在我的工作場所,我的任務是為內部本地開發工作創建一個模擬聊天商店,在這樣做的同時,我做了一些關於Vue 的筆記(我有一些經驗,但沒有hooks),所以這只是我的黑曜石筆記,希望對你有用:)
目錄
- 參考與反應參考
- 觀看與反應
- Pinia 商店整合
- 實際範例
- 最佳實踐
- 常見問題
參考文獻與反應參考文獻
什麼是參考?
ref 是 Vue 使原始值具有響應性的方式。它將值包裝在帶有 .value 屬性的響應式物件中。
import { ref } from 'vue' // Inside Pinia Store export const useMyStore = defineStore('my-store', () => { // Creates a reactive reference const count = ref<number>(0) // To access or modify: function increment() { count.value++ // Need .value for refs } return { count, // When exposed, components can use it without .value increment } }) </number>
商店中的參考文獻類型
// Simple ref const isLoading = ref<boolean>(false) // Array ref const messages = ref<message>([]) // Complex object ref const currentUser = ref<user null>(null) // Ref with undefined const selectedId = ref<string undefined>(undefined) </string></user></message></boolean>
觀察和反應
手錶的基本使用
import { watch, ref } from 'vue' export const useMyStore = defineStore('my-store', () => { const messages = ref<message>([]) // Simple watch watch(messages, (newMessages, oldMessages) => { console.log('Messages changed:', newMessages) }) }) </message>
觀看選項
// Immediate execution watch(messages, (newMessages) => { // This runs immediately and on changes }, { immediate: true }) // Deep watching watch(messages, (newMessages) => { // Detects deep object changes }, { deep: true }) // Multiple sources watch( [messages, selectedId], ([newMessages, newId], [oldMessages, oldId]) => { // Triggers when either changes } )
Pinia 商店集成
帶有引用的儲存結構
export const useMyStore = defineStore('my-store', () => { // State const items = ref<item>([]) const isLoading = ref(false) const error = ref<error null>(null) // Computed const itemCount = computed(() => items.value.length) // Actions const fetchItems = async () => { isLoading.value = true try { items.value = await api.getItems() } catch (e) { error.value = e as Error } finally { isLoading.value = false } } return { items, isLoading, error, itemCount, fetchItems } }) </error></item>
組合商店
export const useMainStore = defineStore('main-store', () => { // Using another store const otherStore = useOtherStore() // Watching other store's state watch( () => otherStore.someState, (newValue) => { // React to other store's changes } ) })
實際例子
自動刷新實現
export const useChatStore = defineStore('chat-store', () => { const messages = ref<message>([]) const refreshInterval = ref<number null>(null) const isRefreshing = ref(false) // Watch for auto-refresh state watch(isRefreshing, (shouldRefresh) => { if (shouldRefresh) { startAutoRefresh() } else { stopAutoRefresh() } }) const startAutoRefresh = () => { refreshInterval.value = window.setInterval(() => { fetchNewMessages() }, 5000) } const stopAutoRefresh = () => { if (refreshInterval.value) { clearInterval(refreshInterval.value) refreshInterval.value = null } } return { messages, isRefreshing, startAutoRefresh, stopAutoRefresh } }) </number></message>
載入狀態管理
export const useDataStore = defineStore('data-store', () => { const data = ref<data>([]) const isLoading = ref(false) const error = ref<error null>(null) // Watch loading state for side effects watch(isLoading, (loading) => { if (loading) { // Show loading indicator } else { // Hide loading indicator } }) // Watch for errors watch(error, (newError) => { if (newError) { // Handle error (show notification, etc.) } }) }) </error></data>
最佳實踐
1. 參考初始化
// ❌ Bad const data = ref() // Type is 'any' // ✅ Good const data = ref<string>([]) // Explicitly typed </string>
2. 觀察清理
// ❌ Bad - No cleanup watch(source, () => { const timer = setInterval(() => {}, 1000) }) // ✅ Good - With cleanup watch(source, () => { const timer = setInterval(() => {}, 1000) return () => clearInterval(timer) // Cleanup function })
3. 計算與觀看
// ❌ Bad - Using watch for derived state watch(items, (newItems) => { itemCount.value = newItems.length }) // ✅ Good - Using computed for derived state const itemCount = computed(() => items.value.length)
4. 店鋪組織
// ✅ Good store organization export const useStore = defineStore('store', () => { // State refs const data = ref<data>([]) const isLoading = ref(false) // Computed properties const isEmpty = computed(() => data.value.length === 0) // Watchers watch(data, () => { // Handle data changes }) // Actions const fetchData = async () => { // Implementation } // Return public interface return { data, isLoading, isEmpty, fetchData } }) </data>
常見問題
- 忘記.value
// ❌ Bad const count = ref(0) count++ // Won't work // ✅ Good count.value++
- 觀看時間
// ❌ Bad - Might miss initial state watch(source, () => {}) // ✅ Good - Catches initial state watch(source, () => {}, { immediate: true })
- 記憶體洩漏
// ❌ Bad - No cleanup const store = useStore() setInterval(() => { store.refresh() }, 1000) // ✅ Good - With cleanup const intervalId = setInterval(() => { store.refresh() }, 1000) onBeforeUnmount(() => clearInterval(intervalId))
記住:在 Pinia 商店
與裁判和手錶合作時,請始終考慮清理、類型安全和適當的組織
以上是了解 Vue 與 Pinia Store 的反應性的詳細內容。更多資訊請關注PHP中文網其他相關文章!
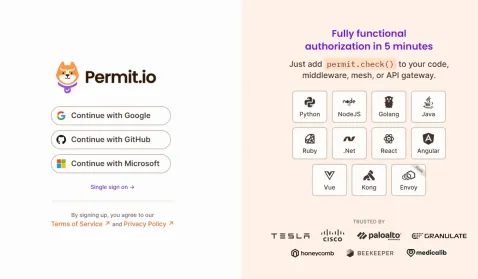
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
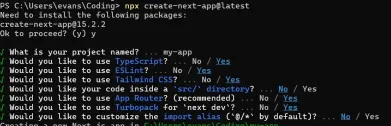
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
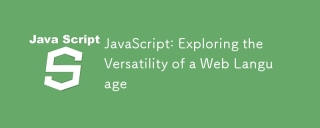
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
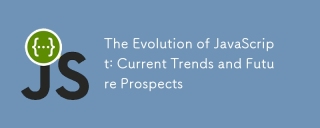
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
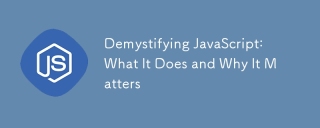
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
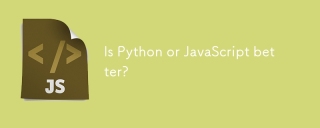
Python更适合数据科学和机器学习,JavaScript更适合前端和全栈开发。1.Python以简洁语法和丰富库生态著称,适用于数据分析和Web开发。2.JavaScript是前端开发核心,Node.js支持服务器端编程,适用于全栈开发。
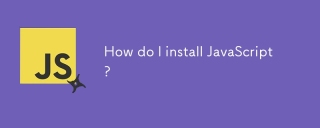
JavaScript不需要安裝,因為它已內置於現代瀏覽器中。你只需文本編輯器和瀏覽器即可開始使用。 1)在瀏覽器環境中,通過標籤嵌入HTML文件中運行。 2)在Node.js環境中,下載並安裝Node.js後,通過命令行運行JavaScript文件。
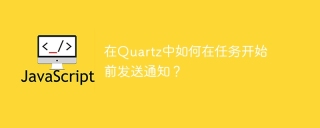
如何在Quartz中提前發送任務通知在使用Quartz定時器進行任務調度時,任務的執行時間是由cron表達式設定的。現�...


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

Atom編輯器mac版下載
最受歡迎的的開源編輯器

mPDF
mPDF是一個PHP庫,可以從UTF-8編碼的HTML產生PDF檔案。原作者Ian Back編寫mPDF以從他的網站上「即時」輸出PDF文件,並處理不同的語言。與原始腳本如HTML2FPDF相比,它的速度較慢,並且在使用Unicode字體時產生的檔案較大,但支援CSS樣式等,並進行了大量增強。支援幾乎所有語言,包括RTL(阿拉伯語和希伯來語)和CJK(中日韓)。支援嵌套的區塊級元素(如P、DIV),

MantisBT
Mantis是一個易於部署的基於Web的缺陷追蹤工具,用於幫助產品缺陷追蹤。它需要PHP、MySQL和一個Web伺服器。請查看我們的演示和託管服務。
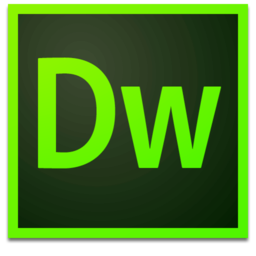
Dreamweaver Mac版
視覺化網頁開發工具

記事本++7.3.1
好用且免費的程式碼編輯器