C 中指向動態分配物件的指標向量中的記憶體管理
向量是C 中強大的資料結構,允許高效儲存和檢索元素。然而,在使用向量時注意記憶體管理以避免潛在的洩漏和錯誤至關重要。需要考慮的一個特定場景是在向量中儲存指向動態分配物件的指標。
內存洩漏預防
當使用指向對象的指針向量時,重要的是請記住,向量將管理指針本身的內存,而不是它們指向的對象。這意味著當向量超出範圍時,它只會釋放指針,而不是它們引用的物件。因此,如果我們不採取適當的預防措施,這可能會導致記憶體洩漏。
考慮以下範例:
#include <vector> struct Enemy { // ... }; std::vector<enemy> enemies;</enemy></vector>
在此範例中,我們有一個向量敵人,用於儲存指向 Enemy 物件的指標。我們動態分配每個Enemy 物件並將其推入向量中:
for (unsigned i = 0; i <p><strong>釋放指針,丟失物件</strong></p><p>當向量敵人超出範圍時,它將釋放它所包含的指標。但是,這些指標指向的物件不會被釋放,從而導致記憶體洩漏。 </p><p><strong>解決方案:明確刪除物件</strong></p><p>為了防止記憶體洩漏,我們需要以確保在向量超出範圍之前刪除 Enemy 物件。我們可以透過在銷毀向量之前手動刪除每個物件來實現這一點:</p><pre class="brush:php;toolbar:false">for (auto enemy : enemies) delete enemy; enemies.clear();
但是,這種方法很容易出錯,並且需要額外的程式碼來處理刪除過程中可能發生的異常。
救援智慧指標
更強大且異常安全的解決方案是使用智慧指標來管理物件的記憶體。智慧型指標在超出作用域時會自動釋放它們指向的對象,從而消除記憶體洩漏的風險。
C 標準函式庫提供兩種類型的智慧型指標:std::unique_ptr 和 std::shared_ptr。
- std::unique_ptr: 表示物件的唯一所有權。當 std::unique_ptr 超出範圍時,它會自動刪除它指向的物件。
- std::shared_ptr: 表示物件的共享所有權。多個 std::shared_ptr 可以指向同一個對象,當最後一個 std::shared_ptr 超出範圍時,該對象將被刪除。
使用唯一指標
我們可以使用 std::unique_ptr 重寫之前的範例來管理 Enemy 物件:
#include <vector> struct Enemy { // ... }; std::vector<enemy> enemies;</enemy></vector>
在此範例中,每個 Enemy 物件現在都包裝在 std::unique_ptr 中。當向量敵人超出範圍時,std::unique_ptr 物件將自動釋放它們指向的 Enemy 對象,確保不會發生記憶體洩漏。
使用共用指標
std::shared_ptr 適用於需要在向量中儲存多個共用物件的情況。下面的範例示範了使用std::shared_ptr:
for (unsigned i = 0; i <p>std::unique_ptr 和std::shared_ptr 都提供了可靠且異常安全的方法來管理動態分配物件的內存,確保潛在的內存洩漏並避免錯誤。 </p><p><strong>替代方案向量</strong></p><p>雖然向量通常是儲存物件指標的合適選擇,但也有專門處理指標管理的替代容器。其中一個容器是 boost::ptr_vector,它在超出範圍時自動刪除其內容。 </p><p><strong>結論</strong></p><p>使用指標向量動態分配物件時,必須考慮對記憶體管理的影響。透過理解向量的行為並採用適當的技術(例如智慧指標或替代容器),我們可以有效地避免記憶體洩漏並確保健壯且無錯誤的程式碼。 </p>
以上是在 C 中使用動態分配物件的指標向量時如何管理記憶體?的詳細內容。更多資訊請關注PHP中文網其他相關文章!
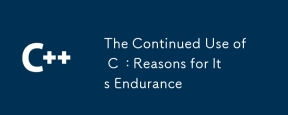
C 持續使用的理由包括其高性能、廣泛應用和不斷演進的特性。 1)高效性能:通過直接操作內存和硬件,C 在系統編程和高性能計算中表現出色。 2)廣泛應用:在遊戲開發、嵌入式系統等領域大放異彩。 3)不斷演進:自1983年發布以來,C 持續增加新特性,保持其競爭力。
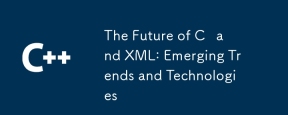
C 和XML的未來發展趨勢分別為:1)C 將通過C 20和C 23標準引入模塊、概念和協程等新特性,提升編程效率和安全性;2)XML將繼續在數據交換和配置文件中佔據重要地位,但會面臨JSON和YAML的挑戰,並朝著更簡潔和易解析的方向發展,如XMLSchema1.1和XPath3.1的改進。
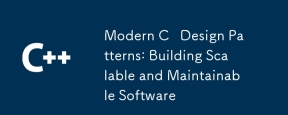
現代C 設計模式利用C 11及以後的新特性實現,幫助構建更靈活、高效的軟件。 1)使用lambda表達式和std::function簡化觀察者模式。 2)通過移動語義和完美轉發優化性能。 3)智能指針確保類型安全和資源管理。
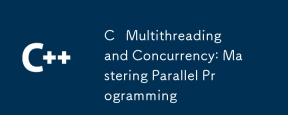
C 多線程和並發編程的核心概念包括線程的創建與管理、同步與互斥、條件變量、線程池、異步編程、常見錯誤與調試技巧以及性能優化與最佳實踐。 1)創建線程使用std::thread類,示例展示瞭如何創建並等待線程完成。 2)同步與互斥使用std::mutex和std::lock_guard保護共享資源,避免數據競爭。 3)條件變量通過std::condition_variable實現線程間的通信和同步。 4)線程池示例展示瞭如何使用ThreadPool類並行處理任務,提高效率。 5)異步編程使用std::as
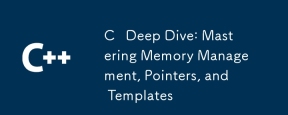
C 的內存管理、指針和模板是核心特性。 1.內存管理通過new和delete手動分配和釋放內存,需注意堆和棧的區別。 2.指針允許直接操作內存地址,使用需謹慎,智能指針可簡化管理。 3.模板實現泛型編程,提高代碼重用性和靈活性,需理解類型推導和特化。
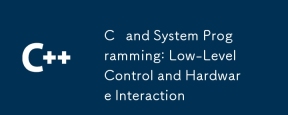
C 適合系統編程和硬件交互,因為它提供了接近硬件的控制能力和麵向對象編程的強大特性。 1)C 通過指針、內存管理和位操作等低級特性,實現高效的系統級操作。 2)硬件交互通過設備驅動程序實現,C 可以編寫這些驅動程序,處理與硬件設備的通信。
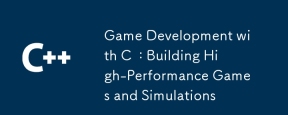
C 適合構建高性能遊戲和仿真係統,因為它提供接近硬件的控制和高效性能。 1)內存管理:手動控制減少碎片,提高性能。 2)編譯時優化:內聯函數和循環展開提昇運行速度。 3)低級操作:直接訪問硬件,優化圖形和物理計算。
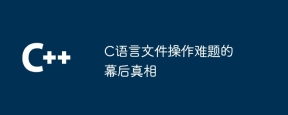
文件操作難題的真相:文件打開失敗:權限不足、路徑錯誤、文件被佔用。數據寫入失敗:緩衝區已滿、文件不可寫、磁盤空間不足。其他常見問題:文件遍歷緩慢、文本文件編碼不正確、二進製文件讀取錯誤。


熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

禪工作室 13.0.1
強大的PHP整合開發環境

Atom編輯器mac版下載
最受歡迎的的開源編輯器
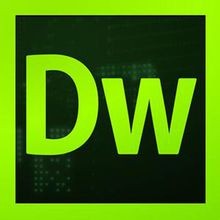
Dreamweaver CS6
視覺化網頁開發工具
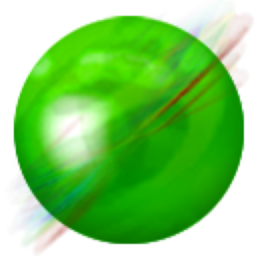
ZendStudio 13.5.1 Mac
強大的PHP整合開發環境
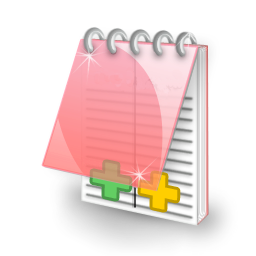
EditPlus 中文破解版
體積小,語法高亮,不支援程式碼提示功能