Express.js 是一種在 Node.js 中建立 Web 應用程式的流行框架,但即使是經驗豐富的開發人員也會遇到難以調試的錯誤。本指南將涵蓋一些最常見的 Express.js 錯誤,解釋它們發生的原因,並提供實用的修復措施以使您的應用程式重回正軌。
1. 錯誤:發送後無法設定標頭
當您嘗試為相同請求傳送多個回應時,通常會發生此錯誤。例如,您可能會不小心在路由處理程序中多次呼叫 res.send() 或 res.json()。
範例:
app.get('/example', (req, res) => { res.send('First response'); res.send('Second response'); // This will throw the error. });
修正:
確保每個請求僅發送一個回應。使用條件邏輯或返回語句來防止發送回應後進一步執行。
app.get('/example', (req, res) => { if (!req.query.param) { return res.status(400).send('Bad Request'); } res.send('Valid Request'); });
2. 錯誤:中間件未執行
當中間件未正確連結或其中未呼叫 next() 時,就會發生這種情況。中介軟體必須明確地將控制權傳遞給下一個中介軟體或路由處理程序。
範例:
app.use((req, res) => { console.log('Middleware executed'); // Forgot to call next() }); app.get('/test', (req, res) => { res.send('Hello, World!'); });
修正:
除非中間件結束回應,否則呼叫 next()。
app.use((req, res, next) => { console.log('Middleware executed'); next(); // Pass control to the next middleware or route });
3. 錯誤:req.body未定義
如果 req.body 未定義,很可能是因為您忘記使用 body 解析中間件,例如express.json() 或express.urlencoded()。
範例:
app.post('/submit', (req, res) => { console.log(req.body); // undefined });
修正:
在應用程式初始化中包含主體解析中間件。
app.use(express.json()); app.use(express.urlencoded({ extended: true })); app.post('/submit', (req, res) => { console.log(req.body); // Now it works res.send('Data received'); });
4. 錯誤:找不到路由 (404)
當沒有路由與傳入請求相符時,會發生此錯誤。預設情況下,Express 不提供 404 處理程序。
修正:
在路由定義末尾新增一個包羅萬象的中間件來處理 404 錯誤。
app.use((req, res) => { res.status(404).send('Page Not Found'); });
5. 錯誤:EADDRINUSE(連接埠正在使用)
當另一個程序已經在使用您的應用程式嘗試綁定的連接埠時,就會發生這種情況。
Error: listen EADDRINUSE: address already in use :::3000
修正:
尋找並終止衝突進程或使用不同的連接埠。您也可以透過程式處理錯誤:
const port = process.env.PORT || 3000; app.listen(port, () => { console.log(`Server running on port ${port}`); }).on('error', (err) => { if (err.code === 'EADDRINUSE') { console.error(`Port ${port} is already in use. Please use a different port.`); } });
Express.js 錯誤可能會令人沮喪,但了解其根本原因可以更輕鬆地解決它們。透過這些常見的修復,您將能夠更好地調試應用程式並保持專案順利運行。
如果您發現本指南有幫助,請點擊 ❤️ 圖示並關注我以獲取更多 JavaScript 提示和技巧!
以上是Express.js 的主要錯誤以及如何修復它們的詳細內容。更多資訊請關注PHP中文網其他相關文章!
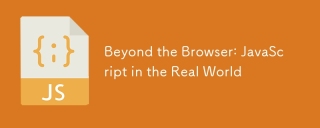
JavaScript在現實世界中的應用包括服務器端編程、移動應用開發和物聯網控制:1.通過Node.js實現服務器端編程,適用於高並發請求處理。 2.通過ReactNative進行移動應用開發,支持跨平台部署。 3.通過Johnny-Five庫用於物聯網設備控制,適用於硬件交互。
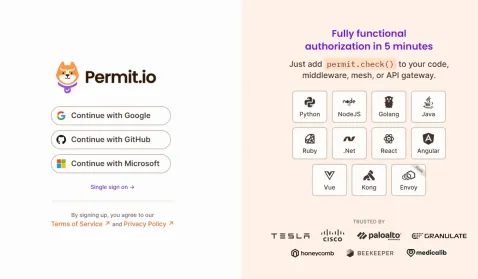
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
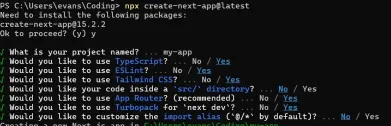
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
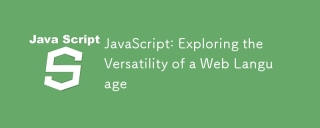
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
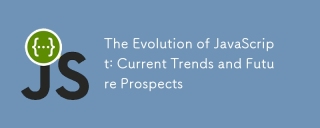
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
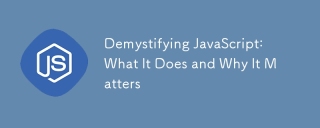
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
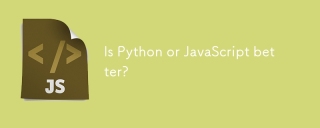
Python更适合数据科学和机器学习,JavaScript更适合前端和全栈开发。1.Python以简洁语法和丰富库生态著称,适用于数据分析和Web开发。2.JavaScript是前端开发核心,Node.js支持服务器端编程,适用于全栈开发。
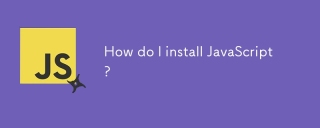
JavaScript不需要安裝,因為它已內置於現代瀏覽器中。你只需文本編輯器和瀏覽器即可開始使用。 1)在瀏覽器環境中,通過標籤嵌入HTML文件中運行。 2)在Node.js環境中,下載並安裝Node.js後,通過命令行運行JavaScript文件。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

MinGW - Minimalist GNU for Windows
這個專案正在遷移到osdn.net/projects/mingw的過程中,你可以繼續在那裡關注我們。 MinGW:GNU編譯器集合(GCC)的本機Windows移植版本,可自由分發的導入函式庫和用於建置本機Windows應用程式的頭檔;包括對MSVC執行時間的擴展,以支援C99功能。 MinGW的所有軟體都可以在64位元Windows平台上運作。

SublimeText3 Linux新版
SublimeText3 Linux最新版

DVWA
Damn Vulnerable Web App (DVWA) 是一個PHP/MySQL的Web應用程序,非常容易受到攻擊。它的主要目標是成為安全專業人員在合法環境中測試自己的技能和工具的輔助工具,幫助Web開發人員更好地理解保護網路應用程式的過程,並幫助教師/學生在課堂環境中教授/學習Web應用程式安全性。 DVWA的目標是透過簡單直接的介面練習一些最常見的Web漏洞,難度各不相同。請注意,該軟體中

Atom編輯器mac版下載
最受歡迎的的開源編輯器

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。