char* text); #endif // LOGGER_H </string.h></stdio.h>') { strncpy(enabled_modules[i], module, MODULE_NAME_LENGTH - 1); enabled_modules[i][MODULE_NAME_LENGTH - 1] = '
#include "logger.h" #include <stdio.h> #include <time.h> #include <string.h> static LogLevel current_log_level = INFO; void set_log_level(LogLevel level) { current_log_level = level; } void log_message(LogLevel level, const char* module, const char* text) { if (level '; break; } } } void disable_module(const char* module) { for (int i = 0; i #include "logger.h" #include <stdio.h> #include <stdlib.h> static FILE* log_file = NULL; void init_logging(const char* filename) { if (filename) { log_file = fopen(filename, "a"); if (!log_file) { fprintf(stderr, "Failed to open log file: %s\n", filename); exit(EXIT_FAILURE); } } else { log_file = stdout; // Default to standard output } } void close_logging() { if (log_file && log_file != stdout) { fclose(log_file); log_file = NULL; } } void log_message(const char* text) { if (!log_file) { fprintf(stderr, "Logging not initialized.\n"); return; } time_t now = time(NULL); fprintf(log_file, "[%s] %s\n", ctime(&now), text); fflush(log_file); // Ensure the message is written immediately } </stdlib.h></stdio.h></string.h></time.h></stdio.h>'; break; } } } static int is_module_enabled(const char* module) { for (int i = 0; i
用法(main.c):
#include "logger.h" int main() { init_logging("application.log"); log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); close_logging(); return 0; }
編譯並執行:
gcc -o app main.c logger.c ./app
透過實現動態配置,您可以調整日誌記錄行為而無需重新編譯應用程序,這在生產環境中特別有用。
自訂日誌格式
自訂日誌訊息的格式可以使其資訊更豐富且更易於解析,尤其是在與日誌分析工具整合時。
實作(logger.c):
#include "logger.h" #include <pthread.h> static pthread_mutex_t log_mutex = PTHREAD_MUTEX_INITIALIZER; void log_message(const char* text) { pthread_mutex_lock(&log_mutex); // Existing logging code if (!log_file) { fprintf(stderr, "Logging not initialized.\n"); pthread_mutex_unlock(&log_mutex); return; } time_t now = time(NULL); fprintf(log_file, "[%s] %s\n", ctime(&now), text); fflush(log_file); pthread_mutex_unlock(&log_mutex); } </pthread.h>
範例輸出:
#include "logger.h" #include <pthread.h> void* thread_function(void* arg) { char* thread_name = (char*)arg; for (int i = 0; i <p>對於結構化日誌記錄,請考慮以 JSON 格式輸出日誌:<br> </p> <pre class="brush:php;toolbar:false">gcc -pthread -o app main.c logger.c ./app
這種格式適合日誌管理工具解析。
內部錯誤處理
日誌系統本身可能會遇到錯誤,例如無法開啟檔案或資源分配問題。妥善處理這些錯誤並向開發人員提供回饋非常重要。
實作(logger.c):
#ifndef LOGGER_H #define LOGGER_H #include <stdio.h> #include <time.h> // Function prototypes void log_message(const char* text); #endif // LOGGER_H </time.h></stdio.h>
透過在使用前檢查資源狀態並提供有意義的錯誤訊息,您可以防止崩潰並幫助排除日誌系統本身的問題。
性能和效率
日誌記錄會影響應用程式效能,尤其是在日誌記錄範圍廣泛或同步執行的情況下。為了緩解這種情況,請考慮緩衝日誌或非同步執行日誌記錄操作等技術。
非同步日誌實作(logger.c):
#include "logger.h" void log_message(const char* text) { if (!text) { fprintf(stderr, "Invalid log message\n"); return; } time_t now = time(NULL); printf("[%s] %s\n", ctime(&now), text); }
用法(main.c):
#include "logger.h" int main() { log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); return 0; }
使用非同步日誌記錄可以減少主應用程式執行緒在日誌記錄上花費的時間,從而提高整體效能。
安全最佳實踐
日誌可能會無意中暴露敏感訊息,例如密碼或個人資料。避免記錄此類資訊並保護日誌檔案免遭未經授權的存取至關重要。
實作(logger.c):
gcc -o app main.c logger.c ./app
設定檔權限:
[Mon Sep 27 14:00:00 2021 ] Application started [Mon Sep 27 14:00:00 2021 ] Performing operation... [Mon Sep 27 14:00:00 2021 ] Operation completed.
推薦:
- 清理輸入:確保日誌訊息中不包含敏感資料。
- 存取控制:對日誌檔案設定適當的權限以限制存取。
- 加密:如果日誌檔案包含敏感訊息,請考慮對其進行加密。
- 日誌輪替:實作日誌輪替以防止日誌無限增長並管理暴露。
遵循這些做法,您可以增強應用程式的安全性並遵守資料保護法規。
與日誌記錄工具集成
現代應用程式通常與外部日誌記錄工具和服務集成,以實現更好的日誌管理和分析。
系統日誌整合(logger.c):
#include "logger.h" #include <stdio.h> #include <time.h> #include <assert.h> #define BUFFER_SIZE 256 static_assert(BUFFER_SIZE >= 64, "Buffer size is too small"); void log_message(const char* text) { char buffer[BUFFER_SIZE]; time_t now = time(NULL); if (!text) { fprintf(stderr, "Error: Null message passed to log_message\n"); return; } snprintf(buffer, BUFFER_SIZE, "[%s] %s", ctime(&now), text); printf("%s", buffer); } </assert.h></time.h></stdio.h>
用法(main.c):
#ifndef LOGGER_H #define LOGGER_H #include <stdbool.h> void enable_module(const char* module); void disable_module(const char* module); void log_message(const char* module, const char* text); #endif // LOGGER_H </stdbool.h>
遠端記錄服務:
要將日誌傳送到 Graylog 或 Elasticsearch 等遠端服務,您可以使用網路套接字或專用函式庫。
使用套接字的範例(logger.c):
#include "logger.h" #include <stdio.h> #include <string.h> #define MAX_MODULES 10 #define MODULE_NAME_LENGTH 20 static char enabled_modules[MAX_MODULES][MODULE_NAME_LENGTH]; void enable_module(const char* module) { for (int i = 0; i #ifndef LOGGER_H #define LOGGER_H typedef enum { DEBUG, INFO, WARNING, ERROR } LogLevel; void set_log_level(LogLevel level); void log_message(LogLevel level, const char* module, const char* text); #endif // LOGGER_H </string.h></stdio.h>') { strncpy(enabled_modules[i], module, MODULE_NAME_LENGTH - 1); enabled_modules[i][MODULE_NAME_LENGTH - 1] = '
#include "logger.h" #include <stdio.h> #include <time.h> #include <string.h> static LogLevel current_log_level = INFO; void set_log_level(LogLevel level) { current_log_level = level; } void log_message(LogLevel level, const char* module, const char* text) { if (level '; break; } } } void disable_module(const char* module) { for (int i = 0; i #include "logger.h" #include <stdio.h> #include <stdlib.h> static FILE* log_file = NULL; void init_logging(const char* filename) { if (filename) { log_file = fopen(filename, "a"); if (!log_file) { fprintf(stderr, "Failed to open log file: %s\n", filename); exit(EXIT_FAILURE); } } else { log_file = stdout; // Default to standard output } } void close_logging() { if (log_file && log_file != stdout) { fclose(log_file); log_file = NULL; } } void log_message(const char* text) { if (!log_file) { fprintf(stderr, "Logging not initialized.\n"); return; } time_t now = time(NULL); fprintf(log_file, "[%s] %s\n", ctime(&now), text); fflush(log_file); // Ensure the message is written immediately } </stdlib.h></stdio.h></string.h></time.h></stdio.h>'; break; } } } static int is_module_enabled(const char* module) { for (int i = 0; i
用法(main.c):
#include "logger.h" int main() { init_logging("application.log"); log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); close_logging(); return 0; }
與外部工具整合可以提供集中日誌管理、即時監控和警報等進階功能。
測試和驗證
徹底的測試確保日誌系統在各種條件下都能正常運作。
單元測試範例(test_logger.c):
gcc -o app main.c logger.c ./app
編譯並執行測試:
#include "logger.h" #include <pthread.h> static pthread_mutex_t log_mutex = PTHREAD_MUTEX_INITIALIZER; void log_message(const char* text) { pthread_mutex_lock(&log_mutex); // Existing logging code if (!log_file) { fprintf(stderr, "Logging not initialized.\n"); pthread_mutex_unlock(&log_mutex); return; } time_t now = time(NULL); fprintf(log_file, "[%s] %s\n", ctime(&now), text); fflush(log_file); pthread_mutex_unlock(&log_mutex); } </pthread.h>
檢定策略:
- 單元檢定:驗證各個函數。
- 壓力測試:類比高頻日誌記錄。
- 多執行緒測試: 同時從多個執行緒記錄。
- 故障注入:模擬磁碟已滿或網路故障等錯誤。
透過嚴格測試日誌系統,您可以在問題影響生產環境之前識別並修復問題。
跨平台文件記錄
跨平台相容性是現代軟體的必要條件。雖然前面的範例在基於 Unix 的系統上運作良好,但由於檔案處理 API 的差異,它們可能無法在 Windows 上運作。為了解決這個問題,您需要一個跨平台的日誌機制。
實作(logger.c):
#ifndef LOGGER_H #define LOGGER_H #include <stdio.h> #include <time.h> // Function prototypes void log_message(const char* text); #endif // LOGGER_H </time.h></stdio.h>
用法(logger.c):
#include "logger.h" void log_message(const char* text) { if (!text) { fprintf(stderr, "Invalid log message\n"); return; } time_t now = time(NULL); printf("[%s] %s\n", ctime(&now), text); }
透過隔離特定於平台的詳細信息,您可以確保主要日誌記錄邏輯保持乾淨且一致。
總結一切
設計日誌系統乍看之下似乎是一項簡單的任務,但正如我們所見,它涉及許多影響功能、效能和可維護性的決策。透過使用設計模式和結構化方法,您可以建立一個健全、可擴展且易於整合的日誌系統。
從組織文件到實現跨平台相容性,每一步都建立在前一步的基礎上,形成一個有凝聚力的整體。系統可以按模組過濾日誌,透過日誌等級調整詳細程度,支援多個目的地,並正確處理資源。它確保線程安全,允許外部配置,支援自訂格式,並遵守安全最佳實踐。
透過採用無狀態設計、動態介面和抽象層等模式,您可以避免常見的陷阱並使您的程式碼庫面向未來。無論您是在開發小型實用程式還是大型應用程序,這些原則都是非常寶貴的。
您在建立精心設計的日誌系統方面投入的精力會得到回報,減少調試時間,更好地洞察應用程式行為,並提高利害關係人的滿意度。有了這個基礎,您現在就可以處理最複雜專案的日誌記錄需求。
額外:增強日誌系統
在這個額外的部分中,我們將解決先前確定的一些需要改進的領域,以增強我們建立的日誌系統。我們將專注於改進程式碼一致性、改進錯誤處理、闡明複雜概念以及擴展測試和驗證。每個主題都包含介紹文字、可編譯的實際範例以及供進一步學習的外部參考資料。
1. 程式碼一致性和格式
一致的程式碼格式和命名約定提高了可讀性和可維護性。我們將使用 Snake_case 標準化變數和函數名稱,這在 C 程式設計中很常見。
更新的實作 (logger.h):
#ifndef LOGGER_H #define LOGGER_H #include <stdio.h> #include <time.h> // Function prototypes void log_message(const char* text); #endif // LOGGER_H </time.h></stdio.h>
更新的實作 (logger.c):
#include "logger.h" void log_message(const char* text) { if (!text) { fprintf(stderr, "Invalid log message\n"); return; } time_t now = time(NULL); printf("[%s] %s\n", ctime(&now), text); }
更新用法(main.c):
#include "logger.h" int main() { log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); return 0; }
編譯並執行:
gcc -o app main.c logger.c ./app
外部參考:
- GNU 編碼標準:命名約定
- Linux 核心編碼風格
2. 改進的錯誤處理
強大的錯誤處理功能確保應用程式能夠優雅地處理意外情況。
增強的錯誤檢查(logger.c):
[Mon Sep 27 14:00:00 2021 ] Application started [Mon Sep 27 14:00:00 2021 ] Performing operation... [Mon Sep 27 14:00:00 2021 ] Operation completed.
外部參考:
- C 中的錯誤處理
- C 語言的斷言
3. 澄清非同步日誌記錄
非同步日誌記錄透過將日誌記錄流程與主應用程式流程分開來提高效能。這裡有一個實際例子的詳細解釋。
實作(logger.c):
#include "logger.h" #include <stdio.h> #include <time.h> #include <assert.h> #define BUFFER_SIZE 256 static_assert(BUFFER_SIZE >= 64, "Buffer size is too small"); void log_message(const char* text) { char buffer[BUFFER_SIZE]; time_t now = time(NULL); if (!text) { fprintf(stderr, "Error: Null message passed to log_message\n"); return; } snprintf(buffer, BUFFER_SIZE, "[%s] %s", ctime(&now), text); printf("%s", buffer); } </assert.h></time.h></stdio.h>
用法(main.c):
#ifndef LOGGER_H #define LOGGER_H #include <stdbool.h> void enable_module(const char* module); void disable_module(const char* module); void log_message(const char* module, const char* text); #endif // LOGGER_H </stdbool.h>
編譯並執行:
#include "logger.h" #include <stdio.h> #include <string.h> #define MAX_MODULES 10 #define MODULE_NAME_LENGTH 20 static char enabled_modules[MAX_MODULES][MODULE_NAME_LENGTH]; void enable_module(const char* module) { for (int i = 0; i #ifndef LOGGER_H #define LOGGER_H typedef enum { DEBUG, INFO, WARNING, ERROR } LogLevel; void set_log_level(LogLevel level); void log_message(LogLevel level, const char* module, const char* text); #endif // LOGGER_H </string.h></stdio.h>') { strncpy(enabled_modules[i], module, MODULE_NAME_LENGTH - 1); enabled_modules[i][MODULE_NAME_LENGTH - 1] = '
#include "logger.h" #include <stdio.h> #include <time.h> #include <string.h> static LogLevel current_log_level = INFO; void set_log_level(LogLevel level) { current_log_level = level; } void log_message(LogLevel level, const char* module, const char* text) { if (level '; break; } } } void disable_module(const char* module) { for (int i = 0; i #include "logger.h" #include <stdio.h> #include <stdlib.h> static FILE* log_file = NULL; void init_logging(const char* filename) { if (filename) { log_file = fopen(filename, "a"); if (!log_file) { fprintf(stderr, "Failed to open log file: %s\n", filename); exit(EXIT_FAILURE); } } else { log_file = stdout; // Default to standard output } } void close_logging() { if (log_file && log_file != stdout) { fclose(log_file); log_file = NULL; } } void log_message(const char* text) { if (!log_file) { fprintf(stderr, "Logging not initialized.\n"); return; } time_t now = time(NULL); fprintf(log_file, "[%s] %s\n", ctime(&now), text); fflush(log_file); // Ensure the message is written immediately } </stdlib.h></stdio.h></string.h></time.h></stdio.h>'; break; } } } static int is_module_enabled(const char* module) { for (int i = 0; i
說明:
- 生產者-消費者模型:主執行緒產生日誌訊息並將它們加入佇列。日誌工作執行緒消耗佇列中的消息並將其寫入日誌檔案。
- 執行緒同步:互斥體和條件變數確保對共享資源的執行緒安全存取。
- 正常關閉:logging_active 標誌和條件變數會在日誌記錄關閉時通知工作執行緒退出。
外部參考:
- 生產者-消費者問題
- POSIX 執行緒程式設計
4. 擴大測試和驗證
測試對於確保日誌系統在各種條件下正常運作至關重要。
使用 Unity 測試框架:
Unity 是一個輕量級的 C 測試框架。
設定:
- 從官方儲存庫下載Unity:GitHub 上的Unity
- 在您的測試文件中包含 unity.h。
測試檔(test_logger.c):
#include "logger.h" int main() { init_logging("application.log"); log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); close_logging(); return 0; }
編譯並執行測試:
gcc -o app main.c logger.c ./app
說明:
- setUp 和tearDown: 在每次設定和清理測試之前和之後運行的函數。
- 斷言: 使用 TEST_ASSERT_* 巨集來驗證條件。
- 測試案例:測試涵蓋記錄到標準輸出和檔案。
外部參考:
- 統一測試框架
- C 語言的單元測驗
5. 安全增強
確保日誌系統的安全至關重要,尤其是在處理敏感資料時。
使用 TLS 安全傳輸:
要透過網路安全地傳送日誌,請使用 TLS 加密。
使用 OpenSSL 實作(logger.c):
#ifndef LOGGER_H #define LOGGER_H #include <stdio.h> #include <time.h> // Function prototypes void log_message(const char* text); #endif // LOGGER_H </time.h></stdio.h>
外部參考:
- OpenSSL 文件
- 使用 OpenSSL 進行安全程式設計
遵守資料保護法規:
記錄個人資料時,確保遵守 GDPR 等法規。
推薦:
- 匿名化:刪除或掩蓋日誌中的個人識別碼。
- 存取控制:限制對日誌檔案的存取。
- 資料保留策略:定義日誌的儲存時間。
外部參考:
- 歐盟 GDPR 合規性
- HIPAA 安全規則
6.利用現有的日誌庫
有時,使用完善的日誌庫可以節省時間並提供額外的功能。
zlog簡介:
zlog 是一個可靠、執行緒安全且高度可設定的 C 日誌庫。
特徵:
- 透過檔案進行設定。
- 支援多種日誌類別和等級。
- 非同步日誌記錄功能。
使用範例:
- 安裝:
#include "logger.h" void log_message(const char* text) { if (!text) { fprintf(stderr, "Invalid log message\n"); return; } time_t now = time(NULL); printf("[%s] %s\n", ctime(&now), text); }
- 設定檔(zlog.conf):
#include "logger.h" int main() { log_message("Application started"); log_message("Performing operation..."); log_message("Operation completed."); return 0; }
- 實作(main.c):
gcc -o app main.c logger.c ./app
- 編譯並執行:
[Mon Sep 27 14:00:00 2021 ] Application started [Mon Sep 27 14:00:00 2021 ] Performing operation... [Mon Sep 27 14:00:00 2021 ] Operation completed.
外部參考:
- zlog官方網站
- log4c 項目
與自訂實作的比較:
-
使用圖書館的優點:
- 節省開發時間。
- 提供進階功能。
- 經過充分測試和維護。
-
缺點:
- 可能包含不必要的功能。
- 新增外部相依性。
- 對內部運作的控制較少。
7. 加強結論
最後,讓我們強化關鍵要點並鼓勵進一步探索。
最後的想法:
建立強大的日誌系統是軟體開發的關鍵面向。透過專注於程式碼一致性、錯誤處理、清晰度、測試、安全性並在適當的時候利用現有工具,您可以為增強應用程式的可維護性和可靠性奠定基礎。
號召性用語:
- 應用概念:將這些增強功能整合到您的專案中。
- 進一步探索: 研究更高級的日誌記錄功能,例如日誌輪換、過濾和分析工具。
- 保持更新:隨時了解日誌記錄和軟體開發方面的最佳實踐和新興技術。
其他資源:
- 日誌記錄的藝術
以上是用 C 建立健壯的日誌系統的詳細內容。更多資訊請關注PHP中文網其他相關文章!
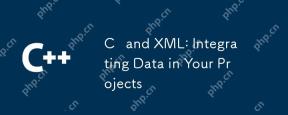
在C 項目中集成XML可以通過以下步驟實現:1)使用pugixml或TinyXML庫解析和生成XML文件,2)選擇DOM或SAX方法進行解析,3)處理嵌套節點和多級屬性,4)使用調試技巧和最佳實踐優化性能。
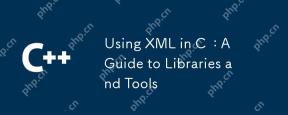
在C 中使用XML是因為它提供了結構化數據的便捷方式,尤其在配置文件、數據存儲和網絡通信中不可或缺。 1)選擇合適的庫,如TinyXML、pugixml、RapidXML,根據項目需求決定。 2)了解XML解析和生成的兩種方式:DOM適合頻繁訪問和修改,SAX適用於大文件或流數據。 3)優化性能時,TinyXML適合小文件,pugixml在內存和速度上表現好,RapidXML處理大文件優異。
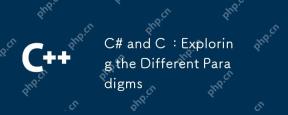
C#和C 的主要區別在於內存管理、多態性實現和性能優化。 1)C#使用垃圾回收器自動管理內存,C 則需要手動管理。 2)C#通過接口和虛方法實現多態性,C 使用虛函數和純虛函數。 3)C#的性能優化依賴於結構體和並行編程,C 則通過內聯函數和多線程實現。
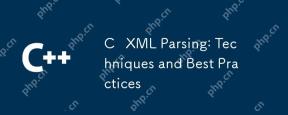
C 中解析XML數據可以使用DOM和SAX方法。 1)DOM解析將XML加載到內存,適合小文件,但可能佔用大量內存。 2)SAX解析基於事件驅動,適用於大文件,但無法隨機訪問。選擇合適的方法並優化代碼可提高效率。
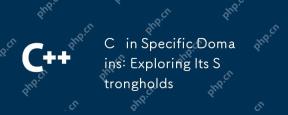
C 在遊戲開發、嵌入式系統、金融交易和科學計算等領域中的應用廣泛,原因在於其高性能和靈活性。 1)在遊戲開發中,C 用於高效圖形渲染和實時計算。 2)嵌入式系統中,C 的內存管理和硬件控制能力使其成為首選。 3)金融交易領域,C 的高性能滿足實時計算需求。 4)科學計算中,C 的高效算法實現和數據處理能力得到充分體現。
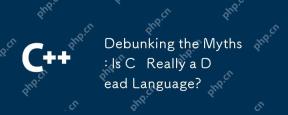
C 沒有死,反而在許多關鍵領域蓬勃發展:1)遊戲開發,2)系統編程,3)高性能計算,4)瀏覽器和網絡應用,C 依然是主流選擇,展現了其強大的生命力和應用場景。
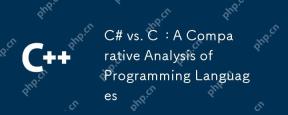
C#和C 的主要區別在於語法、內存管理和性能:1)C#語法現代,支持lambda和LINQ,C 保留C特性並支持模板。 2)C#自動內存管理,C 需要手動管理。 3)C 性能優於C#,但C#性能也在優化中。
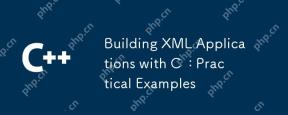
在C 中處理XML數據可以使用TinyXML、Pugixml或libxml2庫。 1)解析XML文件:使用DOM或SAX方法,DOM適合小文件,SAX適合大文件。 2)生成XML文件:將數據結構轉換為XML格式並寫入文件。通過這些步驟,可以有效地管理和操作XML數據。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

SublimeText3 Linux新版
SublimeText3 Linux最新版
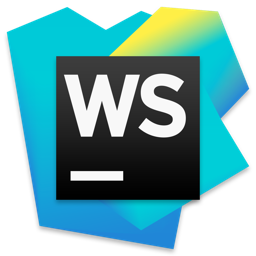
WebStorm Mac版
好用的JavaScript開發工具