我的程式碼可以混合蘋果和橘子嗎?
在您的 C 專案中,您使用大量「使用」來闡明變數的預期用途,主要用於 std::string 識別符,例如 PortalId 或 CakeId。您目前的方法允許以下操作:
using PortalId = std::string; using CakeId = std::string; PortalId portal_id("2"); CakeId cake_id("is a lie"); portal_id = cake_id; // OK
但這種靈活性是不可取的。您尋求編譯時類型檢查以防止混合不同的物件類型,同時保留原始物件的功能。
C 可以滿足此要求嗎?
是的,C 提供了解決方案為了你的困境。使用 C 17 和一些巧妙的編碼,可以建立一個防止混合類型的強型別識別碼。結果看起來像這樣:
SAFE_TYPEDEF(std::string, PortalId); SAFE_TYPEDEF(std::string, CakeId); int main() { PortalId portal_id("2"); CakeId cake_id("is a lie"); std::map<cakeid portalid> p_to_cake; // OK p_to_cake[cake_id] = portal_id; // OK p_to_cake[portal_id] = cake_id; // COMPILER ERROR portal_id = cake_id; // COMPILER ERROR portal_id = "1.0"; // COMPILER ERROR portal_id = PortalId("42"); // OK return 0; }</cakeid>
在此程式碼中,不允許不同類型之間的賦值,向容器添加不相容的類型將導致編譯器錯誤。
什麼秘密?
魔法在於 SAFE_TYPEDEF 巨集。這是滿足您所有要求的更新版本:
#include <iostream> #include <string> #include <map> #include <unordered_map> // define some tags to create uniqueness struct portal_tag {}; struct cake_tag {}; // a string-like identifier that is typed on a tag type template<class tag> struct string_id { using tag_type = Tag; // needs to be default-constuctable because of use in map[] below string_id(std::string s) : _value(std::move(s)) {} string_id() : _value() {} // provide access to the underlying string value const std::string& value() const { return _value; } private: std::string _value; // will only compare against same type of id. friend bool operator const std::string& { return r._value; } friend auto operator std::ostream& { return os ()(sid._value); return seed; } }; // let's make it hashable namespace std { template<class tag> struct hash<string_id>> { using argument_type = string_id<tag>; using result_type = std::size_t; result_type operator()(const argument_type& arg) const { return hash_code(arg); } }; } // create some type aliases for ease of use using PortalId = string_id<portal_tag>; using CakeId = string_id<cake_tag>; using namespace std;</cake_tag></portal_tag></tag></string_id></class></class></unordered_map></map></string></iostream>
此程式碼定義了一個 string_id 類,用作強類型識別碼。它被標記為portal_tag 或cake_tag 以確保唯一性。該類別也重載了諸如
透過此實現,您可以強制執行強類型並防止在您的程式碼中混合類型。代碼。它涵蓋了您問題中提到的所有場景,並為您的需求提供了穩定的解決方案。
以上是C 可以幫助我防止在程式碼中混合蘋果和橘子嗎?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
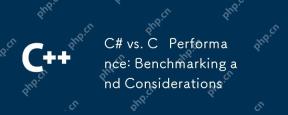
C#和C 在性能上的差異主要體現在執行速度和資源管理上:1)C 在數值計算和字符串操作上通常表現更好,因為它更接近硬件,沒有垃圾回收等額外開銷;2)C#在多線程編程上更為簡潔,但性能略遜於C ;3)選擇哪種語言應根據項目需求和團隊技術棧決定。
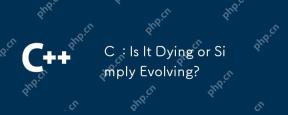
1)c relevantduetoItsAverity and效率和效果臨界。 2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
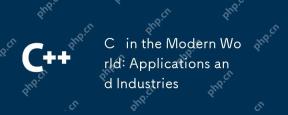
C 在現代世界中的應用廣泛且重要。 1)在遊戲開發中,C 因其高性能和多態性被廣泛使用,如UnrealEngine和Unity。 2)在金融交易系統中,C 的低延遲和高吞吐量使其成為首選,適用於高頻交易和實時數據分析。
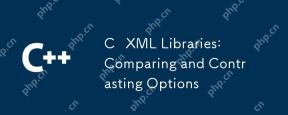
C 中有四種常用的XML庫:TinyXML-2、PugiXML、Xerces-C 和RapidXML。 1.TinyXML-2適合資源有限的環境,輕量但功能有限。 2.PugiXML快速且支持XPath查詢,適用於復雜XML結構。 3.Xerces-C 功能強大,支持DOM和SAX解析,適用於復雜處理。 4.RapidXML專注於性能,解析速度極快,但不支持XPath查詢。
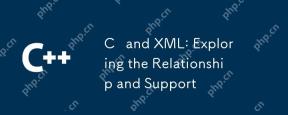
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
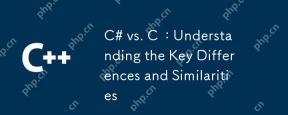
C#和C 的主要區別在於語法、性能和應用場景。 1)C#語法更簡潔,支持垃圾回收,適用於.NET框架開發。 2)C 性能更高,需手動管理內存,常用於系統編程和遊戲開發。
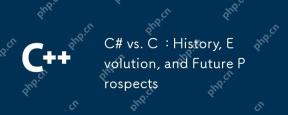
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
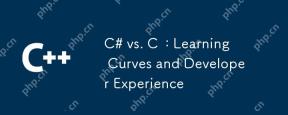
C#和C 的学习曲线和开发者体验有显著差异。1)C#的学习曲线较平缓,适合快速开发和企业级应用。2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具
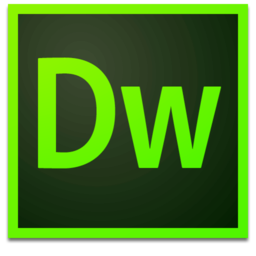
Dreamweaver Mac版
視覺化網頁開發工具
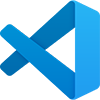
VSCode Windows 64位元 下載
微軟推出的免費、功能強大的一款IDE編輯器
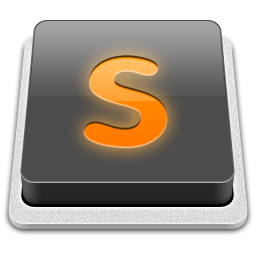
SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

Safe Exam Browser
Safe Exam Browser是一個安全的瀏覽器環境,安全地進行線上考試。該軟體將任何電腦變成一個安全的工作站。它控制對任何實用工具的訪問,並防止學生使用未經授權的資源。
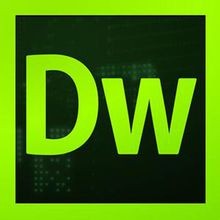
Dreamweaver CS6
視覺化網頁開發工具