Python 的簡單性使開發人員能夠快速編寫函數式程序,但先進的技術可以使您的程式碼更有效率、可維護和優雅。這些高級技巧和範例將把您的 Python 技能提升到一個新的水平。
1. 利用生成器提高記憶體效率
處理大型資料集時,使用生成器而不是列表來節省記憶體:
# List consumes memory upfront numbers = [i**2 for i in range(1_000_000)] # Generator evaluates lazily numbers = (i**2 for i in range(1_000_000)) # Iterate over the generator for num in numbers: print(num) # Processes one item at a time
原因:產生器即時建立項目,避免了將整個序列儲存在記憶體中的需要。
2. 使用資料類來簡化類
對於主要儲存資料的類,資料類減少了樣板代碼:
from dataclasses import dataclass @dataclass class Employee: name: str age: int position: str # Instead of defining __init__, __repr__, etc. emp = Employee(name="Alice", age=30, position="Engineer") print(emp) # Employee(name='Alice', age=30, position='Engineer')
為什麼:資料類別自動處理 __init__ 、 __repr__ 和其他方法。
3.掌握上下文管理器(附語句)
自訂上下文管理器簡化資源管理:
from contextlib import contextmanager @contextmanager def open_file(file_name, mode): file = open(file_name, mode) try: yield file finally: file.close() # Usage with open_file("example.txt", "w") as f: f.write("Hello, world!")
原因:即使發生異常,上下文管理器也能確保正確的清理(例如,關閉檔案)。
4。利用函數註解
註釋提高了清晰度並支持靜態分析:
def calculate_area(length: float, width: float) -> float: return length * width # IDEs and tools like MyPy can validate these annotations area = calculate_area(5.0, 3.2)
原因:註解使程式碼自我記錄並幫助在開發過程中捕獲類型錯誤。
5.應用裝飾器以實現程式碼重複使用
裝飾器在不改變原始功能的情況下擴展或修改功能:
def log_execution(func): def wrapper(*args, **kwargs): print(f"Executing {func.__name__} with {args}, {kwargs}") return func(*args, **kwargs) return wrapper @log_execution def add(a, b): return a + b result = add(3, 5) # Output: Executing add with (3, 5), {}
原因:裝飾器減少了日誌記錄、身份驗證或計時功能等任務的重複。
6. 使用 functools 實現高階功能
functools 模組簡化了複雜的函數行為:
from functools import lru_cache @lru_cache(maxsize=100) def fibonacci(n): if n <p><strong>原因:</strong>像 lru_cache 這樣的函數透過記住昂貴的函數呼叫的結果來最佳化效能。 </p> <hr> <h2> 7.了解收藏的力量 </h2> <p>集合模組提供高階資料結構:<br> </p> <pre class="brush:php;toolbar:false">from collections import defaultdict, Counter # defaultdict with default value word_count = defaultdict(int) for word in ["apple", "banana", "apple"]: word_count[word] += 1 print(word_count) # {'apple': 2, 'banana': 1} # Counter for frequency counting freq = Counter(["apple", "banana", "apple"]) print(freq.most_common(1)) # [('apple', 2)]
原因:defaultdict 和 Counter 簡化了計算出現次數等任務。
8.使用concurrent.futures進行並行化
對於 CPU 密集或 IO 密集型任務,並行執行可加快處理速度:
from concurrent.futures import ThreadPoolExecutor def square(n): return n * n with ThreadPoolExecutor(max_workers=4) as executor: results = executor.map(square, range(10)) print(list(results)) # [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
為什麼:並發.futures 讓多執行緒和多處理變得更容易。
9。使用pathlib進行檔案操作
pathlib 模組提供了一種直覺且強大的方法來處理檔案路徑:
from pathlib import Path path = Path("example.txt") # Write to a file path.write_text("Hello, pathlib!") # Read from a file content = path.read_text() print(content) # Check if a file exists if path.exists(): print("File exists")
原因:與 os 和 os.path 相比,pathlib 更具可讀性和通用性。
10. 使用模擬編寫單元測試
透過模擬依賴關係來測試複雜系統:
# List consumes memory upfront numbers = [i**2 for i in range(1_000_000)] # Generator evaluates lazily numbers = (i**2 for i in range(1_000_000)) # Iterate over the generator for num in numbers: print(num) # Processes one item at a time
原因: 模擬隔離了測試中的程式碼,確保外部相依性不會幹擾您的測試。
結論
掌握這些先進技術將提升您的 Python 編碼技能。將它們合併到您的工作流程中,編寫的程式碼不僅實用,而且高效、可維護且具有 Python 風格。快樂編碼!
以上是改進 Python 程式碼的高階技巧的詳細內容。更多資訊請關注PHP中文網其他相關文章!
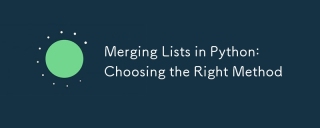
Tomergelistsinpython,YouCanusethe操作員,estextMethod,ListComprehension,Oritertools
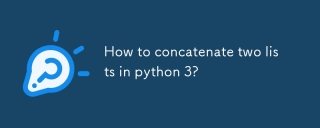
在Python3中,可以通過多種方法連接兩個列表:1)使用 運算符,適用於小列表,但對大列表效率低;2)使用extend方法,適用於大列表,內存效率高,但會修改原列表;3)使用*運算符,適用於合併多個列表,不修改原列表;4)使用itertools.chain,適用於大數據集,內存效率高。
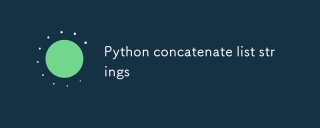
使用join()方法是Python中從列表連接字符串最有效的方法。 1)使用join()方法高效且易讀。 2)循環使用 運算符對大列表效率低。 3)列表推導式與join()結合適用於需要轉換的場景。 4)reduce()方法適用於其他類型歸約,但對字符串連接效率低。完整句子結束。
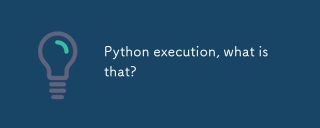
pythonexecutionistheprocessoftransformingpypythoncodeintoExecutablestructions.1)InternterPreterReadSthecode,ConvertingTingitIntObyTecode,whepythonvirtualmachine(pvm)theglobalinterpreterpreterpreterpreterlock(gil)the thepythonvirtualmachine(pvm)
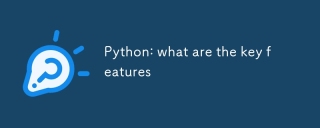
Python的關鍵特性包括:1.語法簡潔易懂,適合初學者;2.動態類型系統,提高開發速度;3.豐富的標準庫,支持多種任務;4.強大的社區和生態系統,提供廣泛支持;5.解釋性,適合腳本和快速原型開發;6.多範式支持,適用於各種編程風格。
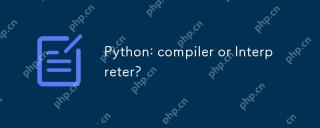
Python是解釋型語言,但也包含編譯過程。 1)Python代碼先編譯成字節碼。 2)字節碼由Python虛擬機解釋執行。 3)這種混合機制使Python既靈活又高效,但執行速度不如完全編譯型語言。
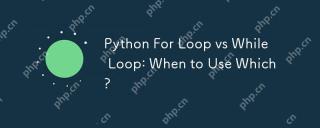
UseeAforloopWheniteratingOveraseQuenceOrforAspecificnumberoftimes; useAwhiLeLoopWhenconTinuingUntilAcIntiment.forloopsareIdealForkNownsences,而WhileLeleLeleLeleLeleLoopSituationSituationsItuationsItuationSuationSituationswithUndEtermentersitations。
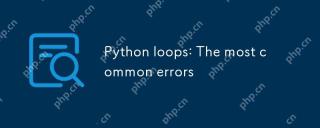
pythonloopscanleadtoerrorslikeinfiniteloops,modifyingListsDuringteritation,逐個偏置,零indexingissues,andnestedloopineflinefficiencies


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

SAP NetWeaver Server Adapter for Eclipse
將Eclipse與SAP NetWeaver應用伺服器整合。

SublimeText3 英文版
推薦:為Win版本,支援程式碼提示!

SecLists
SecLists是最終安全測試人員的伙伴。它是一個包含各種類型清單的集合,這些清單在安全評估過程中經常使用,而且都在一個地方。 SecLists透過方便地提供安全測試人員可能需要的所有列表,幫助提高安全測試的效率和生產力。清單類型包括使用者名稱、密碼、URL、模糊測試有效載荷、敏感資料模式、Web shell等等。測試人員只需將此儲存庫拉到新的測試機上,他就可以存取所需的每種類型的清單。
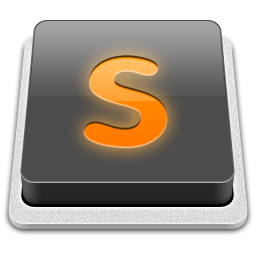
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。